Introducing Appwrite Exceptions Translator: Error Messages That Make Sense

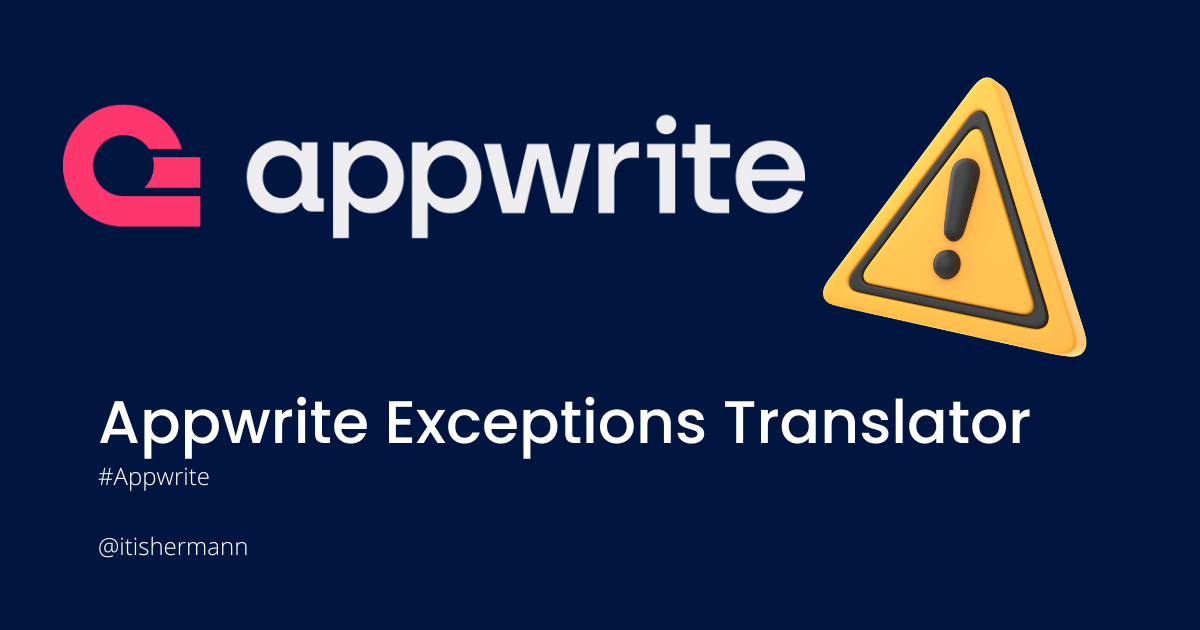
Error messages are often the first interaction users have with your support system. When they're cryptic, technical, or in the wrong language, they create confusion rather than clarity. That's why I built the Appwrite Exceptions Translator - a lightweight library that transforms Appwrite's technical error messages into user-friendly, localized explanations.
The Problem with Raw Error Messages
If you've worked with Appwrite (or any backend service), you're familiar with errors like:
{
"message": "Invalid id: Parameter must be a valid number",
"type": "general_argument_invalid",
"code": 400
}
These messages make perfect sense to developers but can be bewildering to end users. They lack context, often contain technical jargon, and rarely offer guidance on how to resolve the issue.
A Better Way to Handle Errors
The Appwrite Exceptions Translator addresses these issues by:
Translating error codes and types into human-readable explanations
Supporting multiple languages (currently English, French, Spanish, and Arabic)
Providing a consistent error handling pattern across your application
Falling back gracefully when no translation is available
How It Works
Using the translator is straightforward:
import { AppwriteExceptionTranslator } from "@itishermann/appwrite-exceptions-translator";
import { LocalTranslationProvider } from "@itishermann/appwrite-exceptions-translator/providers";
// Initialize the translator
const translator = new AppwriteExceptionTranslator(
new LocalTranslationProvider()
);
// Somewhere in your error handling...
try {
// Appwrite operations
} catch (error) {
// Transform the error into a user-friendly message
const userMessage = translator.translate(error);
// Display to user (instead of the raw error)
showNotification(userMessage);
}
Instead of your users seeing "general_argument_invalid", they'll see something like "The request contains one or more invalid arguments" - or its equivalent in their preferred language.
Flexible and Extensible
The library is designed with flexibility in mind:
Language switching on-the-fly - Change languages without restarting your app
Prioritized translation strategy - Tries error type first, falls back to error code
Custom translation providers - Implement your own provider for different translation sources
Lightweight footprint - No heavy dependencies, just the translations you need
Built for Modern JavaScript Environments
The package is built with TypeScript, includes full type definitions, and supports both ESM and CommonJS. It works seamlessly with:
Node.js applications
React, Vue, Angular, and other frontend frameworks
React Native and other mobile JavaScript frameworks
Bun projects (it's actually built with Bun!)
Getting Started
Installation is simple:
# Using npm
npm install @itishermann/appwrite-exceptions-translator
# Using yarn
yarn add @itishermann/appwrite-exceptions-translator
# Using bun
bun add @itishermann/appwrite-exceptions-translator
Why I Built This
As a developer working with Appwrite, I found myself repeatedly writing code to transform error messages into something more user-friendly. After copy-pasting the same logic across multiple projects, I decided to extract it into a reusable package.
This translator is part of a larger effort to improve the developer and end-user experience when working with Appwrite - an excellent open-source backend that deserves equally excellent tooling.
Contributing
This is an open-source project licensed under AGPL-3.0, and contributions are welcome! Whether you want to:
Add translations for additional languages
Improve existing translations
Extend the functionality
Fix bugs
Check out the contribution guidelines to get started.
Conclusion
Error handling doesn't have to be an afterthought. With Appwrite Exceptions Translator, you can provide clear, helpful error messages to your users in their preferred language, improving the overall user experience of your application.
Give it a try in your next Appwrite project, and turn frustrating errors into helpful guidance.
Check out the repository on GitLab or install it directly from npm.
Subscribe to my newsletter
Read articles from Hermann Kao directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hermann Kao
Hermann Kao
Software developer documenting my journey through web and mobile development. I share what I learn, build, and struggle with—from React to SwiftUI, architecture decisions to deployment challenges. Not an expert, just passionate about coding and learning in public. Join me as I navigate the tech landscape one commit at a time.