Compile your programs faster with Makefile.
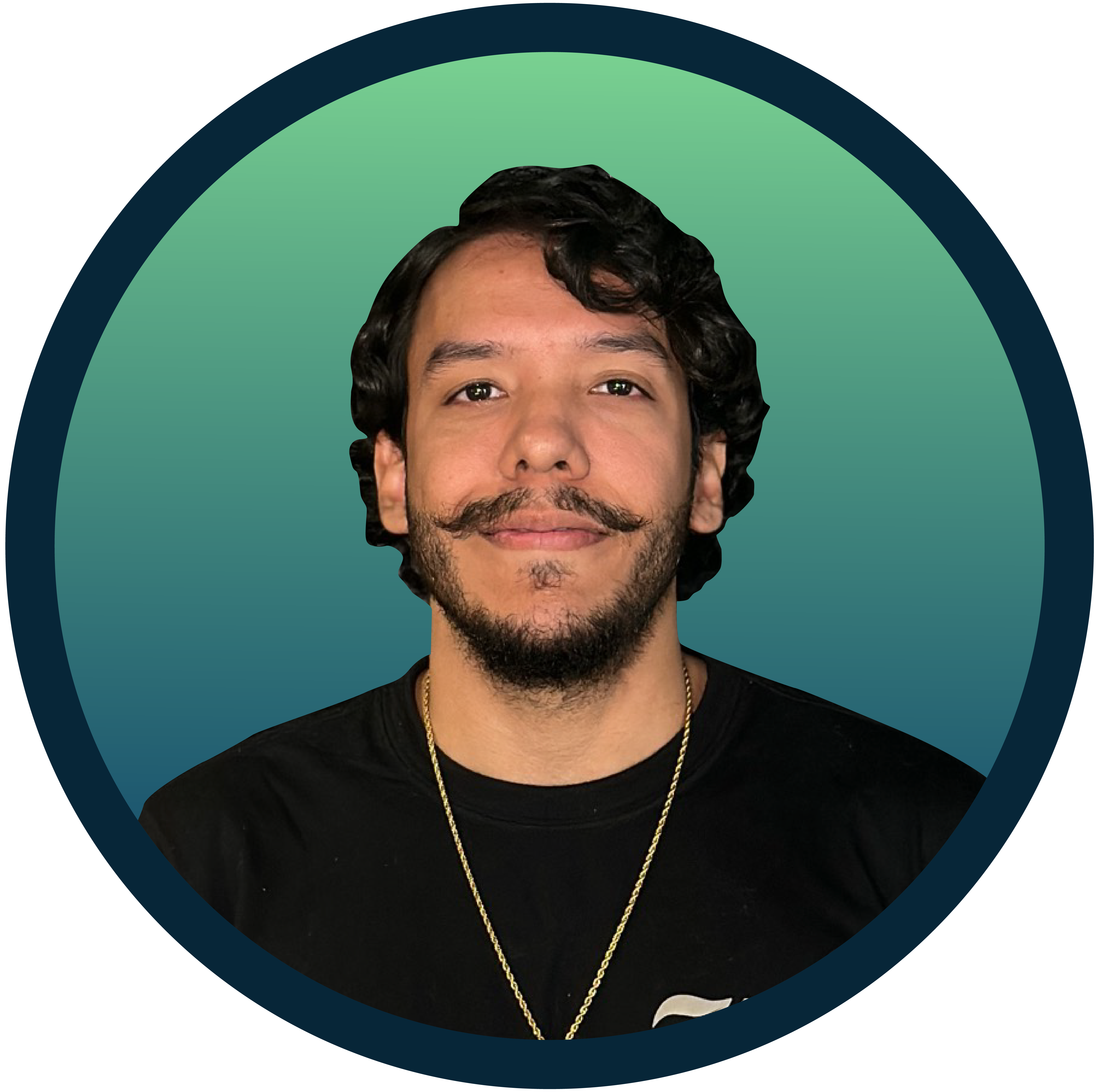
Table of contents
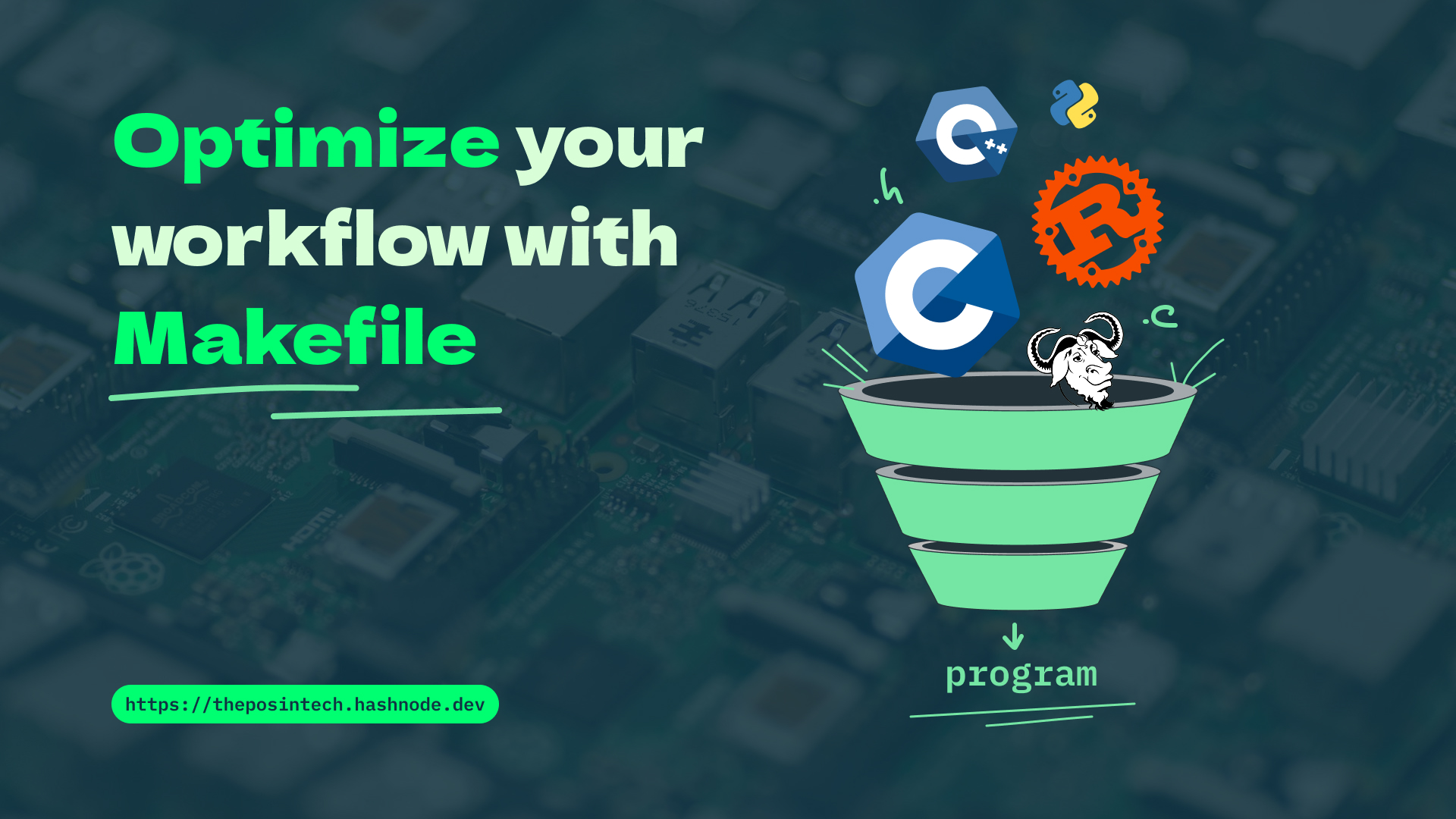
A Necessary Contrast
Before we talk about Makefiles, it's important to understand the difference between compiled and interpreted languages:
Interpreted languages (like Python or JavaScript) execute code line by line, without requiring a prior compilation step. You just write the code and run it directly with the interpreter.
Compiled languages (like C or C++) require an intermediate step: converting the source code into an executable file (machine language or binary) using a compiler.
And this is where the problem arises. In small projects, compiling a single file is easy, but in larger projects, doing it manually becomes unmanageable.
