From Chat to Action: How Entent Automates Your Conversations
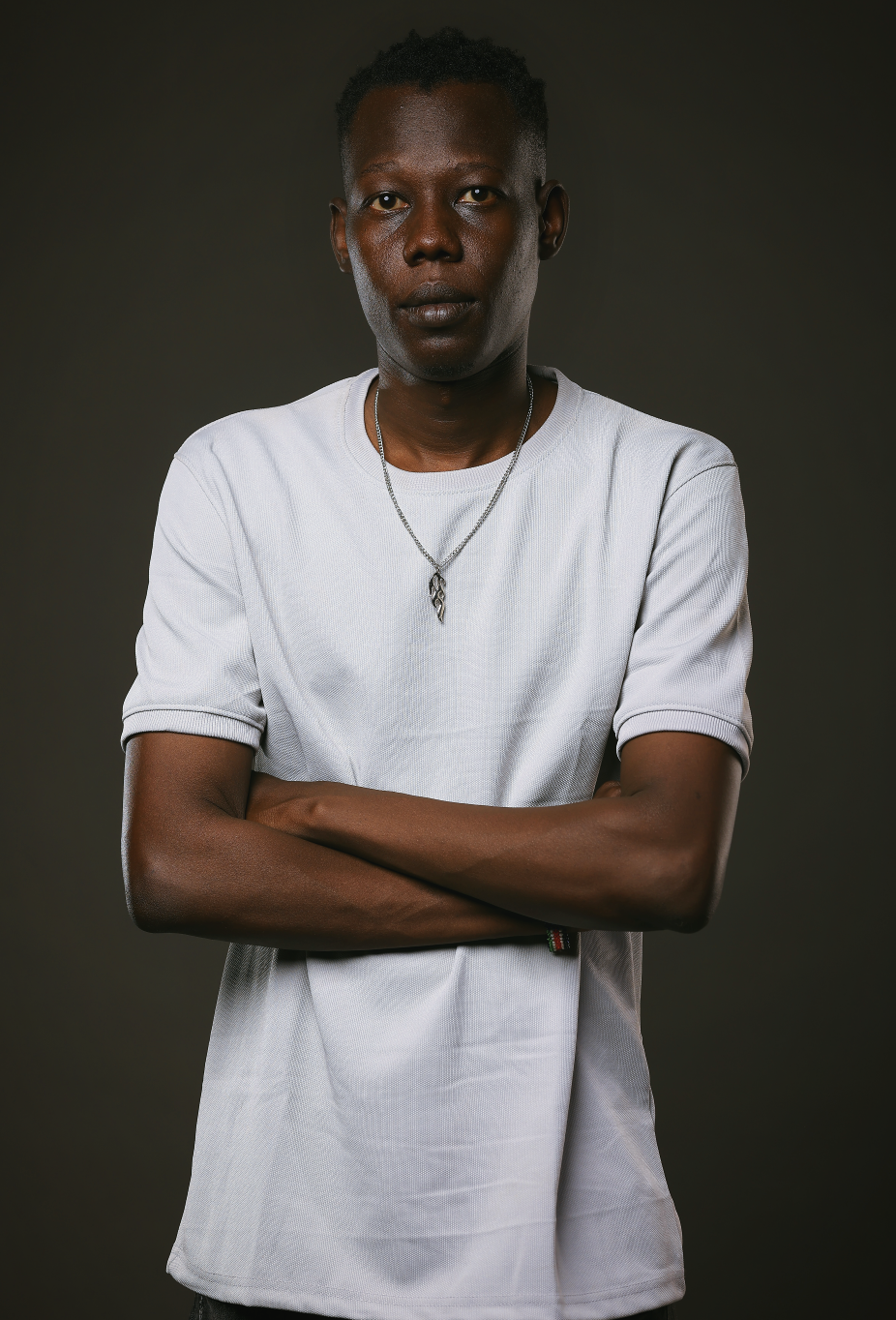

TL;DR: I built Entent, an open-source platform that lets AI models access your real-time data through API integrations. Using the Model Control Protocol (MCP), Entent connects LLMs to services like GitHub and Discord. Users trigger powerful workflows with simple emoji reactions - no more "I don't have access to that information" from your AI assistant.
