Exploring the Architecture of Microsoft D365 FnO

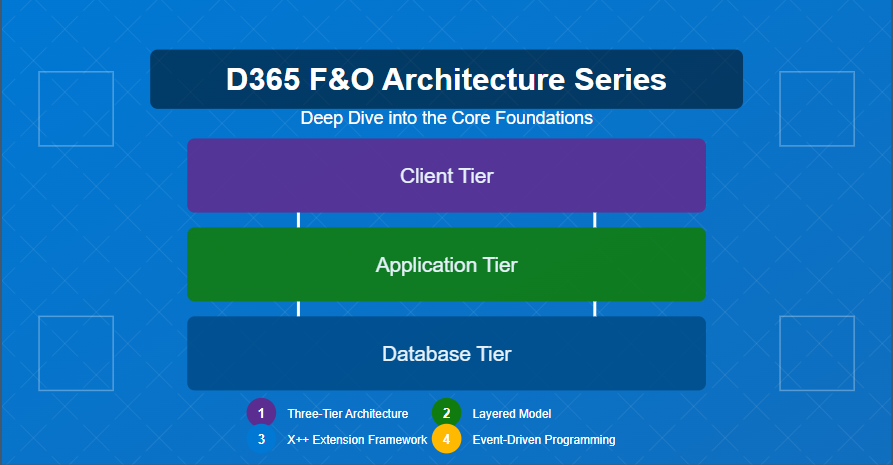
Introduction: The Beginning of Our Journey
Welcome to the first post in what will be a comprehensive series exploring the architecture and design patterns of Microsoft Dynamics 365 Finance and Operations. Over the coming months, we'll dive deep into the intricate details of this powerful enterprise platform, equipping you with the knowledge needed to architect robust, maintainable solutions.
What This Series Will Cover
This journey will take us through:
The fundamental architecture of D365 F&O
The layered model and extension framework
Event-driven programming techniques
Design patterns implemented throughout the platform
Real-world case studies and implementation strategies
Performance optimization and best practices
Focus: The Three-Tier Architecture
In this inaugural post, we're starting with the foundation: the three-tier architecture that forms the backbone of D365 F&O. Understanding how the client, application, and database tiers interact is essential before we can explore more advanced concepts in future installments.
D365 F&O Architecture: The Evolution to Cloud
Before diving into the current architecture, it's worth briefly considering the evolution from Axapta to Dynamics AX and finally to D365 F&O. This evolution has seen a significant shift from an on-premises, tightly-coupled system to a cloud-native, services-oriented platform.
The move to Azure has fundamentally transformed how the application is architected, deployed, and maintained. What was once a monolithic application is now a distributed system taking full advantage of cloud services.
The Three-Tier Architecture Overview
D365 F&O follows a classic three-tier architectural pattern, but with modern cloud-specific enhancements:
As shown in the diagram above, the three primary tiers are:
Client Tier*: The presentation layer that users interact with*
Application Tier*: The business logic layer where processing happens*
Database Tier*: The data storage and management layer*
Let's explore each of these tiers in detail.
Client Tier: The User Interface Layer
The client tier represents all interfaces through which users interact with D365 F&O. Unlike previous versions which required a rich client, D365 F&O uses a browser-based HTML5 client as its primary interface.
Web Browser Client
HTML5/JavaScript UI: Renders forms, dialogs, and workspaces
Responsive Design: Adapts to different screen sizes and devices
Client-side Validation: Performs basic validations before server calls
Local Caching: Improves performance for frequently used data
Mobile Experiences
Warehouse Mobile App: Optimized for warehouse operations
Expense Management: Mobile receipt capture and submission
Inventory Management: On-the-go inventory operations
Retail Point of Sale: Store operations and customer transactions
Integration with Office and Power Platform
Excel Add-ins: Direct data editing and analysis in Excel
Power Apps: Custom mobile and tablet experiences
Power BI: Embedded analytics and reporting
Outlook Integration: Email-based approvals and notifications
Code Example: Client-Side Form Control
// X++ Form definition
//For mental Model not Production ready
public class Form extends FormRun
{
FormControl CustomerNameControl;
public void init()
{
super();
// Set control properties
CustomerNameControl.mandatory(true);
CustomerNameControl.visible(SecurityPrivileges::canViewCustomerDetails());
}
public boolean validate()
{
boolean isValid = true;
if (!CustomerNameControl.text())
{
isValid = checkFailed("Customer name is required");
}
return isValid && super();
}
}
This form definition generates HTML elements with corresponding JavaScript that handles client-side validation and server communication.
Application Tier: The Business Logic Engine
The application tier hosts the Application Object Server (AOS) and supporting services that execute business logic, process transactions, and manage workflows.
Application Object Server (AOS)
X++ Runtime Environment*: Executing X++ code on the .NET CLR*
Business Logic Processing*: Implementing business rules and validations*
Data Access Coordination*: Managing database connections and queries*
Session Management*: Handling user sessions and state*
Key Application Tier Components
1. Batch Processing Framework
Schedules and executes background tasks
Manages task dependencies and priorities
Provides monitoring and error handling
Supports recurrence patterns for periodic tasks
2. Workflow Engine
Implements approval processes
Manages workflow states and transitions
Supports conditional branching and parallel execution
Integrates with email notifications and task assignments
3. Reporting Services
Integrates with SQL Server Reporting Services
Generates financial and operational reports
Provides export capabilities to various formats
Supports Electronic Reporting Framework for regulatory reports
4. Integration Services
Exposes OData endpoints for external access
Manages data import/export processes
Publishes and subscribes to business events
Supports file-based integration scenarios
X++ Runtime Environment
The X++ runtime is a critical part of the application tier:
A Example Code demonstrating the Service + Data Contract pattern that is widely used in Dynamics 365 F&O.
