Mastering Advanced Prompt Engineering

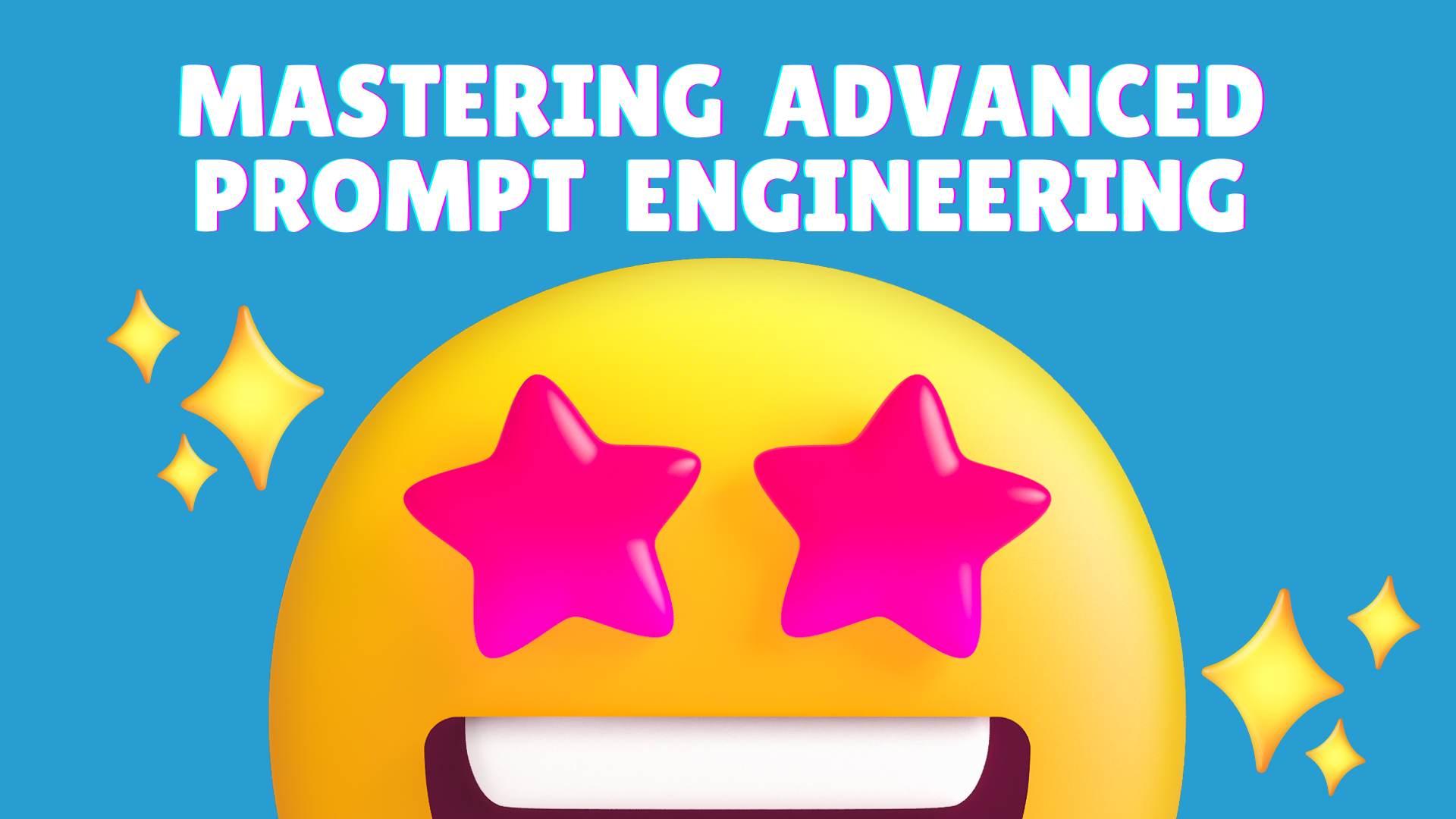
Table of Contents
Overview
Introduction
Advanced Prompting Techniques
Prompt Chaining
Parameter Control
Formatting Control
Delimiting Input
Refinement & Re-prompting
Reverse Prompting
Combining Multiple Prompting Techniques
Project: AI-Powered Recipe Generator
Building the Flask API
Testing the API with Postman
Conclusion
Overview
Advanced prompt engineering is the art of crafting effective AI prompts to generate high-quality responses. This guide explores various techniques, including prompt chaining, parameter control, formatting control, delimiting input, refinement, reverse prompting, and combining multiple methods to achieve precise and meaningful AI interactions.