The Problem: AI Models Trapped in Time
Have you ever asked ChatGPT about your GitHub repository or tried scheduling a meeting with Claude, only to be told "I don't have access to that"? Today's AI models are limited to the data they were trained on, disconnected from your actual tools and workflows.
Introducing Entent
I built Entent, an open-source platform that connects LLMs to real-time APIs through a standardized protocol. It transforms interactions from:
Entent currently integrates with Discord and GitHub, with Slack and Google Meet/Calendar coming soon.
Current reality:
You: "Can you help fix this bug?"
AI: "I'd be happy to help! Can you share the code?"
You manually share code
AI: "Based on what you've shared..."
With Entent:
You: "Can you help fix our authentication bug?"
AI: "I'll check your repository... I see the auth module has a failed test in JWT validation. There's a timing attack vulnerability in the token comparison. Here's the exact line that needs fixing..."
How Entent Works: Model Control Protocol
The Model Control Protocol (MCP) provides a standardized bridge between language models and external services:
MCP Servers: Each service has a dedicated server exposing standardized tools
LLM Integration: AI models request specific data or actions through these servers
User Interface: Emoji reactions trigger sophisticated workflows
Emoji Reactions: Simple Triggers for Complex Workflows
While designing Entent, I discovered emoji reactions provide the perfect interface:
π Creates a GitHub issue from a Discord message
π Schedules a meeting based on a conversation
π Generates documentation from a technical discussion
Behind each simple reaction is a complex workflow, but all the user sees is: add reaction β magic happens.
Real-World Examples
Meeting Scheduling (π ): When a user adds this reaction, Entent analyzes the conversation, checks calendars, suggests optimal times, handles voting, creates invites, and generates an agenda.
Knowledge Capture (π): This reaction analyzes technical discussions, extracts key information and code examples, generates documentation, publishes to the knowledge base, and cross-references existing docs.
Building Your Own MCP Server
Implementing an MCP server is straightforward. Here's a simplified example for Discord:
import { McpServer } from "@modelcontextprotocol/sdk/server/mcp.js";
import { StdioServerTransport } from "@modelcontextprotocol/sdk/server/stdio.js";
import { z } from "zod";
// Create MCP server
const server = new McpServer({
name: "discord",
version: "1.0.0",
});
// Register tools
server.tool(
'get-discord-messages',
'Get messages from a particular Discord channel',
{
channel: z.string().describe("Channel name"),
limit: z.number().optional().describe("Maximum messages")
},
async ({ channel, limit = 25 }) => {
// Implementation to fetch messages
const messages = await discordService.fetchChannelMessages(channelId, limit);
return {
content: [
{
type: "text",
text: messages.join("\n")
}
]
};
}
);
// Connect the server
async function main() {
const transport = new StdioServerTransport();
await server.connect(transport);
}
main();
Iβm open-sourcing the platform, hereβs the Github repo.
What workflows in your organization could benefit from emoji-triggered AI automation? Let me know in the comments!
