Authorization Bypass in Next.js Middleware: How to Secure Your Application

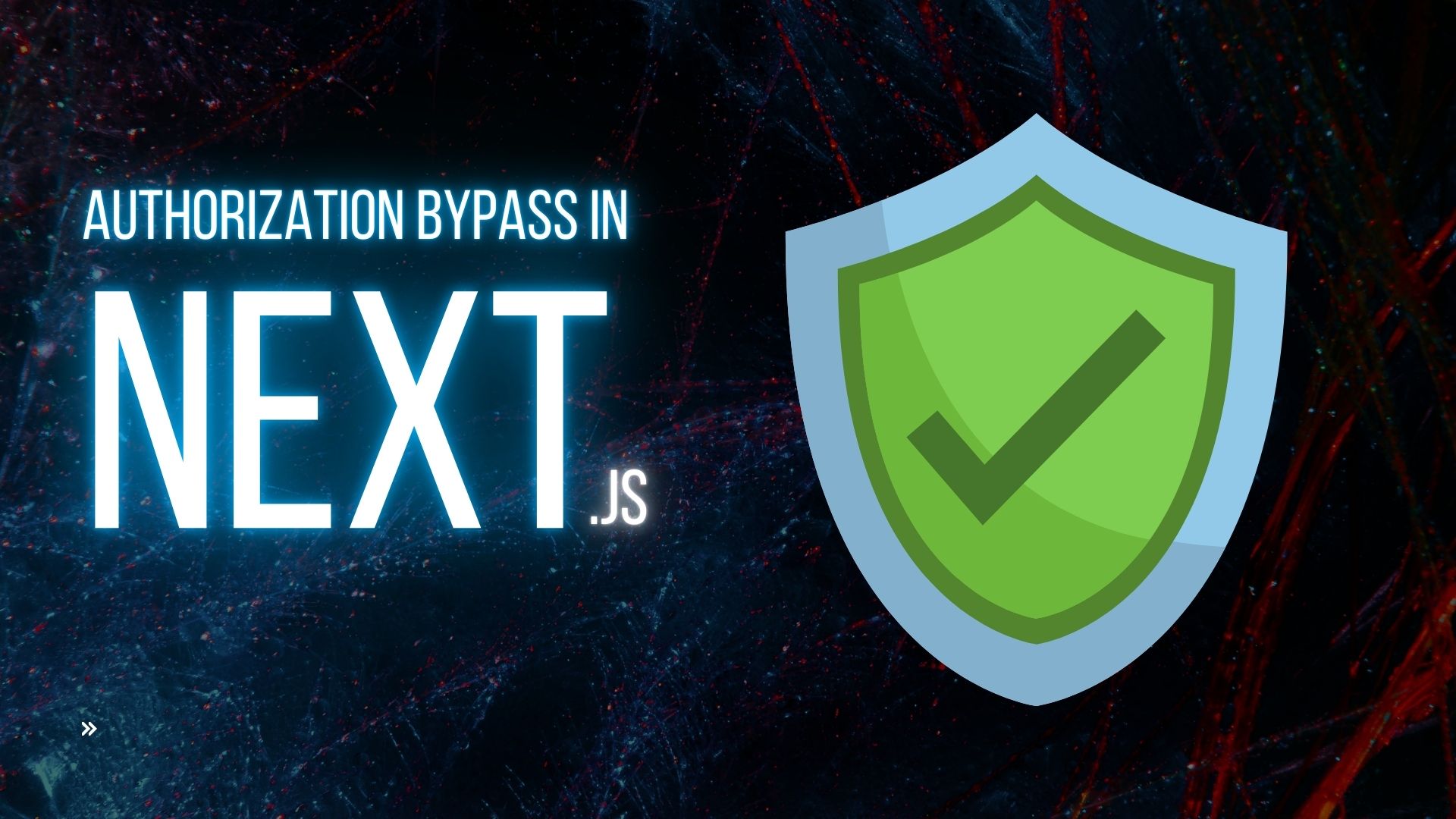
Imagine this: You’ve built a Next.js application with authentication middleware to protect sensitive routes. Everything looks secure—until an attacker finds a way to bypass your middleware and access restricted content without proper authorization.
Yes, authorization bypass vulnerabilities in Next.js Middleware are real, and if your application isn’t properly secured, you could be exposing admin dashboards, user data, and confidential APIs to unauthorized users.
🔹 Are you sure your authentication checks are foolproof?
🔹 Could a small configuration mistake expose your entire application?
🔹 Is your API properly secured, or can someone bypass middleware and access it directly?
💡 In this guide, we’ll uncover how attackers exploit middleware weaknesses and, more importantly, how you can secure your Next.js app against them.
🚀 Let’s dive in and make sure your application is truly secure!
🔍 What is Next.js Middleware, and Why is It Critical for Security?
Next.js Middleware is a function that runs before a request reaches its destination. It allows you to:
✅ Authenticate users before loading protected pages
✅ Redirect users based on roles and permissions
✅ Modify headers for security and performance
✅ Filter out unauthorized access to sensitive routes
💡 But here’s the catch: If not implemented correctly, attackers can bypass middleware, accessing restricted resources without authentication or authorization.
🚀 Supercharge Your Project Management with ByteScrum!
Struggling to keep your projects on track? ByteScrum is the ultimate agile project management tool designed to help teams collaborate efficiently and deliver results faster!
✅ Streamline workflows with powerful Scrum & Kanban boards
✅ Boost team productivity with real-time collaboration
✅ Track progress effortlessly with intuitive dashboards
✅ Stay organized with task management & automation
🚀 Ready to take your projects to the next level?
