Implementing change password functionality in programmersdiary project
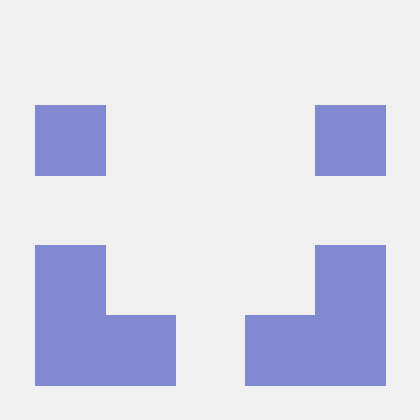
Hi. This is the twenty-fifth part of the diary about developing the “Programmers’ diary” blog. Previous part: https://hashnode.programmersdiary.com/implementing-double-submit-csrf-in-microservices. The open source code of this project is on: https://github.com/TheProgrammersDiary. The first diary entry explains why this project was done: https://medium.com/@vievaldas/developing-a-website-with-microservices-part-1-the-idea-fe6e0a7a96b5.
Next entry:
—————
2024-01-27
Let’s implement password change functionality.
Starting with TDD, we write these tests in blog microservice
@Test
void changesPassword() {
String jwt = mother
.loginNewUser("abc", "abc@gmail.com", "currentPassword")
.getHeader(HttpHeaders.SET_COOKIE)
.split("jwt=")[1]
.split(";")[0];
controller.changePassword(new UserPassword("currentPassword", "newPassword"));
controller.logout(new FakeHttpServletRequest(Map.of("Authorization", "Bearer " + jwt)));
mother.login("abc", "abc@gmail.com", "newPassword");
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
assertNotNull(authentication);
assertTrue(authentication.isAuthenticated());
}
@Test
void failsToChangePasswordWhenOldAndNewPasswordsMismatch() {
mother.loginNewUser("abc", "abc@gmail.com", "currentPassword");
assertThrows(
RuntimeException.class,
() -> controller.changePassword(
new UserPassword("wrongPassword", "newPassword")
)
);
}
@ParameterizedTest
@NullSource
@ValueSource(strings = {"", "nonEmptyPassword"})
void failsToChangePasswordWhenUsingOauth(String password) throws IOException {
loginViaOauth2(UUID.randomUUID().toString());
assertThrows(
RuntimeException.class,
() -> controller.changePassword(
new UserPassword(password, "newPassword")
)
);
}
private void loginViaOauth2(String username) throws IOException {
DefaultOidcUser user = new DefaultOidcUser(
Collections.emptyList(),
new OidcIdToken(
"fakeToken", Instant.now(), Instant.now().plus(Duration.ofMinutes(10)),
Map.of("email", UUID.randomUUID().toString(), "name", username, "sub", username)
)
);
For failsToChangePasswordWhenUsingOauth test we write parameterized tests which includes null password, empty password and non empty password. Since OAuth does not have a password stored in our side the password field is null. So a test is written to minimize changes of security breaches where someone could login with null password.
To make the test pass we start by creating a class to handle password change:
public class UserPassword {
private final String currentPassword;
private final String newPassword;
public UserPassword(String currentPassword, String newPassword) {
this.currentPassword = currentPassword;
this.newPassword = newPassword;
}
public void changePassword(UserRepository repository, String username, PasswordEncoder encoder) {
if(repository.findPasswordByUsername(username).isEmpty()) {
throw new RuntimeException("Can't change password because when using OAuth!");
}
if(!encoder.matches(currentPassword, repository.findPasswordByUsername(username).get())) {
throw new RuntimeException("Old and new passwords do not match!");
}
repository.save(
UserRepository.UserEntry.withChangedPassword(
encoder.encode(newPassword), repository.findByUsername(username).get()
)
);
}
}
One case will reject OAuth users’ password change (since again, we don’t store these users’ passwords), anotrher case will check for the case if entered password is incorrect.
Next, in UserRepository we add a query:
@Query("SELECT user.password FROM blog_user user WHERE username = :username")
Optional<String> findPasswordByUsername(String username);
and a method allowing password change:
public static UserEntry withChangedPassword(String newPassword, UserEntry userEntry) {
userEntry.password = newPassword;
return userEntry;
}
Instead of using mocks we implement a fake repo to handle the query:
@Override
public Optional<String> findPasswordByUsername(String username) {
return entries
.values()
.stream()
.filter(user -> user.getUsername().equals(username) && user.getPassword() != null)
.map(UserEntry::getPassword)
.findFirst();
}
In UserController:
@PatchMapping("/change-password")
void changePassword(@RequestBody UserPassword userPassword) {
userPassword.changePassword(
userRepository, SecurityContextHolder.getContext().getAuthentication().getName(), encoder
);
}
We use PATCH, which is similar to PUT. PUT updates the object, while PATCH updates part of an object. Since we are updated only password (and we leave username unchanged), let’s use PUT.
