Serverless Forms: Leveraging FormSubmit with React
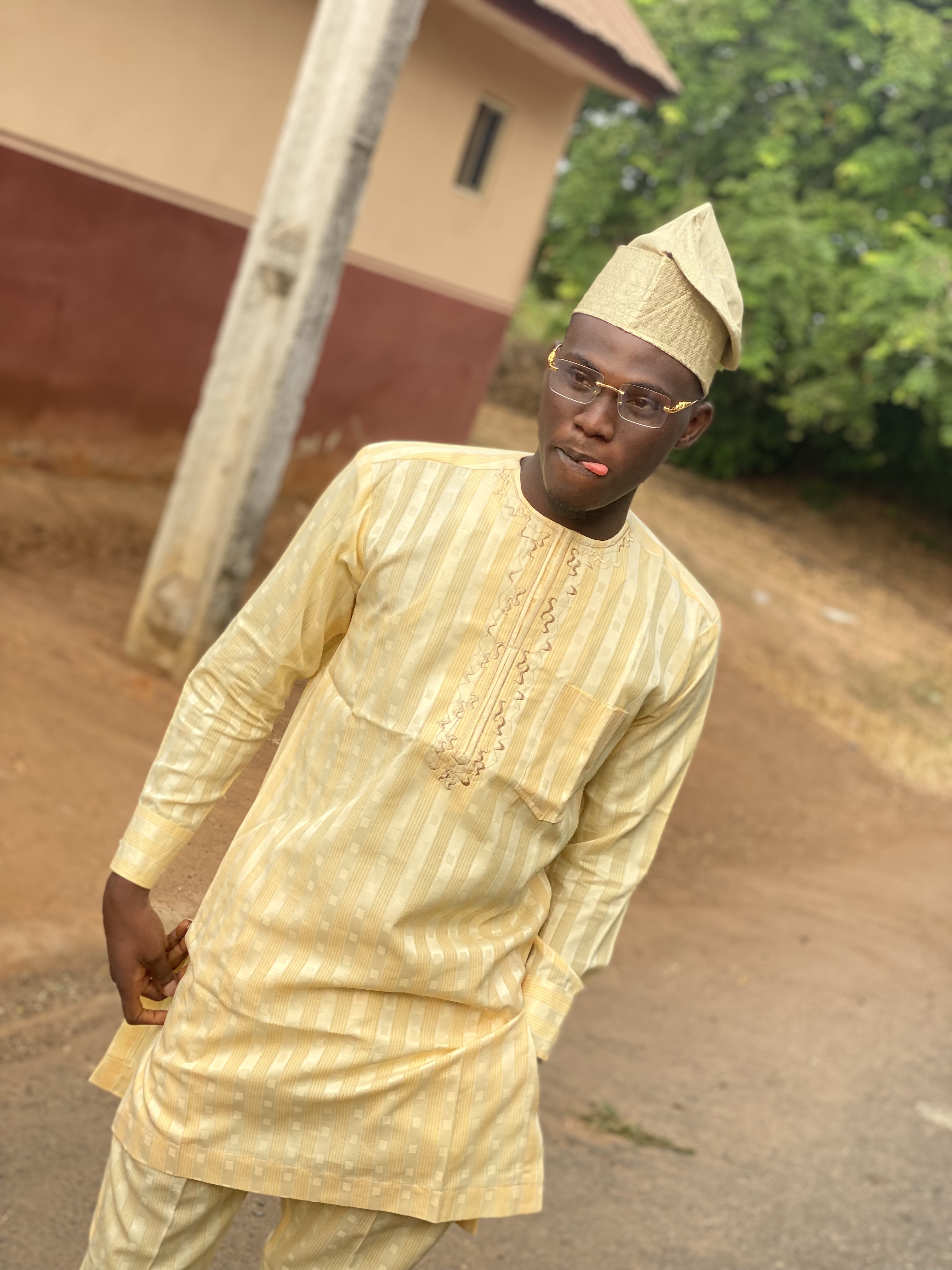
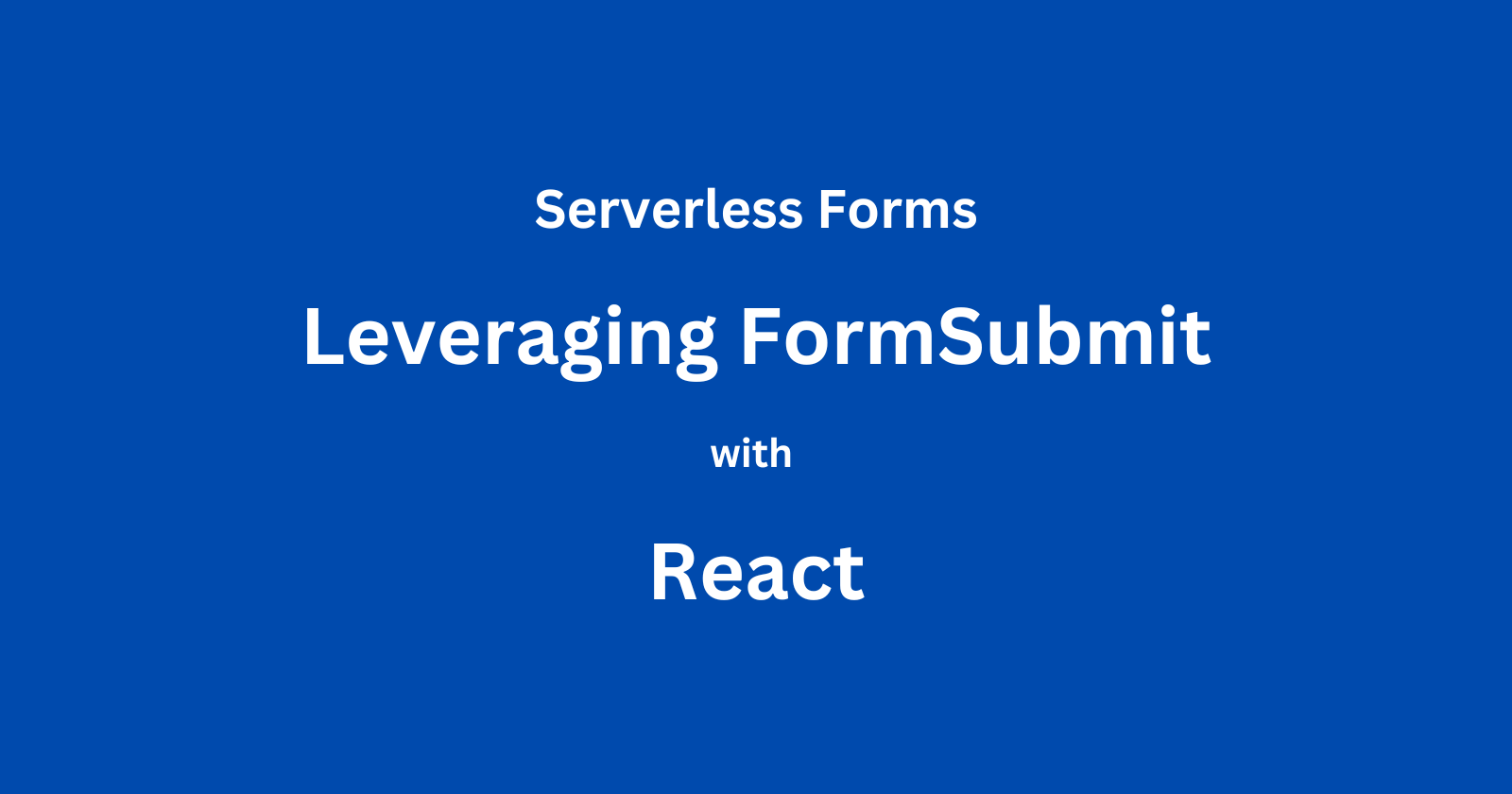
Introduction
Forms are a very important section of any website, they help collect information or data from users. Website owners utilize forms for different purposes such as completing registrations, collecting leads for their businesses, and so on. All these form data are stored on a server created and managed by the website owner. In this article, we will explore how form data can be received without creating or managing any server.
Understanding the concept of "serverless" in the context of form handling
Let's take a look at what happens behind the scenes when a form gets submitted. When a form is submitted, the browser sends the data to a server-side script which processes the information.
Depending on the form's purpose, the server-side script might be asked to store the form data in a database, process an online payment, or update a user's account information. This script runs on a server you created, managed, and maintained.
