Mastering Iterators: Comprehensive Insights You Need
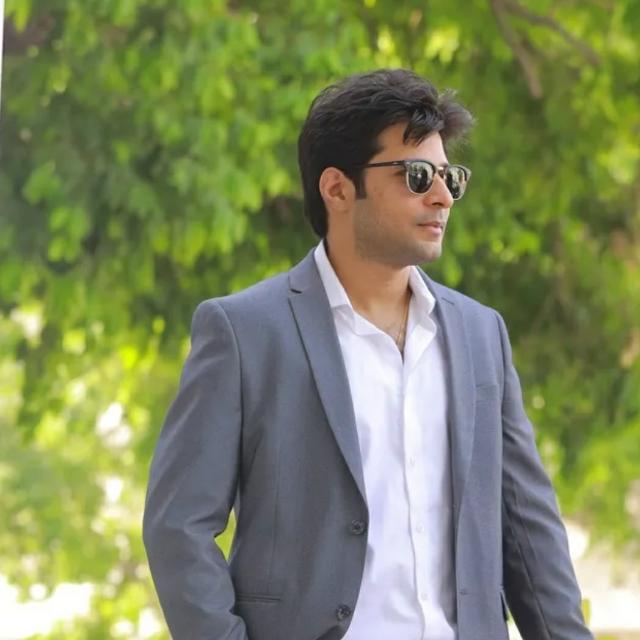
Table of contents
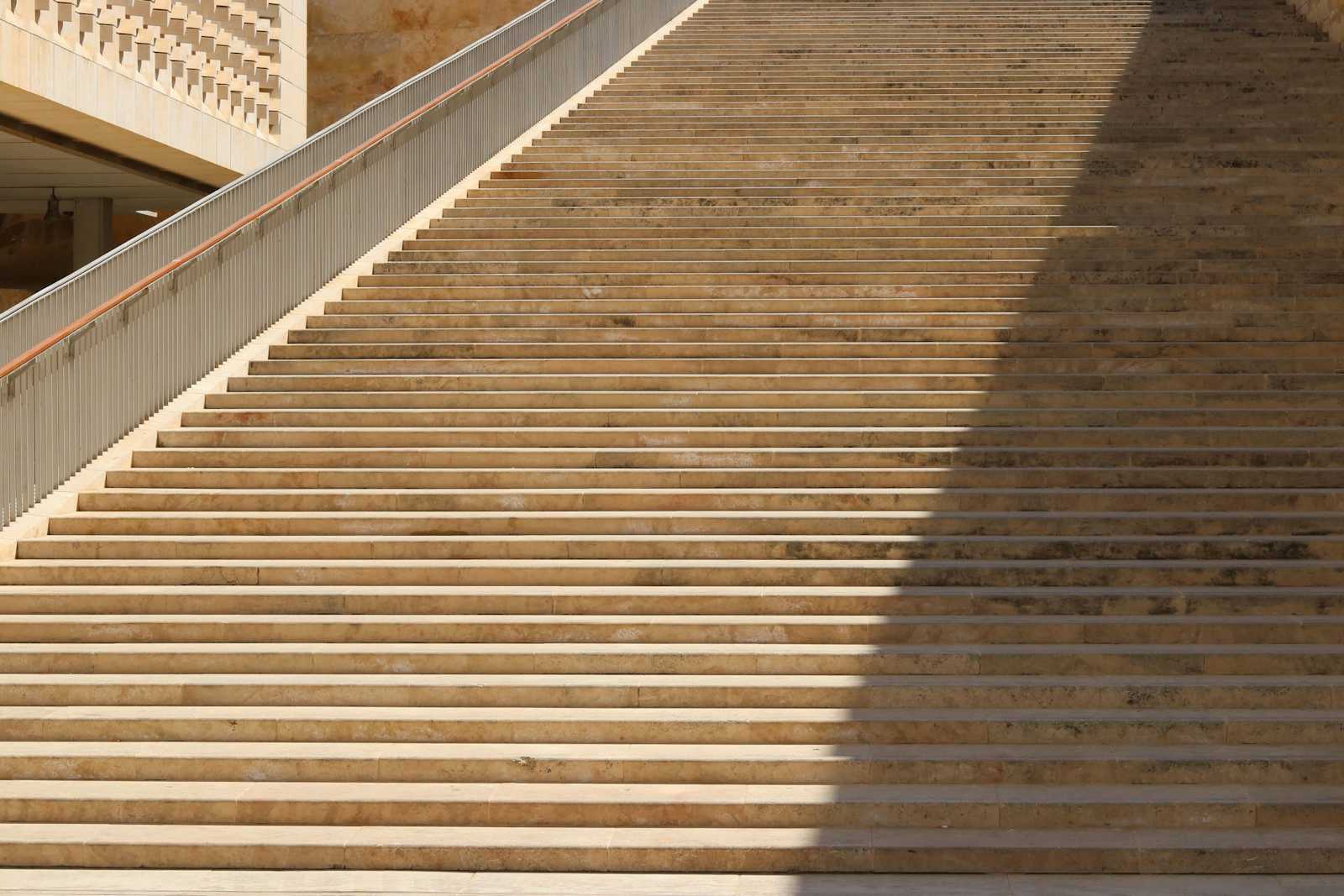
Iterators are a fundamental concept in programming that enable you to process a sequence of items, such as elements in a collection, one at a time, without revealing the collection's internal structure. They form the backbone of operations like looping, filtering, and mapping over collections. When you encounter constructs like these, you can liken them to functional or stream-based idioms found in languages such as C# or Java.
When you see something like:
collection.iter()
.map(...)
.filter(...)
.take(...)
.collect();
Why Iterators Are Useful
Abstraction: They hide the underlying container’s implementation. You don’t need to know if it’s an array, a linked list, or some more specialized structure.
Safety / Encapsulation: With an iterator, you typically avoid directly indexing into a collection’s memory. This reduces off-by-one errors, out-of-bounds errors, or concurrency issues.
Composability: Iterators can be transformed, filtered, and combined to express complex operations in a clean, functional style.
Iterators in Rust
Rust’s iterator system is powerful and versatile, offering developers a robust toolset for managing collections efficiently. It simplifies the code and reduces the potential for errors. This not only makes the code more readable but also aligns with functional programming paradigms, making it easier for developers familiar with languages like C# or Java to adapt.
They Are Lazy
In Rust, when you call methods like
.map()
,.filter()
, or.take()