Lastly, we update CORS to allow a HTTP request with PATCH method:
config.setAllowedMethods(List.of("GET", "POST", "OPTIONS", "PATCH"));
Backend changes are now implemented. Since post microservice deals with posts only we don’t need to update it at all. This is a nice feature of microservices.
However, we need to update FE:
We need user icon where we will store functionality for password change. Let’s install FontAwesome using terminal: npm install --save @fortawesome/react-fontawesome @fortawesome/free-solid-svg-icons @fortawesome/fontawesome-svg-core
When the user is logged in, we will display a user icon, along with logout button:
<div className="flex items-center">
<Link href="/account" className="px-8">
<FontAwesomeIcon icon={faUser} style={{ color: "white", fontSize: 36 }} />
</Link>
<Link href="/logout" className="text-white bg-blue-700 hover:bg-blue-800 font-medium rounded-lg text-sm px-5 py-2.5 me-2 mb-2 dark:bg-blue-600 dark:hover:bg-blue-700">
Logout
</Link>
</div>
We will need to check if user logged in with our local login or with OAuth2. If user logged in via OAuth2 the password change functionality should be invisible.
Therefore, let’s rename CsrfProvider to MemoryStorage and add loginType tracking:
const [loginType, setLoginType] = useState(null);
// Other code.
<AppContext.Provider value={{ csrf, setCsrf, loginType, setLoginType }}>
Note that we could switch to a state management library like Redux, but for now MemoryStorage component is OK.
To continue, In local login page let’s set loginType as local the same way we set CSRF:
setCsrf(response.csrf);
setLoginType("local");
In Oauth login we set login type to oauth:
setCsrf(csrf);
setLoginType("oauth");
Now we can use the login type to prevent the OAuth user seeing the change password functionality. Here is the account page:
"use client"
import "../../globals.css";
import { useForm } from "react-hook-form";
import { blogUrl } from "../../next.config.js";
import { useAppContext } from "../MemoryStorage";
import { useState } from "react";
export default function Account() {
const { register, setError, handleSubmit, formState } = useForm();
const { csrf, loginType } = useAppContext();
const [responseMessage, setResponseMessage] = useState(<p></p>);
return (
<div className="relative flex flex-col items-center justify-center min-h-screen overflow-hidden">
<div className="w-full p-6 bg-white rounded-md shadow-md lg:max-w-xl">
<h1 className="text-3xl font-bold text-center text-gray-700">Account settings</h1>
{loginType == "local"
&& (
<div>
<form className="mt-6" onSubmit={handleSubmit(onSubmit)}>
<div className="mb-4">
<label
htmlFor="currentPassword"
className="block text-sm font-semibold text-gray-800"
>
Current password
</label>
<input
{...register("currentPassword", { required: true })}
name="currentPassword"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mb-4">
<label
htmlFor="repeatedCurrentPassword"
className="block text-sm font-semibold text-gray-800"
>
Repeat current password
</label>
<input
{...register("repeatedCurrentPassword", { required: true })}
name="repeatedCurrentPassword"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mb-2">
<label
htmlFor="newPassword"
className="block text-sm font-semibold text-gray-800"
>
New password
</label>
<input
{...register("newPassword", { required: true })}
name="newPassword"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mt-2">
<button className="w-full px-4 py-2 tracking-wide text-white transition-colors duration-200 transform bg-gray-700 rounded-md hover:bg-gray-600 focus:outline-none focus:bg-gray-600">
Change password
</button>
</div>
</form>
{formState.errors.repeatedCurrentPassword && (
<p className="text-red-500">{formState.errors.repeatedCurrentPassword.message}</p>
)
}
{responseMessage}
</div>
)
}
</div>
</div>
);
async function onSubmit(data, event) {
setResponseMessage(<p></p>);
event.preventDefault();
const body = { "currentPassword": data.currentPassword, "newPassword": data.newPassword };
if (data.currentPassword !== data.repeatedCurrentPassword) {
setError("repeatedCurrentPassword", {
type: "manual",
message: "Current password and repeated current password do not match.",
});
return;
}
await fetch(
blogUrl + "/users/change-password",
{
method: "PATCH",
body: JSON.stringify(body),
headers: { "Content-Type": "application/json", "X-CSRF-TOKEN": csrf },
credentials: "include"
}
As you see a simple
loginType == "local"
&&
shows the components after && only if login type is local.