"Serverless" in the context of form handling does not imply the total absence of servers but the absence of server-side processing of form submissions. This allows developers to focus on building and integrating forms without the need to manage and maintain any backend server.
Importance of serverless forms in reducing server maintenance
Typically, handling form submissions requires maintaining a server which can be a complex task and can also be time-consuming. With serverless forms, the burden is reduced in the following ways;
Reduces server setup and configuration
Simplifies security management
Server scaling is not needed
Overview of FormSubmit as a solution
FormSubmit is a free and easy-to-set-up service that handles your forms without needing your server. It provides an API endpoint which is a special URL, that connects your form with their form endpoint and your form submissions will be sent to you via email.
It takes care of all the server-side processes, allowing developers to focus on building and integrating their forms. It also offers features like spam filtering, file upload capabilities, and so on.
Pre-requisites
Need a hosted react form with basic components and input fields.
Basic understanding of react, html forms, and javaScript (including libraries like axios).
Setting up a FormSubmit account and obtaining the API endpoint
The good thing about using FormSubmit is that you don't have to create an account to get started with FormSubmit. There is no sign-up process, you only need to obtain the API endpoint. Here's how it is done;
Visit this website, https://formsubmit.co/ajax-documentation since we will be using the axios library in this tutorial, locate and copy the unique URL highlighted in the image below;
Once you have located and copied the URL, it should look like this "https://formsubmit.co/ajax/your@email.com". You need to change the "your@email.com" to the email address you intend to use in receiving your form submissions. In this scenario, I would be using a temporary email provided by a temporary email service "ponege3067@dovesilo.com".
Finally, you have to create your unique FormSubmit API endpoint and it should look like this "https://formsubmit.co/ajax/ponege3067@dovesilo.com". With this unique API endpoint, you should be able to connect your form to FormSubmit.
Connecting your form
Creating your unique FormSubmit API endpoint is just the first step. In this section, we will go through how to connect your form to the FormSubmit endpoint using the unique API endpoint you created.
Sending form data as a POST request to the API endpoint using axios
There are other ways of sending form data as a POST request to the API endpoint but in this example, I would be using axios. Using axios allows your form data to be sent without ever making your users leave your website.
To achieve this you need to install axios using your terminal by running the code;
npm install axios
After the completion of the installation, make sure you import it into your project using the code below;
import axios from 'axios';
Next, a handleSubmit function needs to be created for handling form submission. This function uses axios to send a POST request to your API endpoint (Your unique URL).
const handleSubmit = (e) => {
e.preventDefault();
axios.defaults.headers.post['Content-Type'] = 'application/json';
axios.post('https://formsubmit.co/ajax/ponege3067@dovesilo.com', formData)
.then(response => {
console.log(response);
});
})
.catch(error => {
console.log(error);
});
};
Finally, attach handleSubmit to your form onSubmit event.
<form className="form" onSubmit={handleSubmit}>
<input
type="text"
name="firstName"
placeholder="First Name"
value={formData.firstName}
onChange={handleChange}
/>
<input
type="text"
name="lastName"
placeholder="Last Name"
value={formData.lastName}
onChange={handleChange}
/>
<input
type="email"
name="email"
placeholder="Email"
value={formData.email}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
When the form gets submitted, the handleSubmit function gets triggered, which sends the form data to your API endpoint using axios.
Handling success and error responses
Handling success and error responses can be done through the handleSubmit function that was created initially.
const handleSubmit = (e) => {
e.preventDefault();
axios.defaults.headers.post['Content-Type'] = 'application/json';
axios.post('https://formsubmit.co/ajax/ponege3067@dovesilo.com', formData)
.then(response => {
console.log(response);
//To handle success reponses (e.g., show success message)
});
})
.catch(error => {
console.log(error);
//To handle error responses (e.g., show error message)
});
};
Let's take for instance, for a success response, I would like to reset my form each time it gets submitted. Here's how it going to be done;
const handleSubmit = (e) => {
e.preventDefault();
axios.defaults.headers.post["Content-Type"] = "application/json";
axios
.post("https://formsubmit.co/ajax/ponege3067@dovesilo.com", formData)
.then((response) => {
console.log(response);
// Reset form values
setFormData({
firstName: "",
lastName: "",
email: "",
});
})
.catch((error) => {
console.log(error);
// handle error (e.g., show error message)
});
};
It can also be a simple success or error message depending on what project you're working on.