on an iterator, it does not immediately create a new collection. Instead, these methods return a new iterator that, when advanced, processes items on demand.This means you can build up chained iterator calls without incurring overhead until you actually consume the iterator (e.g., in a for loop, or when calling a terminal method like
.collect()
).
Three Main “Iterator” Traits
Iterator
: The trait for iterators that produce items by value. Typically you implement this when you want to define how to produce a sequence of items.IntoIterator
: For types (likeVec
, slices, and so on) that can be converted into an iterator.DoubleEndedIterator
: An extension that allows “reverse” iteration (calling.next_back()
in addition to.next()
from the front).
Ownership, Borrowing, and Lifetimes
Rust’s type system enforces rules to ensure safety. For iterators, this means that when you iterate over a collection, either you move (take ownership of) the collection or you borrow from it in a well-defined way.
iter()
borrows each element by reference.into_iter()
consumes (takes ownership of) the elements, so the iterator yields the items by value.iter_mut()
borrows each element mutably, allowing you to modify items in place.
Common Methods
map(f)
: Apply a functionf
to each item.filter(pred)
: Keep only items wherepred(item)
istrue
.fold(init, f)
: Accumulate items into a single value, starting frominit
, applyingf
in a fold/reduction manner.collect()
: Consume an iterator and gather the items into a collection (like aVec
,HashSet
, etc.).take(n)
,skip(n)
,enumerate()
, etc.: Additional combinators for slicing, offset, or enumerating.
