How I Built My First React Native App: A Waste Management Solution

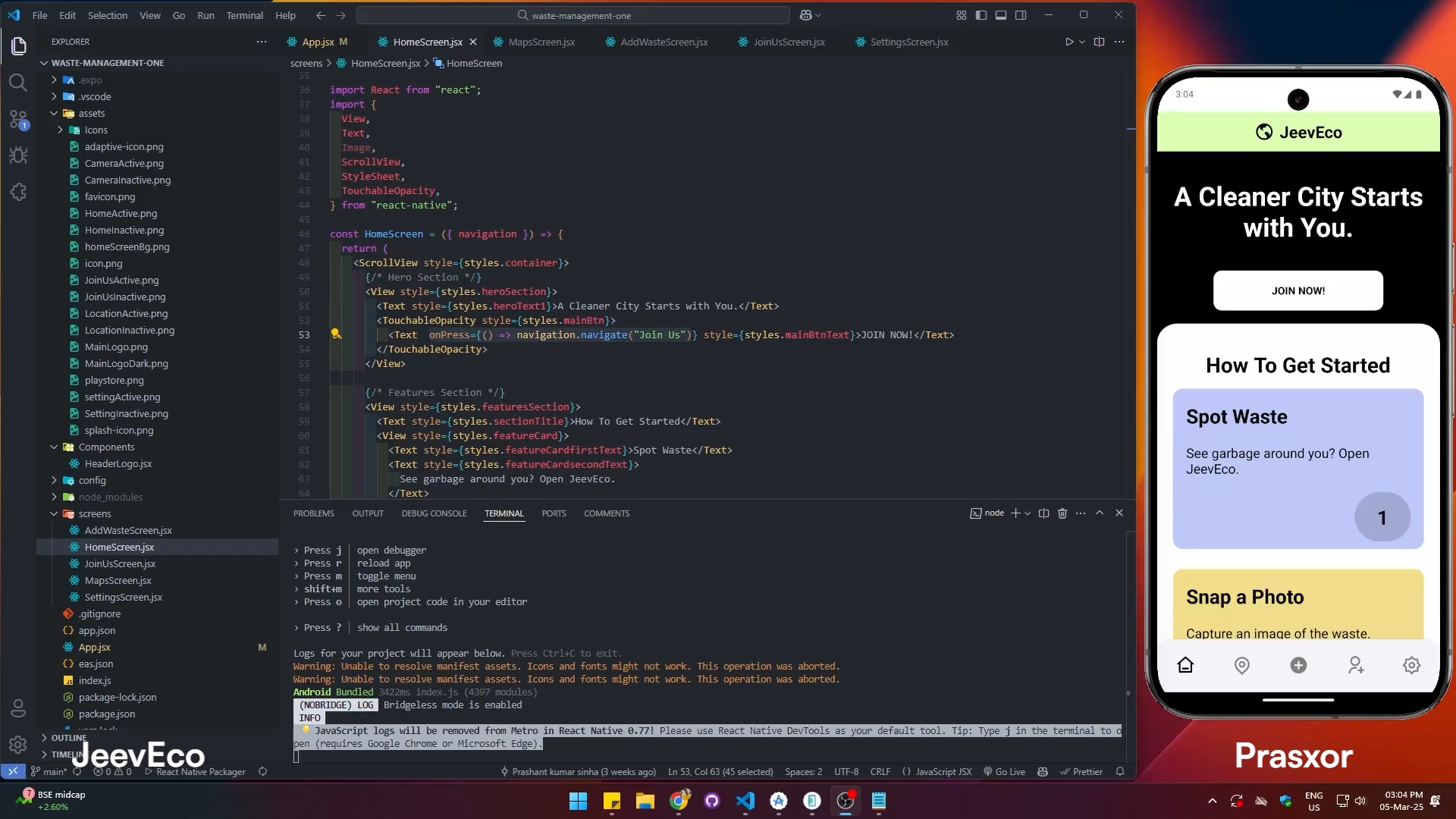
How I Built My First React Native App: A Waste Management Solution
Introduction
As a developer passionate about solving real-world problems, I recently built a waste management app using React Native and Firebase. The goal was to create a zero-budget solution where users can report waste locations and help keep their surroundings clean.