Introduction
Prompt engineering is crucial in maximizing AI capabilities, ensuring structured, creative, and accurate responses. By mastering advanced techniques, developers and AI practitioners can significantly enhance response quality, optimize interactions, and improve AI-driven solutions' overall efficiency.
Basic Setup for Prompt
Install the OpenAI Python Library Ensure you have the OpenAI library installed:
pip install openai
Set Up Your OpenAI API Key Replace
'your-api-key'
with your actual OpenAI API key,Define Functions to Interact with the ChatGPT API Create a function to send messages to the API,
Use the
send_message
function to expand your blog outlineimport openai openai.api_key = 'your-api-key' def send_message(message): response = openai.ChatCompletion.create( model="gpt-3.5-turbo", temperature=0.9, messages=[ {"role": "system", "content": "You are a helpful assistant."}, {"role": "user", "content": message} ] ) return response['choices'][0]['message']['content'].strip() prompt = f"Hi, How are you?" response = send_message(prompt) print(response)
Prompt Chaining (Breaking Complex Tasks)
Imagine you're teaching a dog a complicated trick. You wouldn't just say, "Do the whole thing!" You'd break it down into smaller, easier steps: "Sit," then "Stay," then "Roll Over," then "Play Dead." Prompt chaining is the same idea, but for AI.
Concept Breaking a complex task into smaller prompts and chaining responses together, Instead of giving the AI one giant, complex instruction, you give it a series of smaller, simpler prompts, where the output of one prompt becomes the input for the next. Each prompt builds on the previous one, guiding the AI step-by-step to achieve the final, complex goal.
Example
def get_blog_outline():
return "1. Introduction to AI Ethics\n2. Key Challenges\n3. Solutions\n4. Conclusion"
def expand_outline(outline):
return f"Expanded details for:\n{outline}"
outline = get_blog_outline()
expanded_content = expand_outline(outline)
print(expanded_content)
Parameter Control (Controlling AI’s Creativity & Precision)
Think of an AI model like a painter. You can give the painter instructions (the prompt), but you also have some knobs to control how the painter interprets those instructions.
Concept Adjusting temperature
and max_tokens
for creative or factual responses. Parameter control is like adjusting those knobs. It lets you influence the AI's:
Creativity: How wildly imaginative or strictly factual the AI should be. Turn up the "creativity knob" for brainstorming, turn it down for accurate summaries.
Precision: How focused and specific the AI should be. Do you want a general overview or a detailed analysis?
Example
def generate_response(prompt, temperature):
return f"Response to: {prompt} with temperature {temperature}"
print(generate_response("Tell a fantasy story.", 0.9)) # Creative
print(generate_response("Explain quantum mechanics.", 0.2)) # Factual
Formatting Control (Ensuring Structured Output)
Imagine you're asking a friend to write a report for you. You wouldn't just say, "Write a report!" You'd tell them
"Use headings and subheadings."
"Put the key points in bullet points."
"Include a table with this data."
Concept Instructing AI to follow a specific format (bullets, tables, JSON, etc.). Formatting control is doing the same thing with AI. It's telling the AI how to structure its output, not just what to say.
Example
prompt = "List 3 benefits of exercise in bullet points."
response = "- Improves health\n- Boosts mood\n- Increases energy"
Delimiting Input (Avoiding Misinterpretation with Clear Boundaries)
Imagine you're giving a recipe to a chef, but you accidentally mix in some random notes about your day. The chef might get confused about what's actually part of the recipe!
Concept Using delimiters ("""
, <tags>
, etc.) for better text parsing. Delimiting input is like putting clear boundaries around the important information you're giving to the AI. It's using special markers to tell the AI, "This is the only part you should pay attention to."
Example
prompt = """
Generate a Python script to create a basic HTML product listing page.
Requirements:
* Use hardcoded product data (name, description, price).
* Generate HTML with product info and "Add to Cart" buttons.
* Include a `calculate_total` function (error handling for non-numbers).
Example Product Data: `[{'name': 'A', 'desc': 'B', 'price': 10}, {'name': 'C', 'desc': 'D', 'price': 20}]`
<Output HTML Structure:> `<h1>Products</h1> <h2>{ProductName}</h2> <p>{Description}</p> <p>{Price}</p> <button>Add to Cart</button>`
"""
Refinement & Re-prompting (Iterating for Better Responses)
Imagine you're sculpting clay. You don't get the perfect statue on your first try! You shape it, look at it, see what's wrong, and then adjust it.