Consuming vs. Adapting Iterators
In Rust’s library documentation, you might see a distinction between “consuming” vs. “adapting” iterators:Consuming: These methods take ownership of the iterator to produce a final value or another data structure (e.g.,
collect()
,fold()
). Once consumed, the iterator cannot be used again.Adapting: These methods produce a new iterator (e.g.,
map
,filter
). These can be chained before any final consumption.
Examples
Below is some "cookbook"-style collection of common and practical iterator patterns in Rust. This will help you become more comfortable and familiar with iterators, allowing you to later explore them in greater depth.
1. Iterate and Collect into a New Vector
A basic example: transform and collect.
fn example_collect() {
let numbers = vec![1, 2, 3, 4, 5];
// Double each number and collect into a new Vec<i32>
let doubled: Vec<i32> = numbers.iter().map(|x| x * 2).collect();
println!("{:?}", doubled); // Output: [2, 4, 6, 8, 10]
}
iter()
borrows each element (so x
is &i32
). map(|x| x * 2)
applies a function to each element. |x| x * 2
is closure. collect()
finalizes the lazy chain, producing the Vec<i32>
.
2. Filter Items Based on a Condition
Use filter
to keep only items meeting a criterion.
fn example_filter() {
let numbers = vec![10, 15, 20, 25, 30];
// Keep only multiples of 10
let multiples_of_ten: Vec<i32> = numbers
.into_iter() // Now x is i32, not &i32
.filter(|x| x % 10 == 0)
.collect();
println!("{:?}", multiples_of_ten); // Output: [10, 20, 30]
}
into_iter()
consumes numbers
and yields i32
(by value). filter(...)
returns a new iterator keeping only those items where the predicate is true.
3. Filter and Map in One Step: filter_map
Sometimes you want to transform items and discard certain items altogether. filter_map
combines filter
and map
in one step, working with Option
.
let inputs = vec!["42", "93", "hello", "128", "xyz"];
// Try parsing each string as an integer.
// If successful, keep the integer; otherwise, skip.
let parsed: Vec<i32> = inputs
.iter()
.filter_map(|s| s.parse::<i32>().ok())
.collect();
// parsed: [42, 93, 128]
s.parse::<i32>().ok()
returns Some(i32)
if parse succeeded, or None
otherwise. filter_map
only yields Some
items.
4. Combine Multiple Iterators: chain
Use chain
to create a single iterator that yields items from two or more sources sequentially.
let nums1 = vec![1, 2, 3];
let nums2 = vec![4, 5, 6];
let chained: Vec<i32> = nums1
.into_iter()
.chain(nums2.into_iter())
.collect();
// chained: [1, 2, 3, 4, 5, 6]
chain
takes two iterators and iterates over the first fully, then the second. In this example, nums1
and nums2
are both consumed (moved) by into_iter()
.
5. Enumerate Items with enumerate
Sometimes you need both the index and the value.
let animals = vec!["cat", "dog", "bird"];
for (index, animal) in animals.iter().enumerate() {
println!("{}: {}", index, animal);
}
// Output:
// 0: cat
// 1: dog
// 2: bird
enumerate()
yields (index, item)
pairs. The index
starts at 0 by default.
6. Find the First Matching Item: find
The find
method returns the first item that satisfies a condition (as an Option
).
let numbers = vec![1, 3, 5, 7, 9];
// Find the first number that is divisible by 3
if let Some(found) = numbers.iter().find(|&&x| x % 3 == 0) {
println!("Found: {}", found); // Output: Found: 3
} else {
println!("No match found");
}
find
stops searching after the first match. Returns None
if no match is found.
7. Take While a Condition is True: take_while
Use take_while
to yield items only as long as a predicate holds. (Note: This is available via Iterator::take_while
in stable Rust 1.52+.)
let numbers = vec![2, 4, 6, 8, 10, 1, 12];
// Take only even numbers until we reach the first odd
let evens_up_to_odd: Vec<i32> = numbers
.into_iter()
.take_while(|x| x % 2 == 0)
.collect();
println!("{:?}", evens_up_to_odd); // Output: [2, 4, 6, 8, 10]
Once take_while
sees a value that doesn’t satisfy the predicate, it stops altogether.
