"this" Keyword in Java

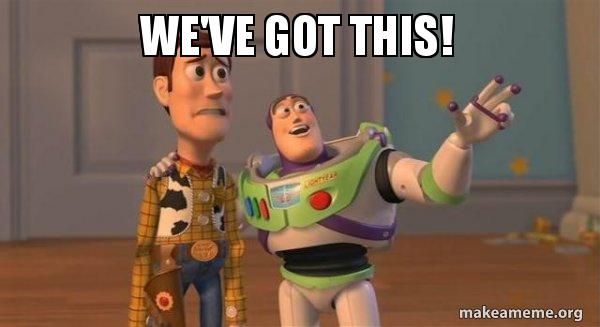
If you’ve been coding in Java for a while, you’ve probably met this. No, not that “this” where you forget why you walked into a room. We're talking about Java’s “this” a small but powerful keyword that saves us from naming disasters, constructor confusion, and much more.
Let’s dive in and see why this is more than just a way to refer to yourself in third person.
What is the this
Keyword?
In Java, this
is a reference to the current object the instance of the class where this
is used. It helps resolve naming conflicts, improve code readability, and even enables constructor chaining.
Using this
to Resolve Variable Shadowing
One of the most common use cases of this
is when a local variable has the same name as an instance variable. Without this
, Java would assume you're referring to the local variable.
Example: Without this
public class Car {
private String name; // Instance variable
public Car(String name) { // Parameter with the same name
name = name; // Uh-oh! This does nothing
}
public String getName() {
return name;
}
}
In the constructor, name = name;
simply assigns the parameter to itself, completely ignoring the instance variable just like our gym subscription! 🥲
Example: Using this
to Fix It
public class Car {
private String name;
public Car(String name) {
this.name = name; // Now we're correctly assigning the parameter to the instance variable
}
public String getName() {
return this.name; // Optional, but improves clarity
}
}
Now, this.name
correctly refers to the instance variable, and name
(without this
) refers to the constructor parameter.
Calling Another Constructor Using this()
Java allows constructor chaining, where one constructor calls another within the same class. The this()
method does exactly that.
Example: Constructor Chaining
public class Car {
private String name;
private String model;
public Car(String name) {
this(name, "Unknown Model"); // Calls the two-argument constructor
}
public Car(String name, String model) {
this.name = name;
this.model = model;
}
public void display() {
System.out.println("Car: " + this.name + ", Model: " + this.model);
}
}
Here, when new Car("Tesla")
is called, it automatically invokes Car(String, String)
using this(name, "Unknown Model")
.
Accessing the Outer Class in Nested Classes
When you have a nested (inner) class inside another class, you can use this
to reference the outer class.
Example: Accessing Outer Class
class Outer {
private String message = "Hello from Outer!";
class Inner {
public void show() {
System.out.println(Outer.this.message); // Refers to Outer class' message
}
}
}
public class Main {
public static void main(String[] args) {
Outer outer = new Outer();
Outer.Inner inner = outer.new Inner();
inner.show();
}
}
Here, Outer.this.message
ensures we're referring to the message
variable of Outer
, not Inner
.
Passing the Current Object (this
) as an Argument
Sometimes, a method needs to work on the current object itself. Instead of saying, "Hey, take this object I just created," we can just pass this
.
Example: Passing this
to a Method
class Engine {
void start(Car car) {
System.out.println("Starting the car: " + car.getName());
}
}
public class Car {
private String name;
public Car(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public void ignite() {
Engine engine = new Engine();
engine.start(this); // Passing the current object
}
public static void main(String[] args) {
Car myCar = new Car("Tesla");
myCar.ignite();
}
}
Method Chaining using this
YES! You heard that right. Method chaining is possible in Java, and this
makes it happen!
Instead of writing clunky, repetitive setter calls, we can return this to allow for a fluent API style.
class Car {
private String name;
private String model;
public Car setName(String name) {
this.name = name;
return this; // Returning the current object
}
public Car setModel(String model) {
this.model = model;
return this;
}
public void display() {
System.out.println("Car: " + this.name + ", Model: " + this.model);
}
public static void main(String[] args) {
Car myCar = new Car()
.setName("Tesla")
.setModel("Model S"); // Method chaining!
myCar.display();
}
}
So next time you see method chaining in Java, just nod and say, "Yep, this
made it possible." 😎
this
is More Than You Think!
✅ Resolves variable name conflicts
✅ Enables constructor chaining
✅ Lets nested classes reference outer classes
✅ Passes the current object to methods
✅ Supports method chaining
Subscribe to my newsletter
Read articles from Santhosh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santhosh
Santhosh
I’m a software developer passionate about exploring new technologies and continuously learning. On my blog, I share what I discover—whether it’s cool tricks, coding solutions, or interesting tools. My goal is to document my journey and help others by sharing insights that I find useful along the way. Join me as I write about: Programming tips & tricks Lessons from everyday coding challenges Interesting tools & frameworks I come across Always curious, always learning—let’s grow together! 🚀