๐ Learning Go: Syntax, Variables, Loops, and Functions #1

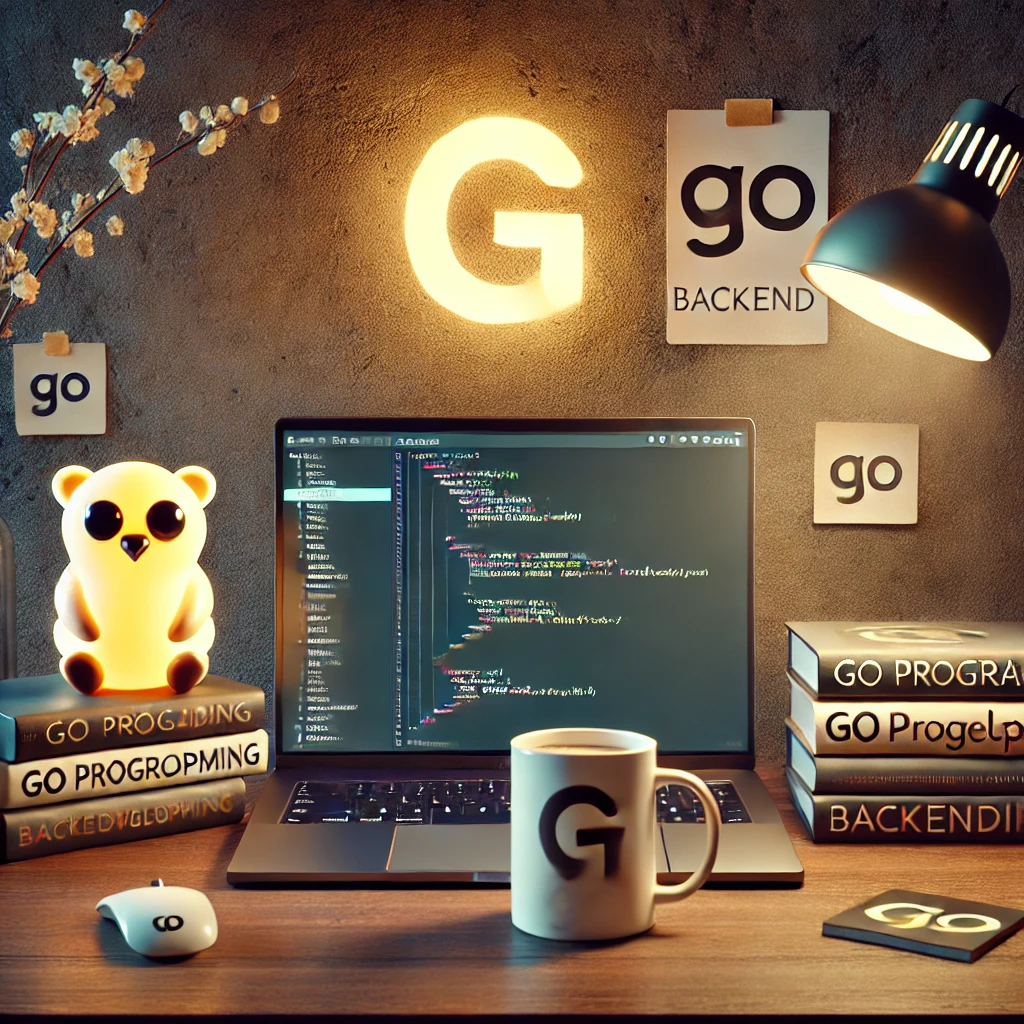
Iโll share what Iโm learning about Go (Golang) today. I'll cover the basics like syntax, variables, loops, and functions with examples. I'll keep it simple and to the point so you can easily follow along. Let's dive in!
๐ 1. Syntax in Go
Go is a statically typed, compiled language known for its simplicity and performance. Hereโs a basic Go program structure:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
โ Explanation:
package main
โ Every Go program starts with amain
package.import "fmt"
โ Imports thefmt
package to print output.func main()
โ Themain
function is the entry point of the program.fmt.Println()
โ Prints text to the console.
