AJAX: The Unsung Hero behind Modern Web Apps
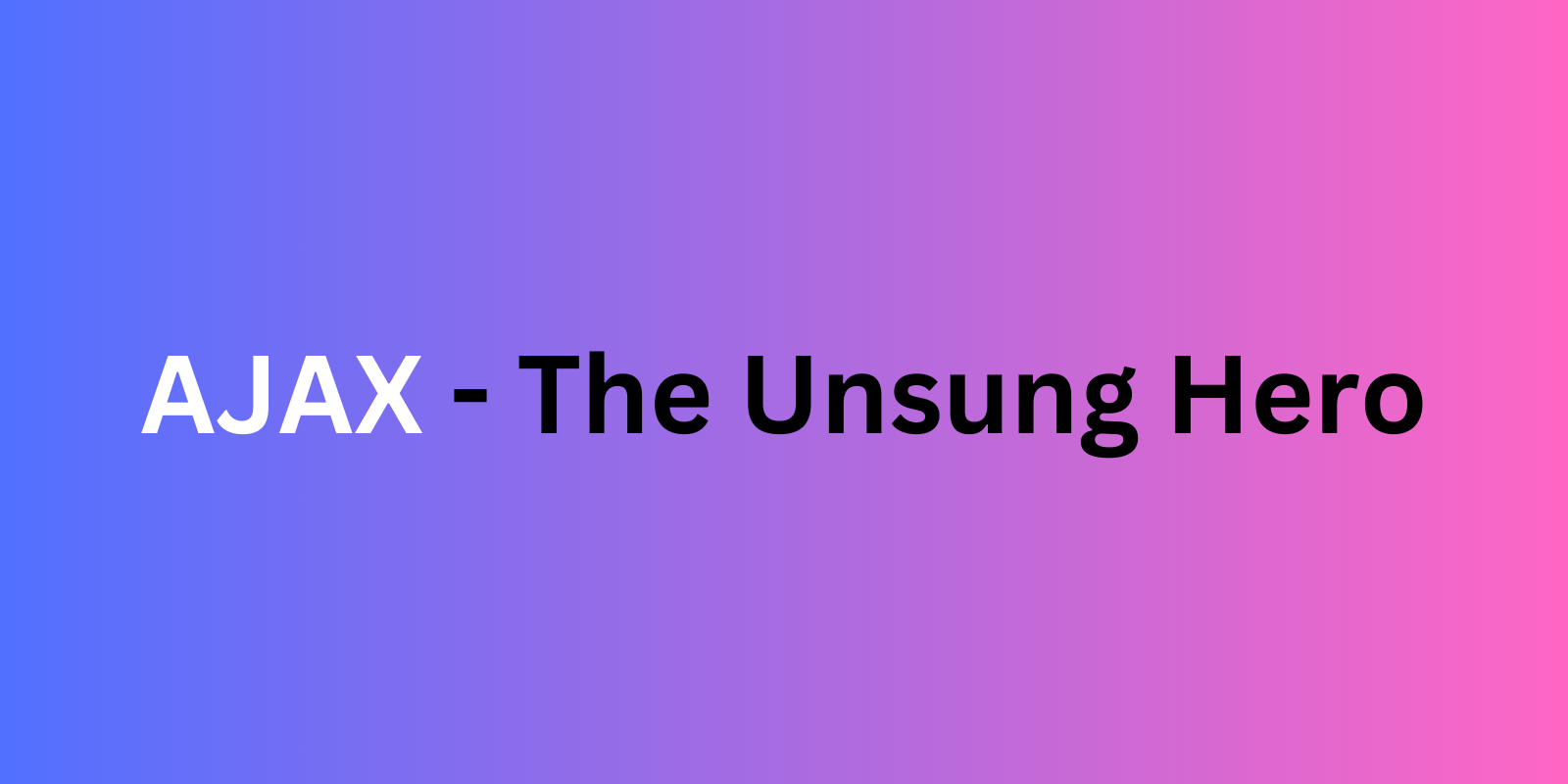
Introduction
Ever wondered how Gmail loads emails instantly or how Twitter updates your feed in real-time? The technology making this possible is AJAX (Asynchronous JavaScript and XML).
AJAX changed the web by allowing pages to send and receive data from a server without reloading. While newer methods like the Fetch API and WebSockets have emerged, AJAX laid the foundation for modern web development.
In this article, we'll explore:
What AJAX is and why it was revolutionary
How AJAX was done in the past (XMLHttpRequest)
The modern way of handling AJAX (Fetch API)
A step-by-step guide to using both methods
By the end, you’ll understand AJAX inside out and know how to implement it in your own projects!
