Good Code vs. Bad Code: Understanding the Difference and Their Impact

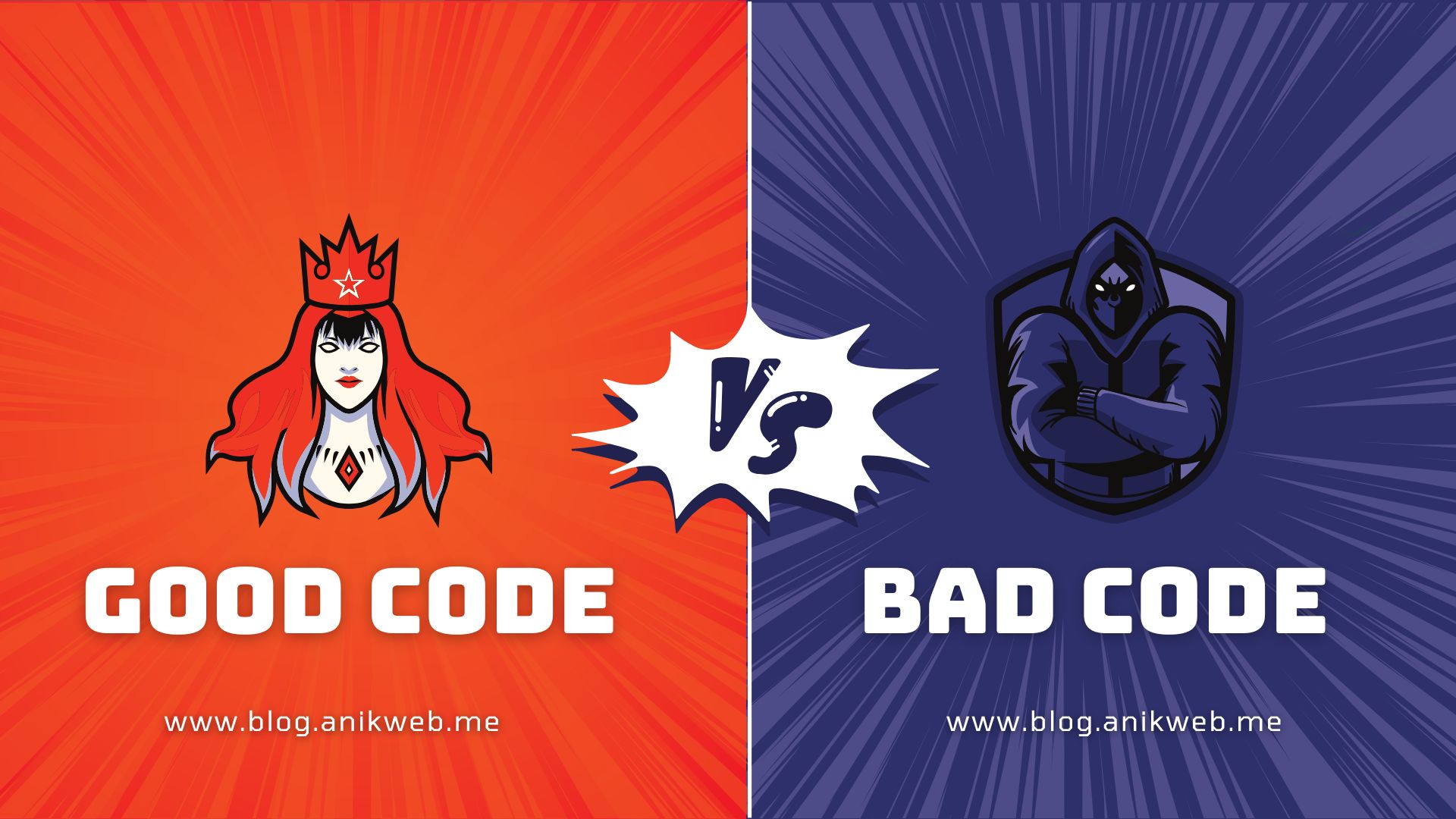
Introduction
In software development, the difference between good code and bad code can make or break a project. Good code ensures maintainability, scalability, and efficiency, while bad code can lead to technical debt, higher development costs, and frustration for both developers and users. In this blog, we will explore the key differences between good and bad code, their pros and cons, and best practices for writing better code.
What is Good Code?
Good code is well-structured, easy to read, and maintainable. It follows best coding practices and maintains a consistent style. Here are some key characteristics of good code:
Characteristics of Good Code
Readability – Good code is easy to read and understand.
Maintainability – It is easy to modify and extend.
Scalability – It can handle growth and increased workload efficiently.
Reusability – Good code is modular and can be reused in different parts of the application.
Performance Optimization – It runs efficiently without unnecessary processing overhead.
Error Handling – It includes proper exception handling to prevent crashes and unexpected behavior.
Consistency – Follows a uniform coding style and best practices.
Minimal Complexity – Avoids unnecessary complexity and keeps the logic simple.
Example of Good Code in PHP
class User {
private string $name;
private string $email;
public function __construct(string $name, string $email) {
$this->name = $name;
$this->email = $email;
}
public function getName(): string {
return $this->name;
}
public function getEmail(): string {
return $this->email;
}
}
This code is readable, structured, and follows object-oriented principles, making it easy to maintain and extend.
