Algorithmic Trading Strategies for Bots
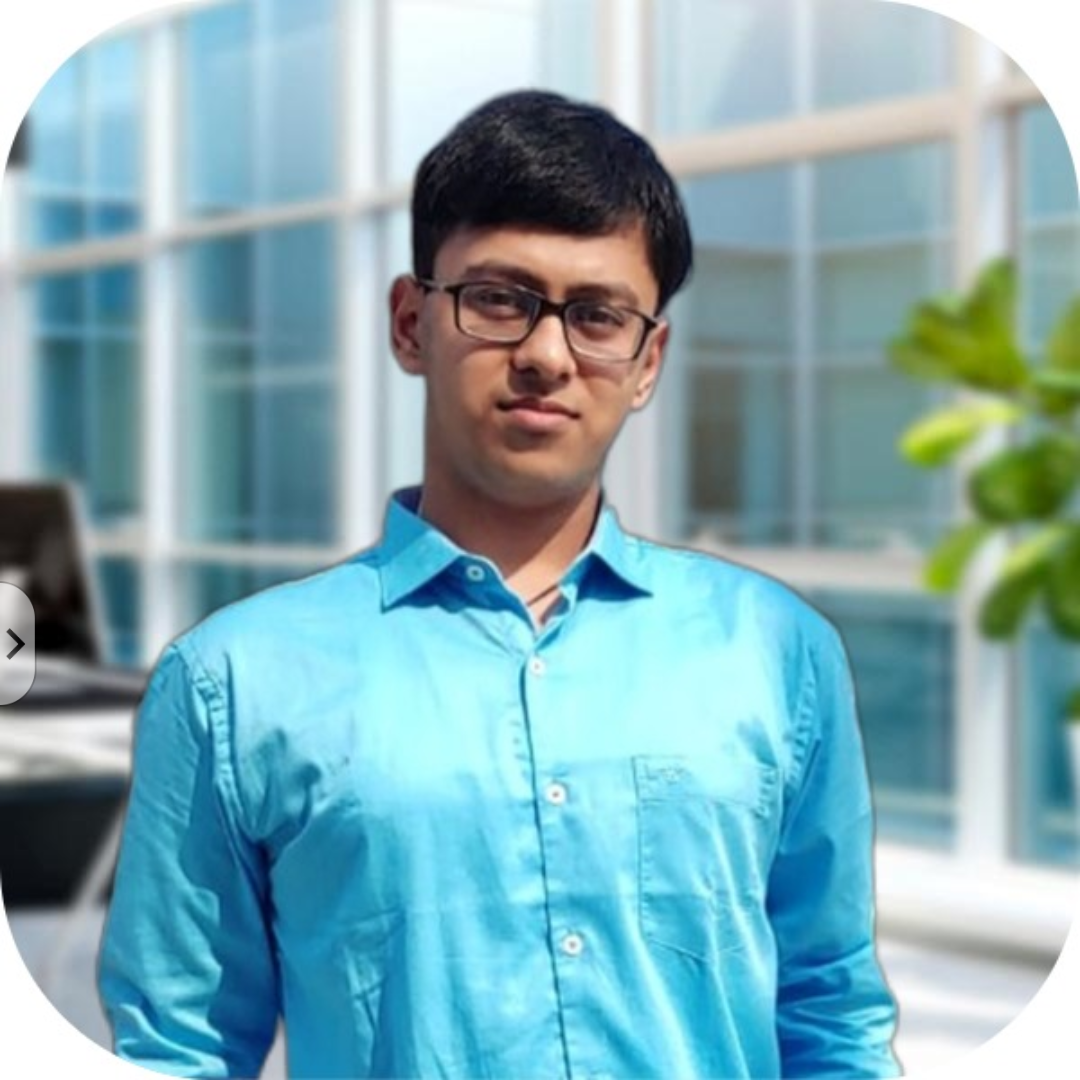
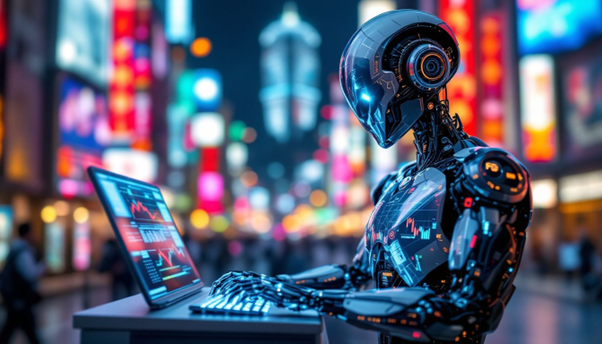
Detailed Trading Bot Strategy and Performance Summary
Our trading bot was meticulously crafted using a combination of advanced trading strategies, cutting-edge algorithmic techniques, and performance optimization methods. The goal was to create a bot capable of adapting to diverse market conditions, optimizing risk-to-reward ratios, and delivering consistent performance. Below, we outline the trading strategies and methodologies used, detailing their contribution to the bot's overall success and performance.
