Understanding How Python Works from the Inside
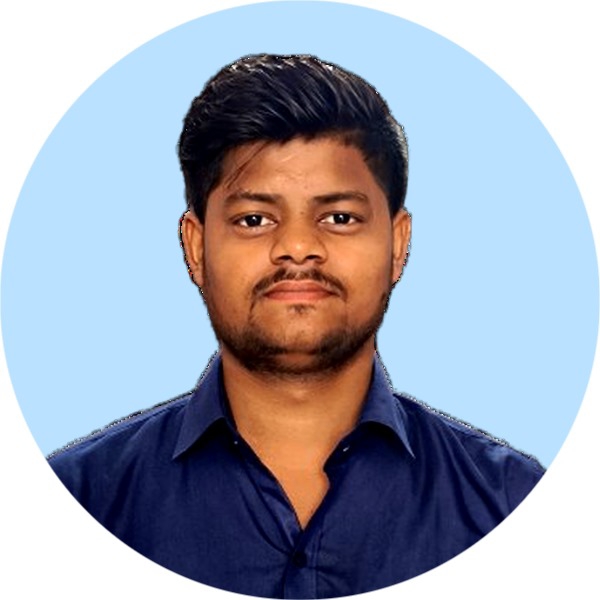
Hello and welcome! Today we’re taking a deep dive into the world of Python to understand what happens under the hood. Whether you’re on Windows, macOS, or Linux, you can download and install Python from python.org. Before we start exploring Python’s internals, make sure you have Python installed on your machine.
Getting Started: Your First Python Script
First, let’s write a simple script to get our feet wet. Create a folder named python_working
and inside it, create two files: main.py
and module.py
.
Step 1: Running Your Main Script
In main.py
, add the following code:
# python_working/main.py
print("Hello World!")
def printChai(name):
print(name)
printChai("lemon tea")
Run this script in your terminal. You should see the output:
Hello World!
lemon tea
Congratulations—you’ve just run your first Python script!
Step 2: Creating and Running a Module
Now, create another file called module.py
with the following code:
# python_working/module.py
from main import printChai
printChai("ginger tea")
Run module.py
by executing the command:
python module.py
You should now see the outputs:
The greeting from
main.py
(if it gets executed on import)lemon tea
(if any module-level code frommain.py
runs)ginger tea
Well done—you’ve taken the second step!
What’s Happening Behind the Scenes?
After running your module in Visual Studio Code (or another IDE), you might notice that a folder named __pycache__
appears. Inside this folder, there will be a file with a name similar to module.cpython-312.pyc
.
Why Does Python Create These Files?
These .pyc
files are compiled Python files containing bytecode. Let’s break this down:
Compilation to Bytecode:
When you run a Python script, the interpreter first compiles your source code into a lower-level, platform-independent representation called bytecode. This process makes your code run a bit faster since it’s easier for the Python Virtual Machine (PVM) to execute.Role of the
.pyc
Files:
The compiled bytecode is stored in.pyc
files within the__pycache__
directory. These files help speed up subsequent runs of your program. Note that this caching happens only for imported modules, not for the top-level script you run directly (likemain.py
).The Python Virtual Machine (PVM):
The PVM is a runtime engine that interprets the bytecode. Think of it as the “brain” of Python that loops through your bytecode and executes it. The Python interpreter you run (often referred to as CPython—the standard implementation) combines both the compiler (which creates bytecode) and the virtual machine.
Key Points to Remember
Bytecode is Not Machine Code:
Bytecode is an intermediate, low-level representation of your source code that is not directly executed by your computer’s hardware. Instead, the PVM interprets it.Compiled Files in
__pycache__
:
These files store your compiled bytecode along with information about the Python version used and a hash of the source file. They help speed up program execution by reusing compiled code when possible.Python Implementations:
While CPython is the standard and most widely used implementation of Python, there are others like Jython, IronPython, and PyPy. Each has its own way of handling Python code, but the concept of compiling to bytecode remains central.
Conclusion
Through this tutorial, you’ve taken your first steps into understanding the inner workings of Python—from writing and running simple scripts to learning about bytecode and the Python Virtual Machine. I hope this article helps deepen your Python knowledge and inspires you to explore even more.
In addition to this article, you might find this video by Chai aur Code very helpful. It offers a detailed explanation of the concepts discussed here. Watch the Video.
Happy coding, and thanks for joining me on this journey—stay tuned for more fun explorations into programming, tech, and coding.
Subscribe to my newsletter
Read articles from Vivek Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
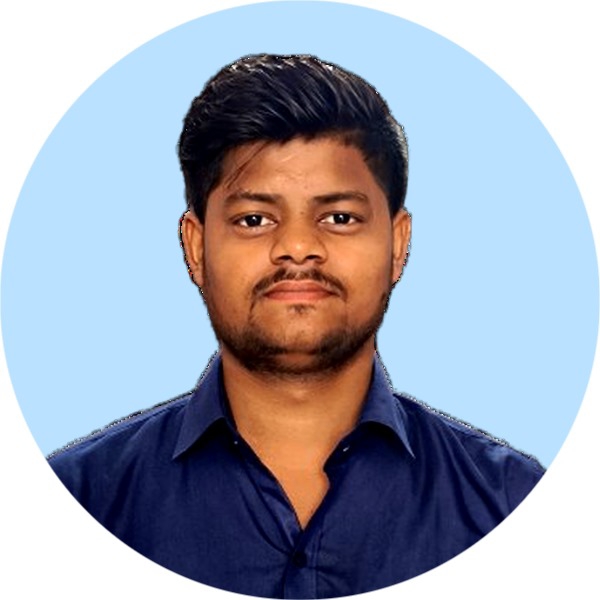