GraphQL API in Node.js
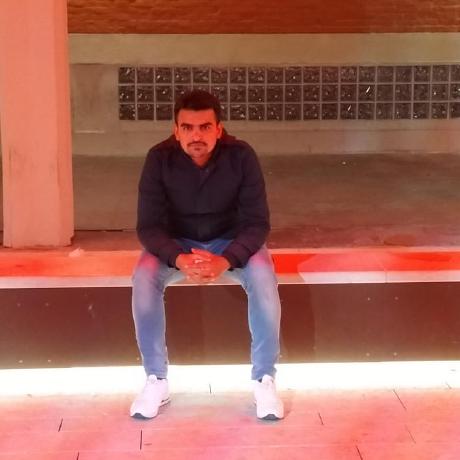
GraphQL, a modern query language for APIs, has become increasingly popular due to its flexibility, efficiency, and powerful features compared to traditional REST APIs. When combined with Node.js, GraphQL allows developers to create fast, scalable, and efficient APIs that are easy to maintain and extend.
Unlike REST APIs, which rely on multiple endpoints for different operations, GraphQL simplifies the process by offering a single endpoint. This single endpoint allows clients to request the exact data they need, in a flexible and structured manner. This gives clients control over the data they retrieve, resulting in more efficient use of bandwidth and reducing the issues of over-fetching or under-fetching data. Furthermore, GraphQL supports real-time updates through subscriptions and can aggregate data from multiple sources seamlessly.
In this article, we will explore how to implement a GraphQL API using Node.js. We will discuss the core concepts of GraphQL, how to set up a GraphQL API, best practices, and the advantages it offers for modern web and mobile applications.
1. Setting Up
To set up a GraphQL API in Node.js, we need a few tools and libraries. The key libraries are:
Express: A minimal and flexible Node.js web application framework.
Apollo Server: A popular library that provides a powerful, production-ready GraphQL server for Node.js.
GraphQL: The core GraphQL specification.
Step-by-Step Setup
Create a New Node.js Project
Start by initializing a new Node.js project:
mkdir graphql-node-api cd graphql-node-api npm init -y
Install Dependencies
Install the required dependencies:
npm install express apollo-server-express graphql
Create the Server
Create a new file
server.js
and set up an Express server with Apollo Server.const express = require('express'); const { ApolloServer, gql } = require('apollo-server-express'); // Define GraphQL schema const typeDefs = gql` type Query { hello: String } `; // Define resolvers const resolvers = { Query: { hello: () => 'Hello, world!', }, }; // Set up ApolloServer const server = new ApolloServer({ typeDefs, resolvers }); const app = express(); server.applyMiddleware({ app }); // Start server app.listen({ port: 4000 }, () => console.log(`Server ready at http://localhost:4000${server.graphqlPath}`) );
Run the Server
Run the server with the following command:
node server.js
Your GraphQL API is now accessible at
http://localhost:4000/graphql
.
2. GraphQL Schema
The schema defines the types of data that can be queried and mutated in your GraphQL API. It serves as a contract between the client and server. The GraphQL schema is defined using the GraphQL Schema Definition Language (SDL).
In the example above, we defined a simple schema with one query, hello
, that returns a string.
Example
type Query {
hello: String
user(id: ID!): User
}
type User {
id: ID
name: String
email: String
}
Query: This type defines the read operations available in your API.
User: A type representing a user in the system, with fields for
id
,name
, andemail
.ID!: The exclamation mark indicates that the
id
field is non-nullable.
3. Resolvers
Resolvers are functions that handle the logic for fetching or modifying data when a query or mutation is made. They map the fields in the schema to corresponding business logic or data retrieval.
Example Resolver
const resolvers = {
Query: {
hello: () => 'Hello, world!',
user: async (parent, args, context, info) => {
const user = await getUserById(args.id); // Assume getUserById fetches user from a database
return user;
},
},
};
Resolvers take four parameters:
parent
: The result of the previous resolver in the chain (for nested queries).args
: The arguments passed to the query or mutation.context
: Shared state like authentication or database connections.info
: Information about the query (useful for advanced use cases like field resolution).
4. Error Handling and Validation
Error handling and validation are crucial for building robust APIs. GraphQL allows you to return detailed error messages to the client, which can be used to inform users about issues such as invalid queries or missing data.
For validation, you can use libraries like Joi or yup. Here's an example of how to validate inputs:
const { gql } = require('apollo-server-express');
const Joi = require('joi');
const userSchema = Joi.object({
name: Joi.string().required(),
email: Joi.string().email().required(),
});
const resolvers = {
Mutation: {
createUser: async (parent, { name, email }) => {
const { error } = userSchema.validate({ name, email });
if (error) throw new Error('Invalid input data');
return await User.create({ name, email });
},
},
};
5. Testing and Optimizing the GraphQL API
Testing GraphQL APIs can be done using libraries like Jest and Apollo Server Testing. For optimizing performance, consider using batching and caching mechanisms such as DataLoader and Apollo Server's built-in caching.
Example of DataLoader
const DataLoader = require('dataloader');
const userLoader = new DataLoader(async (keys) => {
return await User.find({ _id: { $in: keys } });
});
const resolvers = {
Query: {
users: async (parent, { ids }) => {
return await userLoader.loadMany(ids);
},
},
};
Conclusion
GraphQL with Node.js provides a powerful and efficient way to build modern APIs. By following best practices such as schema design, validation, error handling, and authentication, you can create secure and maintainable APIs. The advantages of GraphQL, including flexible data retrieval, a single endpoint, and real-time capabilities, make it an attractive choice for web and mobile applications.
For further learning and official documentation, refer to these resources:
GraphQL Official Documentation: https://graphql.org/learn/
Apollo Server Documentation: https://www.apollographql.com/docs/apollo-server/
Node.js Documentation: https://nodejs.org/en/docs/
By exploring these resources, you can deepen your understanding and apply best practices to your GraphQL projects.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
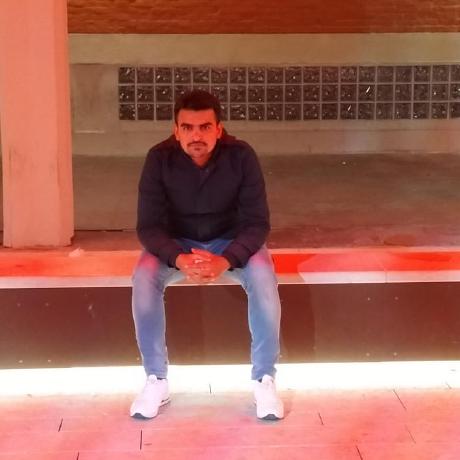
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.