๐ 2. Variables and Data Types in Go
In Go, variables are defined using the var
keyword or the :=
shorthand.
โ Declare and Assign Variables
var name string = "Chirag"
var age int = 25
var isActive bool = true
Or use shorthand:
name := "Chirag"
age := 25
isActive := true
โ Data Types in Go
Type | Example | Description |
string | "Go" | Sequence of characters |
int | 25 | Integer values |
float64 | 25.5 | Floating-point numbers |
bool | true/false | Boolean values |
rune | 'a' | Unicode character |
byte | 123 | Alias for uint8 |
uint | 123 | Unsigned integer |
โ Example of Different Types:
var score int = 90
var price float64 = 99.99
var available bool = true
var letter rune = 'G'
var initial byte = 65
๐ 3. Loops in Go
Loops help execute code repeatedly. Go mainly supports for
loops.
โ For Loop
Standard for
loop:
for i := 0; i < 5; i++ {
fmt.Println(i)
}
โ While-Style Loop
You can use for
as a while loop:
counter := 0
for counter < 5 {
fmt.Println(counter)
counter++
}
โ Infinite Loop
You can create an infinite loop using for
without conditions:
for {
fmt.Println("Running...")
break // Use break to exit the loop
}
โ Loop Over a String
You can loop over a string using range
:
message := "Hello i am chirag"
for index, char := range message {
fmt.Printf("Index: %d, Char: %c\n", index, char)
}
๐ 4. Functions in Go
Functions allow you to group reusable code.
โ Basic Function
func greetUser() {
fmt.Println("Hello, Chirag!")
}
Call it using:
// call it inside main function
greetUser()
// output would be
// Hello, Chirag!
โ Function with Parameters and Return Value
func sum(a int, b int) int {
return a + b
}
result := sum(10, 20)
fmt.Println("Sum is:", result)
// output is:
// Sum is: 30
โ Multiple Parameters with the Same Type
Instead of writing a int, b int, c int
, you can combine types:
func sumThree(a, b, c int) int {
return a + b + c
}
sumThree(10, 20, 30)
// output is:
// 60
โ Named Return Value
You can define a named return value directly:
func subtract(a int, b int) (result int) {
result = a - b
return
}
subtract(10, 5)
// output is:
5
โ ๏ธ 5. Error Handling in Go
Go handles errors using the error
type.
โ Function with Error Handling
func divide(a float32, b float32) (float32, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide by zero")
}
return a / b, nil
}
divide(10,0)
divide(10,5)
// output is :
// 1. cannot divide by zero
// 2. 2.0
// it will return float data type value having decimal number
โ Use it Like This:
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
// and you can either user _ or catch in another variable
// but golang , restrict it , to use your declare variable what you hace declared
๐ฏ 6. Use of _
in Golang
The underscore _
is used to ignore values you donโt want to use.
โ Example:
If a function returns two values and you only need one:
result, _ := divide(10, 2)
fmt.Println("Result:", result)
In this example, the second return value (error
) is ignored using _
.
๐ Complete Example
Here's a complete example combining functions, error handling, and loops:
package main
import "fmt"
func greetUser() {
fmt.Println("Welcome to Go!")
}
func sum(a, b int) int {
return a + b
}
func divide(a, b float32) (float32, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide by zero")
}
return a / b, nil
}
func main() {
greetUser()
result := sum(10, 20)
fmt.Println("Sum is:", result)
quotient, err := divide(100, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Quotient is:", quotient)
}
for i := 0; i < 5; i++ {
fmt.Println("Count:", i)
}
message := "Hello"
for _, char := range message {
fmt.Printf("Char: %c\n", char)
}
}
๐ฎ Whatโs Coming Next
Next week, Iโll cover:
โ
if-else, else and switch cases
โ
array , slices
โ
Struct, Maps, and Pointers
Stay tuned for more! ๐ and do follow me
Subscribe to my newsletter
Read articles from CHIRAG KUMAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

CHIRAG KUMAR
CHIRAG KUMAR
Full stack Developer