1. Momentum Trading
Objective: To capture market trends and trade in the direction of price movements.
How It Works: Momentum trading is predicated on the principle that assets experiencing strong trends (upward or downward) are likely to continue in that direction. The bot identifies these trends and makes trades based on market momentum.
Technical Indicators Used:
Moving Averages (SMA and EMA): These indicators help identify the prevailing market trend. The crossover of short-term moving averages above longer-term averages signals upward momentum (buy signal), while the reverse indicates downward momentum (sell signal).
Relative Strength Index (RSI): The RSI is used to determine whether a market is overbought (above 70) or oversold (below 30), helping the bot avoid entering trades when prices are likely to reverse.
Signal Generation:
Buy Signal: When the market exhibits upward momentum (rising prices with a short-term moving average crossing above a long-term moving average), the bot initiates a buy.
Sell Signal: Conversely, the bot sells when downward momentum is detected (prices dropping with a short-term moving average crossing below a long-term moving average).
prices = np.array([1.105, 1.110, 1.115, 1.120, 1.125, 1.130, 1.135]) |
Impact: Momentum trading enabled the bot to effectively identify profitable opportunities in trending markets, capitalize on rapid price movements, and maximize returns by staying aligned with prevailing market trends.
2. Grid Trading
Objective: To profit from price fluctuations in range-bound or sideways markets.
How It Works: Grid trading places buy and sell orders at predetermined intervals around the current market price. The bot profits from price oscillations within a set price range rather than relying on long-term trends.
Grid Setup:
- The bot establishes a grid by defining price intervals, such as 100 pips (for forex markets). Orders are automatically placed above and below the current market price within this range.
class GridTradingBot: |
Profit Mechanism:
- The bot buys when the price drops to a lower grid level and sells when it rises to a higher level. This enables the bot to profit from smaller price fluctuations in non-trending markets.
Impact: Grid trading was particularly advantageous in range-bound markets where prices lacked strong directional trends. This strategy allowed the bot to generate consistent profits from smaller, more frequent price movements, delivering steady returns in stagnant market conditions.
3. DCA (Dollar Cross Averaging) Trading
Objective: To capture large, long-term trends by leveraging moving average crossovers.
How It Works: DCA trading involves monitoring key moving average crossovers, such as the Golden Cross (bullish signal) and the Death Cross (bearish signal). The bot uses these crossovers to time entries and exits during significant market trends.
Signal Generation:
Golden Cross: A short-term moving average crossing above a long-term moving average signals a potential uptrend (buy signal).
Death Cross: A short-term moving average crossing below a long-term moving average signals a potential downtrend (sell signal).
Dynamic Adjustment: The bot adjusts its trade size based on the strength and duration of a trend, maximizing profits during strong uptrends by holding positions longer.
prices = pd.Series([1.105, 1.110, 1.115, 1.120, 1.125, 1.130, 1.135]) |
Impact: The DCX strategy enabled the bot to capture large market moves by staying in the market during major trends, ensuring the bot benefitted from sustained price shifts while avoiding premature exits.
