Modern Java Features: Lambdas & Streams

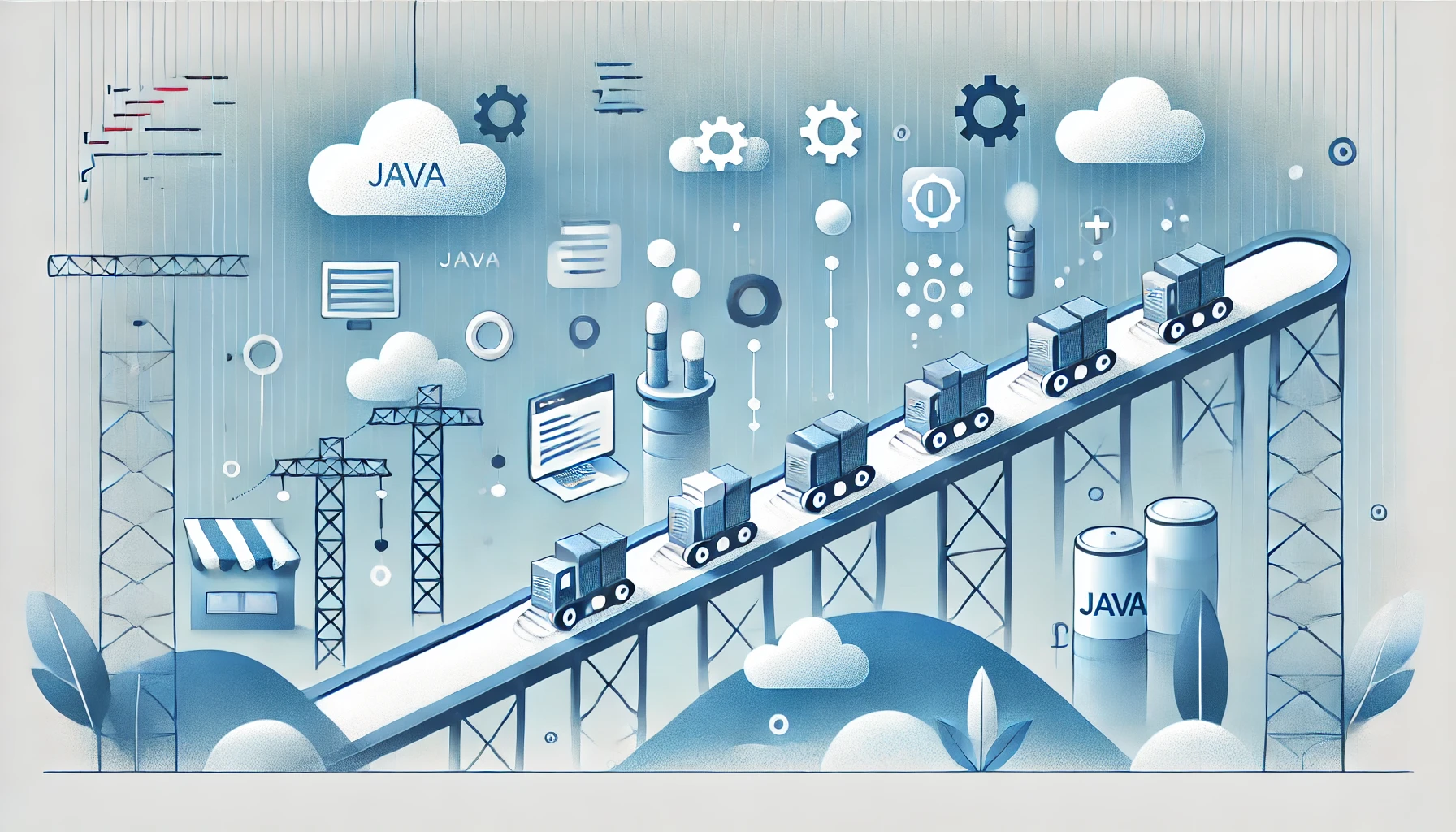
Part 1: Understanding Lambdas for Beginners
π€ Whatβs a Lambda?
A lambda expression is a short way to write anonymous functions (a function without a name):
π Basic Syntax
(parameters) -> { body }
Or shorter if it's one line:
(param) -> doSomething
Lambdas are meant to be simple and short, though you can define multi-line lambdas very often they are one-liners. When you use one-liner lambdas code tends to be more readable and cleaner. Check out this example:
β Example: Sorting with Lambdas
Before Lambdas:
Collections.sort(list, new Comparator<String>() {
public int compare(String a, String b) {
return a.compareTo(b);
}
});
With Lambdas:
Collections.sort(list, (a, b) -> a.compareTo(b));
So much cleaner!
