Transfer tokens to "ANY" Solana wallet.
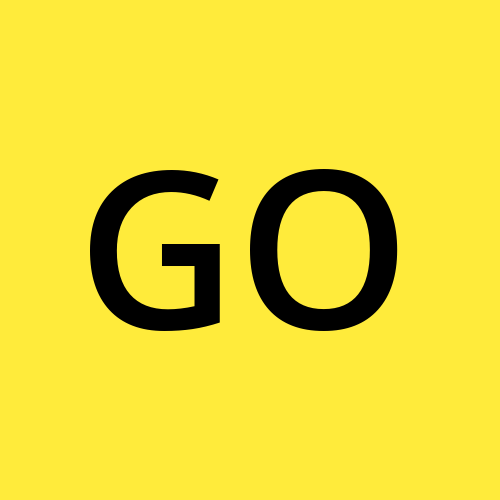
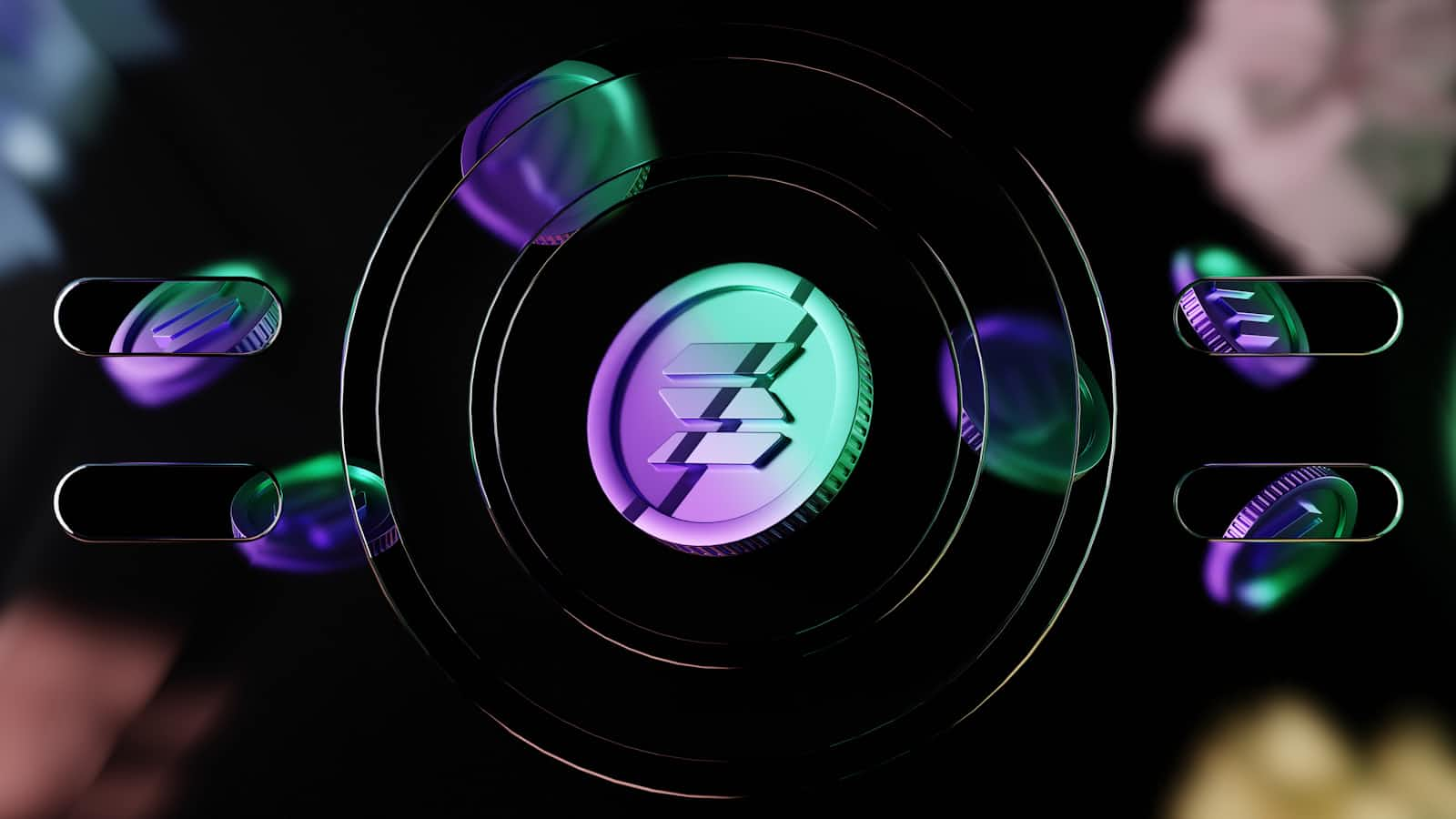
Introduction
Unlike other blockchains, Solana token model uses Associated Token Accounts (ATAs) to store information about a token, its balance, and its owner. This architecture enables Solana's high performance but requires a deeper understanding of the underlying mechanisms. This practical guide will walk through the code for transferring any token on Solana.
