First ABAP Program: Crafting Readable Reports with Comments, Formatting, and Lines (Part 2)
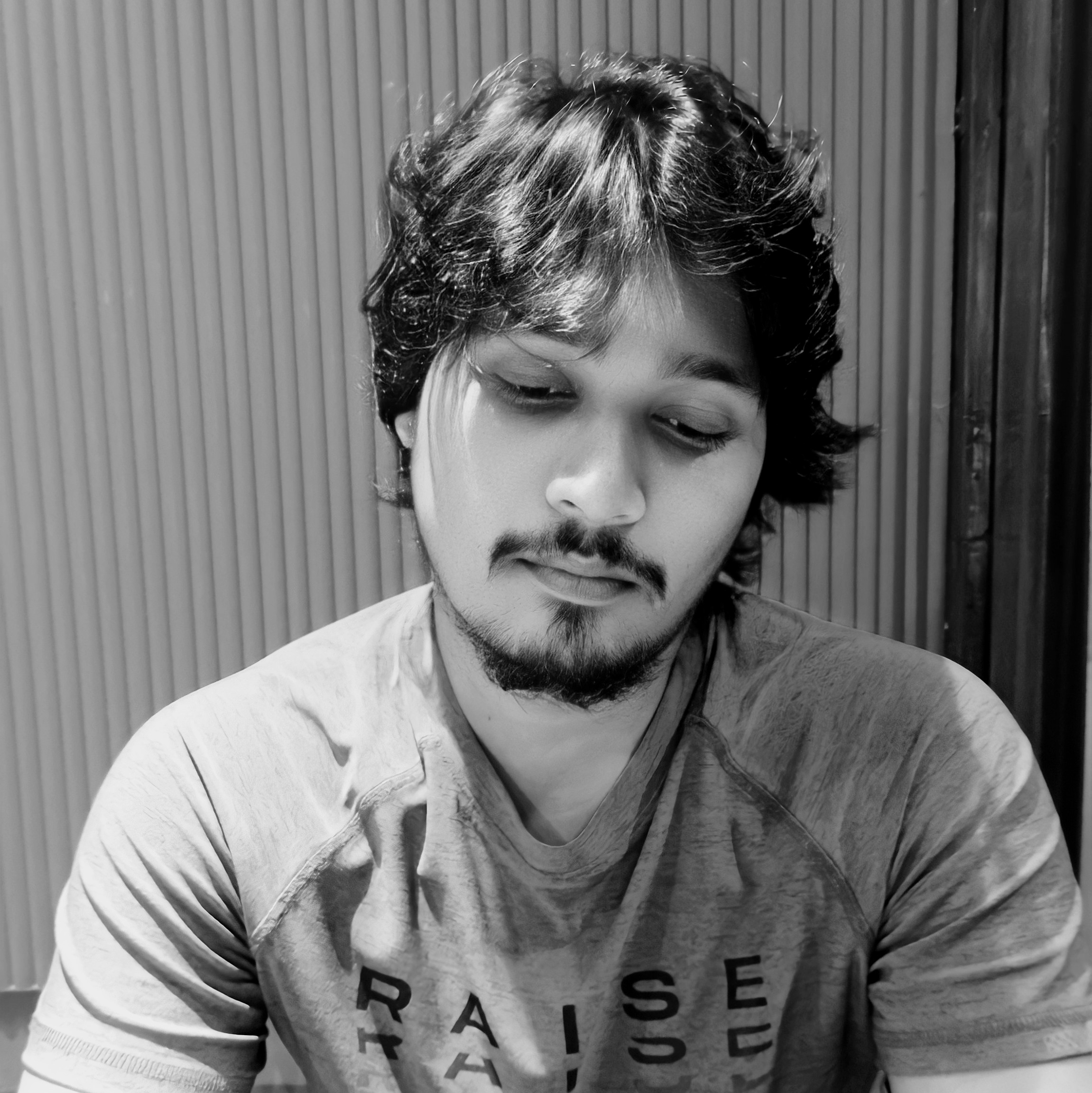
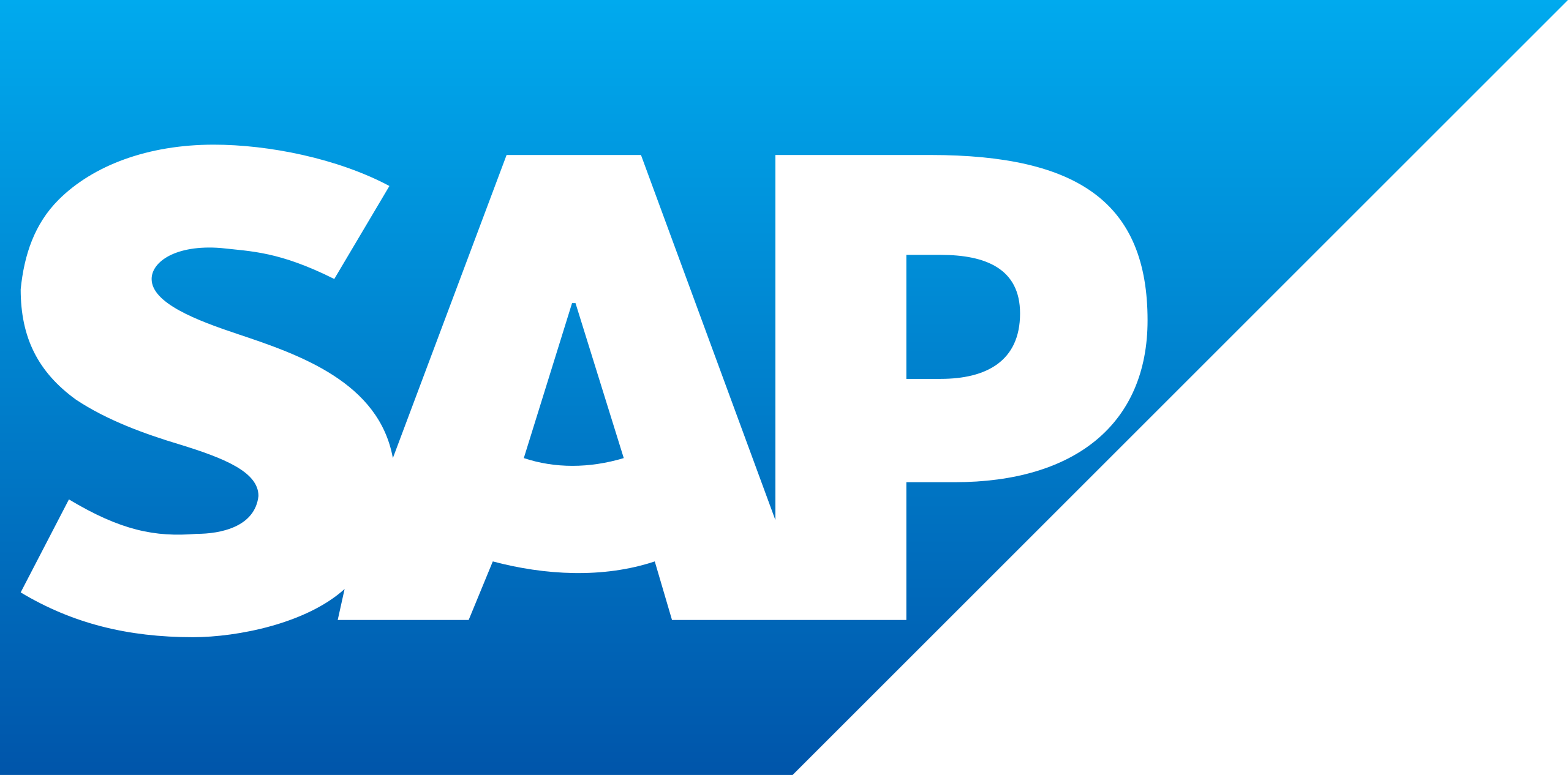
Welcome back to our ABAP tutorial series! 🚀 In Part 1, we dipped our toes into the basics of First ABAP programming. Now, let’s level up by learning how to write clean, professional reports that even your future self (or teammates) will thank you for. In this tutorial, we’ll cover:
Adding Comments (Because nobody likes cryptic code)
Formatting Output with Precision
Creating Tables with Vertical/Horizontal Lines
Real-World Project: Consultant Directory Report
Let’s turn that spaghetti code into a Michelin-star dish! 🍝 → 🍽️
🗨️ Step 1: Comments – Your Code’s Best Friend
Why Comments Matter
Imagine reading a recipe without labels—just a list of steps. Confusing, right? Comments are like sticky notes in your code, explaining what, why, and how. They’re essential for:
Collaboration: Helping others understand your logic.
