How to Secure Your Web Applications: Best Practices

Table of contents
- How to Secure Your Web Applications: Best Practices
- 1. Use HTTPS Instead of HTTP
- 2. Validate and Sanitize User Input
- 3. Implement Strong Authentication and Authorization
- 4. Protect Against SQL Injection
- 5. Secure Your APIs
- 6. Regularly Update Dependencies
- 7. Use Content Security Policy (CSP)
- 8. Monitor and Log Activity
- 9. Perform Regular Security Audits
- 10. Educate Your Team
- Final Thoughts
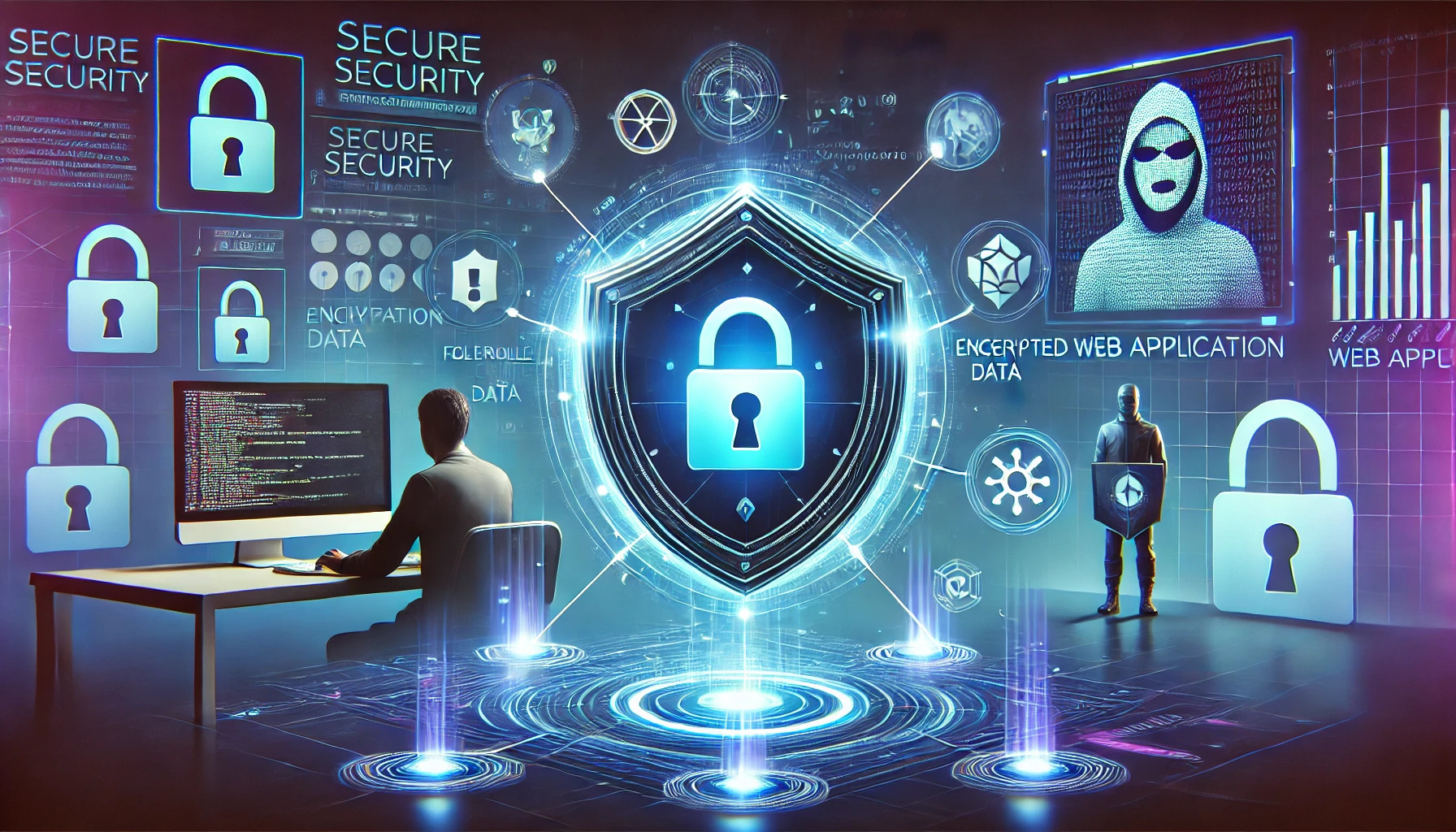
How to Secure Your Web Applications: Best Practices
In today’s digital age, web applications are the backbone of businesses, services, and online interactions. However, with the increasing reliance on web applications comes the growing threat of cyberattacks. From data breaches to DDoS attacks, the risks are real and can have devastating consequences. Securing your web applications is no longer optional—it’s a necessity. In this article, we’ll explore the best practices for securing your web applications and ensuring your users’ data remains safe.
If you’re looking to monetize your programming or web development skills, consider joining MillionFormula. It’s a free platform where you can make money online without needing credit cards or debit cards. Now, let’s dive into the best practices for securing your web applications.
