How to Use JavaScript Executor in Selenium for Web Automation

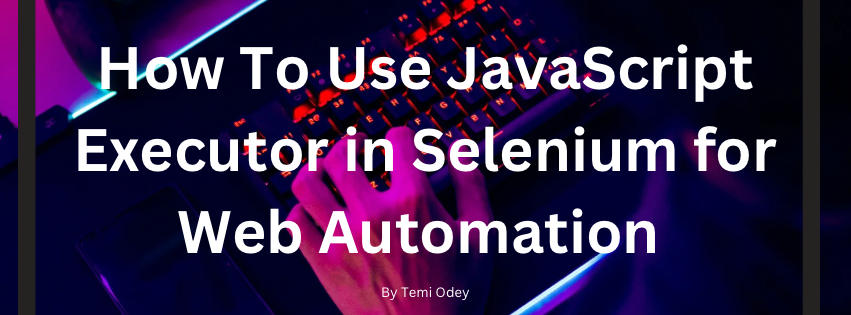
When performing web automation tests using Selenium, we interact with web elements and execute various WebDriver methods, such as get("String")
for navigating to URLs, click()
for interacting with buttons and links, and sendKeys()
for entering data. However, these actions may occasionally result in exceptions when running tests in Selenium.
These exceptions may arise due to underlying causes such as unexpected window pop-ups that prevent the WebDriver from locating the expected elements or hidden elements interfering with the intended web element, causing an action—such as a click—to fail. When this happens, the console displays exception messages like:
NoSuchElementException
(Element not found)ElementClickInterceptedException
(Click intercepted by another element)
To resolve such issues, we can use JavaScript Executor, as JavaScript interacts directly with the HTML structure of a web application, making it highly effective for handling such exceptions.
What is JavaScript Executor?
JavaScript Executor is a Selenium interface that enables us to execute JavaScript in web automation. It allows us to manipulate the DOM, interact with hidden elements, and perform operations that may not be possible using standard WebDriver methods.
To use JavaScript Executor in Selenium, after creating a test class, you need to initialize it by importing the JavaScript Executor package into your Java class:
javaCopy codeimport org.openqa.selenium.JavascriptExecutor;
In your script, create the following syntax:
javaCopy codeJavascriptExecutor js = (JavascriptExecutor) driver;
This indicates that the WebDriver instance can execute JavaScript, providing access to JavaScript-based interactions.
Components of JavaScript Executor in Selenium
There are two main methods for executing JavaScript commands in Selenium automation:
1. executeScript
Method – executeScript();
This method executes JavaScript in the context of the current window or frame, allowing direct interaction with elements across a web application. It takes a script and arguments as parameters, executing an anonymous function. If the script has a return statement, the return type depends on the data type:
Returns
Long
(for decimal and non-decimal data values)Returns
WebElement
(for an HTML element)Returns
List<WebElement>
(for multiple HTML elements)Returns
Boolean
(for a Boolean data type)
2. executeAsyncScript
Method – executeAsyncScript();
This executes an asynchronous JavaScript snippet on the current window or frame. It is particularly useful for handling dynamically loading elements and delayed actions, as it allows the script to run in the background while the webpage continues loading. This improves page load performance and responsiveness.
Test Case Example Using JavaScript Executor
Let’s consider a sample test case where we automate a function test on a product page selecting some items to cart using TestNG.
Preconditions:
Load URL: www.saucedemo.com
Enter username:
standard_user
Enter password:
secret_sauce
Click the Login button
Test Steps:
Click the item "Sauce Labs Backpack" to add it to the cart
Click the item "Sauce Labs Onesie" to add it to the cart
Postconditions:
- Quit the browser
The test can be executed using JavaScript Executor in Selenium as follows:
package ui;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.JavascriptExecutor;
import io.github.bonigarcia.wdm.WebDriverManager;
public class SauceDemoProductTest {
public static void main(String[] args) throws InterruptedException {
//chrome browser setup
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
JavascriptExecutor js = (JavascriptExecutor) driver;
driver.manage().window().maximize();
driver.get("https://www.saucedemo.com");
//enter username "standard_user" using javascript executor
js.executeScript("document.getElementById('user-name').value='standard_user';");
//enter password "secret_sauce" using javascript executor
js.executeScript("document.getElementById('secret_sauce').value='standard_user';");
//click login button
js.executeScript("document.getElementById('login-button').click();");
//Test steps - clicking items using their xpaths in javascript
// find webelemnt using xpath and then create script and input the webelement argument
WebElement backpack = driver.findElement(By.xpath("//*[@id="add-to-cart-sauce-labs-backpack"]"));
js.executeScript("argument[0].click();", backpack);
WebElement onesies = driver.findElement(By.xpath("//*[@id="add-to-cart-sauce-labs-onesies"]"));
js.executeScript("argument[0].click();", onesies);
//post-condition - quit browser
driver.quit();
}
}
Note: The above example illustrates JavaScript execution using a learning-based website - SauceDemo.
Scrolling to the Bottom of a Web Page Using executeAsyncScript
Another example is using the executeAsyncScript
method to scroll to the bottom of a webpage using TestNG. This can be done as follows:
package nameofchoice;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
import org.openqa.selenium.JavascriptExecutor;
public class ScrollTest {
@Test
public void scrollToBottom() {
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
JavascriptExecutor js = (JavascriptExecutor) driver;
driver.get("https://www.ebay.com");
driver.manage().window().maximize();
js.executeAsyncScript("window.scrollBy(0,document.body.scrollHeight)");
// The '0' represents the x-axis, while 'document.body.scrollHeight' represents the y-axis, enabling vertical scrolling.
}
}
Explanation:
The above script:
Opens the web browser and navigates to www.ebay.com
Maximizes the window
Uses
executeAsyncScript
to scroll down to the bottom of the page
Other Uses of JavaScript Executor
Besides the examples discussed above, the executeScript
and executeAsyncScript
methods can be used for various automation tasks, including:
Handling alert pop-ups
Retrieving page titles
Scrolling to specific elements
Clicking elements that are otherwise unclickable
When using the executeScript
method, most IDEs provide additional suggestions for functions that can be performed, making JavaScript Executor a versatile tool for Selenium automation.
Conclusion
Mastering JavaScript Executor methods is essential for Selenium testers. Utilizing both executeScript
and executeAsyncScript
provides an effective solution for efficiently handling potential issues when interacting with web elements and managing dynamic content. you can navigate complex automation scenarios with greater efficiency and precision. These techniques not only enhance your automation expertise but also improve the stability and reliability of your Selenium test scripts.
Ready to take your web automation skills to the next level? Start incorporating JavaScript Executor in your test scripts and watch your efficiency soar! 🚀
Subscribe to my newsletter
Read articles from Temi Odey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