4. 30-70 RIS Trading Strategy
Objective: To optimize risk-to-reward ratios, ensuring higher probability of profitable trades.
How It Works: The 30-70 RIS strategy maintains a fixed risk-to-reward ratio of 30% risk (probability of loss) and 70% reward (probability of gain) per trade. This approach was grounded in statistical modeling and real-time market analysis to fine-tune trade execution.
Risk Assessment: The bot calculates potential risk based on market volatility and adjusts position sizes accordingly, ensuring that the risk of loss remains within 30% for each trade.
Reward Optimization: The bot ensures that each trade offers a minimum of 70% reward potential by focusing on favorable risk-to-reward profiles, considering historical data and volatility patterns.
risk_percentage = 0.30 # |
Impact: This strategy provided a balanced approach to risk management, preventing high-risk trades and prioritizing those with a strong likelihood of profitable outcomes, improving the bot’s win rate and profitability in volatile markets.
5. Sharpe Ratio Optimization and Algorithmic Performance Tuning
Objective: To improve risk-adjusted returns and enhance the bot’s overall performance.
Sharpe Ratio Calculation: The Sharpe ratio, a key metric for assessing risk-adjusted returns, was used to evaluate the bot’s performance relative to its risk. It is calculated as:
Optimization Goals:
- The goal was to maximize the Sharpe ratio, which would improve returns without excessively increasing risk. The bot was tuned to prioritize low-volatility, high-return strategies while avoiding high-risk trades.
Machine Learning: The bot leveraged machine learning to optimize its decision-making process, fine-tuning parameters like stop-loss, take-profit, and trade size based on historical data and real-time market analysis.
Performance Tuning: Backtesting and walk-forward testing were utilized to ensure that the bot’s performance was robust across various market conditions, making it adaptable and effective in different trading environments.
Impact: Through Sharpe ratio optimization and continuous algorithmic refinement, the bot maintained a high Sharpe ratio, delivering strong risk-adjusted returns even during periods of market volatility, enhancing its reliability for real-world trading applications.
Post-Hackathon Performance Analysis
After the hackathon concluded, we continued to monitor the bot’s performance in live markets. Despite encountering some technical challenges, particularly with the API, the bot demonstrated the following key outcomes:
- Consistent Returns: The bot delivered stable returns with low drawdowns, showcasing the efficacy of its momentum and grid trading strategies, especially during periods of market volatility. The DCX strategy also played a crucial role in capturing large, profitable trends.
- Effective Risk Management: The 30-70 RIS strategy ensured that only trades with favorable risk-to-reward ratios were executed, optimizing the bot’s profitability while minimizing potential losses.
Ongoing Optimization: Continuous fine-tuning of the Sharpe ratio and performance optimization algorithms allowed the bot to deliver consistent, high-risk adjusted returns, further solidifying its reliability for real-world trading scenarios.
Final Hybrid Trading Model
After multiple iterations and testing, we successfully refined our approach and developed a hybrid trading model that integrated Grid Trading with RSI-based entry points, Stop-Loss/Take-Profit (SL/TP) mechanisms, and dynamic risk management. This model aimed to create a more adaptive and dynamic strategy, capable of optimizing profits while minimizing downside risk.
Grid Strategy: Ensured continuous buy/sell orders within a predefined price range, capitalizing on market oscillations.
RSI-Based Entry Points: Allowed for better trade execution by identifying overbought and oversold conditions.
SL/TP Mechanism: Automated risk management, ensuring that losses were minimized and profits were locked in when price targets were reached.
Conclusion:
Our trading bot demonstrated a strong ability to adapt to various market conditions, optimize risk management, and consistently generate profitable trades. The strategies used Momentum Trading, Grid Trading, DCX, 30-70 RIS, and Sharpe Ratio optimization all contributed to the bot’s overall success. The live trade results further validated the bot’s potential, and we remain confident in its effectiveness for real-world trading applications.