Try ByteScrum today and experience seamless project management like never before! 🔥
🚨 How Attackers Bypass Authorization in Next.js Middleware
1️⃣ Weak or Missing Authorization Checks
🔴 Vulnerable Code:
export function middleware(req) {
const token = req.cookies.get('authToken'); // Only checking if token exists
if (!token) {
return NextResponse.redirect(new URL('/login', req.url));
}
return NextResponse.next(); // Allows all authenticated users
}
🔴 The Problem: This only checks if a token exists but does NOT verify if the user is authorized to access the route.
✅ Fix: Always verify user roles and permissions before granting access.
import { verifyToken } from './lib/auth';
export async function middleware(req) {
const token = req.cookies.get('authToken');
if (!token) {
return NextResponse.redirect(new URL('/login', req.url));
}
const user = await verifyToken(token);
if (!user || user.role !== 'admin') {
return NextResponse.redirect(new URL('/unauthorized', req.url));
}
return NextResponse.next();
}
✔ Ensures the token is valid
✔ Checks user roles before granting access
✔ Prevents low-privilege users from accessing admin pages
2️⃣ Relying Only on Client-Side Authentication
Client-side authentication (like checking tokens in localStorage) is NOT secure because attackers can manipulate browser storage or modify requests.
🔴 Example of Poor Security:
const user = JSON.parse(localStorage.getItem('user'));
if (user?.role !== 'admin') {
alert('Unauthorized');
router.push('/login');
}
✅ Fix: Always enforce authentication in Next.js Middleware and API routes, NOT just in the frontend.
3️⃣ Exposed API Endpoints Without Middleware Protection
🔴 Issue: Even if middleware protects frontend pages, an attacker can bypass it by calling the API directly.
🔴 Vulnerable API Example:
export default async function handler(req, res) {
res.json({ message: 'Sensitive data' });
}
✅ Fix: Enforce authentication and role-based access control (RBAC) in API routes.
import { verifyToken } from '../../lib/auth';
export default async function handler(req, res) {
const token = req.cookies.authToken;
if (!token) {
return res.status(401).json({ error: 'Unauthorized' });
}
const user = await verifyToken(token);
if (!user || user.role !== 'admin') {
return res.status(403).json({ error: 'Forbidden' });
}
res.json({ message: 'Sensitive data' });
}
✔ Ensures only authenticated users can access the API
✔ Prevents bypassing middleware by calling APIs directly
4️⃣ Middleware Applied to the Wrong Routes
🔴 Issue: If your middleware is not configured correctly, it may not protect all necessary routes, leaving gaps in your security.
🔴 Vulnerable Middleware Configuration:
export const config = {
matcher: ['/dashboard/:path*', '/admin/:path*'], // Only protecting certain routes
};
✅ Fix: Secure everything by default and explicitly allow only public pages.
export const config = {
matcher: ['/((?!login|public|api/public).*)'], // Protects everything except public routes
};
✔ Ensures all pages are protected by default
✔ Prevents accidental exposure of sensitive pages
🔐 Best Practices to Prevent Authorization Bypass
✅ 1️⃣ Always Verify Authentication Tokens Properly
Use server-side token verification, not client-side.
Implement token expiration for better security.
✅ 2️⃣ Enforce Role-Based Access Control (RBAC)
Store user roles in JWTs and verify them in middleware.
Restrict high-privilege routes (e.g., admin dashboards).
✅ 3️⃣ Secure API Routes Separately from Middleware
- Even if middleware protects frontend pages, always validate API requests too.
✅ 4️⃣ Store Authentication Tokens Securely
Use HttpOnly cookies instead of
localStorage
for authentication.Protect against Cross-Site Request Forgery (CSRF) attacks.
✅ 5️⃣ Implement Logging & Monitoring
Log failed authentication attempts for security auditing.
Use rate limiting to prevent brute-force attacks.
✅ 6️⃣ Use HTTPS & Security Headers
Enforce HTTPS to prevent man-in-the-middle (MITM) attacks.
Set strict Content Security Policies (CSPs).
🚀 Boost Your Productivity with UtilsHub!
Looking for a fast, free, and reliable way to handle everyday online tasks? UtilsHub is your ultimate all-in-one utility platform!
✅ Convert, edit, and optimize files effortlessly
✅ Access a wide range of tools – from text manipulation to image editing
✅ Completely free & easy to use – No downloads or sign-ups required!
🔗 Try it now! Click here 👉 UtilsHub and simplify your workflow today! 🚀
🎯 Take Action: Secure Your Next.js Middleware Today!
🚀 Here’s what you should do next:
✅ Review your Next.js middleware code – Are you validating user roles properly?
✅ Check your API endpoints – Can they be accessed directly?
✅ Test your app for vulnerabilities – Can unauthorized users access restricted pages?
✅ Implement best practices – Apply these security fixes today!
🔐 Security is not optional—it’s essential. Take action now to protect your Next.js application from authorization bypass vulnerabilities!
📢 Have questions or need help securing your Next.js app? Drop a comment below! 🚀
Subscribe to my newsletter
Read articles from ajit gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ajit gupta
ajit gupta
Hey! I'm a passionate Full-Stack Developer who loves turning ideas into scalable web apps. I specialize in NestJS, React, and MongoDB, and enjoy working on modern product experiences with clean UI and solid backend architecture. Currently exploring fintech integrations like Razorpay and PayPal. Follow me for practical coding tips, tutorials, and insights from real-world projects. Let’s build something awesome together! 🔥