8. Accumulating/Reducing: fold
Use fold
to accumulate values into a single result. This is often used for sums, products, or more advanced aggregations.
fn example_fold() {
let numbers = vec![1, 2, 3, 4, 5];
let sum = numbers.iter().fold(0, |acc, &x| acc + x);
println!("Sum is {}", sum); // Output: 15
let product = numbers.iter().fold(1, |acc, &x| acc * x);
println!("Product is {}", product); // Output: 120
}
The first argument to fold
is the initial accumulator value. The closure receives the accumulator and the next item.
9. Partition Items into Two Groups: partition
partition
splits items into two collections based on a condition, returning (Vec<T>, Vec<T>)
.
fn example_partition() {
let numbers = vec![1, 2, 3, 4, 5, 6];
let (even, odd): (Vec<i32>, Vec<i32>) = numbers
.into_iter()
.partition(|x| x % 2 == 0);
println!("Even: {:?}", even); // Even: [2, 4, 6]
println!("Odd: {:?}", odd); // Odd: [1, 3, 5]
}
The first Vec
collects items where the predicate is true, and the second collects items where it is false.
10. Zip Two Iterators Together: zip
zip
pairs items from two iterators into (item1, item2)
tuples.
fn example_zip() {
let letters = vec!['a', 'b', 'c'];
let numbers = vec![1, 2, 3, 4];
// Zip together into pairs
let zipped: Vec<(char, i32)> = letters.into_iter().zip(numbers).collect();
println!("{:?}", zipped); // Output: [('a', 1), ('b', 2), ('c', 3)]
}
The iteration stops when the shortest iterator ends. In this example, letters
has 3 items, numbers
has 4, so only 3 pairs are created.
11. Flatten an Iterator of Iterators: flatten
If you have nested iterators (e.g., a Vec<Vec<T>>
), you can flatten it into a single sequence.
fn example_flatten() {
let nested = vec![vec![1, 2], vec![3, 4, 5], vec![6]];
let flattened: Vec<i32> = nested.into_iter().flatten().collect();
println!("{:?}", flattened); // Output: [1, 2, 3, 4, 5, 6]
}
flatten
automatically iterates through each sub-iterator/item. You can also use flat_map
if you need to transform and flatten in one step.
12. Skipping Elements or Taking a Specific Count: skip
/ take
Use skip
to ignore a certain number of items, take
to limit iteration to a certain count.
fn example_skip_take() {
let numbers = vec![10, 20, 30, 40, 50, 60];
// Skip the first 2, then take the next 3
let slice: Vec<i32> = numbers
.iter()
.skip(2)
.take(3)
.copied() // because we had .iter() -> &i32
.collect();
println!("{:?}", slice); // Output: [30, 40, 50]
}
skip(n)
ignores the first n
items. take(n)
yields only the next n
items, then stops. .copied()
turns &i32
into i32
; you could also use .cloned()
or just leave them as references if that’s acceptable.
IntoIterator Trait
IntoIterator
is a trait that defines how a type can be converted into an iterator. It's one of the fundamental traits in Rust's collections and iteration system.
pub trait IntoIterator {
type Item;
type IntoIter: Iterator<Item = Self::Item>;
fn into_iter(self) -> Self::IntoIter;
}
This trait has three key components:
Item
: The type of item that the iterator will produceIntoIter
: The specific iterator type that will be returnedinto_iter()
: The method that consumes the collection and returns an iterator.
How IntoIterator Is Used
For loops: When you write a for
loop in Rust, the compiler automatically calls into_iter() on the collection you're iterating over:
for element in collection {
// This is actually doing: for element in collection.into_iter() { ... }
}
When you write a for
loop or manually call collection.into_iter()
, Rust decides which implementation to call based on whether you’re iterating over the collection by value (collection.into_iter()
), by reference ((&collection).into_iter()
), or by mutable reference ((&mut collection).into_iter()
).