// Service class handling bank account operations
//Treat this an example code to build mental model
[ServiceClass]
public class BankAccountService
{
[AifPermission]
public BankAccount getByAccountNumber(AccountNum _accountNum)
{
BankAccountTable bankAccountTable;
// Data access using X++ query
select firstonly * from bankAccountTable
where bankAccountTable.AccountNum == _accountNum;
// Create data contract from table record
BankAccount bankAccount = new BankAccount();
bankAccount.parmAccountNum(bankAccountTable.AccountNum);
bankAccount.parmCurrency(bankAccountTable.Currency);
bankAccount.parmBalance(bankAccountTable.Balance);
return bankAccount;
}
}
This X++ code compiles to IL (Intermediate Language) and executes within the .NET CLR in the application tier.
Database Tier: The Data Foundation
The database tier is responsible for storing and managing all application data. In D365 F&O, this tier consists primarily of Azure SQL Database.
Azure SQL Database
The primary database components include:
Transactional Tables: Store operational data (customers, orders, etc.)
Financial Ledgers: Maintain accounting records
Reference Data: Store configuration and lookup values
System Tables: Track metadata, versions, and configuration
Entity Store
The Entity Store provides analytics capabilities:
Aggregate Measurements: Precalculated KPIs and metrics
Denormalized Views: Optimized for analytical queries
Real-time Analytics: Near real-time operational insights
Power BI Integration: Direct connection to visualization tools
BYOD (Bring Your Own Database)
BYOD enables customers to:
Replicate D365 Data: Copy data to external databases
Custom Analytics: Build custom reporting solutions
Data Warehousing: Integrate with enterprise data warehouses
Legacy System Integration: Combine with data from other systems
Data Access Example
Query records from a table (BankAccountTable
)
//Using a List of containers - Just for Mental model (not production ready)
public List retrieveActiveAccounts()
{
List results = new List(Types::Container);
BankAccountTable bankAccount;
while select AccountNum, Currency, Balance from bankAccount
where bankAccount.Status == BankAccountStatus::Active
{
results.addEnd([bankAccount.AccountNum, bankAccount.Currency, bankAccount.Balance]);
}
return results;
}
Communication Between Tiers
Understanding how the tiers communicate is essential for building performant applications:
Client to Application Tier
HTTP/HTTPS Protocol*: Secure web communication*
JSON Data Format*: Lightweight data exchange*
OData Services*: RESTful API access*
Authentication*: Azure AD integrated security*
Application to Database Tier
Connection Pooling: Efficient database connections
Parameterized Queries: Secure query execution
Entity Framework: Object-relational mapping
Processing Flow
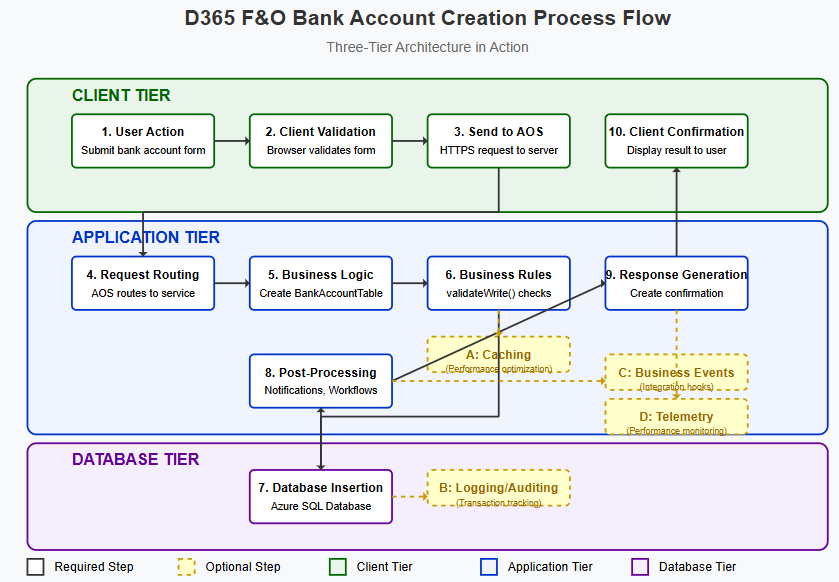
Deployment Topologies
Development Environment
One-box Configuration: All tiers on a single VM
Visual Studio Integration: Direct debugging capabilities
Local Build and Test: Rapid development cycle
Cloud Production Environments
Tier 2+ Environments: Separate VMs for application and database tiers
Scale Units: Additional capacity for specific modules
High Availability: Redundant components and failover
Geo-Replication: Cross-region disaster recovery
Performance Considerations
Client Tier Performance
Browser caching strategies
Network latency mitigation
Progressive loading patterns
JavaScript optimization
Application Tier Performance
X++ code optimization
Batch task distribution
Workflow processing efficiency
Memory management
Database Tier Performance
Index optimization
Query plan management
Data archiving strategies
Partitioning large tables
Conclusion and Best Practices
Understanding the three-tier architecture of D365 F&O provides a solid foundation for development:
Design with Tier Separation*: Keep appropriate logic in the right tier*
Optimize Network Communication*: Minimize round-trips between tiers*
Consider Performance Impact*: Each tier has different scaling characteristics*
Follow Security Best Practices*: Each tier requires specific security approaches*
By respecting the architectural boundaries between tiers, you'll create more maintainable and scalable solutions.
What's Coming Next
Now that we've established a solid understanding of D365 F&O's three-tier architecture, our next post will explore the layered model that enables extensibility without modifying base code. We'll examine how the SYS, GLS, FPK, VAR, ISV, and CUS layers work together, and demonstrate best practices for layer selection.
Subscribe to my newsletter
Read articles from Challa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
