Comprehensive Guide on Using the Perplexity API
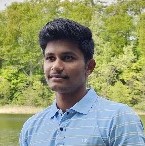
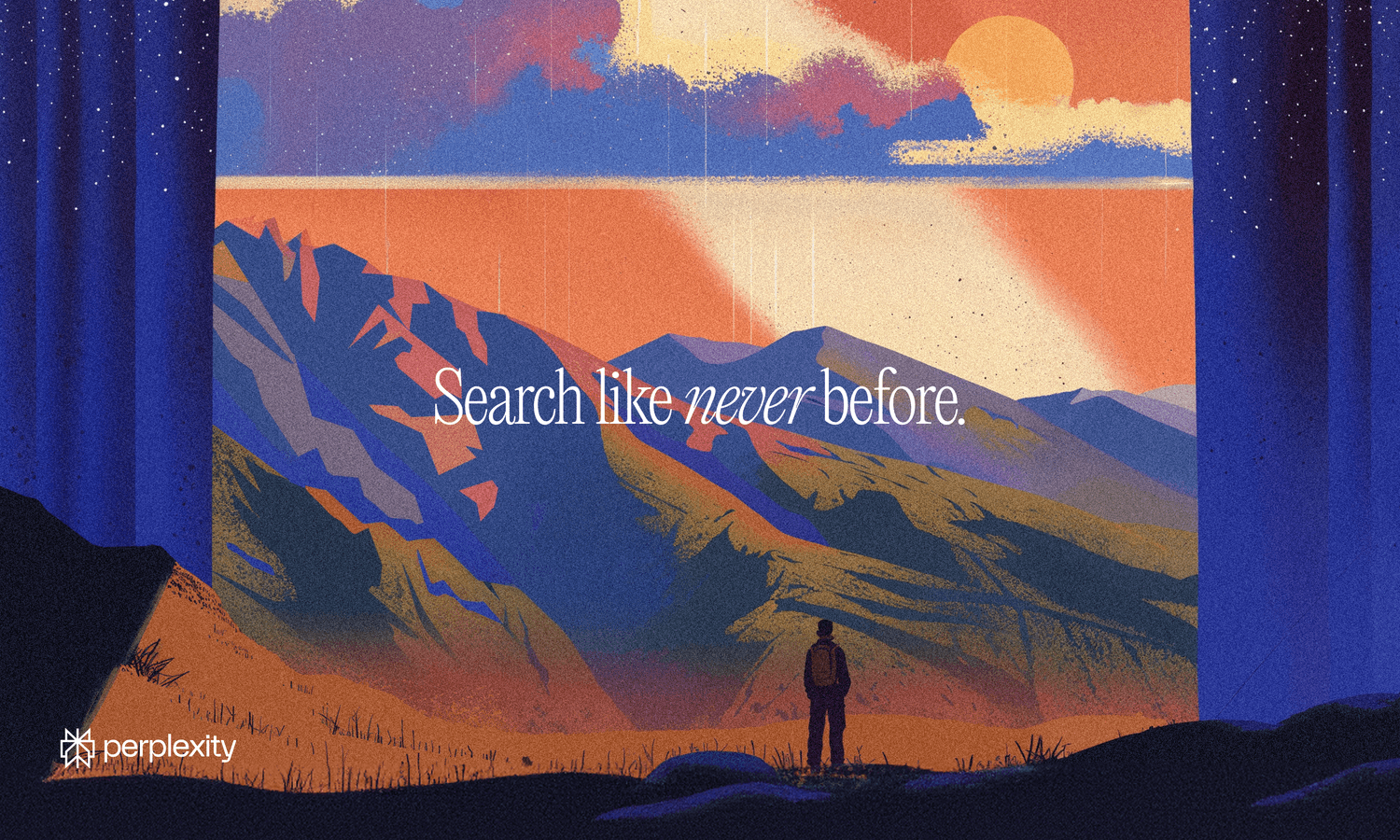
Introduction
Perplexity API, developed by Perplexity, a leading AI search engine, offers developers a powerful tool to integrate AI-powered search and conversational capabilities into their applications. It is designed to provide real-time, accurate responses to user queries, making it particularly suitable for applications requiring up-to-date information, such as news aggregators, research tools, and customer support systems. This guide will walk you through the process of using the Perplexity API, from setup to advanced usage, ensuring you can leverage its full potential.
An unexpected aspect of Perplexity API is its focus on real-time information processing, which enhances its utility for dynamic, time-sensitive applications, setting it apart from traditional AI models that may not access current web data.
Getting Started
To use the Perplexity API, follow these steps:
Sign Up and Register Credit Card:
Visit Perplexity's website and navigate to the API settings page, typically found under account settings or a dedicated API section. For instance, you can access it via Perplexity API Settings.
Register your credit card. This step does not charge your card immediately; it stores payment information for future API usage, ensuring a seamless billing process.
Purchase Credits:
Once your payment information is registered, you can purchase credits to use the API. The credit system is essential, as API keys are only generated with a nonzero balance.