Converting collections to iterators:
You can explicitly call into_iter() to convert a collection into an iterator:
let vec = vec![1, 2, 3];
let iter = vec.into_iter(); // Consumes vec, returns an iterator
Different Implementations
Most collections in Rust implement IntoIterator
in multiple ways:
For the collection itself (by value): Consumes the collection, returning an iterator that takes ownership of the elements:
impl<T> IntoIterator for Vec<T> {
type Item = T;
type IntoIter = std::vec::IntoIter<T>;
fn into_iter(self) -> Self::IntoIter { /* ... */ }
}
For references to the collection (&): Creates an iterator that borrows the elements:
impl<'a, T> IntoIterator for &'a Vec<T> {
type Item = &'a T;
type IntoIter = std::slice::Iter<'a, T>;
fn into_iter(self) -> Self::IntoIter { /* ... */ }
}
For mutable references (&mut): Creates an iterator that mutably borrows the elements:
impl<'a, T> IntoIterator for &'a mut Vec<T> {
type Item = &'a mut T;
type IntoIter = std::slice::IterMut<'a, T>;
fn into_iter(self) -> Self::IntoIter { /* ... */ }
}
std::slice::Iter<'a, T>
, std::slice::IterMut<'a, T>
and std::vec::IntoIter<T>
are different iterator types you're seeing in Rust. Each one corresponds to a different way of iterating over a collection, based on ownership and mutability.
Understanding into_iter() and Ownership
The into_iter()
method can handle different ownership patterns, making Rust's iteration system super flexible. Let's discuss how it works with values, references, and mutable references, and how iter()
fits into this system.
How into_iter() works with different ownership types
The into_iter()
method behaves differently depending on whether you call it on:
Value (T): Consumes the collection, taking ownership
Reference (&T): Borrows the collection immutably
Mutable reference (&mut T): Borrows the collection mutably
This is achieved through separate implementations of the IntoIterator
trait for each case.
// For Vec<T> as an example:
// Takes ownership (consumes the Vec)
impl<T> IntoIterator for Vec<T> {
type Item = T; // Iterator yields owned values
// ...
}
// Borrows immutably
impl<'a, T> IntoIterator for &'a Vec<T> {
type Item = &'a T; // Iterator yields references
// ...
}
// Borrows mutably
impl<'a, T> IntoIterator for &'a mut Vec<T> {
type Item = &'a mut T; // Iterator yields mutable references
// ...
}
Examples:
let v = vec![1, 2, 3];
// Takes ownership - consumes v
let iter1 = v.into_iter(); // Iterator yields values (T)
// v is no longer usable here
let v = vec![1, 2, 3];
// Borrows immutably
let iter2 = (&v).into_iter(); // Iterator yields references (&T)
// v is still usable here
// Borrows mutably
let mut v = vec![1, 2, 3];
let iter3 = (&mut v).into_iter(); // Iterator yields mutable references (&mut T)
// v is still usable after iter3 is dropped
// Standard collection methods
let v = vec![1, 2, 3];
let iter_a = v.iter(); // Always yields &T
let iter_b = v.iter_mut(); // Always yields &mut T
let iter_c = v.into_iter(); // Consumes v, yields T
The flexibility of into_iter()
is why for
loops in Rust work with all three ownership models. The compiler automatically chooses the appropriate implementation based on how you use the collection in the loop.
Iterator Trait
The Iterator
trait provides a way to process sequences of items one at a time, and it serves as the foundation for many functional programming patterns. Iterator
and IntoIterator
are two related but different traits that help in working with collections and iterators.
Iterator
is used when you already have an iterator and want to iterate over it. The next()
method is used to fetch elements one by one.
Example:
let numbers = vec![1, 2, 3];
let mut iter = numbers.iter(); // Create an iterator over `numbers`
println!("{:?}", iter.next()); // Some(1)
println!("{:?}", iter.next()); // Some(2)
println!("{:?}", iter.next()); // Some(3)
println!("{:?}", iter.next()); // None (end of iteration)
numbers.iter()
creates an iterator and calling next()
moves through the elements.