Example of Good Code in Laravel
use App\Models\User;
use Illuminate\Support\Facades\Log;
class UserController extends Controller {
public function getUserByEmail(string $email) {
try {
return User::where('email', $email)->firstOrFail();
} catch (ModelNotFoundException $e) {
Log::error("User not found: " . $email);
return response()->json(['error' => 'User not found'], 404);
}
}
}
This Laravel code follows best practices by using Eloquent ORM, handling exceptions, and logging errors properly.
Example of Good Code in JavaScript
async function fetchData(url) {
try {
let response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
return await response.json();
} catch (error) {
console.error('Fetch error:', error);
}
}
This JavaScript function is well-structured, includes proper error handling, and follows best practices for asynchronous programming.
Example of Good Code in MySQL
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(255) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
-- Using parameterized queries to prevent SQL injection
PREPARE stmt FROM 'SELECT * FROM users WHERE email = ?';
SET @email = 'user@example.com';
EXECUTE stmt USING @email;
This MySQL code creates a well-structured users
table and uses a parameterized query to prevent SQL injection, improving security and efficiency.
What is Bad Code?
Bad code is hard to understand, difficult to maintain, and often causes bugs and performance problems. It is typically written without following best practices.
Characteristics of Bad Code
Lack of Readability – Difficult to read and understand.
Poor Maintainability – Hard to update and fix bugs.
Performance Issues – Inefficient code that slows down execution.
No Error Handling – Leads to unexpected crashes.
Inconsistent Coding Style – Uses different conventions across the codebase.
Too Many Hardcoded Values – Reduces flexibility and increases maintenance overhead.
Code Duplication – Repeated code instead of using functions or reusable components.
Lack of Documentation – No comments or explanations, making it harder for others to understand.
Example of Bad Code in PHP
function getUser() {
$conn = new mysqli("localhost", "root", "", "users_db");
$result = $conn->query("SELECT * FROM users");
while ($row = $result->fetch_assoc()) {
echo $row["name"] . " - " . $row["email"] . "<br>";
}
$conn->close();
}
Problems with this Code:
No Error Handling – If the database connection fails, it will cause issues.
No Prepared Statements – Vulnerable to SQL injection attacks.
Hardcoded Database Credentials – A security risk.
Mixing Logic with Output – Should separate logic from presentation.
Example of Bad Code in Laravel
class UserController extends Controller {
public function getUserByEmail($email) {
$user = DB::select("SELECT * FROM users WHERE email = '$email'");
if (!$user) {
return "User not found";
}
return $user;
}
}
Problems with this Code:
SQL Injection Risk – Using raw SQL queries with user input is dangerous.
No Exception Handling – If the query fails, the function breaks.
Inconsistent Response – Returns a string instead of a structured JSON response.
No Logging – Errors are not logged, making debugging harder.
Example of Bad Code in JavaScript
function fetchData(url) {
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => alert("Something went wrong"));
}
Problems with this Code:
No Proper Error Handling – Only shows a generic alert instead of logging useful information.
Lack of Response Validation – Assumes the response will always be in JSON format without checking for errors.
No Return Value – Does not return a promise, limiting reusability.
Example of Bad Code in MySQL
SELECT * FROM users WHERE email = 'user@example.com';
Problems with this Code:
Vulnerable to SQL Injection – If user input is concatenated, it can be exploited.
No Indexing – Could lead to slow query performance in large databases.
No Parameterization – Should use prepared statements for security.
Impact of Good Code vs. Bad Code
Factor | Good Code | Bad Code |
Performance | Optimized and efficient | Slow and inefficient |
Maintainability | Easy to modify and extend | Difficult to update and debug |
Security | Follows best security practices | Prone to vulnerabilities |
Scalability | Can handle future growth | Struggles with increased load |
Collaboration | Easy for teams to understand and work with | Confusing and frustrating for developers |
Best Practices to Write Good Code
Follow Coding Standards – Use industry-standard guidelines such as PSR for PHP.
Write Modular Code – Break down large functions into smaller, reusable components.
Use Meaningful Names – Variable and function names should be descriptive.
Implement Proper Error Handling – Use try-catch blocks and meaningful error messages.
Use Version Control – Maintain code history using Git.
Write Unit Tests – Ensure your code works as expected.
Avoid Code Duplication – Use functions or classes instead of repeating code.
Write Documentation – Add comments and document your codebase for clarity.
Conclusion
Good code is the foundation of a successful software project, ensuring efficiency, security, and easy maintenance. Bad code, however, results in technical debt, security risks, and higher development costs. By following best practices and prioritizing code quality, developers can build strong and scalable applications that last over time.
Subscribe to my newsletter
Read articles from Anik Kumar Nandi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anik Kumar Nandi
Anik Kumar Nandi
Hi there! I'm Anik Kumar Nandi, passionate programmer, strongly focus on backend technologies. I specialize in PHP and Laravel, and I'm always exploring new ways to enhance the robustness and efficiency of my code. In addition to PHP and Laravel, my skill set includes RESTful API development, JavaScript, jQuery, Bootstrap, Ajax, and WordPress customization. Also deeply interested in AWS. Feel free to check out my GitHub profile and my portfolio site to learn more about my work and projects. Let's connect and create something amazing together!