Boost Your Productivity with Multiple AI Agents Using CrewAI

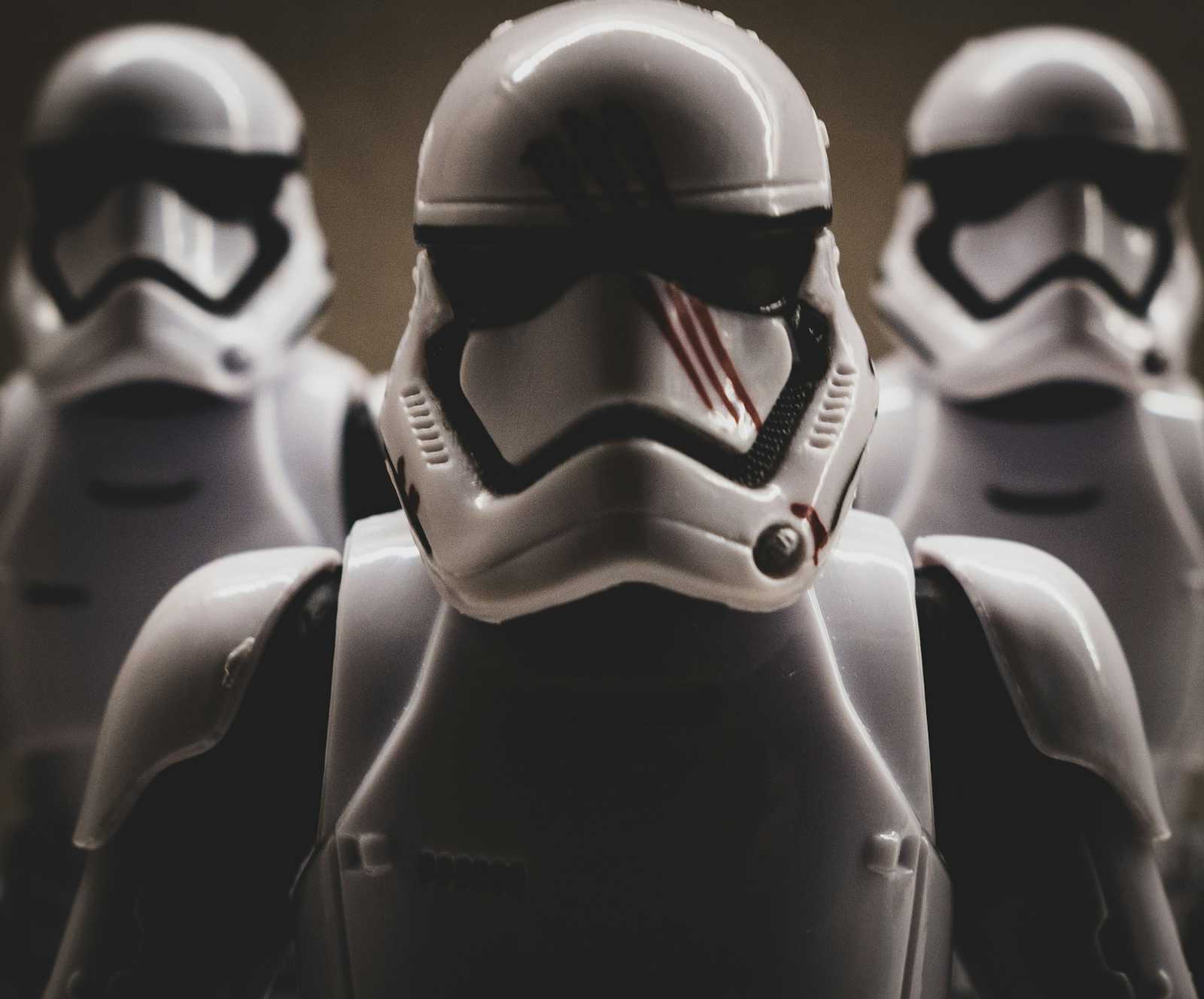
Artificial Intelligence (AI) is revolutionizing the way we work by automating tasks, improving efficiency, and boosting productivity. One powerful way to leverage AI is by using multiple AI agents that collaborate to complete complex tasks. CrewAI framework provides a structured approach to building and managing these AI-powered teams.
