An Introduction to Data Structures and Algorithms

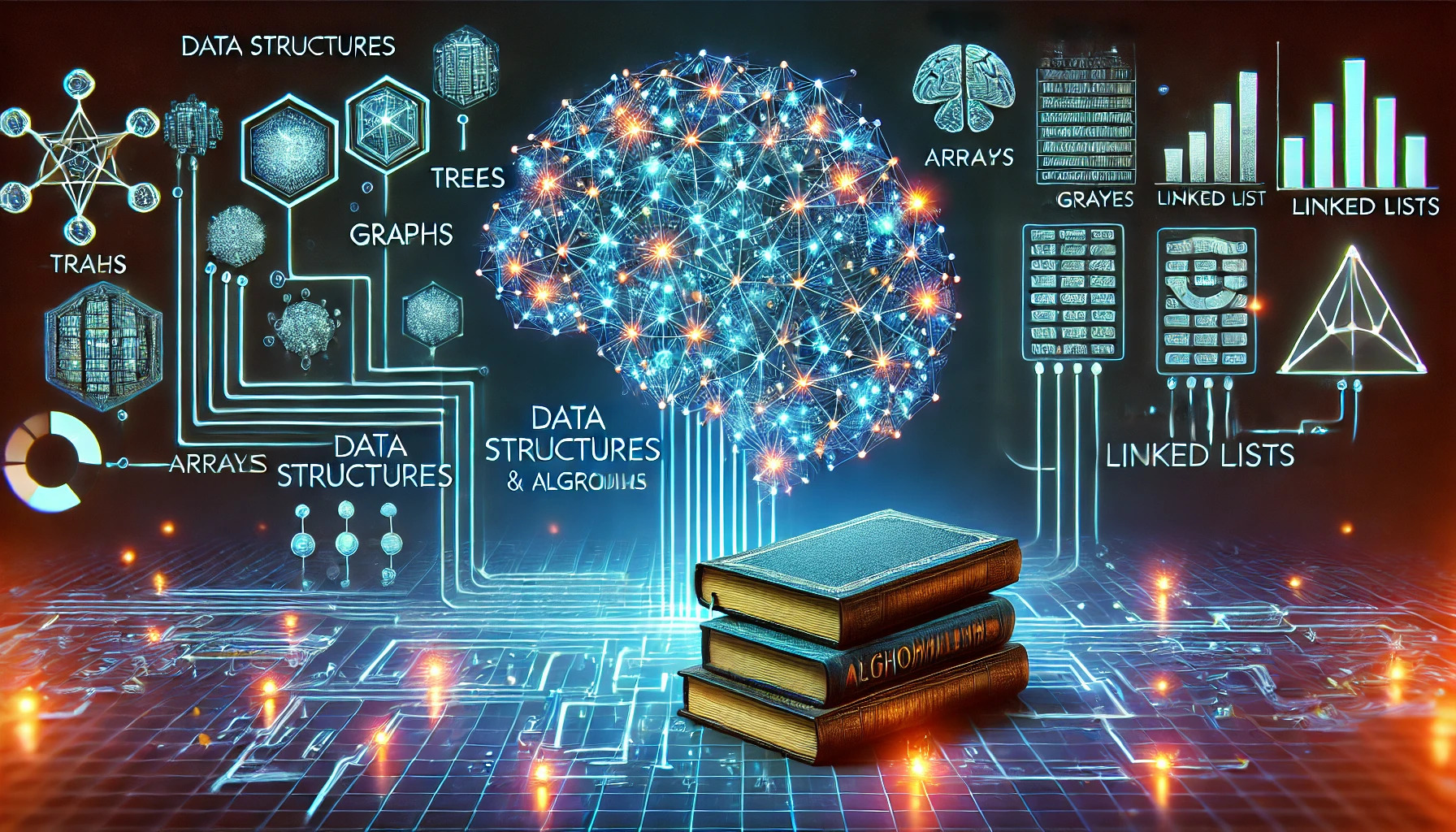
An Introduction to Data Structures and Algorithms
Data Structures and Algorithms (DSA) form the backbone of computer science and software development. Whether you're a beginner or an experienced programmer, mastering DSA is crucial for writing efficient, scalable, and optimized code. In this article, we'll explore the fundamentals of data structures and algorithms, their importance, and how they are applied in real-world scenarios.