Pro users receive $5 of free credits each month. If you're a new Pro subscriber, allow up to 10 minutes for these credits to appear in your account, facilitating initial testing without additional cost.
Generate API Key:
With a nonzero balance, you can generate an API key. This key is a long-lived access token, used until manually refreshed or deleted, providing flexibility for long-term projects.
Securely manage the API key using environment variables (e.g., export PERPLEXITY_API_KEY='your_api_key_here') or a .env file with python-dotenv, ensuring security and ease of integration.
Make API Requests:
- Use the API key in the Authorization header of your HTTPS requests to the Perplexity API, ensuring authenticated access to its services.
The pricing and credit system is crucial for managing costs. Perplexity operates on a credit-based model, where each API call consumes credits based on the model used and request complexity. Pro users benefit from monthly credits, but for detailed pricing, refer to Perplexity's official documentation, as rates may vary.
Basic Usage
Perplexity API is compatible with the OpenAI API format, specifically the chat completions endpoint, making it accessible for developers familiar with OpenAI. This compatibility simplifies integration, allowing you to use existing libraries and workflows.
Example Using Python and OpenAI Library
To illustrate, here's how to make a basic chat completion request:
First, install the OpenAI library:
pip install openai
Then, set up your API key and base URL:
import openai openai.base_url = "https://api.perplexity.ai" openai.key = "your_api_key_here"
Now, make a chat completion request:
messages = [
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "What is the capital of France?"}
]
response = openai.ChatCompletion.create(
model="sonar-pro",
messages=messages
)
print(response['choices'][0]['message']['content'])nse['choices'][0]['message']['content'])
This example demonstrates a simple query, returning the response, such as "The capital of France is Paris."
Advanced Features
Perplexity API offers several advanced features to enhance functionality:
Streaming Responses:
The API supports streaming responses, allowing you to receive the response in real-time as it's generated. This is particularly useful for applications where you want to display the response incrementally, improving user experience.
Example:
response = openai.ChatCompletion.create(
model="sonar-pro",
messages=messages,
stream=True
)
for chunk in response:
print(chunk['choices'][0]['delta'].get('content', ''))
- This code prints each chunk as it arrives, suitable for real-time chat interfaces.
Different Models:
Perplexity offers various models with varying capabilities, such as "sonar-pro" and "llama-3.1-sonar-small-128k-online," each optimized for different use cases. For instance, "sonar-pro" is noted for its conversational abilities, while online models like "llama-3.1-sonar-small-128k-online" excel in real-time web data access.
Developers should check the official documentation for the full list of available models and their specifications, ensuring the right choice for their application.
Other Parameters:
You can specify additional parameters such as temperature (e.g., 0.2 for more focused responses) and max_tokens (e.g., 1000 for longer answers), similar to OpenAI's API. These parameters allow fine-tuning of the response generation.
Refer to Perplexity's documentation for the complete list of supported parameters, as they may include options for sources, files, and language settings, enhancing query customization.
Error Handling
When using the Perplexity API, it's important to handle potential errors gracefully to ensure robust applications. Common errors include:
Authentication Errors: Occur if the API key is invalid or missing, typically returning a 401 status code.
Insufficient Credits: If you've run out of credits, requests will be blocked, returning a 402 status code, prompting you to add more credits.
Rate Limit Exceeded: If you've made too many requests in a short period, you'll receive a 429 status code, requiring you to wait or optimize request frequency.
To handle these, check the response status code and error messages:
try:
response = openai.ChatCompletion.create(
model="sonar-pro",
messages=messages
)
except openai.error.AuthenticationError as e:
print("Authentication error:", e)
except openai.error.APIError as e:
print("API error:", e)
This approach ensures your application can gracefully handle errors, maintaining user experience.
Best Practices
To maximize the effectiveness of Perplexity API usage, consider the following:
Manage Credits Wisely: Keep track of your credit usage to avoid unexpected charges or downtime. Configure "Automatic Top Up" to refresh your balance when it drops below $2, ensuring continuous service.
Optimize Requests: Minimize the number of API calls by batching queries where possible and optimizing your prompts to get comprehensive responses in fewer requests, saving credits.
Use Streaming for Large Responses: For long responses, use streaming to manage memory efficiently and improve user experience, especially in real-time applications.
Stay Updated: Regularly check Perplexity's documentation (https://docs.perplexity.ai) and blog for updates on new features, model improvements, and any changes to the API, ensuring your integration remains current.
Conclusion
Perplexity API offers a robust solution for developers looking to integrate advanced AI search and conversational capabilities into their applications. By following this guide, you can get started with the API, make basic and advanced requests, handle errors, and follow best practices to ensure a smooth integration. Its focus on real-time information makes it particularly valuable for dynamic, time-sensitive applications, opening up new possibilities for innovation.
For further exploration, dive into Perplexity's official documentation for in-depth details and stay tuned for updates to leverage the latest enhancements.
References
Subscribe to my newsletter
Read articles from Neel Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
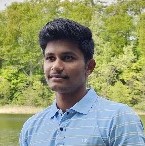