From personal experience, when I started developing in C and working on practice projects, compilation wasn't a problem. An example would be this basic program that shows the classic Hello, World!
message in the terminal:
#include <stdio.h>
int main(void)
{
printf("Hello World!\n");
return (0);
}
To compile it, all we would need to type in the terminal is:
cc main.c -o hello_world // <-- Compile
./hello_world // <-- Run the program
Hello World! // <-- Output
But what if we have multiple files in our project? Imagine we have the following structure:
proyect/
βββ main.c
βββ stack.c
βββ stack.h
If main.c
uses functions in stack.c
, we should compile it like this:
cc -c stack.c # Make stack.o
cc -c main.c # Make main.o
cc main.o stack.o -o program
This compilation process becomes tedious and error-prone in large projects. That's where Makefile comes in.
What is a Makefile?
A Makefile is a file where we can define certain rules to compile a program efficiently using the make
command.
A basic Makefile for our previous example might look like this:
main: main.c stack.o
cc -Wall -g -o main main.c stack.o
stack.o: stack.c stack.h
cc -Wall -c stack.c
But let's understand what each part of this does.
1. The Target
main: main.c stack.o
Here, we define a target, in this case, main
. When we run make main
, make
will look for a target with that name and check its dependencies.
main:
β Defines the target we want to build.main.c stack.o
β These are the files necessary to buildmain
(the dependencies).
π‘ This means that make
will only build main
if these files exist. If one is missing, it will try to generate it automatically.
2. The Command
This is the command that will run if we need to build main
:
cc -Wall -g -o main main.c stack.o
cc
β The compiler we're using (could becc
).-Wall
β Enables all common warnings.-g
β Includes debugging information.-o main
β Specifies the name of the generated executable.main.c stack.o
β These are the files being compiled to createmain
.
π‘ Important note: lines with commands must be indented with a tab, not spaces. If you use spaces, make
will throw an error.
3. The Rule
stack.o: stack.c stack.h
cc -Wall -c stack.c
Here, we tell make
how to build stack.o
.
stack.o:
β The target we want to generate.stack.c stack.h
β These are the dependencies needed to create it.
If stack.o
doesn't exist or if stack.c
has changed since the last compilation, make
will run this command:
cc -Wall -c stack.c
-c
β Indicates we want to compilestack.c
without generating an executable, just an intermediate object file (stack.o
).
π‘ This means that if stack.c
changes, make
will recompile only that file instead of recompiling the entire project, saving time.
What Happens When We Run make main
?
make
checks ifmain
exists and if itβs up to date.If
main
doesnβt exist or any of its files have changed, it checksmain.c
andstack.o
.If
stack.o
doesn't exist, it follows its rule and compilesstack.c
intostack.o
.Finally, it compiles
main.c
along withstack.o
to generatemain
.
π Rules for Deleting Files in Makefile
So far, we've seen that compilation generates many intermediate files (.o
files and executables). These files can clutter up our workspace and should be deleted before sharing the code. As weβve seen, deleting them manually every time we compile isnβt ideal.
Instead of doing it manually, we can define rules in the Makefile to do it automatically.
π 1. Defining the clean
Rule
.PHONY: clean
clean:
rm -rf *.o main
π‘ What does this do?
.PHONY: clean
- Indicates that
clean
is a command, not a file, to avoid confusion with any files that may have the same name.
- Indicates that
clean:
- Defines the cleaning task.
rm -rf *.o main
rm
β The Linux command to remove files.-r
β Removes directories recursively.-f
β Forces removal without confirmation.*.o
β Removes all object files (.o
).main
β Removes the executablemain
.
Now, when we run:
make clean
It will delete all compilation files, leaving only the source code.
Now that we know how to generate and delete all our files, we can improve our Makefile further by using variables. Letβs see how.
βοΈ Using Variables in Makefile
We can see that certain elements repeat often, like the compiler cc
or the compilation flags -Wall -g
. To avoid repeatedly writing this and make the Makefile cleaner and easier to modify, we can use variables.
Defining variables is easy. Normally, theyβre placed at the beginning of the file, before defining the compilation rules.
