Getting started with Arcjet- Bot detection, Rate limiting etc
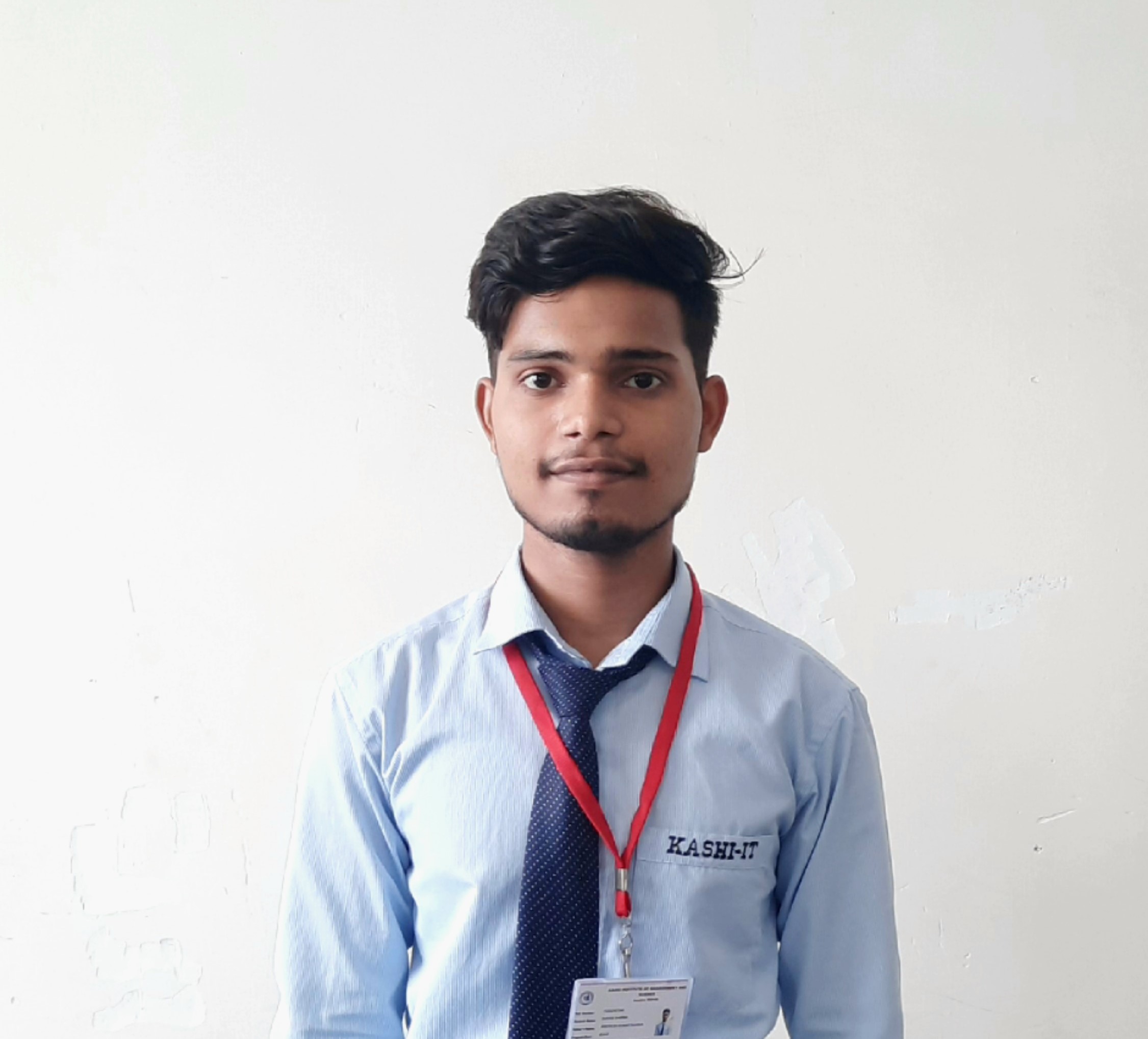
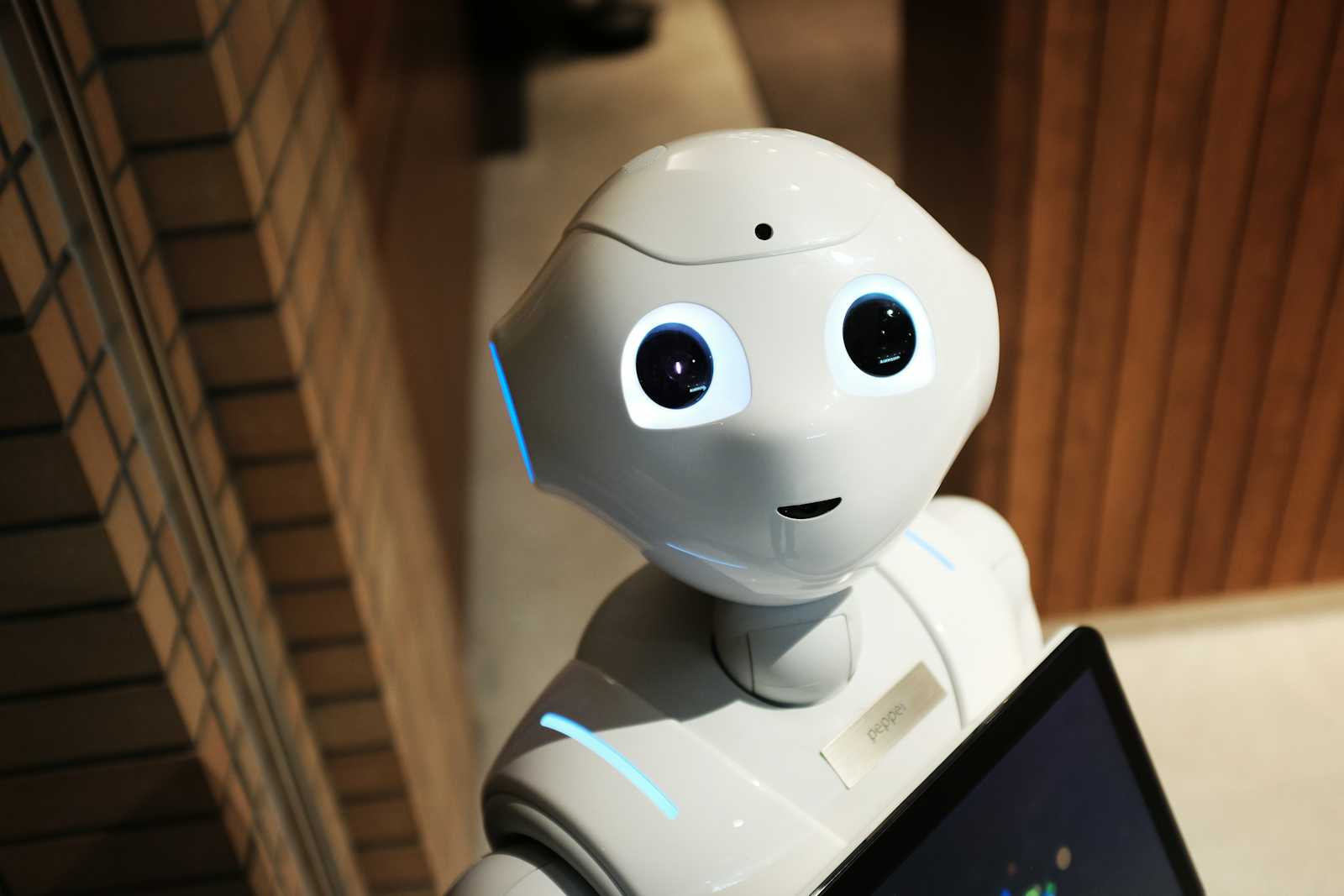
Arcjet helps developers protect their apps in just a few lines of code. Bot detection. Rate limiting. Email validation. Attack protection. Data redaction. A developer-first approach to security.
Get Started with Node.js + Express.js
To follow this tutorial, you need a Node.js 20+ application. For example, the following basic Express server will be enough for this tutorial: This exposes a single /api/hello
endpoint that returns ”Hello, World!”
import express from 'express'
const app = express();
const PORT = 3000;
app.get('/api/hello', (req, res) => {
res.status(200).send("Hello");
)}
app.listen(PORT, () => {
console.log(`Application is running on ${PORT}`);
)}
Set your key
Create a free Arcjet account then follow the instructions to add a site and get a key.
Add your key to a .env
file in your project root. Since Node.js doesn’t set NODE_ENV
for you, you also need to set ARCJET_ENV
in your environment file. This allows Arcjet to accept a local IP address for development purposes.
# NODE_ENV is not set automatically, so tell Arcjet we're in dev
# You can leave this unset in prod
ARCJET_ENV=development
# Get your site key from https://app.arcjet.com
ARCJET_KEY=ajkey_yourkey
3. Add rules
This configures Arcjet rules to protect your app from attacks, apply a rate limit, and prevent bots from accessing your app.
Create a arcjet.js
file because we want to keep it seprate for better code structure and install the package ` npm install @arcjet/node ` And then define the following rules.
import arcjet, { shield, detectBot, tokenBucket } from "@arcjet/node";
import "dotenv/config";
export const arcjetMiddleware = arcjet({
key: process.env.ARCJET_KEY,
characteristics: ["ip.src"], // Track requests by IP
rules: [
// Shield protects your app from common attacks e.g. SQL injection
shield({ mode: "LIVE" }),
detectBot({
mode: "LIVE", // Blocks requests. Use "DRY_RUN" to log only
// Block all bots except the following
allow: [
"CATEGORY:SEARCH_ENGINE", // Google, Bing, etc
// See the full list at https://arcjet.com/bot-list
],
}),
// Create a token bucket rate limit. Other algorithms are supported.
tokenBucket({
mode: "LIVE",
refillRate: 5,
interval: 10,
capacity: 10,
}),
],
});
Protect the Application
Now we have to apply this rule in our express application in order to protect. So as you remember that we already created an express application above.
