My Fav Top 10 Properties of Array in javascript
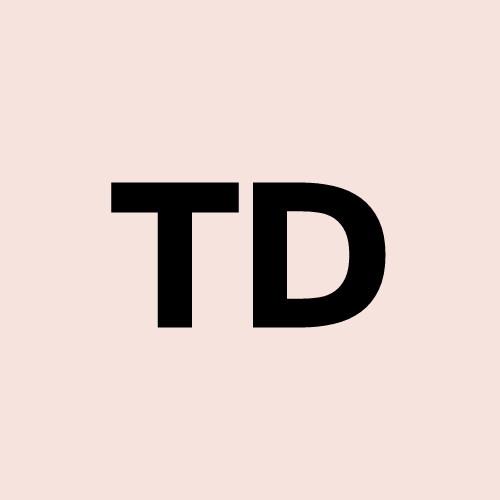
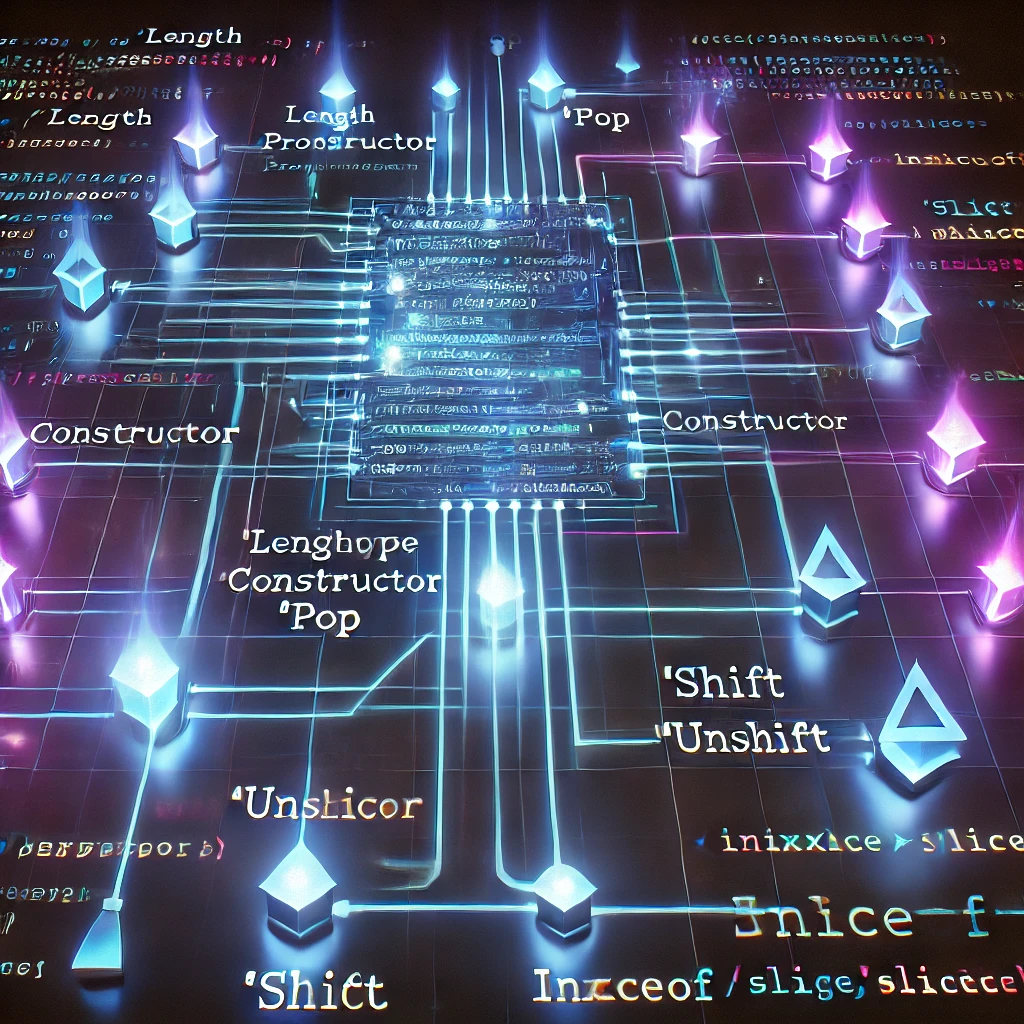
JavaScritpt considers an Array( non-preemptive Data Structure ) as the “Object“ that enables us to store a mix of different data types under a singular name to perform common operations on each data and arrays in Javascript are Resizable.
We Are taking the example of the NBA and specifically the Lakers team to understand the Properties of the Array
1- Defining the Array (Lakers Starting 5 Before Luka Trade)
let startingFive = ["LeBron James", "Anthony Davis", "Austin Reaves", "Rui Hachimura","Jaxson Hayes"];
Alternatively, we can use an array of player objects with additional data like points scored:
let players = [
{ name: "LeBron James", points: 25 },
{ name: "Anthony Davis", points: 28 },
{ name: "Austin Reaves", points: 20 },
{ name: "Rui Hachimura", points: 15 },
{ name: "Jaxson Hayes", points: 10 },
{ name: "Dalton Knect", points: 30},
];
Using these arrays, here are the top 10 most used array properties and methods, along with explanations and NBA team examples:
1. length (Property)
Description: Returns the number of elements in the array.
