Mastering SOLID Principles in React: Writing Scalable and Maintainable Code
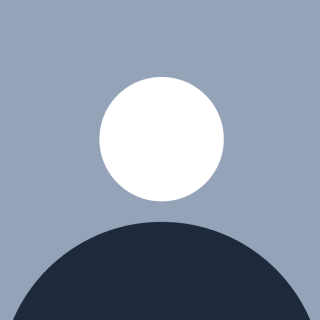
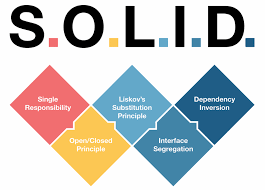
Modern web development demands robust, scalable, and maintainable applications. React, being one of the most popular frontend libraries, provides a powerful way to build user interfaces. However, as applications grow, codebases often become tangled, difficult to maintain, and prone to bugs. To tackle these challenges, developers can apply the SOLID principles. The SOLID principle is a set of five design principles that promote cleaner, more efficient, and scalable software architecture.
In this article, we will explore how to apply the SOLID principles “Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion” to React applications.
Single Responsibility Principle (SRP)
A component should have only one reason to change.
In React, this means ensuring that a component is responsible for only one functionality. Mixing concerns, such as fetching data and rendering UI in the same component, can make it harder to maintain and test.
Example of SRP Violation:
function UserProfile() {
const [user, setUser] = useState(null);
useEffect(() => {
fetch('/api/user')
.then(res => res.json())
.then(setUser);
}, []);
return <div>{user?.name}</div>;
}
The UserProfile
component is responsible for both fetching data and rendering UI, which violates SRP. This method makes it dificult for the result to be dynamic and can easily cause vain repetition all over your code base.
Correcting SRP with a Custom Hook:
function useUserData() {
const [user, setUser] = useState(null);
useEffect(() => {
fetch('/api/user')
.then(res => res.json())
.then(setUser);
}, []);
return user;
}
function UserProfile() {
const user = useUserData();
return <div>{user?.name}</div>;
}
In contrast to the first example, this principle stores the data-fetching logic and userProfile
in a component which in turn can be used in any part of your code base. Now, useUserData
handles the data-fetching logic, while UserProfile
is solely responsible for rendering the UI.
Open/Closed Principle (OCP)
Components should be open for extension but closed for modification.
Rather than modifying existing components to add new functionality, we should design components to be easily extended.
Example of OCP Violation:
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
What if we need different button styles? Instead of modifying this component directly, we can use props to extend it.
Applying OCP with Props:
function Button({ label, onClick, variant = "primary" }) {
return <button className={`btn ${variant}`} onClick={onClick}>{label}</button>;
}
Now, we can extend the button by simply passing a different variant
without modifying the core Button
component.
Liskov Substitution Principle (LSP)
Subtypes must be substitutable for their base types.
In React, this means child components should maintain expected behavior when used in place of their parent components.
Example of LSP Violation:
function Input({ value, onChange }) {
return <input type="text" value={value} onChange={onChange} />;
}
function PasswordInput({ value, onChange }) {
return <input value={value} onChange={onChange} />;
}
Here, PasswordInput
does not maintain the expected behavior of an input component.
Correcting LSP:
function PasswordInput(props) {
return <Input {...props} type="password" />;
}
Now, PasswordInput
correctly extends Input
while maintaining expected behavior.
Interface Segregation Principle (ISP)
Avoid forcing components to accept props they don’t need.
This principle simple work agains force feeding components with props that they don’t need. React makes use of props to pass data from one component from another but sometimes, passing unnecessary props makes your code much complicated and in most cases aid unexpected behaviors for your app.
Example of ISP Violation:
function UserCard({ name, email, profilePicture, onClick, onDelete, onEdit }) {
return (
<div>
<img src={profilePicture} alt="Profile" />
<h2>{name}</h2>
<p>{email}</p>
<button onClick={onClick}>View</button>
<button onClick={onEdit}>Edit</button>
<button onClick={onDelete}>Delete</button>
</div>
);
}
The UserCard
component forces all implementations to define onClick
, onDelete
, and onEdit
, even if they are unnecessary.
Applying ISP with Composition:
function UserCard({ name, email, profilePicture, children }) {
return (
<div>
<img src={profilePicture} alt="Profile" />
<h2>{name}</h2>
<p>{email}</p>
{children}
</div>
);
}
Now, actions like edit and delete can be added separately as needed:
<UserCard name="John" email="john@example.com" profilePicture="/john.jpg">
<button onClick={handleEdit}>Edit</button>
<button onClick={handleDelete}>Delete</button>
</UserCard>
Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules directly. Instead, depend on abstractions.
Example of DIP Violation:
function UserProfile() {
const [user, setUser] = useState(null);
useEffect(() => {
fetch('/api/user').then(res => res.json()).then(setUser);
}, []);
return <div>{user?.name}</div>;
}
The UserProfile
component is tightly coupled to the API call.
Applying DIP with Dependency Injection:
function UserProfile({ getUser }) {
const [user, setUser] = useState(null);
useEffect(() => {
getUser().then(setUser);
}, [getUser]);
return <div>{user?.name}</div>;
}
Now, we can inject different data sources when needed:
function App() {
const getUser = () => fetch('/api/user').then(res => res.json());
return <UserProfile getUser={getUser} />;
}
Conclusion
Applying SOLID principles in React development leads to cleaner, scalable, and maintainable applications. By ensuring single responsibility, open/closed extensibility, substitutable components, interface segregation, and dependency inversion, we create a robust architecture that is easy to test and extend.
Resources
React Official Documentation (for best practices on component structure)
SOLID Principles Explained with Examples – by freeCodeCamp
Subscribe to my newsletter
Read articles from Olusanya Agbesanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Olusanya Agbesanya
Olusanya Agbesanya
Hey, I'm Toye, a frontend developer and technical writer passionate about building scalable web apps and crafting clear, developer-friendly documentation. I write about React, APIs, and modern web development, making complex topics easy to grasp.