parseInt() vs Number(): Understanding the Key Differences in JavaScript
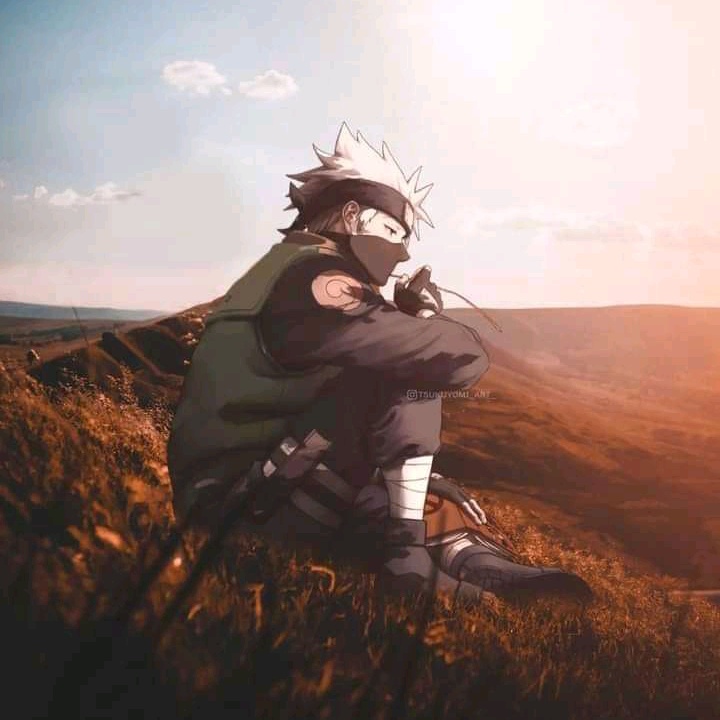
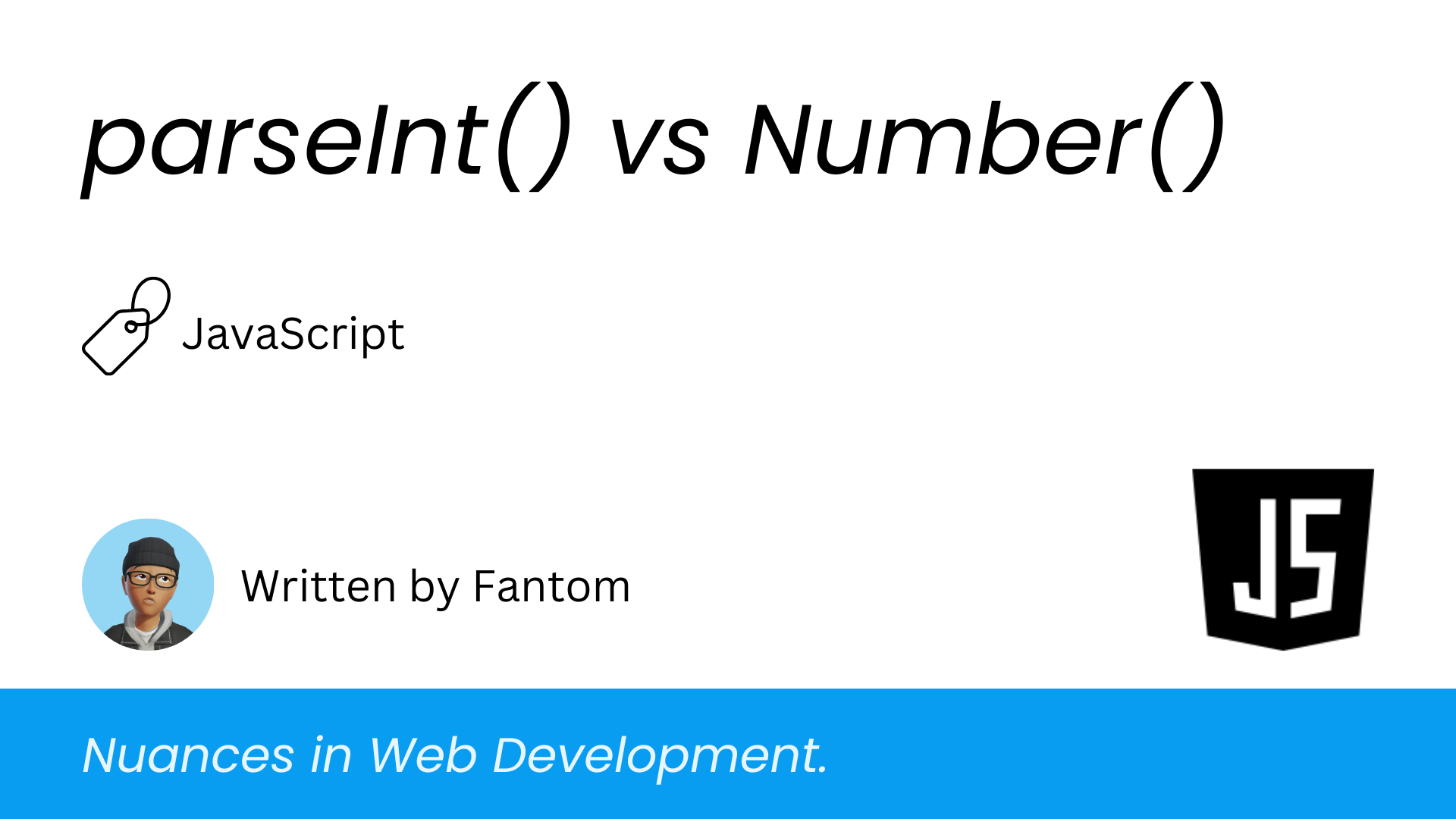
JavaScript provides multiple ways to convert a value into a number, but two commonly used methods—parseInt()
and Number()
—behave quite differently. While they might seem interchangeable at first glance, their nuances can significantly impact your code.
