Client-Side Rendering: Blazor, React, and Angular
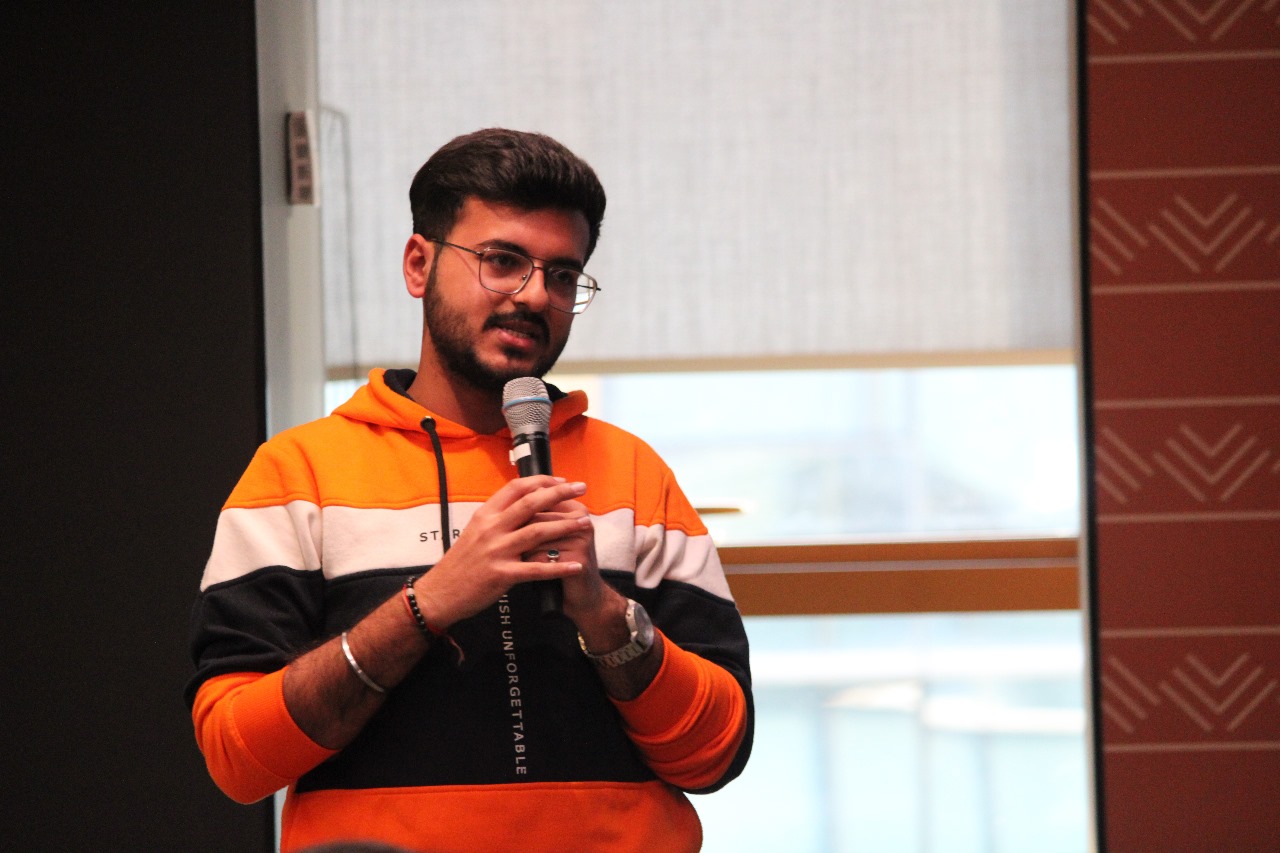
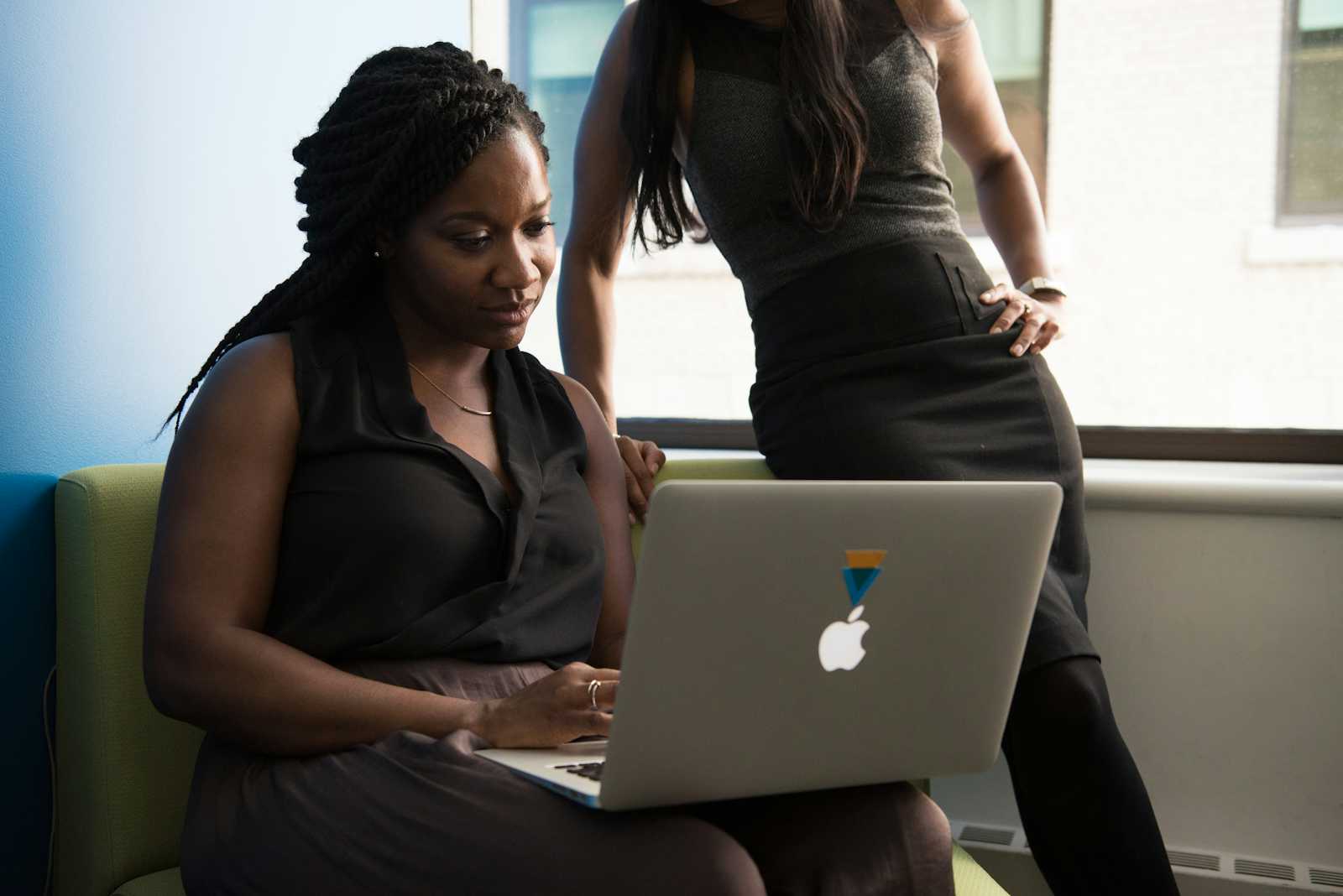
Client-side rendering (CSR) is a popular web development approach where the browser renders the content on the user's device using JavaScript, HTML, and CSS. This contrasts with server-side rendering (SSR), where content is generated on the server and sent as a fully formed HTML document. CSR offers a highly interactive user experience and is widely implemented in modern frameworks like Blazor, React, and Angular. Let’s dive into the details of CSR, comparing these frameworks, and exploring their features and code examples.
Advantages of Client-Side Rendering
Dynamic User Experience:
Enables highly interactive and responsive user interfaces.
Faster updates as only necessary parts of the DOM are updated.