Subscribe to my newsletter
Read articles from Emmanuel Gatwech directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
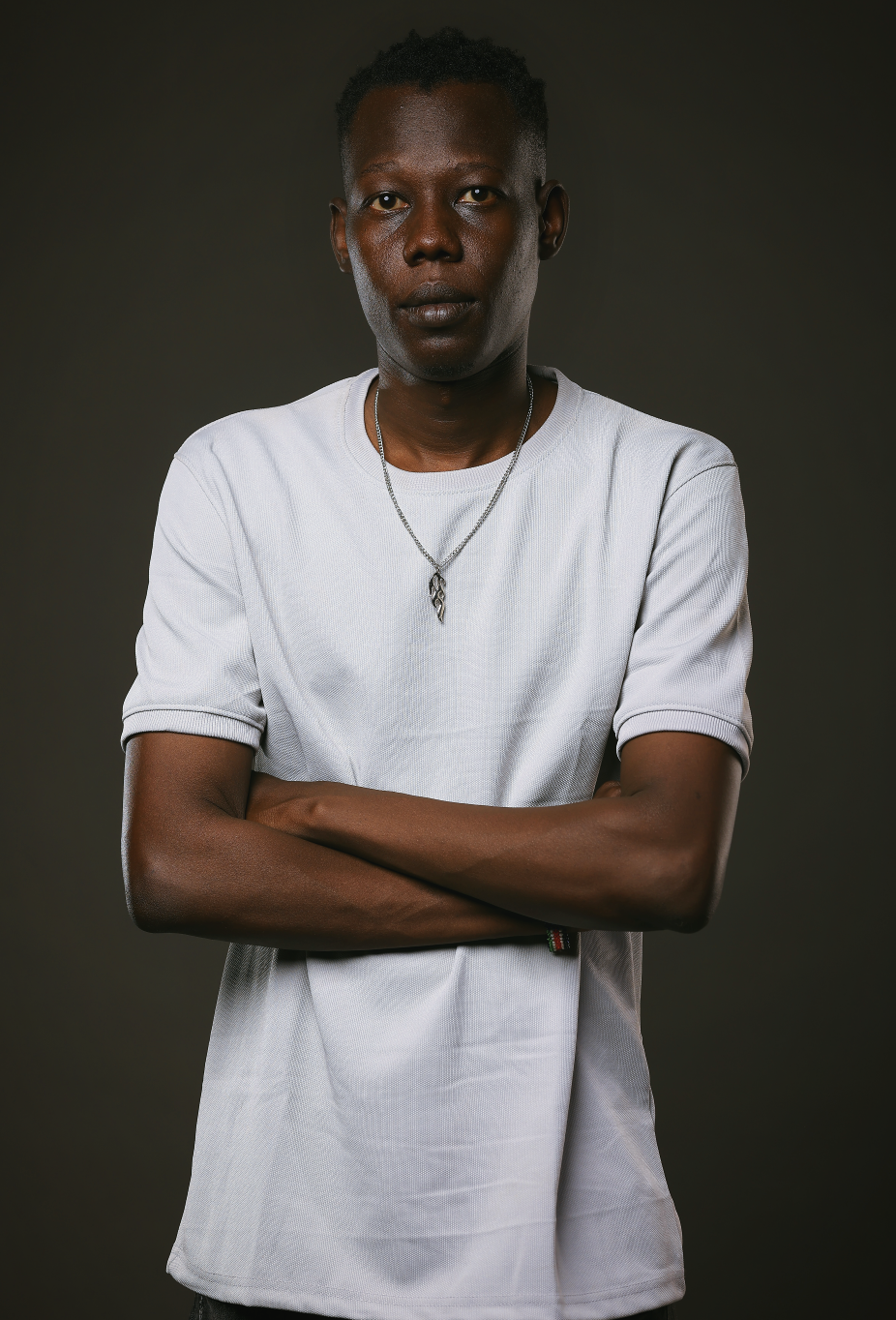
Emmanuel Gatwech
Emmanuel Gatwech
I am a software developer with over four years of experience and a passion for building innovative solutions that address real-world problems, having worked on various projects in various sectors. I am the founder of Sahil, an app that connects small businesses with suppliers and customers in low-income areas. Sahil is a comprehensive platform that simplifies procurement processes, connects businesses with reliable suppliers, and ensures swift, efficient deliveries. In addition to Sahil, I am actively involved in two open-source initiatives: "Planet of the Bugs", an open-source platform that curates unique, curated issues and bugs from popular open-source projects on GitHub, and "VibeCheck", which explores innovative social networking to establish more authentic connections. I am committed to advancing technology for the benefit of all, and my commitment to efficiency and problem-solving is evident in my work. My technical proficiency lies in TypeScript, Next.js, GraphQL, Redis, PostgreSQL, Node.js, and Rust.