Concept Asking AI to refine its response based on quality. Refinement & Re-prompting is the same process, but with AI.
Example
basic_response = "The moon is bright."
refined_prompt = "Describe the moon poetically."
refined_response = "The moon, a glowing pearl in the cosmic ocean, bathes the world in silver light."
Reverse Prompting (Asking AI to Explain Its Response)
Imagine you ask a friend to write a story, and they give you something really weird. Instead of just saying "That's bad," you ask them, "Why did you write it that way? What were you thinking?". Reverse Prompting is doing the same thing with AI.
Concept Asking the AI why it responded a certain way.
Explain its reasoning: "Why did you choose this answer?" "What information did you use to make this decision?"
Justify its choices: "Why is this the best solution?" "What are the pros and cons of this approach?"
Identify assumptions: "What assumptions did you make when answering this question?"
Example
response = "Renewable energy reduces costs due to lower operational expenses."
follow_up = f"You said: {response}. Can you explain why?"
explanation = "Unlike fossil fuels, solar and wind energy have minimal ongoing costs after installation."
Combining Multiple Prompting Techniques
Example 1 - Role-based + Chain-of-Thought + Formatting Control
Prompt
prompt = """
You are a math professor.
Explain how to solve a quadratic equation step by step.
Use bullet points.
"""
prompt = """
You are a math professor explaining the quadratic formula to a student.
First, break down the formula step-by-step, explaining each component (a, b, c, the square root, etc.) and its purpose. Show the formula itself.
Then, provide a simple example problem (e.g., x^2 + 2x + 1 = 0) and demonstrate how to apply the formula to solve it, showing each step clearly.
Finally, summarize the key takeaways in bullet points.
Output the entire explanation in Markdown format.
"""
Example 2 - Few-shot + Role-based + Formatting Control
Prompt
prompt = """
You are a career coach.
Here are two examples of good LinkedIn summaries.
Now, create one for a cybersecurity expert.
"""
Example 3 - Persona-based + Self-Consistency + Reverse Prompting
Prompt
prompt = """
Act like Albert Einstein and explain relativity.
Generate three explanations,
then pick the best one and explain why.
"""
Ultimate Example: Using All Techniques Together
Prompt
prompt = """
You are an expert travel agent specializing in budget-friendly European vacations.
**Task:** Recommend a 7-day itinerary for a solo traveler visiting Italy (Rome, Florence, Venice) on a budget of $700 USD (excluding flights).
**Instructions:**
1. **Chain-of-Thought:** First, research affordable accommodation options (hostels, budget hotels) in each city. Then, identify free or low-cost activities (walking tours, museums with free days). Next, suggest budget-friendly food options (street food, local markets). Finally, create a day-by-day itinerary incorporating these elements.
2. **Delimiting Input:** Only use the information provided in this prompt. Do not access external websites.
3. **Formatting Control:** Output the itinerary as a JSON object with the following structure:
```json
{
"title": "Budget Italy Itinerary (7 Days)",
"budget": 700,
"traveler": "Solo",
"cities": ["Rome", "Florence", "Venice"],
"itinerary": [
{"day": 1, "city": "Rome", "activities": ["...", "..."], "estimated_cost": 50},
{"day": 2, "city": "Rome", "activities": ["...", "..."], "estimated_cost": 45},
// ... (rest of the itinerary)
],
"summary": "...",
"assumptions": "...",
"reasoning": "..."
}
```
4. **Parameter Control:** (Implicit - relies on model's default settings, but could be explicitly set if needed. For example, "Temperature: 0.5" to balance creativity and accuracy).
5. **Reverse Prompting:** After generating the JSON itinerary, briefly explain your reasoning for choosing these specific activities and accommodations. Also, list any assumptions you made about the traveler's preferences (e.g., preferred travel style, food preferences).
"""
Prompt
prompt = """
You are a seasoned software architect designing a microservices architecture for an e-commerce platform.
Task: Outline the key microservices needed for the platform, their responsibilities, and how they interact.
Instructions:
Chain-of-Thought: First, identify the core business functions of an e-commerce platform (e.g., product catalog, order management, payment processing). Then, design a microservice for each function, defining its specific responsibilities and data model. Next, describe how these microservices will communicate with each other (e.g., REST APIs, message queues). Finally, consider potential challenges and solutions related to distributed systems (e.g., data consistency, fault tolerance).