Debugging: Highlighting sections for future fixes.
Clarity: Documenting complex logic.
How to Add Comments in ABAP
ABAP offers two ways:
1. Full-Line Comments
Use *
at the start of a line:
* This is a comment. It won’t execute.
WRITE: 'Hello, ABAP World!'.
2. Inline Comments
Use "
after code:
WRITE: 'Hello, ABAP World!'. " This prints a greeting
Pro Tip:
Keyboard Shortcuts:
Comment: Select lines +
Ctrl + <
Uncomment: Select lines +
Ctrl + >
📐 Step 2: Formatting Output Like a Pro
The Problem
Without formatting, your output looks like this:
John Consultant john@email.com
Jane Developer jane@email.com
Yikes. It’ll be hard to read, if there’s lots of data, right?
The Solution: Positional Formatting
Use numbers in WRITE
statements to align columns:
WRITE: 5 'John', 20 'Consultant', 35 'john@email.com'.
WRITE: /5 'Jane', 20 'Developer', 35 'jane@email.com'.
Output:
John Consultant john@email.com
Jane Developer jane@email.com
Ah, much better!
How It Works:
5
,20
,35
define starting positions for each column.Think of it as setting tab stops in a Word document.
📏 Step 3: Adding Lines for Structure
Horizontal Lines
Use ULINE
to create separators:
WRITE: /5 'John', 20 'Consultant', 35 'john@email.com'.
ULINE AT /5(50). " Draws a line from position 5, 50 characters wide
Vertical Lines
Use the system variable sy-vline
:
WRITE: /5 sy-vline, 20 sy-vline, 35 sy-vline.
Final Code:
* Header
WRITE: /5 sy-vline, 'Name', 20 sy-vline, 'Role', 35 sy-vline, 'Email', 52 sy-vline.
ULINE AT /5(48).
* Data
WRITE: /5 sy-vline, 'John', 20 sy-vline, 'Consultant', 35 sy-vline, 'john@email.com', 52 sy-vline.
WRITE: /5 sy-vline, 'Jane', 20 sy-vline, 'Developer', 35 sy-vline, 'jane@email.com', 52 sy-vline.
ULINE AT /5(48).
Output:
| Name | Role | Email |
---------------------------------------
| John | Consultant | john@email.com |
| Jane | Developer | jane@email.com |
---------------------------------------
Voilà! A polished table.
🛠️ Real-World Project: Consultant Directory Report
Your Mission
Create a report displaying consultant details in this format:
| ID | Name | Role | Email |
---------------------------------------------------------
| 001 | John Doe | ABAP Developer | john.doe@corp.com |
| 002 | Jane Smith | SAP Consultant | jane.smith@corp.com|
---------------------------------------------------------
Requirements:
Use comments to explain sections.
Align columns at positions
5
,12
,25
,50
.Add horizontal and vertical lines.
Include at least 3 consultants.
Starter Code:
* Header Section
WRITE: /5 sy-vline, 'ID', 12 sy-vline, 'Name', 25 sy-vline, 'Role', 50 sy-vline, 'Email', 74 sy-vline.
ULINE AT /5(70).
* Data Section
WRITE: /5 sy-vline, '001', 12 sy-vline, 'John Doe', 25 sy-vline, 'ABAP Developer', 50 sy-vline, 'john.doe@corp.com', 74 sy-vline.
WRITE: /5 sy-vline, '002', 12 sy-vline, 'Jane Smith', 25 sy-vline, 'SAP Consultant', 50 sy-vline, 'jane.smith@corp.com', 74 sy-vline.
ULINE AT /5(70).
💡 Best Practices & Pitfalls
Do:
Use comments liberally.
Test positions with dummy data first.
Use
sy-vline
for vertical lines (not manual|
).
Don’t:
Hardcode positions without testing.
Forget to add separators—your users will hate you.
💬 Let’s Discuss!