This blog post is a breakdown of my journey—what I learned, the challenges I faced, and how I built it.
Why Build a Waste Management App?
In India, waste disposal is a big issue. People often don’t know where to report garbage piles, and existing solutions are either inefficient or not widely used.
I wanted to create an app where:
✅ Anyone can report waste by uploading an image and location.
✅ Other users can track and clean waste from the map.
✅ No login/signup required to keep it simple.
Tech Stack Used
Since I was already working with React Native, I decided to use:
React Native: This is used to build the mobile UI.
Firebase: For storing waste reports.
Cloudinary: For storing images efficiently.
React Navigation: For handling screen transitions.
Expo Location API: To fetch user’s l’s location automatically.
Key Features
📸 1. Capture and Upload Waste Images
The first step was to allow users to take a photo of the waste. Clicking the "Add Waste" button directly opens the camera. Once the image is captured, it’s uploaded to Cloudinary, and the URL is stored in Firebase.
🔹 React Native Camera Implementation:
import { launchCamera } from 'react-native-image-picker';
const openCamera = () => {
launchCamera({ mediaType: 'photo' }, (response) => {
if (response.didCancel) return;
setImage(response.uri); // Save image URI in state
});
};
📍 2. Auto Fetch User’s Location
Instead of asking users to enter the waste location manually, I used Expo Location API to fetch their latitude and longitude automatically.
🔹 Fetching Location:
import * as Location from 'expo-location';
const getLocation = async () => {
let { status } = await Location.requestForegroundPermissionsAsync();
if (status !== 'granted') return;
let location = await Location.getCurrentPositionAsync({});
setLatitude(location.coords.latitude);
setLongitude(location.coords.longitude);
};
🗺️ 3. Displaying Waste Reports on a Map
The app uses React Native Maps to show reported waste locations. Clicking on a waste marker displays details like the image, time since reported, and cleanup status.
🔹 Rendering waste markers:
<MapView>
{wasteData.map((waste) => (
<Marker
coordinate={{ latitude: waste.lat, longitude: waste.lng }}
title="Waste Reported"
/>
))}
</MapView>
✅ 4. Users Can Mark Waste as "Cleaned"
Once a user cleans a waste spot, they can click "Mark as Cleaned," and the data updates in Firebase. This feature prevents duplicate reports.
Challenges I Faced & Lessons Learned
🚀 1. Handling Image Upload Efficiently
Initially, I stored images in Firebase Storage, but it consumed too much space. Switching to Cloudinary helped optimise storage while keeping Firebase lightweight.
📍 2. Accurate Location Fetching
Expo Location API sometimes returned incorrect lat/lng values indoors. The solution? Asking users to step outside for better GPS accuracy.
🔥 3. Keeping the App Lightweight
Since the app doesn't require authentication, I had to be careful about data integrity—ensuring users couldn't falsely mark waste as "cleaned" without verification.
Future Improvements
🔹 Add categories (dry, wet, mixed) for better waste classification.
🔹 Implement admin moderation to prevent false reports.
🔹 Allow volunteers to join cleanup drives through the app.
Conclusion
Building this waste management app was an exciting challenge. It strengthened my knowledge of React Native, Firebase, and real-world problem-solving.
If you're a beginner looking to build a project, I highly recommend working on something meaningful—not just a to-do app! It helps you learn, contribute, and showcase your skills.
👨💻 Would love to hear your thoughts! Have you ever built a real-world React Native project? Let’s discuss it! 🚀
Subscribe to my newsletter
Read articles from PrasxorKumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
