Expressions In Java

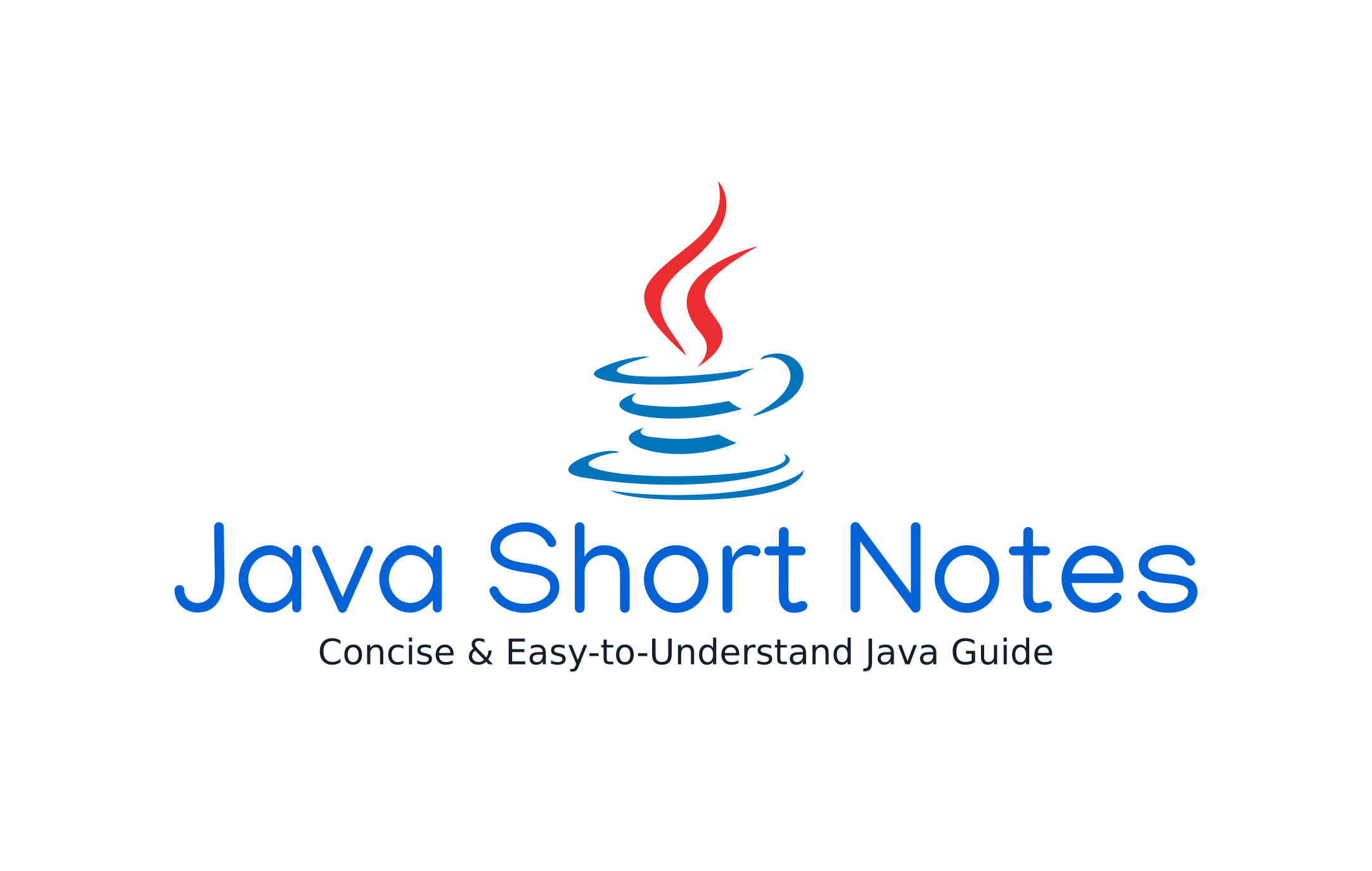
Introduction
In the previous lesson, we explored operators and their role in performing arithmetic, logical, and bitwise operations. Now, we will dive into expressions, which combine variables, constants, operators, and method calls to produce a value. Understanding expressions is fundamental to writing effective Java programs.