# Define variables
CC = cc
CFLAGS = -Wall -g
OBJS = main.o stack.o
main: main.c stack.o
$(CC) $(CFLAGS) -o main main.c stack.o
stack.o: stack.c stack.h
$(CC) $(CFLAGS) -c stack.c
.PHONY: clean
clean:
rm -rf *.o
Now, instead of writing the compiler directly, we use the variable. So, if for some reason we want to switch from cc
to gcc
, we just need to update the value of the CC
variable, and this change will be reflected in all the places where we use that variable.
Now, instead of writing all the commands manually, we can simply run:
make
Make will check if there are changes in stack.c
or main.c
, and it will only recompile the necessary files.
Alternatively, if we want to remove all object files:
make clean
With everything weβve learned so far, letβs see how a real-world projectβs Makefile
might look:
# FLAGS _____________________________
CC = cc
FLAGS = -Wall -Werror -Wextra -g3 -fsanitize=address
RM = rm -f
# CONFS _____________________________
SERVER_EXE = server
CLIENT_EXE = client
# SRCS ______________________________
SERVER_SRC = ./src/server.c
CLIENT_SRC = ./src/client.c
BANNERS_SRC = ./utils/misc/banners.c
LIBFT_DIR = ./utils/libft
PRINTF_DIR = ./utils/ft_printf
LIBFT = $(LIBFT_DIR)/libft.a
PRINTF = $(PRINTF_DIR)/libftprintf.a
# OBJS ______________________________
SERVER_OBJ = $(SERVER_SRC:.c=.o)
CLIENT_OBJ = $(CLIENT_SRC:.c=.o)
BANNER_OBJ = $(BANNERS_SRC:.c=.o)
all: $(SERVER_EXE) $(CLIENT_EXE) $(BANNER_OBJ)
$(BANNER_OBJ): $(BANNERS_SRC)
@$(CC) $(FLAGS) -c $(BANNERS_SRC) -o $(BANNER_OBJ)
$(SERVER_EXE): $(SERVER_OBJ) $(BANNER_OBJ) $(LIBFT) $(PRINTF)
@$(CC) $(FLAGS) $(SERVER_OBJ) $(BANNER_OBJ) $(LIBFT) $(PRINTF) -o $(SERVER_EXE)
@echo "β
$(SERVER_EXE) compiled success."
$(CLIENT_EXE): $(CLIENT_OBJ) $(BANNER_OBJ) $(LIBFT) $(PRINTF)
@$(CC) $(FLAGS) $(CLIENT_OBJ) $(BANNER_OBJ) $(LIBFT) $(PRINTF) -o $(CLIENT_EXE)
@echo "β
$(CLIENT_EXE) compiled success."
$(LIBFT):
@make -sC $(LIBFT_DIR)
$(PRINTF):
@make -sC $(PRINTF_DIR)
%.o: %.c
@$(CC) $(FLAGS) -c $< -o $@
clean:
@$(RM) $(SERVER_OBJ) $(CLIENT_OBJ) $(BANNER_OBJ)
@make -sC $(LIBFT_DIR) clean
@make -sC $(PRINTF_DIR) clean
@echo "All objects files cleaned"
fclean: clean
@$(RM) $(SERVER_EXE) $(CLIENT_EXE) $(LIBFT)
@make -sC $(LIBFT_DIR) fclean
@make -sC $(PRINTF_DIR) fclean
@echo "All executables and libraries cleaned"
re: fclean all
.PHONY: all clean fclean re
π― Conclusion: Why Use Makefile?
β
Automates compilation and avoids manually writing long commands.
β
Optimizes recompilations, only recompiles what's necessary.
β
Eases maintenance, preventing human errors.
β
It's a standard in C and C++ development, used in large projects.
If you're not using Makefiles in your projects yet, now is the perfect time to start! π
π₯ Want a template to use in your projects?