Basic Structure
trait Iterator {
type Item;
fn next(&mut self) -> Option<Self::Item>;
// Many default methods omitted...
}
The trait has only one required method to implement: next()
. Everything else is built on top of this foundation.
Core Components
Associated Type
Item
: Defines what type of elements the iterator will produce.next()
Method: The heart of the iterator pattern.Returns
Some(item)
if there's another item in the sequenceReturns
None
when iteration is completeTakes
&mut self
because advancing the iterator changes its internal state
Implementing Iterator
Here's a simple example of implementing the Iterator
trait:
struct Fibonacci {
curr: u32,
next: u32,
}
impl Iterator for Fibonacci {
type Item = u32;
fn next(&mut self) -> Option<Self::Item> {
let current = self.curr;
self.curr = self.next;
self.next = current + self.next;
Some(current)
}
}
// Create an iterator that generates the Fibonacci sequence
fn fibonacci() -> Fibonacci {
Fibonacci { curr: 0, next: 1 }
}
let fib = fibonacci();
let first_10: Vec<u32> = fib.take(10).collect();
Relationship to IntoIterator
Iterator
defines how to iterate through a sequence. IntoIterator
defines how to create an iterator from a value. These two work hand in hand to make a full iteration system. The IntoIterator::into_iter()
method creates an Iterator
, and when you use a for
loop, it automatically uses IntoIterator
behind the scenes.
This design allows for flexibility and powerful abstractions across Rust's standard library and ecosystem. The combination of these traits enables many of Rust's most elegant patterns for working with collections and sequences.
The iter() Method vs. into_iter()
The iter()
method is not syntactic sugar for &collection.into_iter()
, but they're closely related.
iter()
is a method implemented specifically for collections in the standard library, while into_iter()
comes from the IntoIterator
trait. They serve similar purposes but work differently:
let v = vec![1, 2, 3];
// These two are equivalent:
let iter1 = v.iter();
let iter2 = (&v).into_iter();
// Both produce iterators over &T (references)
Important Distinctions:
iter()
is a method found directly on collection types like Vec and HashMap, whileinto_iter()
comes from theIntoIterator
trait.iter()
always borrows the collection and never consumes it, whereasinto_iter()
might consume the collection, depending on whether it's called on a value or a reference.iter()
always gives you an iterator over references, whileinto_iter()
behaves differently based on how you use it. Anditer_mut()
always provides an iterator over mutable references.
Full Example
Below is a detailed example of a custom Portfolio
struct, which contains a Vec<String>
. This example includes three distinct implementations of the IntoIterator
trait, each designed to allow different types of iteration over the Portfolio
:
Consuming iteration: This type of iteration occurs by value, meaning it takes ownership of the elements. It yields owned
String
values, effectively consuming thePortfolio
as it iterates through its elements. This is useful when you need to take ownership of the data for further processing or transformation.Shared iteration: This iteration happens by shared reference, which means it does not take ownership of the elements. Instead, it yields
&String
, allowing you to read the data without modifying it. This is ideal for scenarios where you need to access the data without altering the originalPortfolio
.Mutable iteration: This iteration is performed by mutable reference, yielding
&mut String
. It lets you change the elements of thePortfolio
as you go through them. This is especially useful when you need to update or transform the data right where it is.
For each of these iteration types, we'll show how they work with a custom iterator type: PortfolioIntoIter
, PortfolioIter
, and PortfolioIterMut
. Each of these custom iterator types implements the Iterator
trait, giving you the tools you need to go through the Portfolio
in the way you want. This setup not only highlights the flexibility of Rust's iteration patterns but also shows how you can create custom iterators to fit your specific needs.
