Using Extensions in xUnit 3

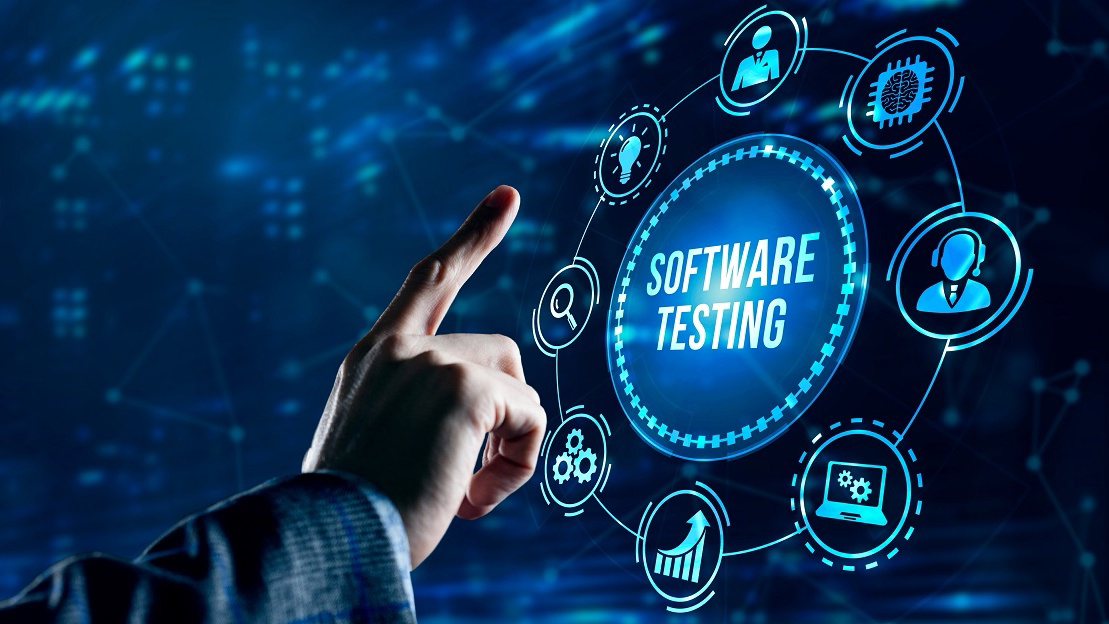
With the arrival of xUnit 3, we have gained new opportunities to streamline and enhance our testing workflows by leveraging custom extensions. Extensions in xUnit 3 allow us to inject additional behaviour directly into the testing lifecycle, tailoring test execution precisely to the project's unique requirements. These extensions offer flexibility and can significantly improve the readability, maintainability, and functionality of test suites. Extensions in xUnit 3 serve as powerful additions that easily integrate into test projects, enabling sophisticated setups and teardowns without cluttering the actual tests. Unlike earlier versions, xUnit 3 simplifies this capability, making extensions more intuitive and approachable even for people unfamiliar with advanced unit-testing practices. Writing custom extensions becomes straightforward, resulting in test code that clearly communicates its intent without verbose or repetitive constructs.
Think about a scenario where specific environment settings or configuration values must be consistently applied before tests execute. Instead of repeating this configuration within each test, an extension can automatically handle these tasks. For example, an extension could initialise certain configuration settings required by all tests and reset them afterwards, ensuring a consistent environment. Here's a brief example:
public class ConfigurationFixture : IAsyncLifetime
{
public AppConfig Config { get; private set; }
public Task InitializeAsync()
{
Config = new AppConfig { Environment = "Test", FeatureToggle = true };
return Task.CompletedTask;
}
public Task DisposeAsync()
{
Config = null;
return Task.CompletedTask;
}
}
In your test class, the extension integrates easily:
public class FeatureServiceTests : IClassFixture<ConfigurationFixture>
{
private readonly ConfigurationFixture _fixture;
public FeatureServiceTests(ConfigurationFixture fixture)
{
_fixture = fixture;
}
[Fact]
public void ShouldEnableFeatureInTestEnvironment()
{
var service = new FeatureService(_fixture.Config);
var isEnabled = service.IsFeatureEnabled();
Assert.True(isEnabled);
}
}
This approach simplifies test methods, removing repetitive configuration setup code and enabling clearer, more maintainable tests. The fixture ensures that each test class receives the necessary configuration without direct management, providing consistency and reliability across the test suite.
Extensions also support scenarios requiring conditional test execution. Suppose certain tests should only run under specific conditions, like feature flags or environment specific tests. xUnit 3's extension model allows creating custom attributes to define these conditions. A test might look like this:
[FeatureEnabled("NewCheckout")]
[Fact]
public void ShouldUseNewCheckoutFlow()
{
var checkout = new CheckoutService();
var result = checkout.Process();
Assert.Equal("NewFlow", result.FlowType);
}
Behind the scenes, the custom attribute checks if the "NewCheckout" feature is enabled and skips the test if conditions aren't met. Such functionality keeps test outcomes meaningful and prevents misleading failures due to unmet conditions.
Creating extensions in xUnit 3 encourages clearer separation of concerns. Test classes can focus purely on asserting correctness, while extensions handle crosscutting concerns such as logging, timing, or configuration setup. Developers benefit from cleaner, more expressive tests that accurately document their expectations without unnecessary complexity. By exploring custom extensions in xUnit 3, we can unlock potential improvements in our testing approach. Extensions provide tailored solutions to common problems, enhance test reliability, and contribute significantly to overall code quality. Investing time in learning and adopting extensions will pay dividends, streamlining test maintenance and improving developer experience substantially.
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