In this article, we’ll break down the differences between parseInt()
and Number()
, exploring their use cases, potential pitfalls, and best practices.
1. parseInt()
: Extracting Integers from Strings
The parseInt()
function is designed to extract an integer from a string. It parses the string character by character, stopping at the first non-numeric character.
parseInt(string, radix)
//string: The value to be parsed.
//radix: (Optional) The base (e.g., 10 for decimal, 16 for hexadecimal).
Examples
console.log(parseInt("42")); // 42
console.log(parseInt("42px")); // 42 (stops at 'p')
console.log(parseInt("3.14")); // 3 (stops at '.')
console.log(parseInt("hello")); // NaN (not a number)
console.log(parseInt("010", 10)); // 10 (explicitly decimal)
console.log(parseInt("010", 8)); // 8 (interpreted as octal)
Key Takeaways
It stops parsing at the first non-numeric character.
It works best for extracting integers from mixed-character strings.
Specifying the radix is crucial to avoid unexpected results.
2. Number()
: A More Strict Conversion
Unlike parseInt()
, the Number()
function attempts to convert the entire value into a valid number. If the conversion fails, it returns NaN
.
Number(value)
Examples
console.log(Number("42")); // 42
console.log(Number("42px")); // NaN (invalid number)
console.log(Number("3.14")); // 3.14 (fully converts decimals)
console.log(Number("")); // 0 (empty string becomes 0)
console.log(Number("hello")); // NaN
console.log(Number(true)); // 1 (boolean conversion)
console.log(Number(false)); // 0
console.log(Number(null)); // 0
console.log(Number(undefined)); // NaN
Key Takeaways
It fully converts the input or returns
NaN
if conversion isn’t possible.Works well for handling decimals, booleans, and null values.
More strict compared to
parseInt()
, making it suitable for numeric validation.
3. Key Differences at a Glance
Feature | parseInt() | Number() |
Stops at non-numeric characters? | Yes | No |
Works with decimals? | No (truncates them) | Yes |
Handles booleans? | No | Yes (true → 1 , false → 0 ) |
Handles empty strings? | NaN | 0 |
Handles null ? | NaN | 0 |
Handles undefined ? | NaN | NaN |
Requires a radix? | Recommended | No |
4. When to Use Each?
Use
parseInt()
when working with strings that contain numbers mixed with other characters (e.g.,"42px"
,"50% discount"
).Use
Number()
when ensuring a value is strictly converted into a number without partial parsing.
Conclusion
While both parseInt()
and Number()
are used for numeric conversions, their subtle differences can greatly impact your JavaScript logic. parseInt()
is useful for extracting integers from strings, whereas Number()
is stricter and works well for full conversions.
This is the first article in our Nuances in Web Development series, where we explore subtle yet useful differences in JavaScript and web technologies. Join the newsletter to ensure you don't miss the next article in the series 🪂. Don't forget to share and leave a like ❤️.
Subscribe to my newsletter
Read articles from Peter Bamigboye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
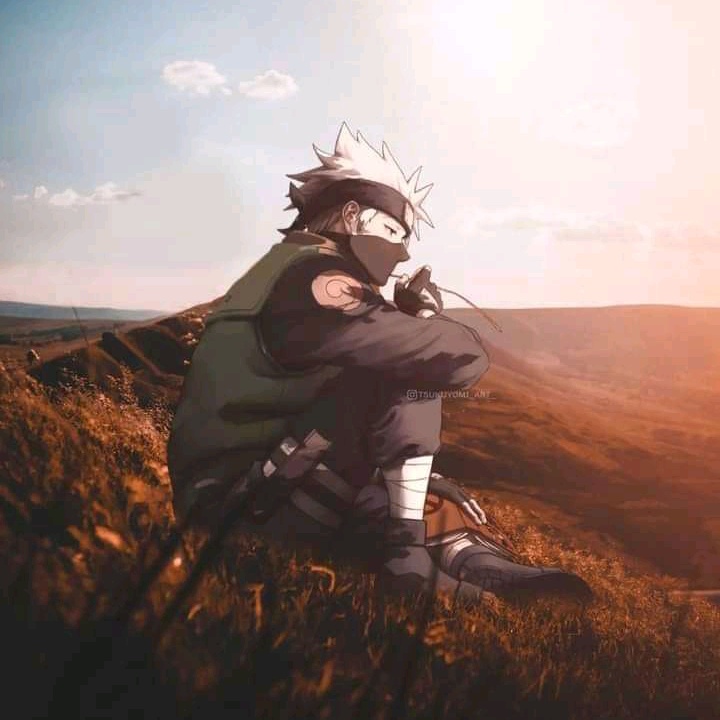
Peter Bamigboye
Peter Bamigboye
I am Peter, a front-end web developer who writes on current technologies that I'm learning or technologies that I feel the need to simplify.