Building a Serverless Cybersecurity x Star Wars API (and Connecting It to My Cloud Resume Website)
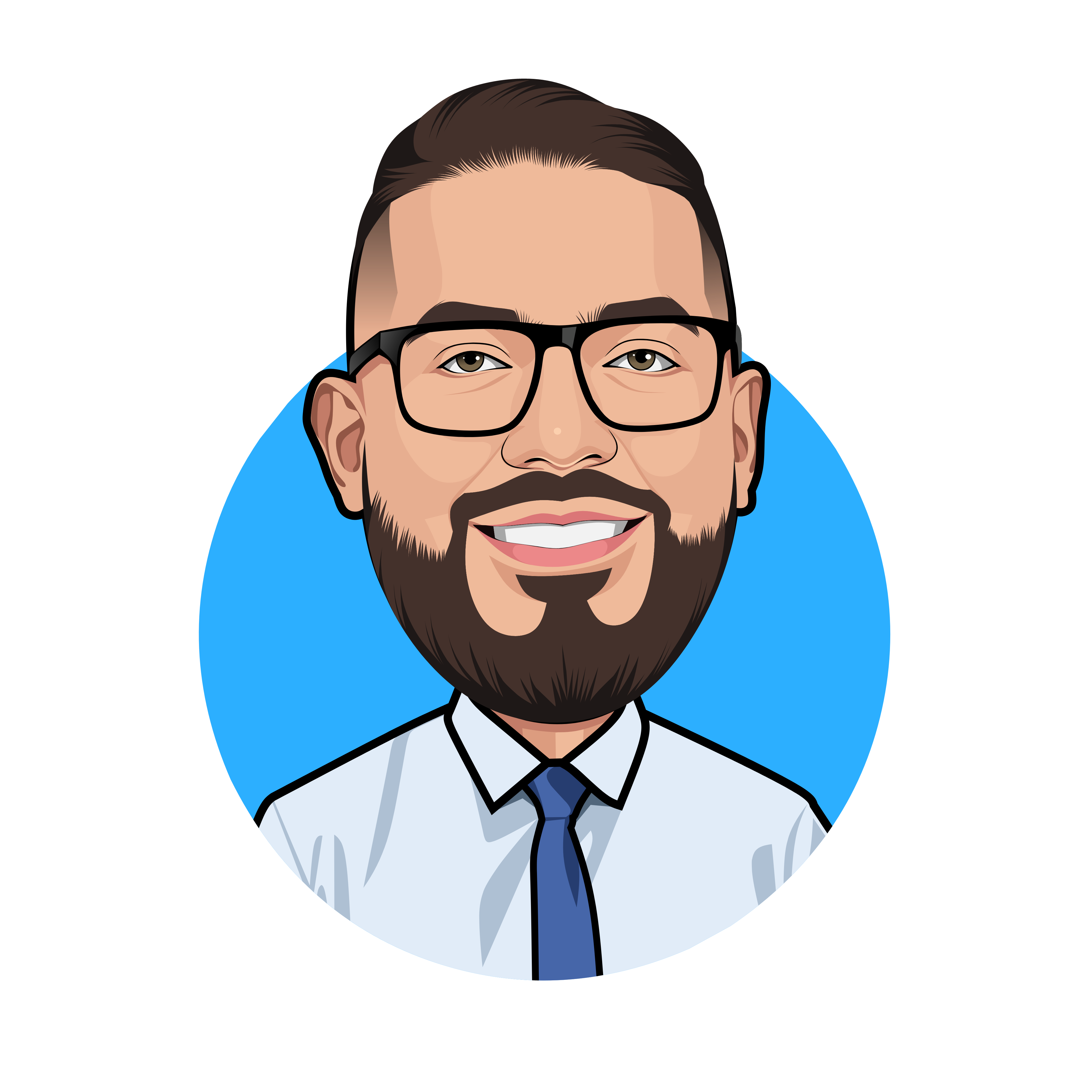
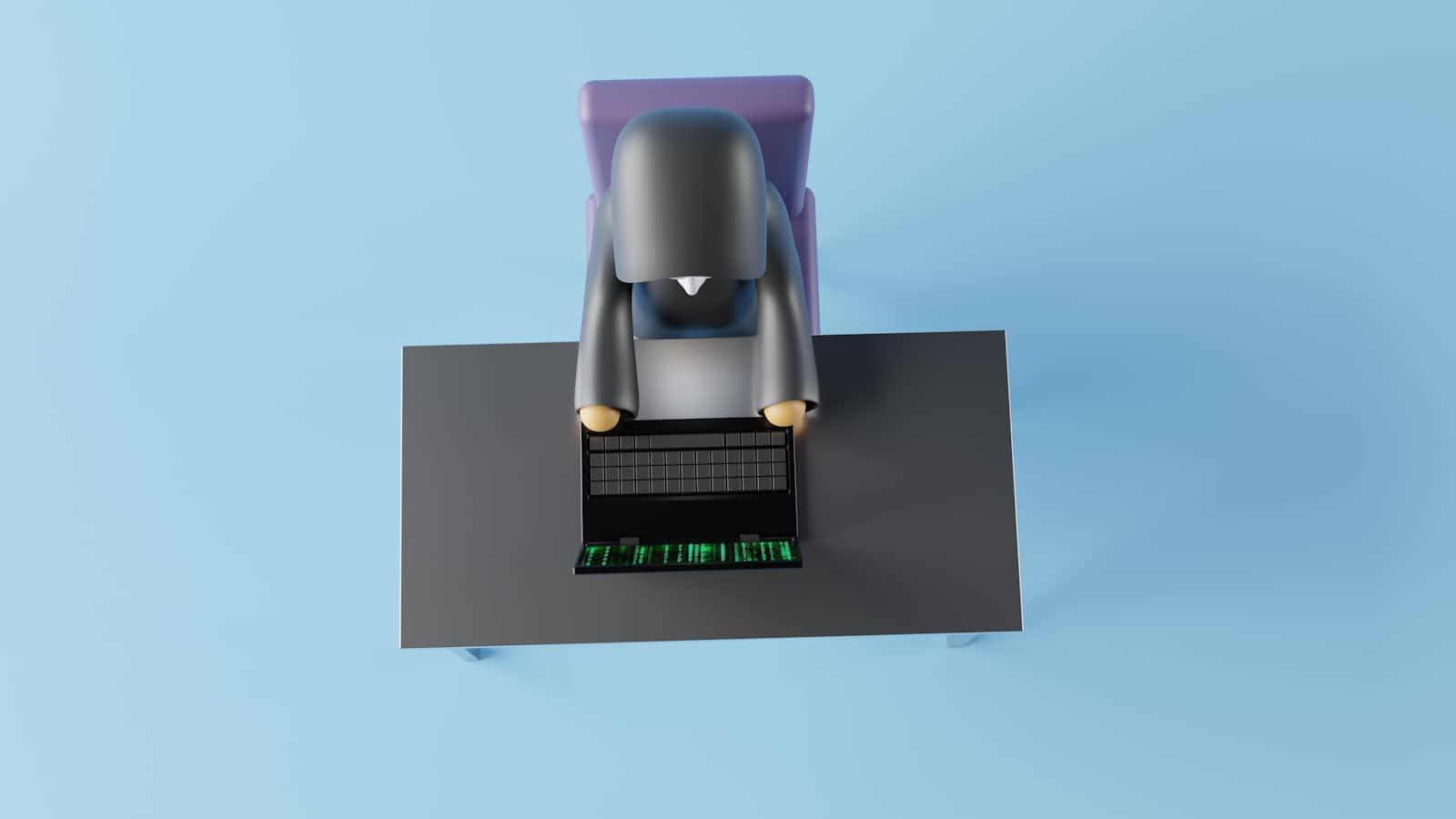
Author: MrCyberLeon
Project Repo: GitHub – AWS Cloud Challenge
Intro
They say a Jedi’s journey begins with a single step — in my case, it was a button click that should’ve returned a random Star Wars fact on my website. What followed was a full-stack, CI/CD, serverless adventure across AWS Lambda, API Gateway, GitHub Actions, CORS headaches, IAM trench runs, and finally... victory.
Here’s how I built, secured, and integrated a Star Wars API into my Cloud Resume Challenge site, and the lessons learned along the way.
Why Build an API?
As part of continuing to level-up my DevSecOps and Cloud skills, I wanted to go beyond static sites and build something interactive. And what better way to do that than with something fun?
Goal:
Create a serverless API that returns a random Star Wars x Cybersecurity fact!