import express from 'express'
const app = express();
import { arcjetMiddleware } from "./arcjet.js";
const PORT = 3000;
app.use(async (req, res, next) => {
try {
const decision = await arcjetMiddleware.protect(req, {
requested: 1,
});
if (decision.isDenied()) {
if (decision.reason.isRateLimit()) {
res.status(429).json({ error: "To many request" });
} else if (decision.reason.isBot()) {
res.status(403).json({ error: "Bot detection denied" });
} else {
res.status(403).json({ error: "Forbidden" });
}
return;
}
if (
decision.results.some(
(result) => result.reason.isBot && result.reason.isSpoofed()
)
) {
res.status(403).json({ error: "Spoofed bot detected" });
return;
}
next();
} catch (error) {
console.log("Arcjet err", error);
next(error);
}
});
app.get('/api/hello', (req, res) => {
res.status(200).send("Hello");
)}
app.listen(PORT, () => {
console.log(`Application is running on ${PORT}`);
)}
Subscribe to my newsletter
Read articles from Shivam Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
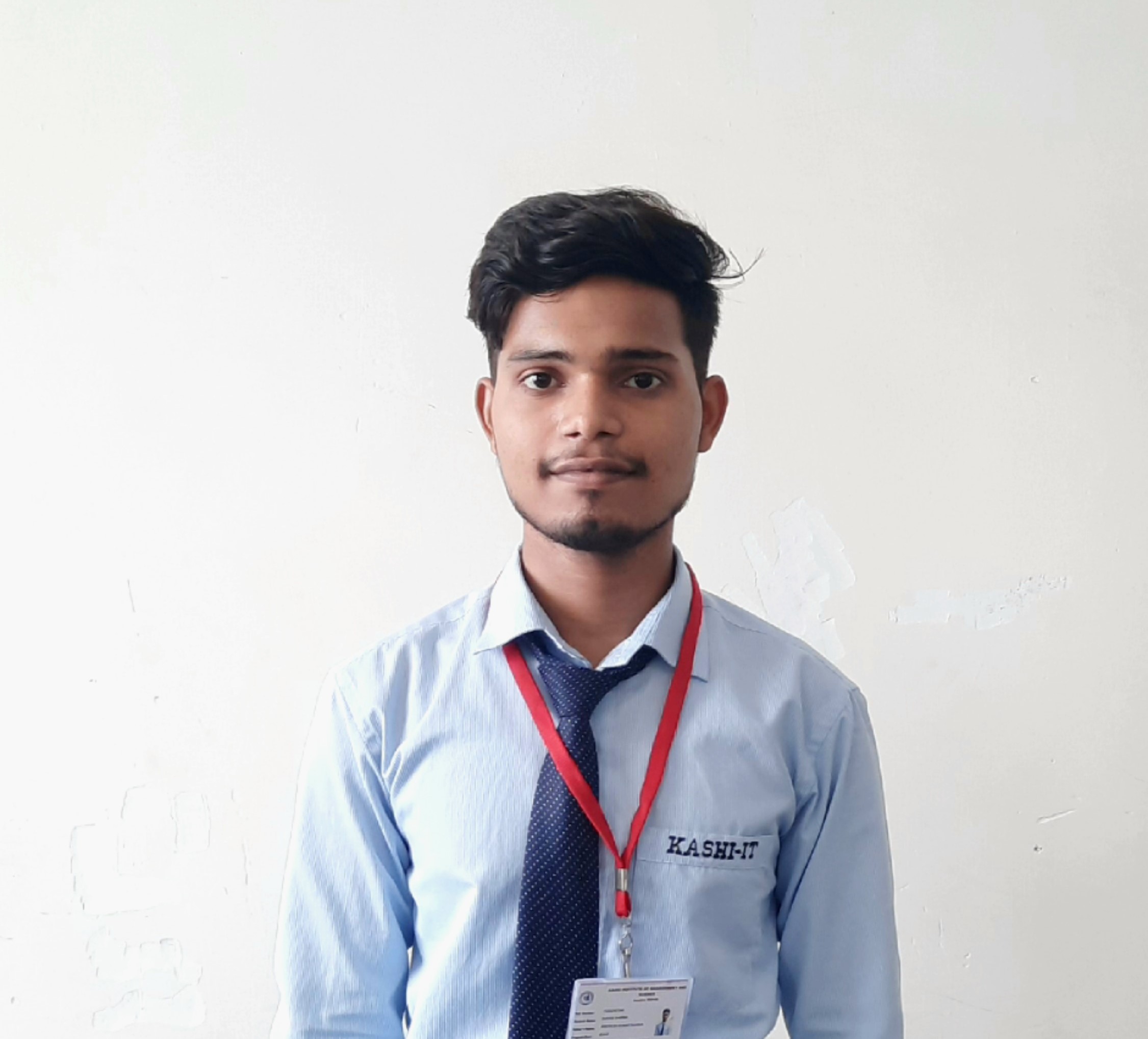
Shivam Sharma
Shivam Sharma
I’m an Open-Source enthusiast & Final year pursuing my Bachelors in Computer Science. I am passionate about Kubernetes, Web Development, DevOps & I enjoy learning new things.