Example: To find out how many players are in the starting lineup:
let numberOfPlayers = startingFive.length; console.log(numberOfPlayers); // Output: 5
NBA Context: This tells the coach there are 5 players out of 15 players in the starting five.
2. push() (Method - new player join the team at the last postion) —> Luka Doncic joins the Team from Dallas
Description: Adds one or more elements to the end of the array and returns the new length.
Example: Adding a new player to the roster:
startingFive.push("Luka Doncic"); console.log(startingFive); // ["LeBron James", "Anthony Davis", "Austin Reaves", "Rui Hachimura", "Jaxson Hayes","Luka Dončić"]
NBA Context: A new player joins the team added to the list. (Trade between teams happens)
After Assign new player his position have to trade our player
["LeBron James", "Luka Dončić", "Austin Reaves", "Rui Hachimura", "Jaxson Hayes", "Anthony Davis"]
3. pop() (Method) → Anthony Davis Traded to Dallas Mavericks
Description: Removes the last element from the array and returns it.
Example: Removing the last player from the roster:
javascript
let removedPlayer = startingFive.pop(); console.log(removedPlayer); // "Anthony Davis" console.log(startingFive); // ["LeBron James", "Luka Dončić", "Austin Reaves", "Rui Hachimura", "Jaxson Hayes", "Anthony Davis"]
NBA Context: Anthony Davis is traded to the Dallas Mavericks
4. shift( ) (Method) - Our star player is out from injury for a few games
Description: Removes the first element from the array and returns it.
Example: Removing the first player from the lineup:
javascript
let firstPlayer = startingFive.shift(); let reservePlayer = startingFive.push("Dalton Knect") console.log(firstPlayer); // "LeBron James" console.log(startingFive); // ["Luka Dončić", "Austin Reaves", "Rui Hachimura", "Jaxson Hayes","Dalton Knect" ]
NBA Context: The leading player (LeBron James) is sidelined due to injury.
5. unshift() (Method)
Description: Adds one or more elements to the beginning of the array and returns the new length.
Example: Adding a star injured star player to the start of the list:
startingFive.unshift("Another New Player");//"LeBron James" let removedPlayer = startingFive.pop(); //"Dalton Knect" console.log(startingFive); //["LeBron James ","Luka Dončić", "Austin Reaves", "Rui Hachimura", "Jaxson Hayes" ]
NBA Context: A high-profile injured player takes a leading spot.
6. indexOf() (Method)
Description: Returns the first index at which a given element is found, or -1 if it’s not present.
Example: Finding the position of a specific player:
let position = startingFive.indexOf("Anthony Davis"); console.log(position); // 1 (assuming the original array)
NBA Context: Checking where a player ranks in the lineup order.
7. slice() (Method)
Description: Returns a shallow copy of a portion of the array, specified by start and end indices (end not included).
Example: Getting the first three players:
javascript
let firstThree = startingFive.slice(0, 3); console.log(firstThree); // ["LeBron James ","Luka Dončić", "Austin Reaves"]
NBA Context: Selecting the top three players for a special play (Coach draws a play for these players in the last minutes of the game).
8. splice() (Method)
Description: Adds or removes elements from the array at a specified position.
Example: Replacing the third player in the lineup:
startingFive.splice(2, 1, "Dalton Knect"); //Replaces 1 player at index 2 (Austin Reaves is replaced with Dalton Knect ) console.log(startingFive); // ["LeBron James ","Luka Dončić", "Dalton Knect", "Rui Hachimura", "Jaxson Hayes"]
NBA Context: Substituting a player due to performance or strategy. (At the last minute coach decides to change player )
9. forEach() (Method)
Description: Executes a provided function once for each array element.
Example: Logging each player’s name:
startingFive.forEach(function(player) { console.log(player); }); // Output: //LeBron James //Luka Dončić //Dalton Knect //Rui Hachimura //Jaxson Hayes
NBA Context: Coach Announcing the Lineup After Half-Time (new starting 5).
10. map() (Method)
Description: Creates a new array with the results of calling a provided function on every element.
Example: Extracting points from the player’s array:
javascript
let pointsArray = players.map(function(player) { return player.points; }); console.log(pointsArray); // [25, 28, 30, 15, 10]
NBA Context: Compiling a list of points scored by each player in a game.(After the game scoreline)
My first article hope you like it.
#chaicode
Subscribe to my newsletter
Read articles from Tarun Dhouni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
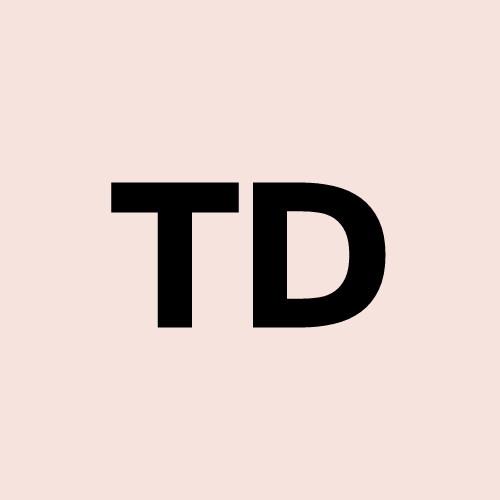