In this guide, we will build a cooperative AI team that simulates the workflow of professionals, increasing efficiency on repetitive or time-consuming tasks. This can be useful for designers, product managers, and business owners looking to optimize their creative processes and plannings.
What are we building?
We will define a set of AI agents working together:
Image Analysis Agent: Describes the content of an image.
Design Improvement Agent: Reviews the image description and suggests improvements.
Product Management Agent: Creates and prioritizes user stories based on the image and suggested improvements.
The AI crew will analyze and process the following image:
The result is a simulation of a typical workflow in IT industries, such as web development. It begins with analysis, suggests UX design based on the requirements, and creates user stories that are ready for development.
Let's get started!
Setting Up
Before we start implementing the AI team, we need to install CrewAI package and its dependencies. Follow these steps to set up your environment:
Step 1: Check Your Python Version
CrewAI requires Python >=3.10 and <3.13
. To check your Python version, run the following command:
python3 --version
If your Python version does not meet the requirements, you may need to upgrade or install a compatible version.
Step 2: Set Up a Virtual Environment (Optional but Recommended)
python -m venv crewai_env
source crewai_env/bin/activate # On Windows use `crewai_env\Scripts\activate`
Step 3: Install Required Packages
pip install crewai crewai_tools pillow python-dotenv
pip freeze
Step 4: Set Up Your API Key
This project requires an OpenAI account and some credits to use their models. CrewAI needs an OpenAI API key to work. Create a new API key and save it in a .env
file in your project directory.
OPENAI_API_KEY=your_openai_api_key_here
The Coding Part
Step 1: Import Modules
Create a new file main.py
and import the necessary modules:
import os
from PIL import Image
from crewai import Agent, Task, Crew, Process, LLM
from crewai_tools import VisionTool
from dotenv import load_dotenv
Step 2: Load API Key
Let's load the environment variables into the project and add a simple error check for when the OpenAI API key is missing:
load_dotenv()
api_key = os.getenv("OPENAI_API_KEY")
if api_key is None:
raise ValueError("OPENAI_API_KEY not found in .env file")
os.environ["OPENAI_API_KEY"] = api_key
Step 3: Initialize LLM, Image Path and VisionTool
For this use case, we use gpt-4o
model from OpenAI, which is efficient and relatively affordable. However, feel free to use other models if you prefer. Also, setting the temperature
to a higher value will generate more creative and diverse results.
llm = LLM(model="gpt-4o", temperature=0.8)
Download the input image from the GitHub repository or use your own image. Place it in the root directory.
# Replace 'image1.png' with the actual filename of your uploaded image
image_path = "image1.png"
image = Image.open(image_path)
Next, create an instance of Vision Tool from CrewAI, which is capable of extracting text from images.
vision_tool = VisionTool()
Step 4: Define AI Agents
As CrewAI docs say, an Agent is an autonomous unit that can perform specific tasks, make decisions based on its role and goal or collaborate with other agents. Think of an agent as a specialized team member with specific skills, expertise, and responsibilities.
Let's define our first Agent.
Agent 1: Image Analyzer
image_analyzer_agent = Agent(
role="Image Describer",
goal=f"Describe the content of the image {image_path} accurately and comprehensively.",
backstory="Expert Designer working for a B2B Restaurant Startup.",
verbose=True,
tools=[vision_tool],
llm=llm
)
You can adapt the Agent's attributes to fit your specific use case.
Next, let's define additional two agents - Designer and Product Manager.
