Go Error Handling

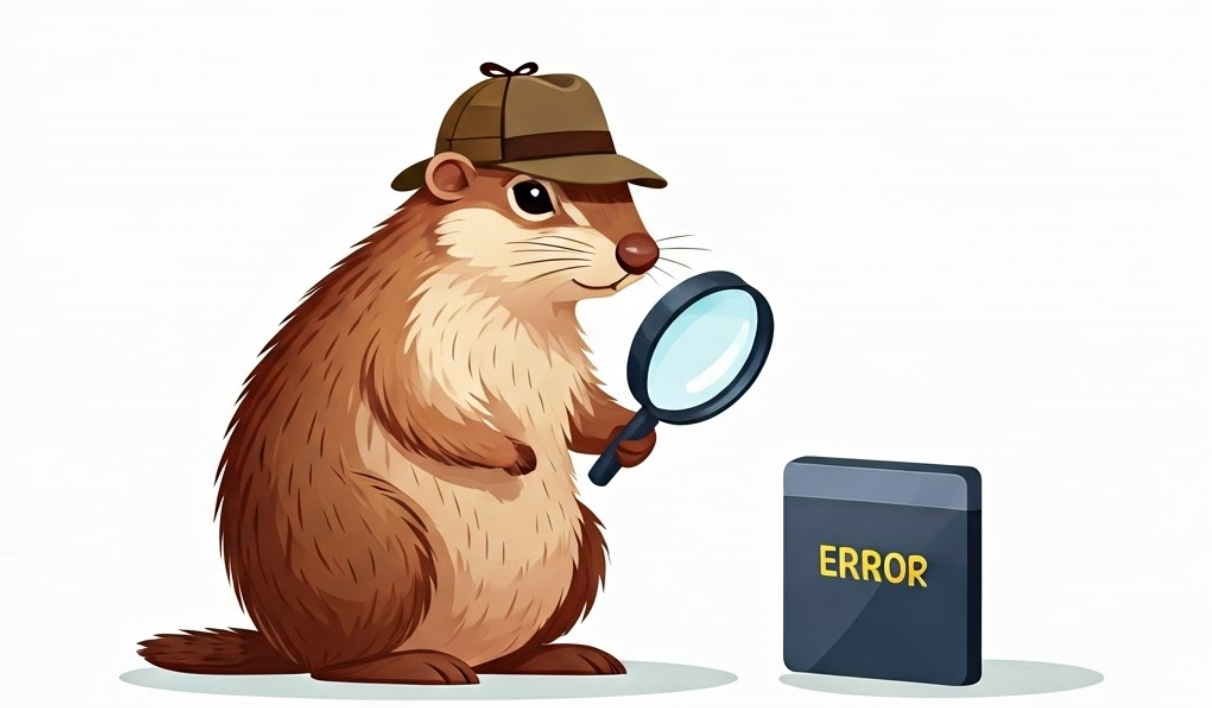
Go's error handling is unique and efficient. Errors aren't exceptions that halt execution; instead, functions return an error value alongside their result. This allows for fine-grained control over how errors are handled. Here's a simple example:
func divide(a, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("division by zero")
}
return a / b, nil
}
This divide
function returns an error
if b
is zero. The caller must explicitly check for this error. This explicit error checking enhances code clarity and prevents unexpected crashes.
result, err := divide(10, 2)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
Go's error
type is an interface, allowing for flexibility in error representation. Custom error types can be created to provide more context or detail. This example demonstrates the use of a custom error:
type MyError struct {
Message string
}
func (e *MyError) Error() string { return e.Message }
func myFunction() error {
return &MyError{Message: "Something went wrong!"}
}
This MyError
type provides a structured way of representing specific errors. The Error()
method satisfies the error
interface requirement, allowing it to be used just like any other error value. The practice of returning errors as function parameters encourages mindful and robust error management practices.
The errors
package provides useful functions for working with errors, such as errors.Is
and errors.As
, facilitating complex error checks without writing verbose conditional statements. A good understanding of error handling enables building secure, robust, and maintainable applications in Go.
Subscribe to my newsletter
Read articles from Hossein Margani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