#[derive(Debug)]
struct Portfolio {
instruments: Vec<String>,
}
// An iterator for consuming `Portfolio` (yields owned `String`)
struct PortfolioIntoIter {
inner: std::vec::IntoIter<String>,
}
// An iterator for `&Portfolio` (yields `&String`)
struct PortfolioIter<'a> {
inner: std::slice::Iter<'a, String>,
}
// An iterator for `&mut Portfolio` (yields `&mut String`)
struct PortfolioIterMut<'a> {
inner: std::slice::IterMut<'a, String>,
}
// Constructor for convenience
impl Portfolio {
fn new(instruments: Vec<String>) -> Self {
Portfolio { instruments }
}
}
Now, let's delve into implementing the three distinct variants of the IntoIterator
trait for our custom iterator types.
// 1) By value (Portfolio -> owned iteration)
impl IntoIterator for Portfolio {
type Item = String;
type IntoIter = PortfolioIntoIter;
fn into_iter(self) -> Self::IntoIter {
PortfolioIntoIter {
// Move out the vector’s own iterator
inner: self.instruments.into_iter(),
}
}
}
// 2) By shared reference (&Portfolio -> shared iteration)
impl<'a> IntoIterator for &'a Portfolio {
type Item = &'a String;
type IntoIter = PortfolioIter<'a>;
fn into_iter(self) -> Self::IntoIter {
PortfolioIter {
// Borrow the underlying vector’s iterator
inner: self.instruments.iter(),
}
}
}
// 3) By mutable reference (&mut Portfolio -> mutable iteration)
impl<'a> IntoIterator for &'a mut Portfolio {
type Item = &'a mut String;
type IntoIter = PortfolioIterMut<'a>;
fn into_iter(self) -> Self::IntoIter {
PortfolioIterMut {
// Borrow the underlying vector’s mutable iterator
inner: self.instruments.iter_mut(),
}
}
}
Now, let's proceed to implement the Iterator
trait for each of our custom iterator types. This step is crucial because it defines how each iterator will behave when traversing through the elements of the Portfolio
. By implementing the Iterator
trait, we specifically implement next()
for different iterators to advance the iterator and yield the value.
impl Iterator for PortfolioIntoIter {
type Item = String;
fn next(&mut self) -> Option<Self::Item> {
self.inner.next()
}
}
impl<'a> Iterator for PortfolioIter<'a> {
type Item = &'a String;
fn next(&mut self) -> Option<Self::Item> {
self.inner.next()
}
}
impl<'a> Iterator for PortfolioIterMut<'a> {
type Item = &'a mut String;
fn next(&mut self) -> Option<Self::Item> {
self.inner.next()
}
}
Example usage in main() where we create a Portfolio
instance and iterate over its elements using the different iterator implementations we have defined above.
fn main() {
// 1) By value: move the Portfolio and get owned Strings
let portfolio = Portfolio::new(vec!["AAPL".into(), "GOOG".into(), "MSFT".into()]);
println!("Iterate by value:");
for instrument in portfolio.into_iter() {
// instrument is String (owned)
println!("{}", instrument);
}
// `portfolio` is consumed here—can't use it again.
// 2) By shared reference: get &String
let portfolio_ref = Portfolio::new(vec!["TSLA".into(), "AMZN".into(), "META".into()]);
println!("\nIterate by shared reference:");
for instrument in &portfolio_ref {
// instrument is &String
println!("{}", instrument);
}
// `portfolio_ref` is still usable (not consumed).
// 3) By mutable reference: get &mut String
let mut portfolio_mut = Portfolio::new(vec!["NFLX".into(), "DIS".into()]);
println!("\nIterate by mutable reference:");
for instrument in &mut portfolio_mut {
// instrument is &mut String
instrument.push_str(" (edited)");
}
println!("{:?}", portfolio_mut);
}
Output
With this setup, the same .into_iter()
method name can produce three different iterator types depending on the context of how you call it (owned, immutable reference, or mutable reference)—exactly like Vec<T>
does in the standard library.