Hereβs another example:
List<String> names = List.of("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
name -> name.startsWith("A")
is a lambda β a function that takesname
and returns a boolean.
Lambdas in Java enable functional programming by allowing you to treat behavior as data β passing functions around just like variables or objects. This leads to more concise, expressive, and modular code, especially when working with streams and callbacks.
Part 2: Getting to Know the Stream API
What is the Java Stream API?
The Java Stream API is a feature introduced in Java 8 that allows you to process collections of data in a functional and declarative style.
Instead of writing loops to iterate over data, you can use streams to filter, transform, group, or aggregate data with clean and expressive code.
β Filter - Keep only the elements that match a condition
Goal: Select items that meet a criterion (like an if
condition in a loop)
List<String> names = List.of("Alice", "Bob", "Charlie");
names.stream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
π€ Output:
Alice
β Transform (Map) convert elements into something else:
Goal: Apply a transformation to each element (like modifying values in a loop)
List<String> words = List.of("java", "stream", "lambda");
words.stream()
.map(String::toUpperCase)
.forEach(System.out::println);
π€ Output:
JAVA
STREAM
LAMBDA
β 3. Group β organize elements by a shared property
List<String> names = List.of("Maria", "Juan", "Abigail", "Pedro", "Amalia", "Pamela", "Ana");
Map<Character, List<String>> initialCharacterToNames =
names.stream().collect(Collectors.groupingBy(name -> name.charAt(0)));
System.out.println(initialCharacterToNames);
π€ Output:
{P=[Pedro, Pamela], A=[Abigail, Amalia, Ana], J=[Juan], M=[Maria]}
β 4. Aggregate (Reduce) β combine elements into a single result
Goal: Collapse a stream into one value (e.g. sum, min, max, join)
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println(sum);
π€ Output:
15
π§ Part 3: Reviewing the Java Stream Pipeline (Simplified)
Now that we've seen how streams can be used to filter, transform, group, and aggregate data, you might be wondering:
How do all these operations fit together behind the scenes?
That's where the Stream pipeline comes in. A stream pipeline is a sequence of steps through which data flows. Each step performs an operation, like filtering, transforming, or collecting.
Think of it like an assembly line: data goes in β gets processed step by step β a result comes out.
This pipeline facilitates performing various steps with the data and finalizing with a result.
β A Stream Pipeline Has 3 Parts:
Part | Description | Example |
Source | Where the data comes from (e.g. List, Set, Array) | List.of("a", "b", "c") |
Intermediate Operations | Steps that transform the data (return a Stream) | .filter(...) , .map(...) |
Terminal Operation | Ends the pipeline and returns a result | .collect(...) , .forEach(...) |
π― Example:
List<String> names = List.of("Alice", "Bob", "Charlie");
names.stream() // β
Source
.filter(name -> name.length() > 3) // π Intermediate
.map(String::toUpperCase) // π Intermediate
.forEach(System.out::println); // π Terminal
π€ Output:
ALICE
CHARLIE
β Conclusion
As you've seen, lambdas and the Stream API introduce a powerful way to write cleaner, more expressive, and more maintainable Java code. These features are a gateway to functional programming in Java β a style that leads to fewer bugs, more modular logic, and better performance in many cases.
Here are 3 solid reasons to start using them in your everyday coding:
β Cleaner, more maintainable code than traditional loops
β Encourage immutability β especially helpful in multi-threaded applications.
β Built-in support for parallel processing with
.parallelStream()
: β opening the door to faster execution with minimal effort.
Whether you're just beginning with modern Java or looking to sharpen your understanding, mastering lambdas and streams is a must.
π Stay tuned for the next part of this series, where we'll continue exploring Modern Java Features β one feature at a time.
Subscribe to my newsletter
Read articles from Mirna De Jesus Cambero directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mirna De Jesus Cambero
Mirna De Jesus Cambero
Iβm a backend software engineer with over a decade of experience primarily in Java. I started this blog to share what Iβve learned in a simplified, approachable way β and to add value for fellow developers. Though Iβm an introvert, Iβve chosen to put myself out there to encourage more women to explore and thrive in tech. I believe that by sharing what we know, we learn twice as much β thatβs precisely why Iβm here.