Pre-requisite
Before proceeding with this tutorial, make sure you have the following prerequisites:
Basic knowledge of TypeScript.
npm or yarn package manager installed. (I prefer yarn, so that’s what I’ll be using)
Understanding Solana Token Architecture
Before diving into the code, let's establish a clear mental model of how tokens work on Solana:
Token Mint: The definition of a token (like USDC, BONK, or RAY)
Token Account: Where token balances are actually stored
Wallet: Controls access to token accounts but doesn't directly hold tokens
On Solana, your wallet doesn't directly hold tokens. Instead, for each token type you own, you have a separate Token Account that your wallet controls.
Associated Token Accounts (ATAs): The Practical Solution
An Associated Token Account (ATA) is a special type of token account with an address that's deterministically derived from:
Your wallet address
The token's mint address
This creates a predictable mapping that anyone can calculate.
In TypeScript, here's how this mapping works:
import { getAssociatedTokenAddressSync, ASSOCIATED_TOKEN_PROGRAM_ID, TOKEN_PROGRAM_ID } from '@solana/spl-token';
// To find the ATA address for any wallet and token mint
const associatedTokenAddress = getAssociatedTokenAddressSync(
mintAddress, // The token's mint address
walletAddress // The wallet address
);
// Under the hood, this function calculates a PDA (Program Derived Address)
// using the wallet address, TOKEN_PROGRAM_ID, and mint address as seeds
Visually, the relationship looks like this:
Wallet Address Token Mint Address
↓ ↓
└────────┬────────┘
↓
Deterministic Calculation
↓
Associated Token Account (ATA)
Code Walkthrough: Transferring Any Token on Solana
Now let's walk through our function for transferring tokens on Solana, explaining each part:
export async function sendSplToken() {
const wallet = // Create wallet ;
const mint = new PublicKey("");
const amount = 1;
const toAddress = new PublicKey("");
const connection = new Connection(SOLANA_RPC_ENDPOINT);
const signer = await wallet.getSigner();
const Ixs: TransactionInstruction[] = [];
We start by setting up our wallet, mint (the token we want to transfer), amount, recipient address, and a connection to Solana. The Ixs
array will hold all our transaction instructions.
Step 1: Handle Native SOL vs SPL Token Transfers
if (new PublicKey(mint).equals(SystemProgram.programId)) {
Ixs.push(
SystemProgram.transfer({
fromPubkey: new PublicKey(wallet.address),
toPubkey: new PublicKey(toAddress),
lamports: BigInt(Math.round(amount * LAMPORTS_PER_SOL)),
}),
);
} else {
// SPL token transfer logic...
}
Why Native SOL Doesn't Require ATAs:
Solana makes a distinction between its native token (SOL) and all other tokens (SPL tokens):
Native SOL is built into Solana's core protocol and is stored directly in wallet accounts
SPL tokens are created using the SPL Token program and require separate token accounts
When transferring native SOL, we simply use SystemProgram.transfer
which moves SOL directly between wallet accounts. No ATAs are involved because SOL doesn't use the token program - it's a fundamental part of Solana itself.
Step 2: SPL Token Transfers - Handle Source and Destination ATAs
Now let's analyse the SPL token transfer logic:
} else {
const sourceAccount = getAssociatedTokenAddressSync(
new PublicKey(mint),
new PublicKey(wallet.address),
);
const destinationTokenAccountInfo = await connection.getAccountInfo(
new PublicKey(toAddress),
"finalized",
);
let destinationTokenAccount: PublicKey | null;
try {
const tokenAccount = await getOrCreateAssociatedTokenAccount(
connection,
signer,
new PublicKey(mint),
new PublicKey(toAddress),
);
destinationTokenAccount = tokenAccount.address;
} catch (error) {
destinationTokenAccount = null;
}
We first determine the sender's ATA using
getAssociatedTokenAddressSync
We check if the recipient already has an account for this token
We try to get or create the recipient's ATA
Important point: The getOrCreateAssociatedTokenAccount
function will throw an error if you try to create an ATA that already exists. This is why we catch the error and set destinationTokenAccount
to null if it fails. The next section of code handles this scenario.
Step 3: Create ATA for Recipient If Needed
if (!destinationTokenAccountInfo || !destinationTokenAccount) {
destinationTokenAccount = getAssociatedTokenAddressSync(
new PublicKey(mint),
new PublicKey(toAddress),
);
Ixs.push(
createAssociatedTokenAccountInstruction(
new PublicKey(wallet.address),
destinationTokenAccount,
new PublicKey(toAddress),
new PublicKey(mint),
),
);
}
This block is critical - if the recipient doesn't have an ATA for this token:
We calculate what the recipient's ATA address should be
We create an instruction to create this account
Why ATA Creation Throws Errors:
If you attempt to create an ATA that already exists, the transaction will fail with an error like "account already exists". This is a protection mechanism that prevents duplicate accounts and ensures each wallet has exactly one ATA per token mint.