What is an Expression?
An expression is any valid combination of variables, constants, operators, and method calls that evaluates to a single value.
Example:
int result = 10 + 5; // Expression: 10 + 5 evaluates to 15
Java expressions can be categorized into several types, which include Arithmetic, Relational (Comparison), Logical, Bitwise, Assignment, Ternary and Method Call Expressions.
Arithmetic Expressions
These expressions use arithmetic operators (+
, -
, *
, /
, %
) to perform mathematical computations.
Example:
public class ArithmeticExpressions {
public static void main(String[] args) {
int a = 10, b = 5;
int sum = a + b; // Addition
int difference = a - b; // Subtraction
int product = a * b; // Multiplication
int quotient = a / b; // Division
int remainder = a % b; // Modulus (remainder)
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
System.out.println("Remainder: " + remainder);
}
}
/*
* Output
* Sum: 15
* Difference: 5
* Product: 50
* Quotient: 2
* Remainder: 0
*/
Relational (Comparison) Expressions
These expressions compare two values and return a boolean result (true
or false
) using relational operators.
Example:
public class RelationalExpressions {
public static void main(String[] args) {
int x = 10, y = 20;
System.out.println(x > y); // false
System.out.println(x < y); // true
System.out.println(x == y); // false
System.out.println(x != y); // true
}
}
Logical Expressions
These use logical operators (&&
, ||
, !
) to evaluate conditions.
Example:
public class LogicalExpressions {
public static void main(String[] args) {
boolean a = true, b = false;
System.out.println(a && b); // false
System.out.println(a || b); // true
System.out.println(!a); // false
}
}
Bitwise Expressions
Bitwise operators manipulate binary representations of numbers.
Example:
public class BitwiseExpressions {
public static void main(String[] args) {
int x = 5, y = 3; // Binary: 5 = 101, 3 = 011
System.out.println(x & y); // Bitwise AND (1)
System.out.println(x | y); // Bitwise OR (7)
System.out.println(x ^ y); // Bitwise XOR (6)
System.out.println(~x); // Bitwise NOT (-6)
System.out.println(x << 1); // Left shift (10)
System.out.println(y >> 1); // Right shift (1)
}
}
Assignment Expressions
Assignment expressions use =
and compound assignment operators (+=
, -=
, *=
, etc.).
Example:
public class AssignmentExpressions {
public static void main(String[] args) {
int a = 10;
a += 5; // a = a + 5;
a *= 2; // a = a * 2;
System.out.println("Value of a: " + a); // 30
}
}
/**
* Output:
* Value of a: 30
*/
Ternary Expressions
Ternary expressions use the ? :
operator for conditional evaluation.
Example:
public class TernaryExpression {
public static void main(String[] args) {
int num = 10;
String result = (num % 2 == 0) ? "Even" : "Odd";
System.out.println("Number is " + result);
}
}
/**
* Output:
* Number is Even
*/
Method Call Expressions
A method call itself is an expression because it returns a value.
Example:
public class MethodCallExpression {
public static int square(int num) {
return num * num;
}
public static void main(String[] args) {
int result = square(5); // Method call expression
System.out.println("Square: " + result); // 25
}
}
Conclusion
Expressions are the building blocks of any Java program. They enable computation, decision-making, and logical flow. Understanding different types of expressions will help you write cleaner, more efficient, and effective Java programs.
Stay tuned for the next topic in Java Short Notes! ๐
Subscribe to my newsletter
Read articles from Emmanuel Enchill directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emmanuel Enchill
Emmanuel Enchill
I am a passionate software engineer in training at ALX. I have a strong preference for back-end development. I am on course to share my learning journey with you.