Agent 2: Designer
designer_agent = Agent(
role="Design Improvement Suggestion Agent",
goal=f"Review image descriptions and provide actionable improvement suggestions to the described scene in {image_path}.",
backstory="You are an expert designer with a keen eye for detail, specializing in B2B restaurant design.",
verbose=True,
llm=llm,
tools=[vision_tool]
)
Agent 3: Product Manager
product_manager_agent = Agent(
role="Product Manager",
goal=f"Create user stories and prioritize them based on the image {image_path}.",
backstory="You are a product manager experienced in B2B restaurant solutions.",
verbose=True,
llm=llm,
tools=[vision_tool]
)
Step 5: Define Tasks
In CrewAI, a Task is a specific assignment completed by an Agent. In each task you define all the necessary details for execution.
We will use sequential
execution flow, in which Tasks are executed in order they are defined.
Task 1: Describe Image
task_describe_image = Task(
description="Describe the content of the image.",
expected_output="A detailed description of the image",
agent=image_analyzer_agent
)
Task 2: Suggest Design Improvements
task_suggest_improvements = Task(
description="Review the image description and suggest improvements to the image. Focus on design and visual elements.",
expected_output="A list of actionable suggestions.",
agent=designer_agent,
context=[task_describe_image]
)
Task 3: Create User Stories
task_create_user_stories = Task(
description="Create user stories based on the design improvements suggested. Prioritize these user stories based on impact and feasibility.",
expected_output="A prioritized list of user stories.",
agent=product_manager_agent,
context=[task_describe_image, task_suggest_improvements]
)
Step 6: Define and Run the AI Team
Now that we defined Agents and Tasks, we are ready to define a Crew and start the execution.
ai_team = Crew(
agents=[image_analyzer_agent, designer_agent, product_manager_agent],
tasks=[task_describe_image, task_suggest_improvements, task_create_user_stories],
process=Process.sequential,
verbose=True
)
result = ai_team.kickoff()
Step 7: Save the Output
We stored the outcome in result
variable, but for better readability, it would be helpful to write it in Markdown and save it to a new file called output.md
:
with open("output.md", "w") as md_file:
md_file.write("## Crew Execution Results in Markdown:\n")
for idx, task_output in enumerate(result.tasks_output):
md_file.write(f"Agent {idx + 1}: {task_output.agent}\n{task_output.raw}\n\n")
Step 8: Run the app & refine
Finally, it's time for executing and see the results:
python3 main.py
🚀 Voila! The result should be stored in the output.md
file, written in Markdown.
## Crew Execution Results in Markdown:
Agent 1: Image Describer
The image shows a user interface of a restaurant management system, tailored for a restaurant named "Pizza Real." The interface features a theme setup section where users can manage different elements of the restaurant's website appearance. Key components visible in the interface include:
1. **Social Media Links**: There are options to manage links to various social media platforms, including TikTok, Facebook, Instagram, and YouTube. These appear to be essential elements for connecting the restaurant's online presence with social media.
2. **Cover Logo Section**: There is a designated area for uploading the restaurant's cover logo, which is crucial for branding purposes.
3. **Background Customization**: Users can choose between setting a background color or an image for the website. The interface indicates a specific color code, #102d50, which is selected for the menu background, suggesting a preference for a particular aesthetic.
4. **Layout and Navigation**: The interface is organized with a left sidebar that likely serves as a navigation menu. The sidebar allows users to switch between different settings and sections within the management system.
Overall, the user interface is designed to provide a comprehensive set of tools for customizing and managing the visual theme of the restaurant's online presence, focusing on branding, aesthetic consistency, and social media integration.
Agent 2: Design Improvement Suggestion Agent
Based on the description of the user interface for the restaurant management system in "Pizza Real," here are some actionable design improvement suggestions:
1. **Enhance Visual Hierarchy**: Ensure that the most critical features, such as the cover logo and social media links, are prominently displayed with distinct visual separation. This could be achieved through size variation, color contrasts, or using dividers.
2. **Social Media Link Customization**: Provide customization options for social media icons, such as size, color, and shape, to align with the restaurant's brand identity, making them more visually integrated with the overall theme.