1. Use HTTPS Instead of HTTP
One of the most fundamental steps in securing your web application is to use HTTPS (Hypertext Transfer Protocol Secure) instead of HTTP. HTTPS encrypts the data transmitted between the user’s browser and your server, preventing attackers from intercepting sensitive information.
To implement HTTPS, you need an SSL/TLS certificate. Many hosting providers offer free SSL certificates through services like Let’s Encrypt. Here’s how you can force HTTPS in an Express.js application:
javascript
Copy
const express = require('express');
const app = express();
// Redirect HTTP to HTTPS
app.use((req, res, next) => {
if (!req.secure) {
return res.redirect(</span><span class="token string">https://</span><span class="token interpolation"><span class="token interpolation-punctuation punctuation">${</span>req<span class="token punctuation">.</span>headers<span class="token punctuation">.</span>host<span class="token interpolation-punctuation punctuation">}</span></span><span class="token interpolation"><span class="token interpolation-punctuation punctuation">${</span>req<span class="token punctuation">.</span>url<span class="token interpolation-punctuation punctuation">}</span></span><span class="token template-punctuation string">);
}
next();
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
2. Validate and Sanitize User Input
User input is one of the most common attack vectors for web applications. Attackers can exploit vulnerabilities like SQL injection, cross-site scripting (XSS), and command injection by injecting malicious code through input fields.
Always validate and sanitize user input on both the client and server sides. For example, use libraries like validator.js in Node.js to sanitize input:
javascript
Copy
const validator = require('validator');
const userInput = '<script>alert("XSS Attack!")</script>';
const sanitizedInput = validator.escape(userInput);
console.log(sanitizedInput); // Output: <script>alert("XSS Attack!")</script>
3. Implement Strong Authentication and Authorization
Weak authentication mechanisms can lead to unauthorized access. Use multi-factor authentication (MFA) to add an extra layer of security. Additionally, implement role-based access control (RBAC) to ensure users only have access to the resources they need.
For example, in a Node.js application, you can use Passport.js for authentication:
javascript
Copy
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
passport.use(new LocalStrategy(
(username, password, done) => {
// Verify user credentials
User.findOne({ username: username }, (err, user) => {
if (err) return done(err);
if (!user) return done(null, false);
if (!user.verifyPassword(password)) return done(null, false);
return done(null, user);
});
}
));
app.post('/login', passport.authenticate('local', {
successRedirect: '/dashboard',
failureRedirect: '/login',
}));
4. Protect Against SQL Injection
SQL injection occurs when attackers manipulate SQL queries by injecting malicious code. To prevent this, use parameterized queries or prepared statements. Most modern ORMs (Object-Relational Mapping) libraries, like Sequelize for Node.js, handle this automatically.
Here’s an example of a parameterized query using Sequelize:
javascript
Copy
const { User } = require('./models');
const username = 'admin';
const password = 'password123';
User.findOne({
where: {
username: username,
password: password,
},
}).then(user => {
console.log(user);
});
5. Secure Your APIs
APIs are a critical component of modern web applications, but they can also be a target for attackers. Use API keys, OAuth, or JWT (JSON Web Tokens) to secure your APIs. Always validate and sanitize API requests and responses.
Here’s an example of securing an API with JWT in Node.js:
javascript
Copy
const jwt = require('jsonwebtoken');
const express = require('express');
const app = express();
app.post('/login', (req, res) => {
const user = { id: 1, username: 'admin' };
const token = jwt.sign(user, 'your-secret-key', { expiresIn: '1h' });
res.json({ token });
});
app.get('/protected', (req, res) => {
const token = req.headers['authorization'];
if (!token) return res.status(401).send('Access Denied');
jwt.verify(token, 'your-secret-key', (err, user) => {
if (err) return res.status(403).send('Invalid Token');
res.json({ message: 'Protected Route', user });
});
});
6. Regularly Update Dependencies
Outdated libraries and frameworks can contain vulnerabilities that attackers can exploit. Regularly update your dependencies and use tools like npm audit or Dependabot to identify and fix security issues.
7. Use Content Security Policy (CSP)
CSP is a security feature that helps prevent XSS attacks by controlling which resources (scripts, styles, etc.) can be loaded on your web page. Implement CSP by adding the following HTTP header: http Copy
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted.cdn.com; style-src 'self' https://trusted.cdn.com;
8. Monitor and Log Activity
Monitoring and logging are essential for detecting and responding to security incidents. Use tools like Sentry or LogRocket to track errors and user activity.
9. Perform Regular Security Audits
Conduct regular security audits to identify and fix vulnerabilities. Use tools like OWASP ZAP or Burp Suite to test your application’s security.
10. Educate Your Team
Security is a team effort. Educate your developers, QA testers, and other stakeholders about secure coding practices and the latest security threats.
Final Thoughts
Securing your web applications is an ongoing process that requires vigilance and proactive measures. By following these best practices, you can significantly reduce the risk of cyberattacks and protect your users’ data.
If you’re looking to monetize your programming or web development skills, check out MillionFormula. It’s a free platform where you can make money online without needing credit cards or debit cards. Start securing your web applications today and take your skills to the next level!
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
