How to Integrate OpenAI API In A ChatBot Project
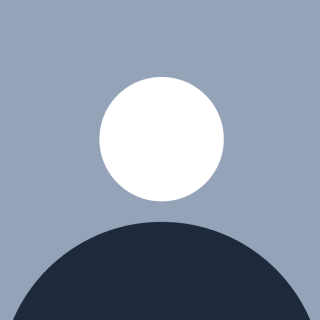
Integrating the OpenAI API into your React project can be done efficiently by following a structured approach. This guide will walk you through the setup process while adhering to the SOLID principles for maintainability and scalability. Additionally, we will explore using both Create React App (CRA) and Vite to bootstrap your project.
Setting Up Your OpenAI API Key
To begin, visit the OpenAI Platform. If you do not have an account, click on the Sign Up button to create one. If you already have an account, simply log in.
Once you’re logged in:
Click on the Start Building button.
Navigate to the API Keys section under your profile settings.
Generate a new API key and store it securely. You will need this key in your application.
Set Up Your React Project
You can initialize a React project using either Create React App (CRA) or Vite. While CRA is widely used, Vite is recommended for better performance and faster builds.
Using Create React App:
npx create-react-app openai-integration
cd openai-integration
Using Vite (Recommended for Performance):
npm create vite@latest openai-integration --template react
cd openai-integration
npm install
Install Dependencies
To facilitate API requests and environment variable management, install the following packages:
npm install axios dotenv
Axios: Handles HTTP requests.
Dotenv: Manages environment variables securely.
Secure Your API Key
To protect your API key, create a .env
file in your project root and add:
REACT_APP_OPENAI_API_KEY=your-api-key-here
Important: Restart your development server after updating environment variables to ensure changes take effect.
Implement API Communication (Single Responsibility Principle)
To maintain clean architecture, API logic should be handled separately from UI components. Following the Single Responsibility Principle (SRP), create a dedicated service module.
Create openaiService.js
Inside src/utils/
import axios from 'axios';
const API_KEY = process.env.REACT_APP_OPENAI_API_KEY;
const API_URL = 'https://api.openai.com/v1/chat/completions';
export const fetchOpenAIResponse = async (prompt) => {
try {
const response = await axios.post(
API_URL,
{
model: 'gpt-3.5-turbo',
messages: [
{ role: 'system', content: 'You are a helpful assistant.' },
{ role: 'user', content: prompt },
],
},
{
headers: {
'Content-Type': 'application/json',
Authorization: `Bearer ${API_KEY}`,
},
}
);
return response.data.choices[0].message.content;
} catch (error) {
console.error('OpenAI API Error:', error);
return 'An error occurred while fetching the response.';
}
};
Why?
- SRP: This ensures that API logic is decoupled from UI components, making the codebase more maintainable and scalable.
Connect the API to Your React Component (Dependency Inversion Principle)
Next, update App.js
to use the API function.
import React, { useState } from 'react';
import { fetchOpenAIResponse } from './utils/openaiService';
const App = () => {
const [prompt, setPrompt] = useState('');
const [response, setResponse] = useState('');
const handleSubmit = async (e) => {
e.preventDefault();
const aiResponse = await fetchOpenAIResponse(prompt);
setResponse(aiResponse);
};
return (
<div style={{ padding: '20px' }}>
<h1>OpenAI Integration</h1>
<form onSubmit={handleSubmit}>
<textarea
rows="4"
cols="50"
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
placeholder="Type your prompt here..."
></textarea>
<br />
<button type="submit">Generate Response</button>
</form>
<h2>Response:</h2>
<p>{response}</p>
</div>
);
};
export default App;
Why?
- Dependency Inversion Principle: By injecting
fetchOpenAIResponse
as an external function, the component remains loosely coupled and easy to test.
Run Your React App
To start the development server, run:
npm start # For Create React App
npm run dev # For Vite
Then, open http://localhost:3000 in your browser.
Test the Integration
Enter a prompt in the text area.
Click Generate Response.
The OpenAI-generated response should appear below.
Key Considerations
OpenAI enforces request limits. Regularly monitor usage in your OpenAI dashboard to prevent unexpected issues.
Never expose your API key in frontend code.
Consider using a server-side proxy for added security in production.
Error Handling
Implement proper error messages for API failures.
Add retry mechanisms for better resilience.
Conclusion
Structuring your integration around SOLID principles, helps you create a maintainable and scalable React application. Choosing Vite over CRA enhances performance, while separating concerns ensures cleaner and more efficient code. This approach allows for better debugging, security, and future scalability, making your application robust and production-ready.
Resources
OpenAI API Documentation – https://platform.openai.com/docs/
- Provides detailed guidance on using OpenAI’s API, authentication, and best practices.
Vite Documentation – https://vitejs.dev/
- Explains why Vite is faster than Create React App and how to use it efficiently.
Axios Documentation – https://axios-http.com/
- Guides on making API calls in React with Axios.
SOLID Principles & Best Practices
SOLID Principles in JavaScript & React – https://www.freecodecamp.org/news/solid-principles-for-react-developers/
- Explains how to apply SOLID principles in React applications.
React Architecture Best Practices – https://kentcdodds.com/blog/colocation
- Covers best practices for structuring React applications.
Security & API Key Management
.env File Best Practices in React – https://create-react-app.dev/docs/adding-custom-environment-variables/
- Official guide on handling environment variables securely.
Why You Should Never Expose API Keys in Frontend – https://blog.bitsrc.io/hiding-api-keys-in-front-end-applications-4ffb36f43b32
Performance & Optimization
Why Vite is Better than Create React App – https://blog.logrocket.com/why-vite-better-create-react-app/
- A comparison of Vite and CRA performance benefits.
Optimizing API Calls in React – https://blog.openreplay.com/how-to-optimize-api-calls-in-react/
Subscribe to my newsletter
Read articles from Olusanya Agbesanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Olusanya Agbesanya
Olusanya Agbesanya
Hey, I'm Toye, a frontend developer and technical writer passionate about building scalable web apps and crafting clear, developer-friendly documentation. I write about React, APIs, and modern web development, making complex topics easy to grasp.