With that, password change functionality is implemented.
To finish a day let’s improve the security of our service.
Let’s also add minimal error handling.
When error occurs, server data might get leaked to the client (Exception message might expose details). Therefore, if server error occurs let’s log it as error and send a message to user that something unexpected happened. If client error occurs we can send the message details to the client (e.g. if password is incorrect it’s the client error we can tell him, however if we screwed up, there could be a security issue so let’s just send HTTP code 500 with no details). Let’s go:
@ControllerAdvice
public class ControllerException {
private static final Logger log = LoggerFactory.getLogger(ControllerException.class);
@ExceptionHandler
public ResponseEntity<String> clientException(BadRequestException e) {
return clientError(e, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler
public ResponseEntity<String> clientException(RestNotFoundException e) {
return clientError(e, HttpStatus.NOT_FOUND);
}
@ExceptionHandler
public ResponseEntity<String> badCredentialsException(BadCredentialsException e) {
return clientError(e, "Bad credentials.", HttpStatus.UNAUTHORIZED);
}
private ResponseEntity<String> clientError(Exception e, HttpStatus status) {
return clientError(e, e.getMessage(), status);
}
private ResponseEntity<String> clientError(Exception e, String responseMessage, HttpStatus status) {
String errorCode = UUID.randomUUID().toString();
log.info("Client error code: {}, ", errorCode, e);
return new ResponseEntity<>(responseMessage + " Error code: " + errorCode, status);
}
@ExceptionHandler
public ResponseEntity<String> exception(Exception e) {
String errorCode = UUID.randomUUID().toString();
log.error("Error code: {}, ", errorCode, e);
return new ResponseEntity<>("Something went wrong. Error code: " + errorCode, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Using controller advice the exceptions are intercepted and modified responses are sent. Client is provided an error code so he could ask for help if he does not understand the message.
Client error classes are created like this:
public class BadRequestException extends RuntimeException {
public BadRequestException(String message) {
super(message);
}
}
These classes are created simply to mark a client error.
We can then throw a client side error in PostController:
@GetMapping(value = "/{id}")
ResponseEntity<PostRepository.PostEntry> getById(@PathVariable String id)
{
return postRepository
.findById(id)
.map(ResponseEntity::ok)
.orElseThrow(() -> new RestNotFoundException("Post with id: " + id + " not found."));
}
and handle it in FE (app/post/[id]/page.tsx)
try {
const response = await fetch(
postUrl + "/posts/" + id,
{ method: "GET", credentials: "omit" }
);
if (response.status === 404) {
const text = await response.text();
throw new Error(text);
}
const responseData = await response.json();
setArticle(responseData);
} catch (error) {
}
};
In this case a blank page without error is displayed.
Let’s also update FE onSubmit method (similar setup as in post microservice was done in blog microservice so it already throws the error on bad login):
async function onSubmit(data, event) {
event.preventDefault();
const body = { "username": data.username, "password": data.password };
try {
const response = await fetch(
blogUrl + "/users/login",
{
method: "POST",
body: JSON.stringify(body),
headers: { "Content-Type": "application/json" },
credentials: "include"
}
);
if (response.status === 401) {
const text = await response.text();
throw new Error(text);
}
const json = await response.json();
setCsrf(json.csrf);
setLoginType("local");
signIn('credentials', {
username: json.username,
redirect: false
});
router.push("/");
} catch (error) {
setLoginErrorMessage(<p className="text-red-500">{error.message}</p>);
}
}
This will no longer show Next.js error popup but instead add an error label in case user login fails:
Note: in the above I noticed in password change functionality I asked to repeat current password. Changed that to ask to repeat new password.
Let’s finish the day having implemented password change functionality and some error handling.
—————
Thanks for reading.
The project logged in this diary is open source, so if you would like to code or suggest changes, please visit https://github.com/TheProgrammersDiary.
Next part: https://hashnode.programmersdiary.com/implementing-password-reset-functionality-in-spring.
Subscribe to my newsletter
Read articles from Evaldas Visockas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
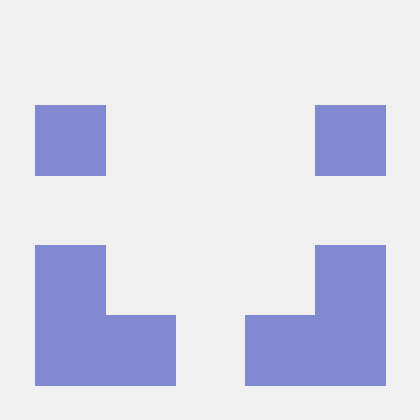