3. **Cover Logo Optimization**: Allow for different display options and sizes for the cover logo to ensure it displays optimally across different devices. Consider adding a preview function so users can see how their logo appears on the site.
4. **Background Customization Preview**: Introduce a live preview feature for background changes (color or image) to allow users to see the impact of their choices in real time. This helps in maintaining aesthetic consistency and making informed decisions.
5. **Sidebar Enhancement**: If the sidebar is used for navigation, consider making it collapsible to maximize the workspace in the main area, especially on smaller screens. Use icons alongside text for better recognition and quicker access.
6. **Color Palette and Theme Consistency**: Offer a set of pre-defined color palettes that users can choose from to maintain a cohesive theme throughout the site. Encourage the use of complementary colors to the #102d50 code to enhance visual appeal.
7. **Tooltips and Help Guides**: Integrate tooltips or a help guide to assist users in understanding different features and settings quickly. This can improve user experience, especially for those new to the system.
8. **Responsive Design Considerations**: Ensure that the user interface is fully responsive. This involves optimizing the display for various devices such as tablets and smartphones, ensuring accessibility and ease of use on any platform.
Implementing these suggestions can lead to a more intuitive and visually appealing user interface, enhancing the management capabilities and overall online presence of "Pizza Real."
Agent 3: Product Manager
Based on the design improvements suggested for the "Pizza Real" restaurant management system interface, here is a prioritized list of user stories:
1. **Responsive Design Optimization**
- As a user, I want the interface to be fully responsive so that it can be easily accessed and used on any device, ensuring accessibility and ease of use.
2. **Cover Logo Display Options**
- As a user, I want different display options and sizes for the cover logo to ensure it appears optimally across devices, and I want a preview function to see how my logo looks on the site.
3. **Sidebar Enhancement**
- As a user, I want the navigation sidebar to be collapsible to maximize workspace on smaller screens, with icons alongside text for better recognition.
4. **Social Media Icon Customization**
- As a user, I want to customize social media icons (size, color, shape) so they align with the restaurant's brand identity and integrate with the theme.
5. **Background Customization Live Preview**
- As a user, I want a live preview for background changes so I can see the impact of my color or image choices in real-time, ensuring aesthetic consistency.
6. **Enhance Visual Hierarchy**
- As a user, I want critical features like cover logo and social media links to be prominently displayed with visual separation for easy access.
7. **Color Palette Options**
- As a user, I want a set of predefined color palettes to maintain a cohesive theme, using complementary colors to the #102d50 code.
8. **Tooltips and Help Guides Integration**
- As a user, I want tooltips or a help guide to assist me in understanding different features and settings quickly, improving my user experience.
These user stories are prioritized based on their impact on user experience and feasibility of implementation. Responsive design and cover logo optimization are top priorities due to their significant impact on usability and branding. Sidebar and social media icon enhancements follow due to their role in navigation and brand alignment. Live previews and visual hierarchy improvements are also important for maintaining an intuitive and visually appealing interface. Color palette options and help guides are prioritized last, as they are enhancements to existing functionalities.
Feel free to adjust the definitions of Agents and Tasks to achieve the desired outcome.
Conclusion
By leveraging CrewAI, we built a team of AI agents that:
analyze images,
suggest design improvements,
and generate user stories.
This project demonstrates the power of multi-agent collaboration in solving real-world problems efficiently. Implementing such AI-powered workflows can significantly enhance productivity, allowing professionals to focus on strategic decision-making while AI handles the repetitive tasks.
I encourage you to experiment with different models, expand the team’s capabilities, and customize the AI agents to fit your specific needs.
Here is the complete GitHub repository for reference.
Thank you for reading!
Subscribe to my newsletter
Read articles from Miroslav Pillár directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Miroslav Pillár
Miroslav Pillár
🚀 Self-taught software developer with a passion for growth and innovation. From an economics background, I transitioned into tech—gaining experience in frontend and fullstack development across financial and healthcare industries. My journey led me to founding Bitloom, a software development company focused on building modern web and mobile solutions.