Role-Based: As a software architect, prioritize scalability, maintainability, and resilience in your design.
Delimiting Input: Focus solely on the microservice architecture. Do not include details about the front-end or specific technologies.
Formatting Control: Output the design as a JSON object with the following structure:
{
"platform": "E-commerce",
"architecture": "Microservices",
"microservices": [
{
"name": "ProductCatalogService",
"responsibilities": ["...", "..."],
"data_model": "{...}",
"communication": "..."
},
{
"name": "OrderManagementService",
"responsibilities": ["...", "..."],
"data_model": "{...}",
"communication": "..."
},
// ... (rest of the microservices)
],
"challenges": ["...", "..."],
"solutions": ["...", "..."]
}
Reverse Prompting: After generating the JSON output, briefly explain your rationale for choosing this particular microservice decomposition. What were the key considerations that influenced your design decisions?
"""
Project AI-Powered Recipe Generator
Building the Flask API
This project demonstrates how to build an AI-powered recipe generator using Flask and OpenAI API. The API generates Indian recipes based on mood, age, and location.
Step 1 Write API Code with Flask
from flask import Flask, request, jsonify
import openai
import json
app = Flask(__name__)
OPENAI_API_KEY = "your_openai_api_key"
openai.api_key = OPENAI_API_KEY
def generate_recipe_with_ai(mood, age, city):
prompt = f"""Generate an Indian recipe suitable for someone who is:
- Feeling: {mood}
- Age: {age} years old
- Location: {city}, India
Create a recipe that:
1. Uses ingredients commonly available in {city}
2. Matches the mood '{mood}'
3. Is age-appropriate for {age} years old
4. Reflects local Indian cooking styles
Format the response EXACTLY as a JSON object with this structure:
{{
"name": "Recipe Name (include Indian name if applicable)",
"prepTime": "preparation time in minutes",
"ingredients": [
"ingredient 1 with quantity",
"ingredient 2 with quantity"
],
"instructions": "1. First step\n2. Second step\n3. Third step",
"mood": "{mood}"
}}
Return ONLY the JSON object without any additional text or formatting."""
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "system", "content": "You are an expert Indian chef."},
{"role": "user", "content": prompt}]
)
try:
response_text = response["choices"][0]["message"]["content"].strip()
recipe_data = json.loads(response_text)
return recipe_data
except Exception as e:
return {"error": "Failed to generate a valid recipe.", "details": str(e)}
@app.route("/generate-recipe", methods=["POST"])
def generate_recipe():
try:
data = request.get_json()
mood = data.get("mood")
age = data.get("age")
city = data.get("city")
if not mood or not age or not city:
return jsonify({"error": "Missing required fields: mood, age, city."}), 400
recipe = generate_recipe_with_ai(mood, age, city)
return jsonify(recipe)
except Exception as e:
return jsonify({"error": "Internal Server Error", "details": str(e)}), 500
if __name__ == "__main__":
app.run(debug=True)
Step 2 Install Dependencies
pip install flask openai
Step 3 Start the Server
python app.py
Testing the API with Postman / Thunder Client
Step 1 Send a Request
Method:
POST
URL:
http://127.0.0.1:5000/generate-recipe
Headers:
Content-Type: application/json
Body (JSON)
{
"mood": "Happy",
"age": 25,
"city": "Mumbai"
}
Step 2 Expected Response
{
"name": "Masala DosaKhichdi",
"prepTime": "30 minutes",
"ingredients": [
"Rice - 2 cups",
"Urad dal - 1 cup",
"Potato - 2 medium",
"Spices and oil1 cup rice",
"1/2 cup lentils",
"1 tsp turmeric",
"1 tsp cumin seeds",
"1 tbsp ghee",
"Salt to taste"
],
"instructions": "1. Soak rice and dal\n2. Grind into batter\n3. Cook dosa and prepare masala filling, Rinse rice and lentils.\n2. Heat ghee and add cumin.\n3. Add rice, lentils, and spices.\n4. Add water and cook until soft.",
"mood": "Happy"
}
Here is full web application https://moody-bomb.onrender.com
Conclusion
By mastering advanced prompt engineering techniques and implementing them in real-world applications like an AI-powered recipe generator, developers can harness the full potential of AI for structured and intelligent interactions.
Connect With Me
Twitter: Rock12231
GitHub: Rock12231
LinkedIn: Rock1223
Email: avinashkumar2rock@gmail.com
Subscribe to my newsletter
Read articles from Avinash Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
