Getting Started with React using Vite
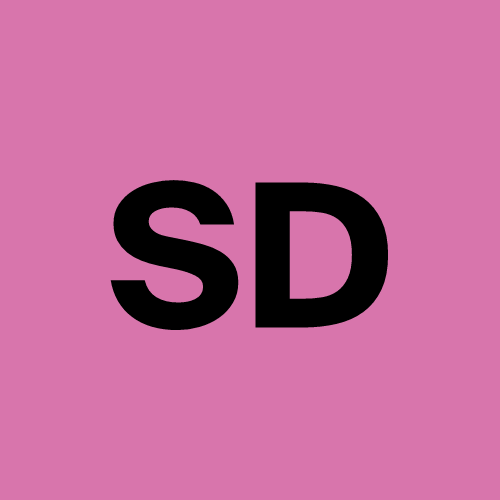
React is one of the most popular JavaScript libraries for building user interfaces, and Vite is a modern build tool that provides an incredibly fast development experience. If you’re looking to get started with React using Vite and JavaScript, you’ve come to the right place! This guide will walk you step by step through the process of initializing a React project with Vite, cleaning up the default code, organizing your app with components and pages, and adding Bootstrap for styling.
Prerequisites
Before we start, ensure that you have the following installed:
Node.js
npm (which comes with Node.js)
Initialize the Project
Create the project: Open your terminal in desired location and run the following command to create a new Vite project. We'll be using the
create-vite
template and choosing React and JavaScript.npm create vite@latest
If asked in install/update
create vite
typey
andenter
.Type your project name (start typing and the demo name will be automatically removed). Do not use any space in the project name.
Use Up/Down arrow key to move the pointer and select
React
from the list of Frameworks.Use Up/Down arrow key to move pointer and select
JavaScript
and the list of option.Open the Project Folder using any code editor.
Install dependencies: Now that we have the basic React setup, run the following command to install the dependencies in the integrated terminal.
npm install
Run the development server: After installing the dependencies, you can run the development server to check that everything is working.
npm run dev
Visit
http://localhost:5173
in your browser, and you should see your React app running.
Clean Up the Default Code
Vite comes with a default template that contains some example code. Let's clean up the code to start fresh.
Open
src/App.jsx
: You’ll find a basicApp.jsx
file with some starter content. For now, remove everything inside the fragments<> </>
tag. Here’s an example of whatApp.jsx
might look like after cleanup:function App() { return ( <div> <h1>Welcome to React!</h1> </div> ); } export default App;
Clean
src/App.css
andsrc/index.css
: You can also clean up the CSS files that come with the template, as we’ll be installing Bootstrap to style the app. You can remove or comment out everything inside these files for now.
Set Up Folder Structure
Create folders for components and pages: Inside the src
directory, create two new folders: components
and pages
.
my-react-app/
│
├── public/
│ └── vite.svg
│
├── src/
│ ├── assets/
│ │ └── react.svg
│ ├── components/ # Add This Folder
│ ├── pages/ # Add This Folder
│ ├── App.jsx
│ ├── index.css
│ ├── main.jsx
│ └── App.css
│
├── .gitignore
├── index.html
├── package.json
├── package-lock.json
└── vite.config.js
Install Bootstrap
Install Bootstrap: You can install Bootstrap by running the following command:
npm install bootstrap
Import Bootstrap CSS and JS: Open the src/App.jsx
file, and import the Bootstrap CSS and JS file at the top:
import 'bootstrap/dist/css/bootstrap.min.css';
import 'bootstrap/dist/js/bootstrap.bundle.min.js';
Create the User List Example
To maintain clarity and consistency in your project, it’s important to follow proper naming conventions for your components:
Component files: Use PascalCase for component file names (e.g.,
UserCard.jsx
,UsersPage.jsx
).Component names: Use PascalCase for component names as well (e.g.,
UserCard
,UsersPage
).
Create a
UserCard
component: Inside thecomponents
folder, create a file namedUserCard.jsx
. This component will display individual user details.src/components/UserCard.jsx
:import React from 'react'; function UserCard({ user }) { return ( <div className="card m-2" style={{ width: '18rem' }}> <div className="card-body"> <h5 className="card-title">{user.name}</h5> <p className="card-text">{user.email}</p> </div> </div> ); } export default UserCard;
Create a
UsersPage
component: In thepages
folder, create a new file namedUsersPage.jsx
. This component will fetch the list of users and display them using theUserCard
component.src/pages/UsersPage.jsx
:import UserCard from '../components/UserCard'; function UsersPage() { const users = [ {name: "John Smith", email: "john@gmail.com"}, {name: "Jane Doe", email: "jane@gmail.com"}, {name: "Bruce Wayne", email: "bruce@gmail.com"}, ] return ( <div className="container mt-5"> <h1>Users</h1> <div className="d-flex"> {users.map((user, index) => ( <UserCard key={index} user={user} /> ))} </div> </div> ); } export default UsersPage;
Update the
App.jsx
: Finally, update theApp.jsx
file to render theUsersPage
component.src/App.jsx
:import React from 'react'; import UsersPage from './pages/UsersPage'; // import this function App() { return ( <div> <UsersPage /> // Add This </div> ); } export default App;
Run the development server again:
npm run dev
Visit
http://localhost:5173
in your browser. You should see a list of user cards displayed on the page!
Happy Coding :)
Subscribe to my newsletter
Read articles from Surajit Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
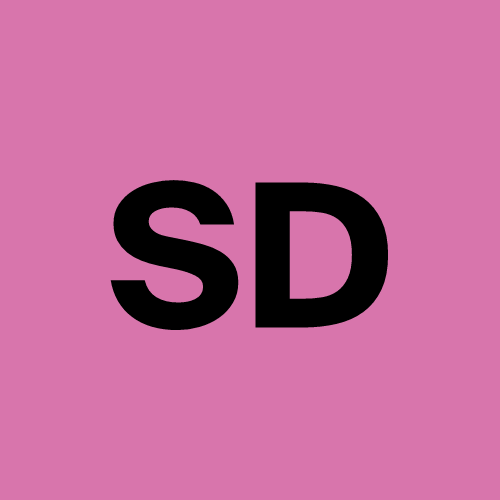