To avoid this error in production code, we first check if the ATA exists before attempting to create it. Our function handles this by:
First trying to use
getOrCreateAssociatedTokenAccount
If that fails, falling back to a manual creation approach
Only pushing the create instruction if we confirm the ATA doesn't exist
Step 4: Create Transfer Instruction
const numberDecimals = await getNumberDecimals(mint, connection);
Ixs.push(
createTransferInstruction(
sourceAccount,
destinationTokenAccount,
new PublicKey(wallet.address),
Math.round(amount * Math.pow(10, numberDecimals)),
),
);
}
Here we:
Get the decimal precision for the token
Create the actual transfer instruction and add it to our array
Why Instruction Order Matters:
In Solana, instructions within a transaction are executed in the exact order they're added to the transaction. This is crucial when creating an ATA and then transferring tokens to it in the same transaction:
First Instruction: Create the destination ATA
Second Instruction: Transfer tokens to the newly created ATA
If we reversed this order, the transfer would fail because it would try to send tokens to an account that doesn't exist yet. This is why in our code, the ATA creation instruction comes before the transfer instruction.
Step 5: Build and Send the Transaction
const latestBlockHash = await connection.getLatestBlockhash();
const messageV0 = new TransactionMessage({
payerKey: new PublicKey(wallet.address),
recentBlockhash: latestBlockHash.blockhash,
instructions: Ixs,
}).compileToV0Message();
const transaction = new VersionedTransaction(messageV0);
const signedTx = await signer.signTransaction(transaction);
const signature = await connection.sendRawTransaction(signedTx.serialize(), {
skipPreflight: true,
});
console.log("signature", signature);
return signature;
}
Finally, we:
Get a recent blockhash (required for all Solana transactions)
Build a transaction message containing our instructions
Create a versioned transaction (the modern transaction format on Solana)
Sign the transaction with our wallet
Send the transaction to the network and return the signature
Practical Considerations
Token Decimals Matter
Different tokens use different decimal places:
USDC: 6 decimals (1 USDC = 1,000,000 units)
Most SPL tokens: 9 decimals (1 token = 1,000,000,000 units)
Our code handles this by fetching the token's decimals and adjusting the amount:
amount * Math.pow(10, numberDecimals)
Rent and Account Creation
Creating an ATA requires SOL to pay for account "rent" (storage space on the blockchain). In our function, the sender pays this cost when creating the recipient's ATA.
As of early 2025, creating an ATA costs approximately 0.00203928 SOL. Keep this in mind when building applications that might create many ATAs.
Error Handling in Practice
In production code, you should add more robust error handling:
try {
const signature = await sendSplToken();
console.log("Transfer successful:", signature);
} catch (error) {
// Common errors to handle:
// - Insufficient funds (either token balance or SOL for fees)
// - Invalid addresses
// - Account already exists errors
console.error("Transfer failed:", error);
}
Conclusion
This practical guide walked through transferring any token on Solana, highlighting key differences between native SOL and SPL tokens, explaining ATA creation requirements, and demonstrating how to structure your transaction instructions in the correct order.
By understanding these mechanisms, you can now build reliable token transfer functionality into your Solana applications. The token architecture may seem complex at first, but it enables Solana's speed and efficiency while providing a predictable system for managing token ownership.
Finished Work : )
You can find the complete working solution in this GitHub gist here:
https://gist.github.com/Gift-Stack/ca900bc338d636ff5e687d76f59a77cb#file-send-spl-token-ts
Next Steps
To extend this functionality, consider:
Adding better error handling
Supporting batch transfers
Implementing approval flows (delegate authority)
Supporting Token2022 tokens with their extended features
Subscribe to my newsletter
Read articles from Gift Opia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
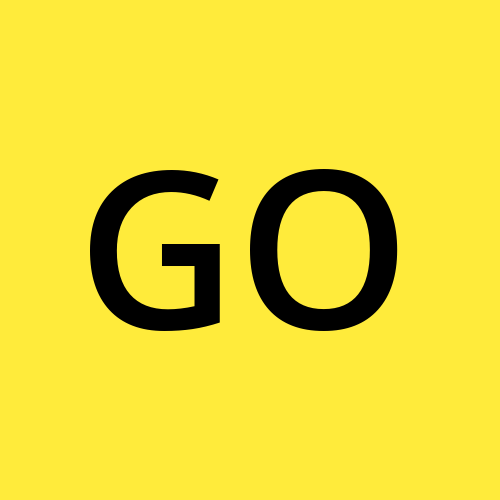