Day 20 Journey: Understanding Binary Trees and Linked Lists

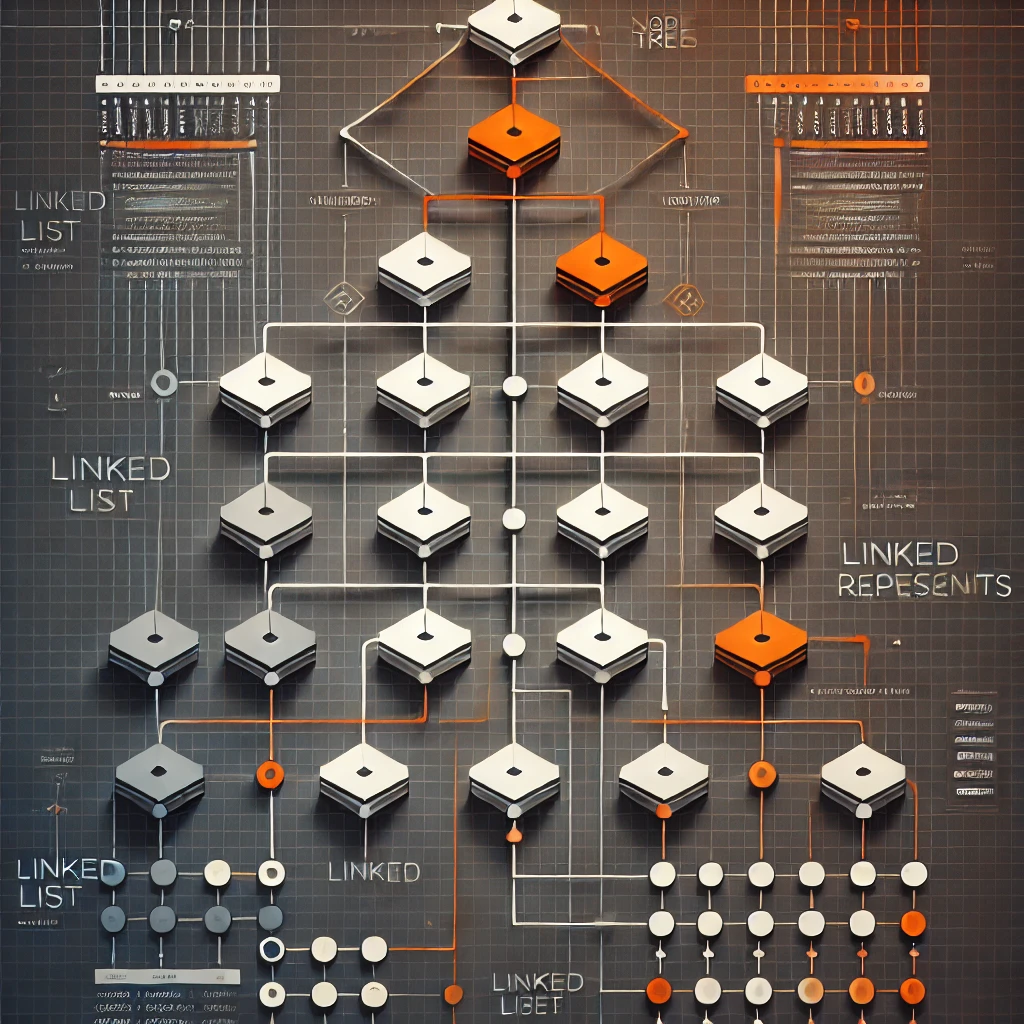
Today's session focused on Binary Trees, and I solved a few Linked List problems. While Linked Lists felt smooth, I need to strengthen my BFS, DFS, and tree traversal strategies.
๐ Problems Solved Today
1๏ธโฃ Invert Binary Tree (โ Easy)
๐ Problem: Given a binary tree, invert it (swap left and right children at every node).
๐น Approach: Used a recursive DFS approach to swap left and right subtrees at each node. An iterative BFS approach using a queue also works.
2๏ธโฃ Maximum Depth of Binary Tree (โ Easy)
๐ Problem: Find the maximum depth (height) of a binary tree.
๐น Approach: Used DFS recursion to calculate the depth of left and right subtrees and took the maximum. An iterative BFS approach using level-order traversal also works.
3๏ธโฃ Diameter of Binary Tree (โ Easy)
๐ Problem: Find the longest path between any two nodes in a binary tree.
๐น Approach: Used DFS recursion to compute the depth of subtrees and maintain a global variable to track the longest path.
4๏ธโฃ Balanced Binary Tree (โ Easy)
๐ Problem: Check if a binary tree is height-balanced (i.e., the depth of left and right subtrees never differs by more than 1).
๐น Approach: Used DFS recursion, calculating height and checking balance condition at each node.
5๏ธโฃ Same Tree (โ Easy)
๐ Problem: Given two binary trees, check if they are identical.
๐น Approach: Used DFS recursion to compare corresponding nodes of both trees.
6๏ธโฃ Subtree of Another Tree (โ Easy)
๐ Problem: Check if a given tree is a subtree of another tree.
๐น Approach: Used DFS recursion, checking if two trees are the same at any node and recursively searching the left and right subtrees.
7๏ธโฃ Lowest Common Ancestor of a Binary Search Tree (โ Medium)
๐ Problem: Given a BST and two nodes, find their lowest common ancestor.
๐น Approach: Used iterative BST traversal, moving left or right based on the values of the nodes.
8๏ธโฃ Binary Tree Level Order Traversal (โ Medium)
๐ Problem: Return a list of values for each level of the binary tree.
๐น Approach: Used BFS with a queue to traverse level by level.
๐ก Key Takeaways & Next Steps
โ
Trees & recursion feel intuitive but need to work on BFS/DFS more.
โ
Linked Lists were easier to work with but should focus on more complex problems.
โ
Next, Iโll work on graph traversal techniques and advanced Linked List-based algorithms.
๐ Plan for tomorrow:
๐น BFS & DFS in graphs
๐น More Linked List-based problems
๐น Some Medium/Hard tree problems
So far, so good! ๐ช Let's keep it going. ๐
Subscribe to my newsletter
Read articles from Tennis Kumar C directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