Here you can π download Makefile Template
Subscribe to my newsletter
Read articles from Cristian Zapata Arias directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
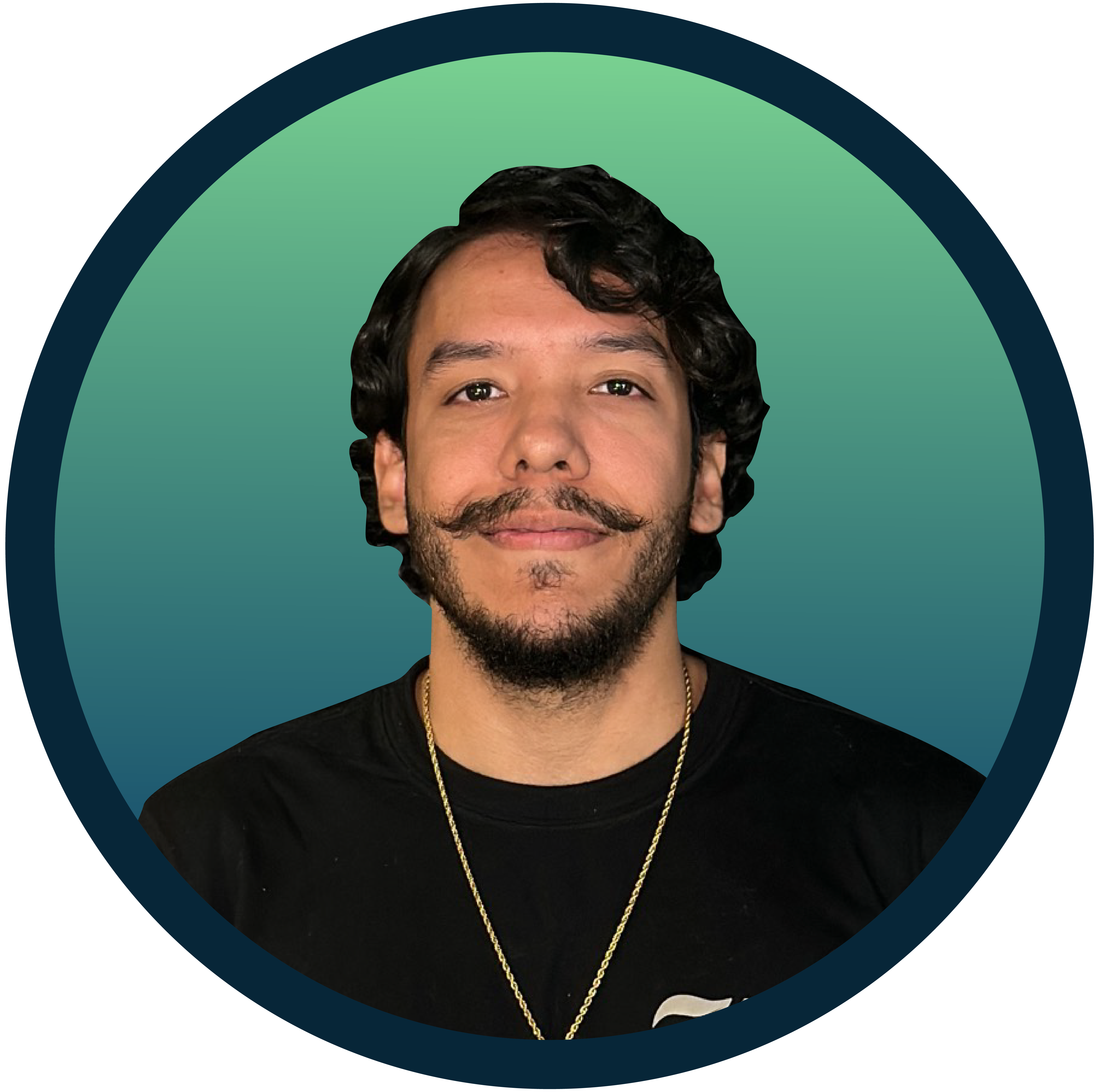
Cristian Zapata Arias
Cristian Zapata Arias
Hey there! Iβm Cristian Zapata π¨βπ» a creative mind shifting gears into the world of embedded systems. With a background in graphic design, visual arts, and front-end development, Iβm now diving into low-level programming, microcontrollers, and real-time operating systems. I love working close to the hardware, writing efficient C/C++ code, and bringing smart, connected devices to life using platforms like STM32 and ESP32. Whether it's debugging firmware or experimenting with new architectures, I'm all about learning, building, and pushing boundaries. Letβs connect and create the future of embedded tech together! β‘π§