What is AJAX?
AJAX is not a programming language—it’s a technique that allows web applications to fetch and send data asynchronously without refreshing the page.
How AJAX Works in 3 Steps
1️⃣ JavaScript makes a request to the server in the background.
2️⃣ The server processes the request and responds with data (usually JSON).
3️⃣ JavaScript updates the webpage dynamically without a full reload.
This was game-changing because, before AJAX, every action (like submitting a form) required a full-page reload, making websites slow and clunky.
The Old Way: AJAX with XMLHttpRequest (Legacy Method)
Before the Fetch API, developers used XMLHttpRequest
to handle AJAX requests. It worked, but it was verbose and hard to manage.
Example: Making a GET Request with XMLHttpRequest
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX with XMLHttpRequest</title>
</head>
<body>
<button onclick="loadData()">Get Data</button>
<p id="output">Data will appear here...</p>
<script>
function loadData() {
let xhr = new XMLHttpRequest();
xhr.open("GET", "https://jsonplaceholder.typicode.com/todos/1", true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
document.getElementById("output").innerText = xhr.responseText;
}
};
xhr.send();
}
</script>
</body>
</html>
How This Works
open("GET", url, true)
: Opens a connection to the server.onreadystatechange
: Waits for a response from the server.xhr.send()
: Sends the request.
Problems with XMLHttpRequest
❌ Complicated syntax – Requires multiple event handlers.
❌ No built-in promise support – Makes it hard to chain requests.
❌ Verbose code – More lines for simple requests.
Developers needed a cleaner solution. That’s where the Fetch API came in!
The Modern Way: AJAX with Fetch API (Recommended Method)
The Fetch API is the modern replacement for XMLHttpRequest
. It’s simpler, uses promises, and integrates well with modern JavaScript practices like async/await.
Example: Making a GET Request with Fetch API
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AJAX with Fetch API</title>
</head>
<body>
<button onclick="loadData()">Get Data</button>
<p id="output">Data will appear here...</p>
<script>
function loadData() {
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then(response => response.json()) // Convert response to JSON
.then(data => {
document.getElementById("output").innerText = JSON.stringify(data, null, 2);
})
.catch(error => console.log("Error:", error));
}
</script>
</body>
</html>
Why Fetch API is Better?
✅ Simpler syntax – No need for event handlers.
✅ Uses promises – More readable and easier to manage.
✅ Better error handling – Uses .catch()
for errors.
Handling POST Requests: Old vs. New
POST Request using XMLHttpRequest
function sendData() {
let xhr = new XMLHttpRequest();
xhr.open("POST", "https://jsonplaceholder.typicode.com/posts", true);
xhr.setRequestHeader("Content-Type", "application/json");
xhr.onload = function () {
if (xhr.status === 201) {
console.log("Success:", xhr.responseText);
} else {
console.log("Error:", xhr.status);
}
};
let data = JSON.stringify({
title: "Hello AJAX",
body: "This is a test post",
userId: 1
});
xhr.send(data);
}
sendData();
POST Request using Fetch API
function sendData() {
fetch("https://jsonplaceholder.typicode.com/posts", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify({
title: "Hello AJAX",
body: "This is a test post",
userId: 1
})
})
.then(response => response.json())
.then(data => console.log("Success:", data))
.catch(error => console.log("Error:", error));
}
sendData();
Key Differences:
Feature | XMLHttpRequest (Old) | Fetch API (New) |
Syntax | Complicated | Simple & readable |
Response Handling | Uses onreadystatechange | Uses .then() & .catch() |
Promise Support | No | Yes |
Readability | Verbose | Clean & concise |
Error Handling | Requires manual handling | Built-in .catch() |
Conclusion
AJAX changed web development forever, making dynamic web applications possible. While the older XMLHttpRequest
method worked, it was cumbersome. The Fetch API is now the modern, recommended approach because it's simpler, cleaner, and more efficient.
Understanding evolution of the AJAX will help understand how fetch API works more.
💡 What’s next?
Try using AJAX in your own projects! Start with Fetch API, then experiment with async/await for even cleaner code.
Subscribe to my newsletter
Read articles from Sumal Surendra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sumal Surendra
Sumal Surendra
I'm Sumal Surendra, an Information Systems undergrad from Sabaragamuwa University of Sri Lanka on a mission to decode the mysteries of the digital world. Think code whisperer, data detective, and all-around tech enthusiast!