Integrate it into my Cloud Resume Challenge site at cloud.mrcyberleon.org
Architecture Overview
Here’s what I ended up building:
User → cloud.mrcyberleon.org (S3 + CloudFront) → JavaScript fetch()
→ API Gateway → Lambda (Node.js) → Response with random Star Wars fact
Stack:
- AWS Lambda (Node.js 22.x)
- API Gateway (HTTP endpoint)
- GitHub Actions for CI/CD
- S3 + CloudFront for hosting
- Serverless Framework for deployment
- IAM for secure access
- CORS... for chaos (temporarily)
Step-by-Step: Building the API
1. Project Setup (Locally in VS Code)
mkdir starwars-api && cd starwars-api
npm init -y
npm install serverless
Created:
handler.js
— main Lambda functionstarwarsFacts.js
— array of fun factsserverless.yml
— Serverless Framework config
2. API Logic (handler.js)
const facts = require("./starwarsFacts");
module.exports.starwarsfact = async (event) => {
const randomFact = facts[Math.floor(Math.random() * facts.length)];
return {
statusCode: 200,
headers: {
"Content-Type": "application/json",
"Access-Control-Allow-Origin": "https://cloud.mrcyberleon.org"
},
body: JSON.stringify({ fact: randomFact })
};
};
3. Serverless Configuration (serverless.yml)
service: starwars-api
frameworkVersion: '4'
provider:
name: aws
runtime: nodejs22.x
region: us-east-1
deploymentBucket:
name: ${env:AWS_S3_BUCKET}
functions:
starwarsfact:
handler: handler.starwarsfact
events:
- http:
path: starwarsfact
method: get
cors:
origin: 'https://cloud.mrcyberleon.org'
CI/CD: GitHub Actions for API Deployments
I added a GitHub Action in .github/workflows/deploy.yml
:
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
SERVERLESS_ACCESS_KEY: ${{ secrets.SERVERLESS_ACCESS_KEY }}
AWS_S3_BUCKET: ${{ secrets.AWS_S3_BUCKET }}
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- uses: actions/setup-node@v3
with:
node-version: 22
- run: npm install
working-directory: backend/starwars-api
- run: npm install -g serverless
- run: serverless deploy
working-directory: backend/starwars-api
I used a Serverless Access Key to authenticate non-interactively in CI.
Common Errors & How I Slayed Them
AWS credentials missing or invalid
Resolved by making sure GitHub Actions used the correct AWS_ACCESS_KEY_ID
and AWS_SECRET_ACCESS_KEY
environment variables.
ssm:PutParameter access denied
Resolved by specifying a custom S3 deployment bucket so Serverless didn’t need SSM at all.
Bonus: I stored the bucket name as a secret for clean code.
502 Bad Gateway
My Lambda function was erroring out because I forgot to return a proper response or the facts file was missing.
Fixed by verifying my starwarsFacts.js
was required correctly and deployed with the function.
CORS Errors
Fixed by explicitly allowing my domain in the cors.origin
setting inside serverless.yml
.
cors:
origin: 'https://cloud.mrcyberleon.org'
Frontend Integration
Inside my static site (assets/index.js
), I added:
async function getStarWarsFact() {
try {
const response = await fetch("https://wrnqhuvrp9.execute-api.us-east-1.amazonaws.com/dev/starwarsfact");
const data = await response.json();
document.getElementById("starwars-fact").innerText = data.fact;
} catch (error) {
console.error("Error fetching Star Wars fact:", error);
document.getElementById("starwars-fact").innerText = "Oops! Couldn't fetch a fact.";
}
}
And in index.html
:
<h2>Need a Star Wars fact?</h2>
<button onclick="getStarWarsFact()">Get Star Wars Fact</button>
<p id="starwars-fact"></p>
Final Result
Live at: https://cloud.mrcyberleon.org
Click the button to get a random Star Wars fact served live via AWS Lambda + API Gateway.
Fully secured, deployed via CI/CD, and integrated with my Cloud Resume Challenge site.
Final Thoughts
This was way more than just a fun button — it became a deep dive into:
- Serverless architecture
- AWS IAM and permissions
- Deployment automation with GitHub Actions
- Error troubleshooting in real-world cloud apps
- Clean, secure infrastructure-as-code
And yes, I now have a Lambda function that tells you Star Wars facts. DevSecOps achievement unlocked.
Next Steps
- Add API key authentication
- Use AWS Secrets Manager instead of GitHub secrets
- Add more endpoints (
/quote
,/character
, etc.) - Log usage to CloudWatch or an analytics dashboard
Thanks for following along my journey. May your APIs be fast, your YAMLs be clean, and your permissions always least-privileged.
— MrCyberLeon
This project is for personal learning purposes only and is not affiliated with, endorsed by, or associated with Lucasfilm Ltd., Disney, or any related entities. All Star Wars-related content and trademarks are the property of their respective owners.
