Building an AI-Powered Othello (Reversi) Game in Python with Pygame
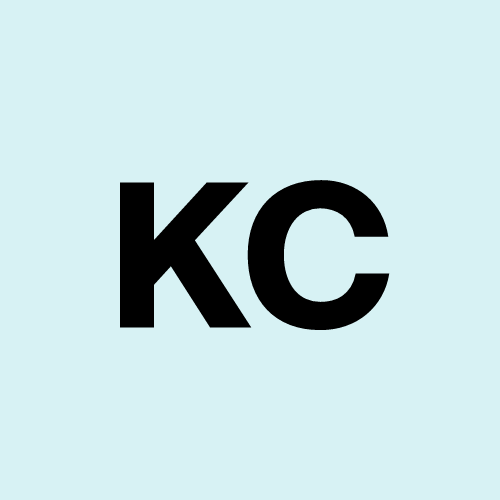
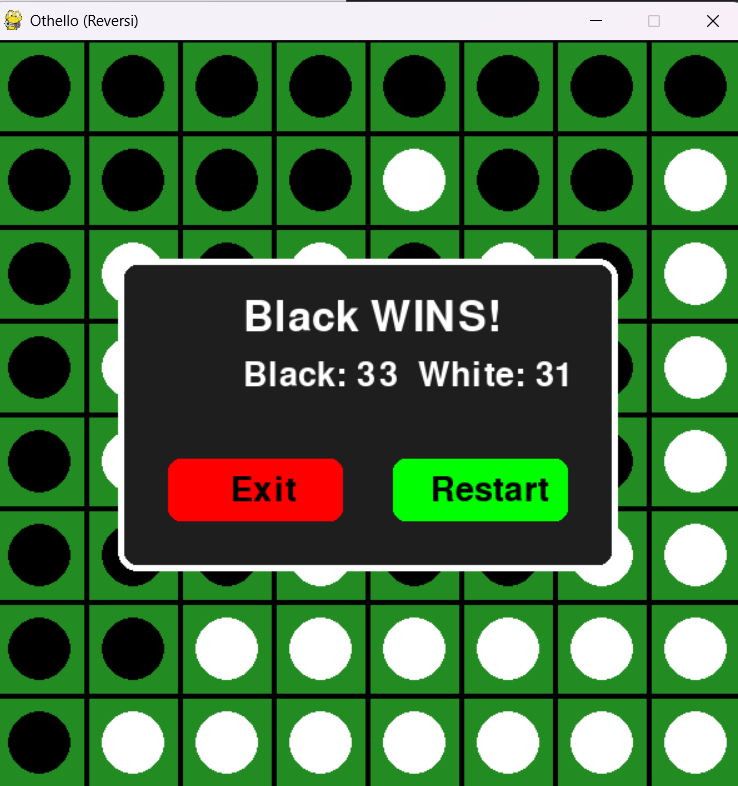
Introduction
Othello, also known as Reversi, is a classic strategy board game that challenges players to outmaneuver their opponents by flipping pieces on an 8×8 grid. In this blog, we’ll walk through the development of an AI-powered Othello game using Python and PyGame. Our AI opponent is built using the Minimax algorithm with Alpha-Beta pruning, making the game both challenging and engaging.
By the end of this post, you'll have a fully functional Othello game with an AI opponent, and you’ll understand how Minimax helps in decision-making for board games.
Features of the Game
✅ Single-player mode – Play against an AI opponent.
✅ AI with Minimax Algorithm – The computer calculates the best moves.
✅ Real-time move validation – Ensures only legal moves are allowed.
✅ Game-over screen – Displays the final score and winner.
✅ Smooth UI with PyGame – A simple and interactive interface.
Technologies Used
Python – The core programming language.
PyGame – For rendering the board, handling input, and managing game events.
Minimax Algorithm with Alpha-Beta Pruning – To implement AI decision-making.
Installation and Setup
1️⃣ Clone the Repository
git clone https://github.com/yourusername/othello-reversi.git
cd othello-reversi
2️⃣ Install Dependencies
pip install pygame
3️⃣ Run the Game
python othello.py
Game Rules and How to Play
Othello follows simple yet strategic gameplay rules:
1️⃣ The game starts with four pieces (two black, two white) placed at the center of an 8×8 grid.
2️⃣ Players take turns placing a piece, ensuring at least one opponent's piece is flipped.
3️⃣ A move is valid only if it results in flipping at least one opponent’s piece.
4️⃣ The player with the most pieces on the board at the end wins.
Understanding the AI: Minimax Algorithm
The Minimax algorithm is a recursive decision-making algorithm used in two-player turn-based games. Here’s how it works:
1️⃣ The AI looks ahead several moves deep into the game tree.
2️⃣ It considers all possible moves and simulates the opponent’s responses.
3️⃣ The AI assigns scores to each move, aiming to maximize its own advantage and minimize the opponent’s advantage.
4️⃣ Alpha-Beta Pruning is applied to improve efficiency by eliminating unnecessary calculations.
Here's a simplified version of how the AI makes a move:
pythonCopyEditdef minimax(board, depth, alpha, beta, maximizing_player, player):
if depth == 0 or not get_valid_moves(board, player):
return evaluate_board(board, player), None
best_move = None
opponent = "B" if player == "W" else "W"
if maximizing_player:
max_eval = float('-inf')
for move in get_valid_moves(board, player):
new_board = make_move(board, move[0], move[1], player)
evaluation, _ = minimax(new_board, depth - 1, alpha, beta, False, opponent)
if evaluation > max_eval:
max_eval = evaluation
best_move = move
alpha = max(alpha, evaluation)
if beta <= alpha:
break
return max_eval, best_move
This allows the AI to plan moves ahead and play strategically rather than making random decisions.
Future Improvements
While this version of Othello is fully playable, there are several features that could enhance it further:
✔ Multiplayer Mode – Allowing two human players to compete.
✔ Difficulty Levels – Adjusting the AI's depth for different skill levels.
✔ Move Suggestions – Indicating valid moves to assist new players.
✔ Better UI/Animations – Making the game more visually appealing.
Conclusion
Building an AI-powered Othello game using Python and PyGame was an exciting challenge that combined game development and artificial intelligence. If you're interested in AI, Minimax, or board game development, this project is a great starting point!
Feel free to fork the project, improve the AI, or add new features!
🎮 Try it out today! 🚀
🔗 GitHub Repository: Othello
Subscribe to my newsletter
Read articles from Kunal Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
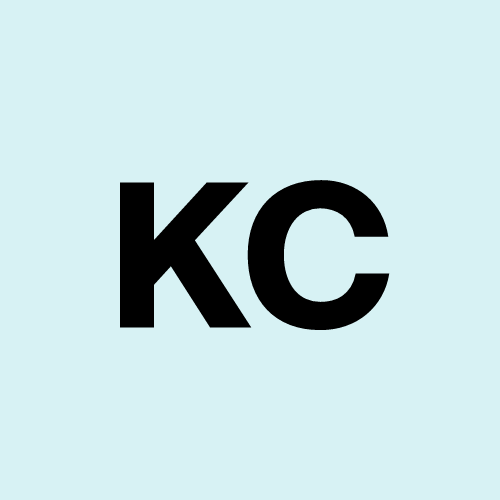