Reduced Server Load:
- Shifts much of the processing to the client’s browser, reducing server-side rendering demands.
Rich Ecosystem:
- JavaScript frameworks provide extensive libraries and tools for seamless development.
Offline Capabilities:
- CSR applications can leverage Progressive Web App (PWA) features to work offline using cached data.
Smooth Navigation:
- Single Page Applications (SPAs) eliminate page reloads, providing fluid navigation.
Blazor for Client-Side Rendering
Blazor is a web framework by Microsoft that enables developers to build interactive web applications using C# instead of JavaScript. With Blazor WebAssembly, CSR is achieved by running .NET code directly in the browser.
Features of Blazor WebAssembly
C# and .NET Ecosystem:
- Reuse .NET libraries and logic across client and server.
WebAssembly:
- Runs directly in the browser with near-native performance.
Strongly-Typed Code:
- Leverages C#'s static typing for fewer runtime errors.
Blazor WebAssembly Example
@page "/counter"
<h3>Counter</h3>
<p>Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
Explanation
@page: Defines the route for this component.
@onclick: Handles the click event.
@code: Contains the component’s logic written in C#.
React for Client-Side Rendering
React is a declarative, component-based JavaScript library developed by Facebook for building user interfaces. It utilizes a virtual DOM to update the UI efficiently.
Features of React
Component-Based Architecture:
- Build reusable components that encapsulate logic and presentation.
Virtual DOM:
- Minimizes DOM manipulation for improved performance.
Rich Ecosystem:
- Supported by a vast library of tools like Redux, React Router, etc.
React Example
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h3>Counter</h3>
<p>Current count: {count}</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
export default Counter;
Explanation
useState: React hook for managing state.
onClick: Binds a click event to the button.
JSX: Combines JavaScript and HTML-like syntax for declarative UI.
Angular for Client-Side Rendering
Angular is a full-fledged TypeScript-based framework developed by Google. It is known for its two-way data binding and powerful dependency injection system.
Features of Angular
Two-Way Data Binding:
- Synchronizes the view and model automatically.
Dependency Injection:
- Encourages modular development by managing dependencies efficiently.
Comprehensive Framework:
- Offers built-in tools for routing, state management, and HTTP requests.
Angular Example
import { Component } from '@angular/core';
@Component({
selector: 'app-counter',
template: `
<h3>Counter</h3>
<p>Current count: {{ count }}</p>
<button (click)="increment()">Click me</button>
`,
})
export class CounterComponent {
count = 0;
increment() {
this.count++;
}
}
Explanation
@Component: Decorator defining a component’s metadata.
{{ count }}: Angular’s interpolation syntax for binding data.
(click): Angular’s event binding syntax.
Comparing Blazor, React, and Angular
Feature | Blazor | React | Angular |
Language | C# | JavaScript/TypeScript | TypeScript |
Learning Curve | Medium | Low | High |
Ecosystem | .NET libraries | Third-party libraries | Built-in and third-party |
Performance | WebAssembly-based | Virtual DOM | Two-way data binding |
Community Support | Growing | Extensive | Extensive |
When to Choose Which Framework?
Use Blazor if:
You are a .NET developer and prefer C# over JavaScript.
You want to share logic and libraries across server and client.
Use React if:
You want a lightweight, flexible library with a massive ecosystem.
You prefer simplicity and a functional programming paradigm.
Use Angular if:
You need a complete solution with built-in tools for complex projects.
You prefer TypeScript and want robust structure and scalability.
Challenges of Client-Side Rendering
SEO Limitations:
- Search engines may struggle to index JavaScript-heavy pages.
Initial Load Time:
- The browser must download and execute JavaScript before rendering content.
Client-Side Resource Usage:
- High CPU and memory consumption on the client device.
Mitigation Strategies
Code Splitting: Load only necessary JavaScript for the current page.
Lazy Loading: Defer loading of non-critical resources.
Server-Side Rendering (Hybrid Approach): Combine CSR and SSR for SEO-friendly and dynamic apps.