Once you are done setting up your code and getting your project ready, you need to activate your form, which is linking your form with FormSubmit, via the email you would be using in receiving your form submissions and yes, this is a one-time activation. Here's how it is done;
Open up your react form, input any random data into the form, and click on the submit button.
After the submission, you should receive an email in a minute or two. Open it up and click on the "activate form" button.
Immediately after activating your form, you should receive the details you inputted into your form initially.
Output
Note: In the output above, the form reset feature was used as the form success response.
Exploring Hidden Fields as an Advanced Feature
FormSubmit has various advanced features that can be used as extra customization for your form. One of the advanced features offered by FormSubmit is hidden fields.
Hidden fields are fields in your code that are invisible to the users of your forms but they are accessed and used during form processing. FormSubmit uses these hidden fields to perform various functions.
I will be going through a few of them in this article and how to integrate them into your form submission code.
Setting email subjects
This is simply setting up the subject line for your email notification. Here's how to integrate it into your code;
First, you need to add _subject: 'The subject line of your choice', to your form data object. Here is what it should look like;
const Form = () => {
const [formData, setFormData] = useState({
firstName: '',
lastName: '',
email: '',
_subject: "You've got a new submission",
});
Then, add the hidden input field <input type="hidden" name="_subject" value={formData._subject} /> in between your form tags <form>...<form/>. Here is what it should look like;
<form className="form" onSubmit={handleSubmit}>
<input type="hidden" name="_subject" value={formData._subject} />
</form>
Redirect URLs
Depending on what your form is meant for, you can redirect your users to another website or another page on your website (thank-you page or confirmation page) after they submit a form. Either way, you can use FormSubmit hidden inputs to get it done. Here is how it is done;
Just like the first example, you need to add _next: 'https://yourdomain.co/thanks.html' to your form data object. Here is what it should look like;
const Form = () => {
const [formData, setFormData] = useState({
firstName: '',
lastName: '',
email: '',
_next: 'https://yourdomain.co/thanks.html',
});
Next, add the hidden input field <input type="hidden" name="_next" value={formData._next} /> in between your form tags <form>...<form/>. Here is what it should also look like;
<form className="form" onSubmit={handleSubmit}>
<input type="hidden" name="_next" value={formData._next} />
</form>
CC addresses
This hidden field allows you to send a copy of your form submissions to another email address. Here is how you can integrate this into your code;
Add _cc: 'another@gmail.com' to your form data object.
const Form = () => {
const [formData, setFormData] = useState({
firstName: '',
lastName: '',
email: '',
_cc: 'another@gmail.com',
});
Finally, add the hidden input field <input type="hidden" name="_cc" value={formData._cc} /> in between your form tags <form>...<form/>.
<form className="form" onSubmit={handleSubmit}>
<input type="hidden" name="_cc" value={formData._cc} />
</form>
File uploads
Just incases whereby you want to collect information such as images, documents, and so on. FormSubmit allows your users to upload files to your form but it is unfortunately limited.
At the moment, FormSubmit ajax endpoint doesn't support file uploads. The method directly offered by FormSubmit for file uploads won't work with a react form because react typically handles form submissions with ajax for a smoother user experience.
The method offered by FormSubmit at the moment supports only HTML forms. Here is how it can be integrated into an HTML form;
You need to add the following attribute to the form tag method = POST, action="https://formsubmit.co/your@email.com" and enctype="multipart/form-data".
<form method="POST" action="https://formsubmit.co/your@email.com" enctype="multipart/form-data">
<input type="email" name="email" placeholder="Your email">
<textarea name="message" placeholder="Details of your problem"></textarea>
<input type="file" name="attachment" accept="image/png, image/jpeg">
<button type="submit">Send Test</button>
</form>
The html form code above uses the FormSubmit standard endpoint https://formsubmit.co/your@email.com (not the ajax endpoint) to which the form data would be sent.
Conclusion
Apart from the limitation surrounding the integration of FormSubmit with react, it is still a very reliable choice when it comes to handling form submissions because it is easy to integrate with react forms and it delivers form data fast.
