How to Automate Your Development Workflow with VSCode Tasks: A Complete Guide
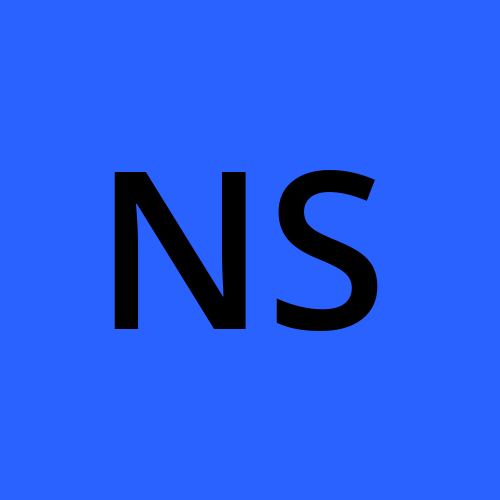
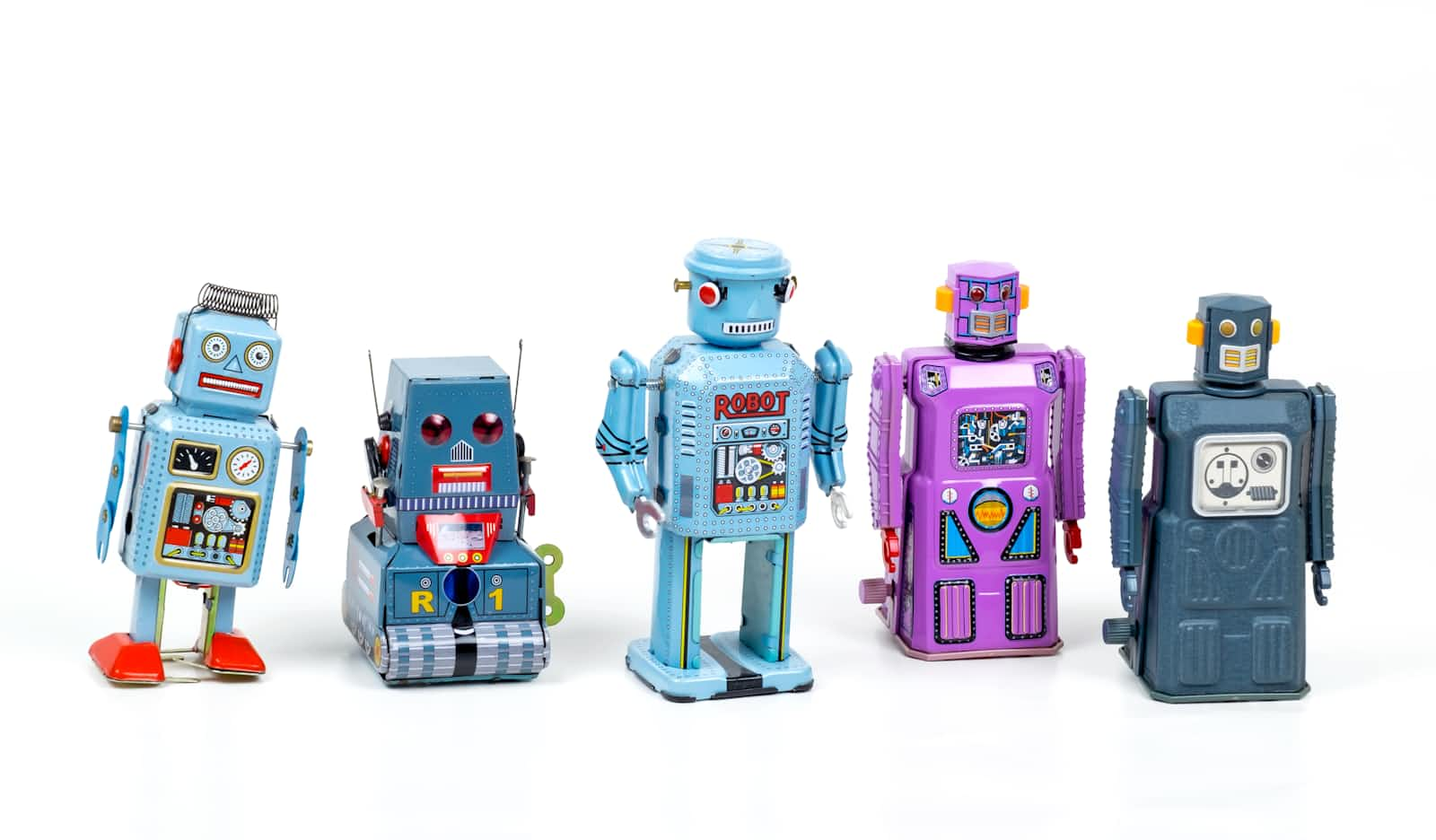
If you're using Visual Studio Code (VSCode), you're probably aware of its versatility. But did you know it can do a lot more than just edit code? One of the features that can drastically improve your productivity is VSCode Tasks. Tasks allow you to automate common processes in your development flow, from running scripts to compiling code and even deploying apps.
In this tutorial, we'll show you how to create your own tasks in VSCode with a hands-on example. Let's dive in!
So, What Exactly Are VSCode Tasks?
VSCode tasks are essentially a way to automate and simplify repetitive development processes directly from your editor. With tasks, you can run everything from scripts and build tools to testing frameworks—all with a single click or shortcut.