Conclusion
Client-side rendering has revolutionized web development by offering highly interactive and responsive user interfaces. Frameworks like Blazor, React, and Angular cater to different needs, allowing developers to choose the best tool based on their expertise and project requirements. Understanding their unique strengths and weaknesses ensures the delivery of optimal web applications.
Subscribe to my newsletter
Read articles from SHIVAM MIDDHA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
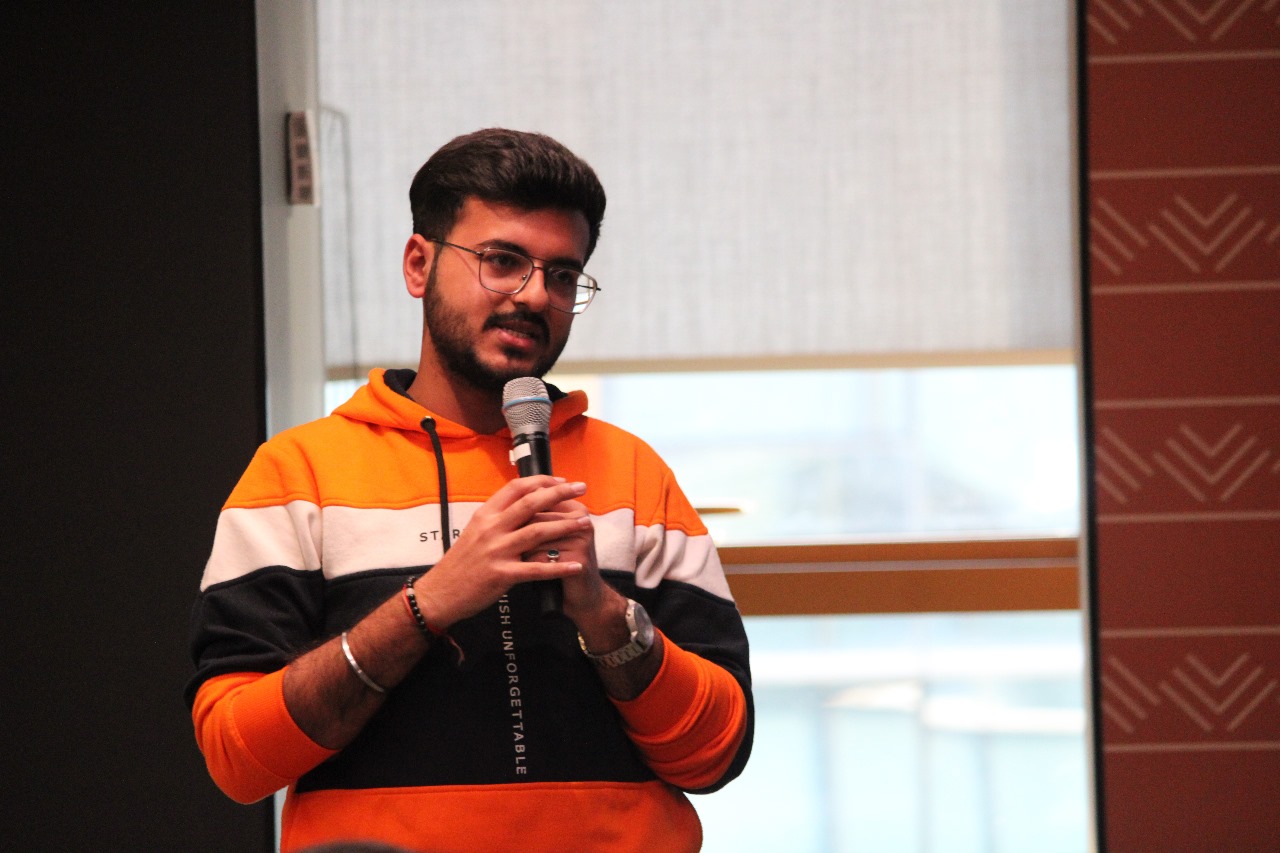
SHIVAM MIDDHA
SHIVAM MIDDHA
Proactive Software Developer #MERNWizard | Fueling Tech Communities | Public Speaker #ImpactThroughCode