If you're looking to make money online using your programming skills, consider joining MillionFormula—a free platform that helps you monetize your expertise without requiring credit or debit cards.
Why Learn Data Structures and Algorithms?
Data structures are ways to organize and store data, while algorithms are step-by-step procedures for performing computations. Together, they help solve complex problems efficiently. Here’s why they matter:
Optimized Performance – Efficient DSA reduces time and space complexity, making programs faster and more resource-friendly.
Problem-Solving Skills – They improve logical thinking and help break down problems into manageable steps.
Interview Preparation – Tech companies like Google, Amazon, and Microsoft heavily test DSA knowledge in coding interviews.
Foundation for Advanced Topics – Machine learning, blockchain, and AI rely on efficient algorithms.
Common Data Structures
1. Arrays
An array is a collection of items stored at contiguous memory locations. python Copy
# Example of an array in Python
numbers = [1, 2, 3, 4, 5]
print(numbers[0]) # Output: 1
Arrays are great for quick access but inefficient for insertions/deletions.
2. Linked Lists
A linked list consists of nodes where each node contains data and a reference to the next node. python Copy
# Node class for a singly linked list
class Node:
def __init__(self, data):
self.data = data
self.next = None
# Creating a simple linked list
head = Node(1)
second = Node(2)
head.next = second
Linked lists allow dynamic memory allocation but have slower access times than arrays.
3. Stacks and Queues
Stack (LIFO – Last In, First Out) python Copy
stack = [] stack.append(1) # Push stack.pop() # Pop
Queue (FIFO – First In, First Out) python Copy
from collections import deque queue = deque() queue.append(1) # Enqueue queue.popleft() # Dequeue
4. Hash Tables
Hash tables store key-value pairs with average O(1) time complexity for search, insert, and delete operations. python Copy
# Dictionary in Python is a hash table
hash_map = {"name": "Alice", "age": 25}
print(hash_map["name"]) # Output: Alice
5. Trees and Graphs
Binary Tree – A hierarchical structure where each node has at most two children.
Graph – A collection of nodes connected by edges (used in social networks, GPS navigation).
Essential Algorithms
1. Sorting Algorithms
Bubble Sort – Simple but inefficient (O(n²)).
Merge Sort – Divide-and-conquer approach (O(n log n)).
Quick Sort – Efficient for large datasets (O(n log n) average case).
2. Searching Algorithms
Linear Search – Checks each element (O(n)).
Binary Search – Works on sorted arrays (O(log n)).
python
Copy
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Graph Algorithms
Breadth-First Search (BFS) – Explores all neighbor nodes before moving deeper.
Depth-First Search (DFS) – Explores as far as possible before backtracking.
Real-World Applications
Databases – Use B-trees for indexing.
Networking – Dijkstra’s algorithm finds the shortest path in routing.
AI & Machine Learning – Optimization algorithms like Gradient Descent rely on DSA.
How to Master DSA
Practice Coding Problems – Websites like LeetCode, HackerRank, and Codeforces offer great challenges.
Implement Data Structures from Scratch – Reinforces understanding.
Read Books – "Introduction to Algorithms" by Cormen is a classic.
Join Coding Communities – Engage in discussions on Stack Overflow and Hashnode.
Conclusion
Data Structures and Algorithms are essential for any programmer aiming to write optimized and scalable code. By mastering these concepts, you enhance your problem-solving skills and open doors to high-paying tech roles.
If you want to earn money online by leveraging your programming expertise, check out MillionFormula—a free platform that helps you monetize your skills effortlessly.
Keep learning, keep coding! 🚀
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