Think of tasks as little helpers that take care of all the things you'd normally type into the terminal, saving you time and effort. For example, you can use tasks to:
Run your build commands
Lint your code
Deploy your application
Minify assets
And much more...
These tasks are defined in a file called tasks.json
inside a .vscode
folder in your project directory.
Why Should You Care About VSCode Tasks?
Using tasks can make your workflow much smoother and faster. Here's why:
Save Time: Run commands with a single click or keyboard shortcut.
Stay Consistent: Ensure commands are executed the same way every time.
Automate Repetitive Tasks: No more typing the same commands over and over.
Better Integration: Tasks can easily tie into build tools and testing frameworks.
Customize Your Workflow: Assign custom shortcuts and tweak tasks to suit your needs.
Let’s Create Your First Task Together!
Ready to dive in? Let's start by creating a simple task that runs a basic Node.js script.
Step 1: Open Your Project in VSCode
If you don’t have a project yet, create a quick Node.js app. If you already have one, open it up in VSCode.
Step 2: Set Up Your tasks.json
File
First, go to Terminal > Configure Default Build Task in VSCode. If this is your first time setting up tasks, VSCode will create a
.vscode
folder with atasks.json
file inside.If that doesn’t happen, you can manually create the
tasks.json
file. Right-click on your project folder and create a new file calledtasks.json
inside a.vscode
folder.
Step 3: Write Your First Task
Here’s an example tasks.json
file that runs a script called start.js
using Node.js:
{
"version": "2.0.0",
"tasks": [
{
"label": "Run Node.js Script",
"type": "shell",
"command": "node",
"args": ["start.js"],
"problemMatcher": []
}
]
}
What’s Happening Here?
version: Specifies the task configuration version (we're using
2.0.0
).tasks: An array where you can define multiple tasks.
label: This is the name of the task. It’ll show up in the task runner, and you can give it any name you like.
type: The task type (here, we’re using
shell
to run a command in the terminal).command: The command to run—in this case,
node
, which executes your Node.js script.args: These are the arguments to pass to the command. Here, we’re passing
start.js
.problemMatcher: We’ve left this empty for now, but it’s useful for matching any issues that pop up when running the command.
Step 4: Run the Task
Now let’s run the task:
Open the Command Palette (
Ctrl+Shift+P
on Windows/Linux,Cmd+Shift+P
on macOS).Type in Tasks: Run Task, then select your task Run Node.js Script.
Your script should run in the terminal, and you'll see the output right there!
Step 5: Assign a Keyboard Shortcut (Optional)
If you want to speed things up, you can assign a keyboard shortcut to the task:
Open the Keyboard Shortcuts (
Ctrl+K Ctrl+S
on Windows/Linux,Cmd+K Cmd+S
on macOS).Search for Tasks: Run Task and assign a shortcut like
Ctrl+Shift+R
.
Now you can run your task with a simple keystroke!
Let’s Explore More Advanced Task Examples
While our first example is simple, tasks can handle more complex workflows. Let’s check out some advanced tasks.
Example 1: Running ESLint to Lint Your Code
If you're using ESLint to keep your JavaScript clean, you can create a task like this:
{
"version": "2.0.0",
"tasks": [
{
"label": "Run ESLint",
"type": "shell",
"command": "npx",
"args": ["eslint", "."],
"problemMatcher": ["$eslint-stylish"]
}
]
}
This task will run ESLint on all files in your project, and it uses the eslint-stylish
problem matcher to show linting issues in VSCode’s Problems panel.
Example 2: Building Your Project with Webpack
For projects using Webpack, you can create a task like this to trigger the build process:
{
"version": "2.0.0",
"tasks": [
{
"label": "Build with Webpack",
"type": "shell",
"command": "npx",
"args": ["webpack", "--config", "webpack.config.js"],
"group": "build",
"problemMatcher": []
}
]
}
This task will run Webpack with the webpack.config.js
configuration file.
Example 3: Running Tests Automatically
If you're using Mocha for testing, here’s how to set up a task to run your tests:
{
"version": "2.0.0",
"tasks": [
{
"label": "Run Mocha Tests",
"type": "shell",
"command": "npx",
"args": ["mocha", "test/**/*.js"],
"problemMatcher": []
}
]
}
This will run all the tests in your test
folder.
Wrapping Up: Make Tasks Work for You
VSCode tasks are a great way to automate your development tasks, making your workflow faster, more consistent, and more organized. Whether you're compiling code, running tests, or deploying your app, tasks can save you a lot of time.
Now that you know how to create and configure tasks, try setting up your own custom workflows and integrating them with your favorite tools. You can even assign shortcuts to them to really speed up your work!
Give tasks a try and watch your development process become more streamlined and efficient.
Subscribe to my newsletter
Read articles from Nani Samireddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
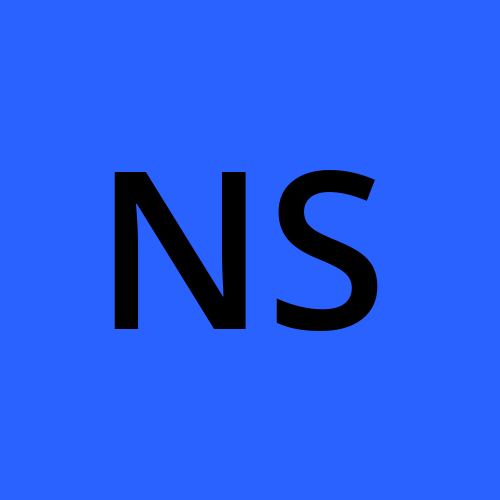