๐ฅJavaScript Promises: A Complete Guide๐

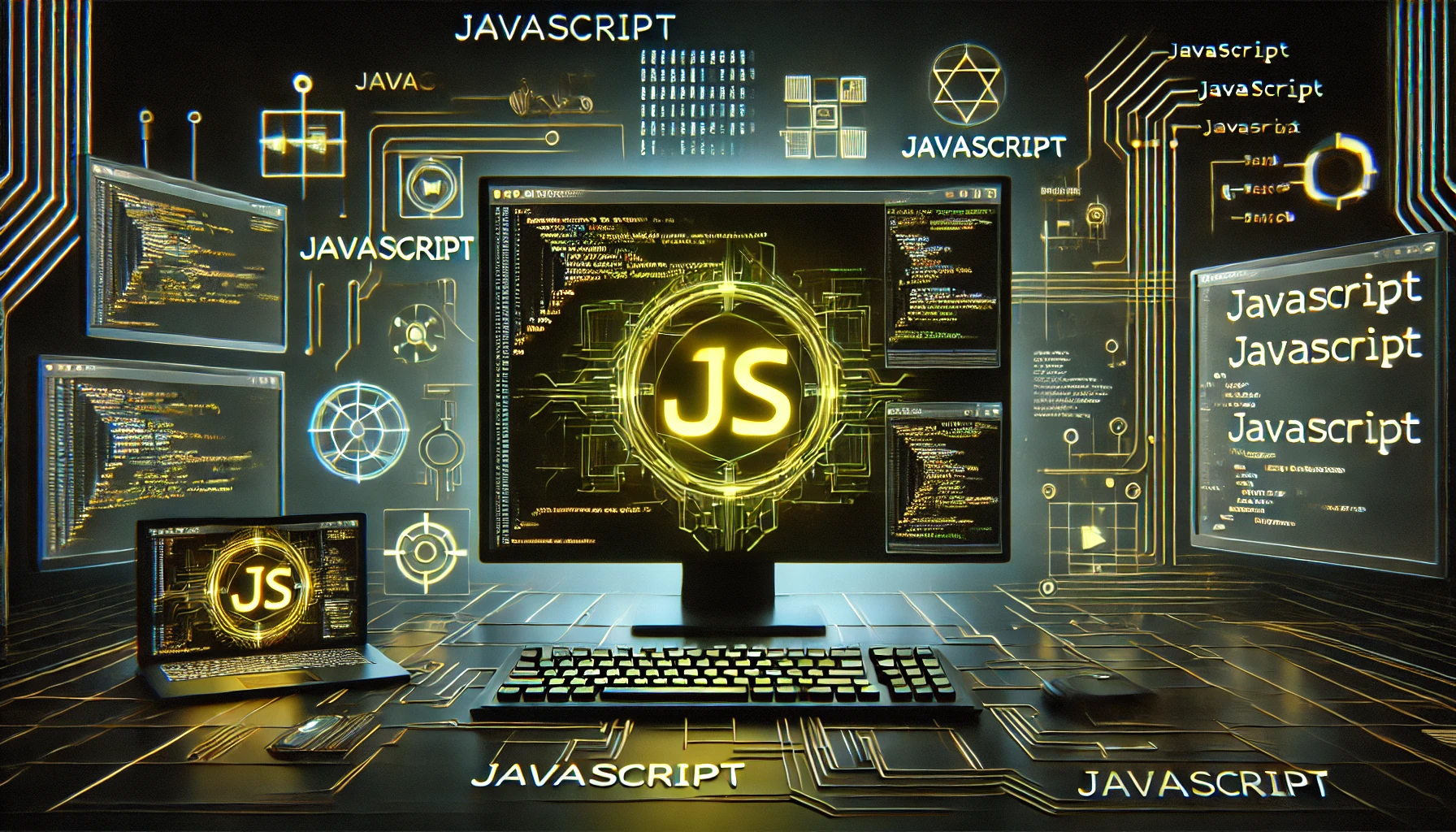
JavaScript is widely used for handling asynchronous operations, and Promises play a crucial role in making it easier. If youโve ever dealt with callbacks and found them messy, Promises are here to make your life easier! Letโs break it down in simple words. ๐
๐ What is a Promise?
A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation. It allows you to write cleaner and more manageable asynchronous code. ๐
A Promise can have three states:
Pending โ The operation is still in progress.
Fulfilled โ The operation completed successfully.
Rejected โ The operation failed.
๐ A Simple Example
let myPromise = new Promise((resolve, reject) => {
let success = true; // Simulating success or failure
setTimeout(() => {
if (success) {
resolve("Operation Successful! โ
");
} else {
reject("Operation Failed! โ");
}
}, 2000);
});
myPromise
.then(response => console.log(response))
.catch(error => console.log(error));
๐ง Explanation:
The
Promise
constructor takes a function with two parameters:resolve
andreject
.If the operation is successful,
resolve
is called; otherwise,reject
is called..then()
handles the success case..catch()
handles the failure case.
๐ก Why Use Promises?
โ
Avoid Callback Hell โ Makes code cleaner and more readable.
โ
Better Error Handling โ .catch()
makes it easy to handle errors.
โ
Chaining โ Allows you to execute multiple async operations in sequence.
๐ฏ Chaining Promises
One of the best features of Promises is chaining, where multiple .then()
statements run sequentially.
let fetchData = new Promise((resolve) => {
setTimeout(() => resolve("Data fetched!"), 1000);
});
fetchData
.then(response => {
console.log(response);
return "Processing data...";
})
.then(response => {
console.log(response);
return "Data processed successfully!";
})
.then(response => console.log(response))
.catch(error => console.log(error));
๐ง Explanation:
Each
.then()
returns a new value that is passed to the next.then()
.This avoids deeply nested callbacks, making the code cleaner.
๐ Using fetch()
with Promises
In real-world applications, Promises are widely used for API calls.
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log("Error fetching data: ", error));
This example fetches data from an API and logs the response.
โก Async/Await: A Cleaner Way to Handle Promises
ES6 introduced async/await
to simplify working with Promises.
async function fetchData() {
try {
let response = await fetch('https://jsonplaceholder.typicode.com/posts/1');
let data = await response.json();
console.log(data);
} catch (error) {
console.log("Error fetching data: ", error);
}
}
fetchData();
๐ง Explanation:
async
makes a function return a Promise.await
pauses execution until the Promise resolves.try...catch
handles errors elegantly.
๐ Conclusion
JavaScript Promises are essential for writing clean and efficient asynchronous code. They help manage async tasks smoothly and eliminate callback hell. Mastering Promises will improve your JavaScript skills significantly! โจ
If you have any questions, drop them in the comments! Happy coding! ๐๐
๐ Next Topic: JavaScript Async/Await โก
Stay tuned for our next blog, where we will explore JavaScript Async/Await in detail! ๐
Subscribe to my newsletter
Read articles from Vivek varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vivek varshney
Vivek varshney
Full-Stack Web Developer | Blogging About Tech, React.js, Angular, Node.js & Web Development. Turning Ideas into Code & Helping Businesses Grow!