๐ผ๏ธ Image Processing with OpenCV & Python: A Beginnerโs Guide

Table of contents
- ๐ Introduction
- ๐ Installing OpenCV for Image Processing
- ๐ Importing OpenCV in Python
- ๐ผ๏ธ Reading and Viewing an Image
- ๐ Image Properties & Shape
- ๐ข Image as Numerical Data
- ๐ Image Resolution & Pixels
- ๐ฅ๏ธ Displaying an Image in OpenCV
- ๐พ Saving an Image
- ๐ Extracting Image Bit Planes
- ๐ผ๏ธ Creating a Synthetic Image in OpenCV
- ๐ ๏ธ FAQs on OpenCV Image Processing
- ๐ Conclusion
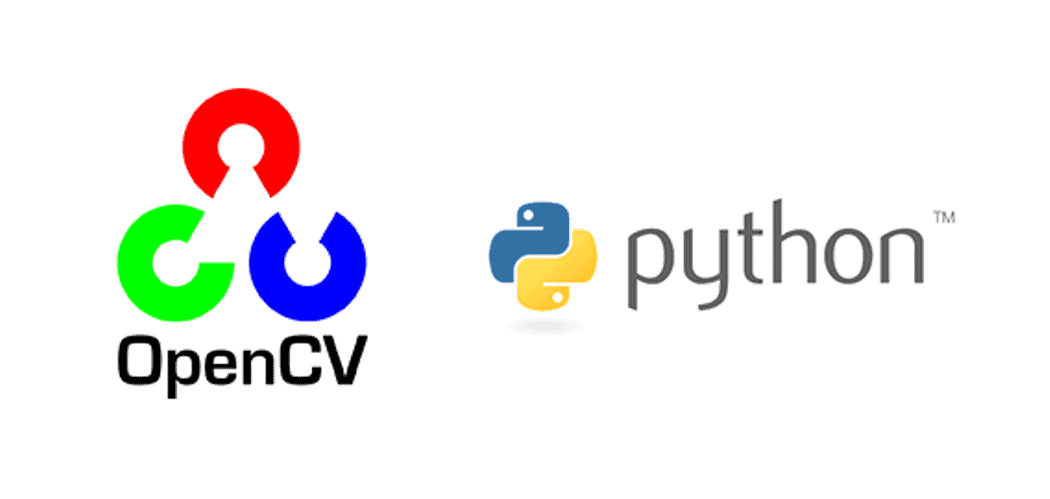
๐ Introduction
Ever wondered how machines interpret images? Image processing is at the heart of computer vision, enabling machines to extract meaningful insights from digital visuals. Whether it's facial recognition, object detection, or image filtering, OpenCV + Python is a powerful combo for building AI-driven applications.
In this guide, weโll dive into image processing with OpenCV, covering installation, basic operations, and essential functions. Whether youโre a beginner or looking to refresh your skills, this tutorial will help you understand the fundamentals of image manipulation in Python.
๐ Installing OpenCV for Image Processing
OpenCV (Open-Source Computer Vision Library) is a fast and efficient tool for image and video processing. To install OpenCV, simply run:
pip install opencv-python
or
pip install opencv-contrib-python
For Anaconda users, replace pip
with conda
:
conda install -c conda-forge opencv
๐ Importing OpenCV in Python
In Python, packages are collections of pre-written code modules. OpenCV is imported using the cv2
module:
import cv2
Now, letโs dive into basic image operations! ๐
๐ผ๏ธ Reading and Viewing an Image
Images come in various types:
โ
Color Images (RGB) โ Contains full-color details
โ
Grayscale Images โ Shades of gray with intensity values
โ
Binary Images โ Black & white pixel representation
โ
Multispectral Images โ Captures different wavelengths
๐ Read an Image in OpenCV
img = cv2.imread("mandrill.jpg", 1) # Reads a color image
๐ 1
โ Reads a color image (default)
๐ 0
โ Reads the image in grayscale
๐ -1
โ Reads the image with alpha/transparency
๐ Image Properties & Shape
Every image has dimensions (height, width) and color channels. To check an imageโs properties:
print(img.shape)
or extract values:
h, w, c = img.shape
print(f"Height: {h}, Width: {w}, Channels: {c}")
โ
Color images return (height, width, 3) (3 = RGB channels)
โ
Grayscale images return (height, width)
๐ข Image as Numerical Data
In Python, images are stored as multi-dimensional NumPy arrays, where each pixel is a numerical value. To check an imageโs type:
print(type(img))
print(img.dtype) # Data type of pixel values
๐ก Each pixel value ranges from 0 to 255, defining brightness and contrast.
๐ Image Resolution & Pixels
Image resolution is the number of pixels in an image. The higher the resolution, the better the quality.
Standard resolutions:
โ
320x240 โ Small screens
โ
1024x768 โ Computer monitors
โ
1920x1080 (Full HD) โ TVs & displays
โ
3840x2160 (4K) โ Ultra HD
To calculate the total pixel count:
total_pixels = h * w
print(f"Total Pixels: {total_pixels}")
๐ฅ๏ธ Displaying an Image in OpenCV
To open and display an image in a window:
cv2.imshow("Mandrill", img)
cv2.waitKey(0) # Waits indefinitely for a key press
cv2.destroyAllWindows() # Closes all windows
๐ก Press ESC
or q
to close the window.
๐พ Saving an Image
Letโs convert an image to grayscale and save it:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imwrite('Mandrill_gray.jpg', gray)
The first parameter is the filename, and the second is the image variable.
๐ Extracting Image Bit Planes
Every image consists of bit planes. We can extract individual bit planes to analyze image details:
import matplotlib.pyplot as plt
import numpy as np
def extract_bit_plane(image):
planes = [np.mod(np.floor(image / (2 ** i)), 2) for i in range(8)]
fig, axes = plt.subplots(2, 5, figsize=(10, 8))
for ax, plane in zip(axes.flat, planes):
ax.imshow(plane, cmap='gray')
plt.show()
extract_bit_plane(gray)
This function separates bit layers of an image for detailed analysis. ๐
๐ผ๏ธ Creating a Synthetic Image in OpenCV
We can generate a simple synthetic image with different intensity values:
con_img = np.zeros([256, 256]) # Create a blank image
con_img[0:32, :] = 40
con_img[:, :32] = 40
con_img[224:, :] = 40
con_img[:, 224:] = 40
con_img[96:160, 96:160] = 220 # Center square
plt.imshow(con_img, cmap='gray')
plt.show()
๐ก This creates four concentric squares with different intensities.
๐ ๏ธ FAQs on OpenCV Image Processing
โ Can OpenCV be used for image processing?
โ Yes! OpenCV provides powerful image processing tools like filtering, transformations, and feature extraction.
โ Which OpenCV module is used for image processing?
โ
OpenCVโs imgproc
module handles image manipulation, including thresholding, contour detection, and edge detection.
โ Is Python good for image processing?
โ Absolutely! Pythonโs simplicity combined with OpenCVโs efficiency makes it a top choice for image processing.
โ Why use OpenCV for image & video processing?
โ OpenCV is fast, lightweight, and supports multiple programming languagesโmaking it perfect for real-time AI applications.
๐ Conclusion
OpenCV + Python is a powerful tool for image processing, AI, and computer vision. With simple functions, we can read, manipulate, and enhance images efficiently. As you progress, explore edge detection, object tracking, and deep learning applications with OpenCV.
Start coding and bring your images to life! ๐๐ฅ
Subscribe to my newsletter
Read articles from Belaid Abdelhadi (Taylor) directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