Subscribe to my newsletter
Read articles from David Kehinde Emmanuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
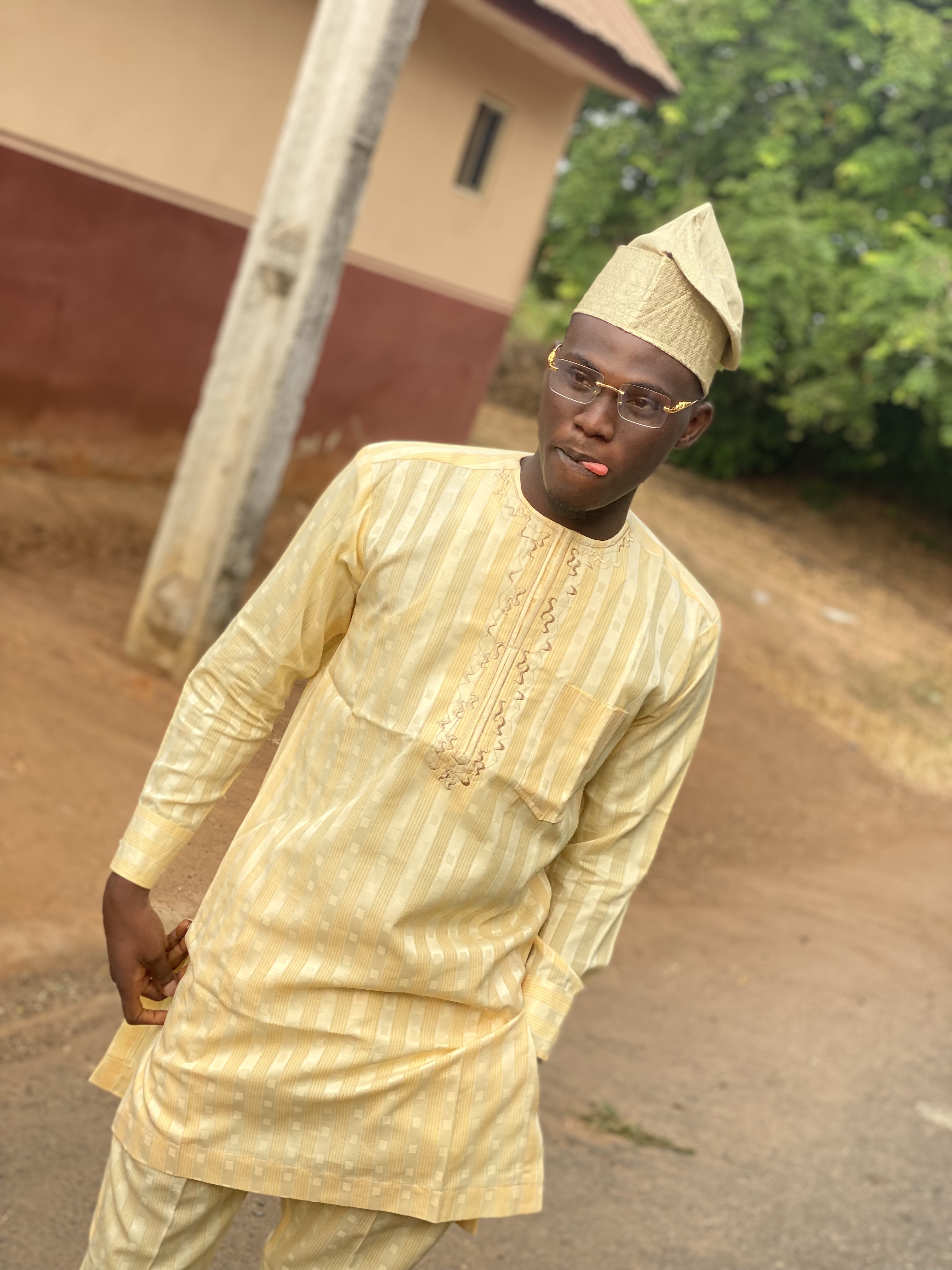
David Kehinde Emmanuel
David Kehinde Emmanuel
Hello, My name is Kehinde David and I am a front-end web developer and technical writer with a passion for creating engaging and effective user experiences. I have a strong background in HTML, CSS, JavaScript, and various front-end frameworks, and skilled in developing responsive and accessible websites that meet modern web standards. I'm here to improve my communication and technical writing skills, so I can able to document complex technical concepts in a clear and concise manner, making it easier for others to understand and utilize them. I enjoy staying up-to-date with the latest web technologies and trends, and I am always looking for new and innovative ways to improve user experiences.