Understanding JavaScript Closures
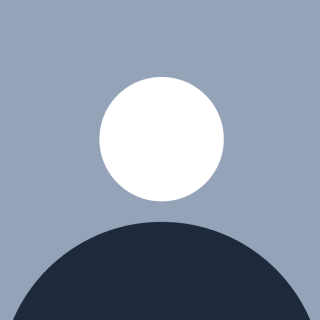
JavaScript closures can be tricky at first, but once you understand them, they become a powerful tool in your coding arsenal.
What is a Closure?
A closure is a function that remembers the variables from its outer scope even after the outer function has finished executing.
Why Are Closures Useful?
Closures help in:
✅ Data privacy (e.g., creating private variables)
✅ Maintaining state between function calls
✅ Callback functions and event handling
Example of a Closure
function outerFunction(outerVariable) { return function innerFunction(innerVariable) { console.log(Outer: ${outerVariable}, Inner: ${innerVariable}); }; }
const newFunction = outerFunction("Hello"); newFunction("World"); // Output: Outer: Hello, Inner: World
Here, innerFunction still has access to outerVariable, even though outerFunction has already executed.
Real-World Use Case: Data Privacy
function createCounter() { let count = 0; // Private variable return function () { count++; console.log(count); }; }
const counter = createCounter(); counter(); // 1 counter(); // 2 counter(); // 3
Since count is inside the closure, it can’t be directly modified from outside, ensuring data encapsulation.
Final Thoughts
Closures are a key part of JavaScript, especially in functional programming. Mastering them will make your code more efficient and modular.
Subscribe to my newsletter
Read articles from Enofua Etue Divine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Enofua Etue Divine
Enofua Etue Divine
I'm a tech enthusiast . I'm a web developer and a student at Altschool Africa currently learning Frontend engineering ...