Subscribe to my newsletter
Read articles from ADITYA BHATTACHARYA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
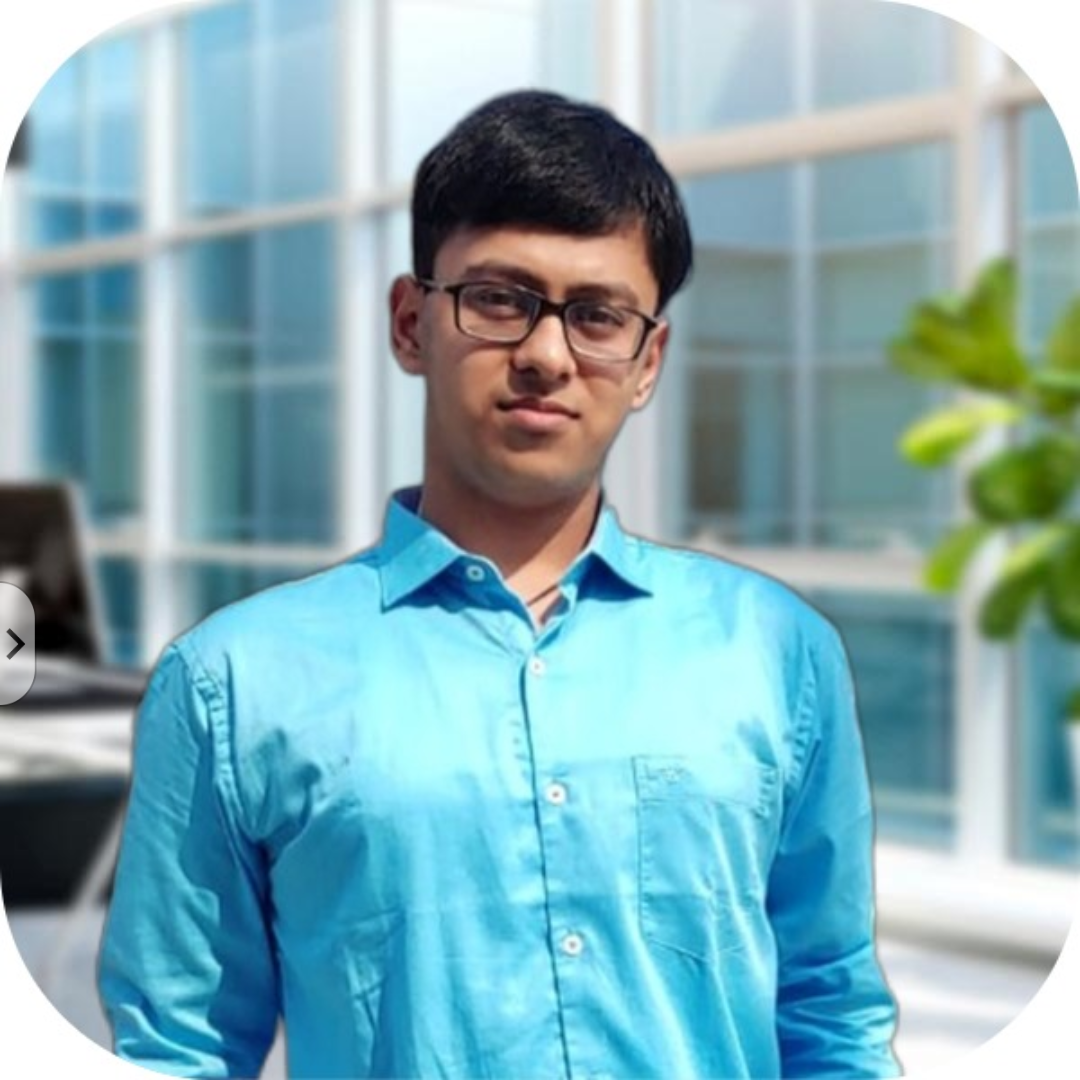
ADITYA BHATTACHARYA
ADITYA BHATTACHARYA
A fervent and a passionate fellow for Computer Science and Research, trying to bridge the gap between real life and code life. From print("Hello World ! ") to writing Scalable Language Codes, I am yet to discover the emerging future of AI in our forecasting life of Modern Technology.