Cascade delete in mongo db[typescript]

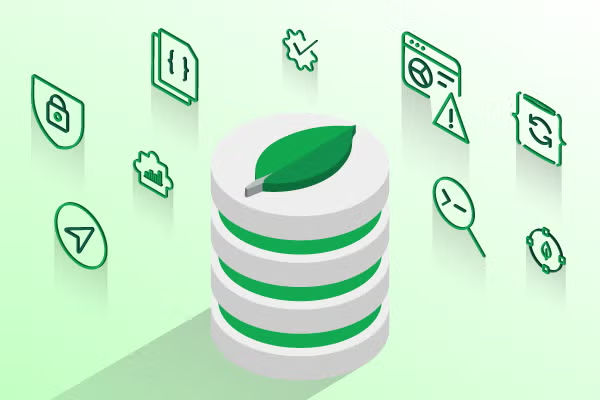
Cascading in MongoDB using Mongoose refers to the process where related documents in different collections are automatically updated or deleted when a document is modified or removed. Although MongoDB doesn't support this feature natively, it can be achieved through Mongoose middleware, specifically using pre
hooks.
In my current e-commerce project, I've set up two collections: one for product images and another for product specifications.
These hooks in Mongoose are beneficial as they allow developers to write less code while achieving more functionality. However, they come with some drawbacks. Hooks can make queries more resource-intensive because they involve additional operations. Moreover, implementing them can be challenging, as multiple hooks are needed for similar tasks, such as updateOne
, updateMany
, and findOneAndUpdate
.
Despite the complexity, using hooks can lead to a cleaner codebase by reducing repetitive code. Here's an example to illustrate this concept.
import { ProductImagesModel } from "./ProductImages.js";
import { z } from "zod";
import mongoose, { Schema, model } from "mongoose";
//product is a zod schema I am not providing here.
export type ProductType = z.infer<typeof Product>
export const ProductSchema = new Schema<ProductType>({
brandName: {
type: String,
required: [true, 'brand name is required.'],
validate: {
validator: (value) => Product.pick({ brandName: true }).safeParse({ brandName: value }).success,
message: (props: { value: string; }) => `${props.value} is not a valid brand name.`
}
},
description: {
type: String,
required: [true, 'description is required'],
validate: {
validator: (value) => Product.pick({ description: true }).safeParse({ description: value }).success,
message: (props: { value: string; }) => `${props.value} is not a valid description.`
}
},
catagory: {
type: String,
validate: {
validator: (v) => Product.pick({ catagory: true }).safeParse({ catagory: v }).success,
message: (prop: { value: string; }) => `${prop.value} is not a valid catagory`
}
},
details: {
type: String,
required: [true, 'details is required'],
validate: {
validator: (value) => Product.pick({ details: true }).safeParse({ details: value }).success,
message: (props: { value: string; }) => `${props.value} is not a valid details.`
}
},
sellerId: {
type: mongoose.Schema.ObjectId,
ref: "client_model",
required: [true, "sellerId is required."]
},
quantity: {
type: Number,
required: [true, 'quantity must be provided'],
validate: {
validator: (v) => Product.pick({ quantity: true }).safeParse({ quantity: v }).success,
message: (prop: { value: string; }) => `${prop.value} is not a valid quantity.`
}
},
price: {
type: Number,
required: [true, "price can't be empty"],
validate: {
validator: value => Product.pick({ price: true }).safeParse({ price: value }).success,
message: (props: { value: string; }) => `${props.value} is not a valid price.`
}
}
}, { timestamps: true });
ProductSchema.pre('deleteOne', { document: false, query: true }, async function (next) {
const product_id = this.getFilter()._id;
//console.log(product_id);
const result = await ProductImagesModel.deleteMany({ productId: product_id });
//console.log(result);
next();
});
export const ProductModel = model<ProductType>('product_model', ProductSchema);
Subscribe to my newsletter
Read articles from Soumabha Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Soumabha Saha
Soumabha Saha
My name is Soumabha and I'm a CSE student and have a hobby of web development. Please subscribe to my channel for the upcoming tutorials.