Stuck? Share your code in the comments!
Help Others? How do you format ABAP reports?
Happy coding! 👨💻👩💻
Follow me for more ABAP tutorials, and smash that like button if this helps! 👍
Subscribe to my newsletter
Read articles from Fahim Al Jadid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
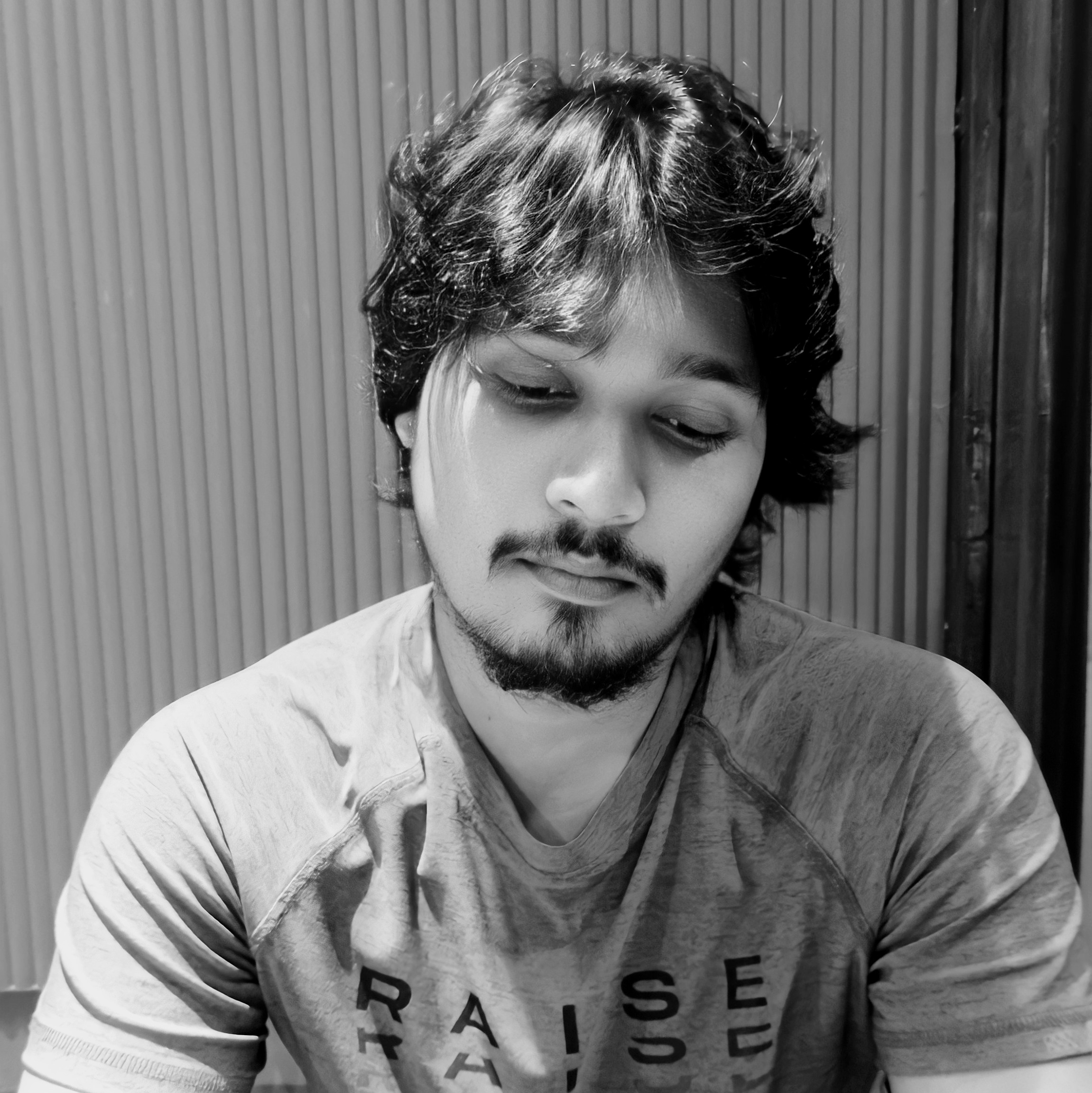