Iterators Without Boilerplate: successors
Instead of writing a custom iterator, you can define your sequence generation logic inline with just a closure using successors. successors
is a function in the Rust standard library (std::iter
) that creates an iterator from an initial value and a “successor function.” Formally:
pub fn successors<T, F>(first: Option<T>, succ: F) -> Successors<T, F>
where
F: FnMut(&T) -> Option<T>,
first
is an Option<T>
– your starting point (may or may not exist) and succ
is a closure or function that, given a reference to the most recently yielded value, returns the next value as an Option<T>
.
The iterator produced will:
Yield the
first
value if it’sSome(...)
.Then repeatedly call
succ
on the last yielded value to get the next.Stop once
succ
returnsNone
.
This means you can generate a sequence on-the-fly without building your own struct
and implementing Iterator
manually.
If you like chaining and pipelining, successors
fits right in. You can follow it with methods like .map(...)
, .filter(...)
, etc., and then .take(...)
or .collect()
as needed.
Here’s a simple example that starts from Some(1)
and then keeps adding 1 until we decide to stop at a certain condition.
use std::iter::successors;
fn main() {
let mut iter = successors(Some(1), |&prev| {
let next = prev + 1;
if next <= 5 {
Some(next)
} else {
None
}
});
// Collect into a vector
let values: Vec<i32> = iter.collect();
println!("{:?}", values); // [1, 2, 3, 4, 5]
}
We pass Some(1)
as the first value, so 1
is yielded immediately when iteration begins. For each subsequent item, we apply the closure |&prev| { ... }
. The iterator yields 1, 2, 3, 4, 5
, then stops.
We can implement our previous example Fibonacci sequence using std::iter::successors
as well.
Here’s the equivalent version of Fibonacci
iterator using successor
.
use std::iter;
fn fibonacci() -> impl Iterator<Item = u32> {
iter::successors(Some((0, 1)), |&(curr, next)| {
Some((next, curr + next))
})
.map(|(curr, _)| curr)
}
fn main() {
let first_10: Vec<u32> = fibonacci().take(10).collect();
println!("{:?}", first_10); // [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
}
Overall Easier Setup: successors(Some(start), |&prev| Some(next))
is all you need to define a sequence and it is more functional-style code.
Conclusion
Iterators are a fundamental concept in programming that provide a powerful and flexible way to traverse and manipulate collections. By abstracting the underlying data structure, iterators offer a safe and efficient means to perform operations like mapping, filtering, and reducing. In Rust, the iterator system is particularly robust, supporting lazy evaluation, ownership, borrowing, and a wide range of combinators that enhance code readability and maintainability. Understanding the different traits and methods associated with iterators, such as Iterator
and IntoIterator
, allows you to write more expressive and efficient code.
Subscribe to my newsletter
Read articles from Siddharth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
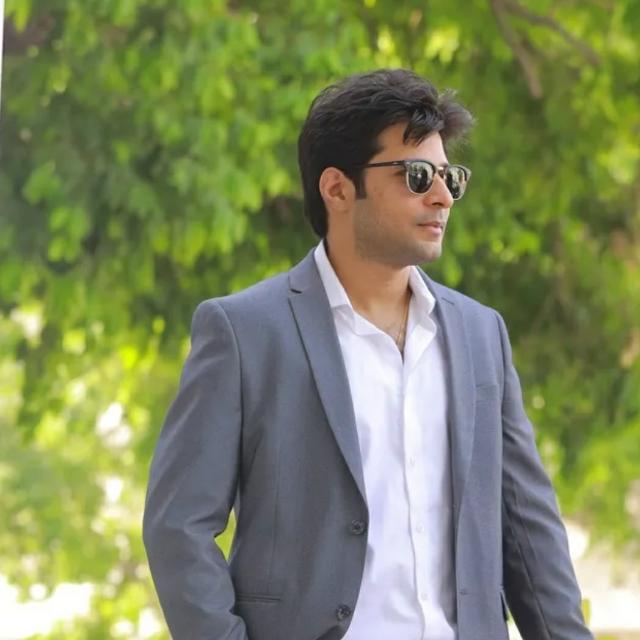
Siddharth
Siddharth
I am Quantitative Developer, Rust enthusiast and passionate about Algo Trading.