Subscribe to my newsletter
Read articles from Jonathan DeLeon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
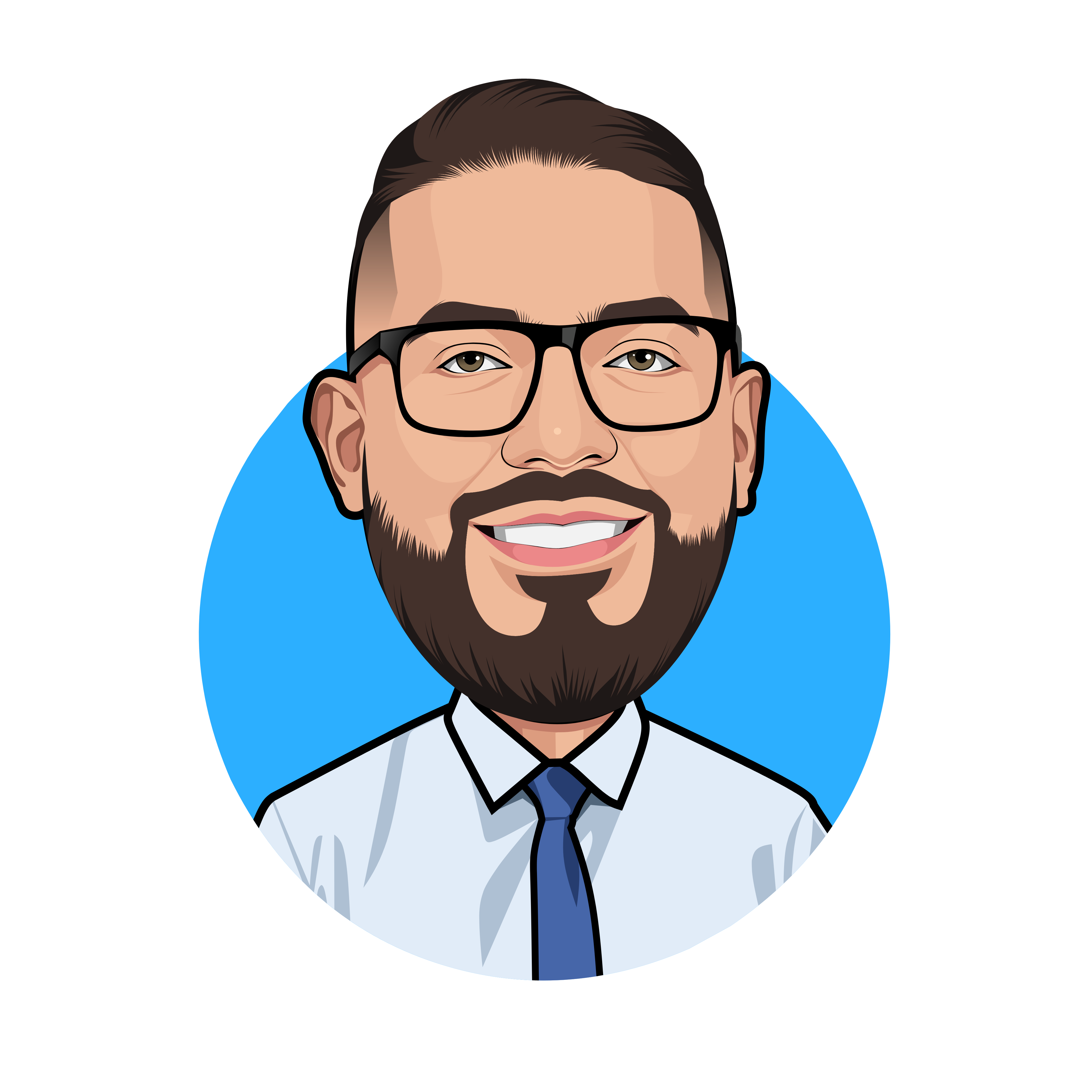
Jonathan DeLeon
Jonathan DeLeon
Hello, my name is Jonathan, and I work as a Cybersecurity Engineer. My expertise includes defending against threats, establishing security controls, and utilizing threat intelligence to gather TTPs (Tactics, Techniques, and Procedures), which I then use to construct custom detections. I am passionate about developing security programs, implementing them, and ensuring that organizations are safeguarded with a comprehensive overview of all resources. I have a particular specialization in Azure Cloud, along with some experience in AWS. My qualifications include the CCSP, CCSK, various Azure Certifications, CompTIA Sec+/Cloud+, among others.