TypeScript in Cheat sheet React JS
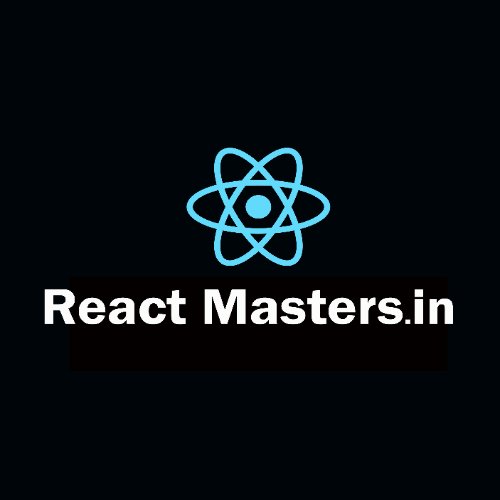
Table of Contents
Introduction to TypeScript in React
Setting Up TypeScript in a React Project
Basic TypeScript Types for React
Defining Props with TypeScript
Handling State with TypeScript
TypeScript and React Hooks
Event Handling in TypeScript
TypeScript and Ref Handling
Conclusion
1. Introduction to TypeScript in React
TypeScript is a strongly typed superset of JavaScript that enhances code reliability, maintainability, and developer experience. Using TypeScript in React ensures type safety, reducing runtime errors and improving code readability.
Why Use TypeScript in React?
Error Prevention: Detects issues at compile time.
Better Code Readability: Provides clear definitions of components and functions.
Improved Developer Experience: Offers IntelliSense and autocomplete support.
Scalability: Ideal for large applications with multiple developers.
2. Setting Up TypeScript in a React Project
New React Project with TypeScript
Run the following command to create a React project with TypeScript:
npx create-react-app my-app --template typescript
Add TypeScript to an Existing React Project
If you already have a React project, install TypeScript and required types:
npm install typescript @types/react @types/react-dom --save-dev
3. Basic TypeScript Types for React
TypeScript provides basic types that improve type safety in React applications.
Type | Description | Example |
string | Text values | let name: string = "React"; |
number | Numeric values | let age: number = 25; |
boolean | True/False values | let isActive: boolean = true; |
array | List of values | let items: string[] = ["One", "Two"]; |
object | Key-value pairs | let user: { name: string; age: number } = { name: "John", age: 30 }; |
4. Defining Props with TypeScript
Props allow passing data between components. Using TypeScript ensures type safety.
Example:
import React from "react";
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return <button onClick={onClick}>{label}</button>;
};
export default Button;
✅ This approach ensures that label
is a string and onClick
is a function.
5. Handling State with TypeScript
Use useState with TypeScript to define the expected state type.
Example:
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState<number>(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
};
✅ The <number>
ensures that count
only stores numbers.
6. TypeScript and React Hooks
Hooks can also be typed for better code safety.
useEffect Example:
import { useEffect } from "react";
const FetchData = () => {
useEffect(() => {
console.log("Component mounted");
}, []);
return <div>Data loaded</div>;
};
useRef Example:
import { useRef } from "react";
const InputFocus = () => {
const inputRef = useRef<HTMLInputElement>(null);
const handleClick = () => {
if (inputRef.current) {
inputRef.current.focus();
}
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleClick}>Focus Input</button>
</div>
);
};
✅ The <HTMLInputElement>
ensures that useRef
is properly typed.
7. Event Handling in TypeScript
Define event types explicitly to prevent errors.
Handling Button Click Event
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
console.log("Button clicked!");
};
Handling Input Change Event
const handleChange = (event: React.ChangeEvent<HTMLInputElement>) => {
console.log(event.target.value);
};
✅ This ensures event handlers receive the correct event type.
8. TypeScript and Ref Handling
Refs allow accessing DOM elements directly.
Example:
import { useRef } from "react";
const VideoPlayer = () => {
const videoRef = useRef<HTMLVideoElement>(null);
const playVideo = () => {
if (videoRef.current) {
videoRef.current.play();
}
};
return (
<div>
<video ref={videoRef} src="video.mp4" />
<button onClick={playVideo}>Play</button>
</div>
);
};
✅ This ensures videoRef
correctly references a video element.
9. Conclusion
Using TypeScript with React improves code quality, maintainability, and error handling. By following this cheat sheet, developers can write safer, scalable, and more efficient React applications.
Key Takeaways:
✅ Use TypeScript types to define props and state.
✅ Leverage TypeScript with hooks like useState
, useEffect
, and useRef
.
✅ Type event handlers properly to prevent runtime errors.
✅ TypeScript enhances readability and makes debugging easier.
Start using TypeScript in React today to write better, more reliable applications! 🚀
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
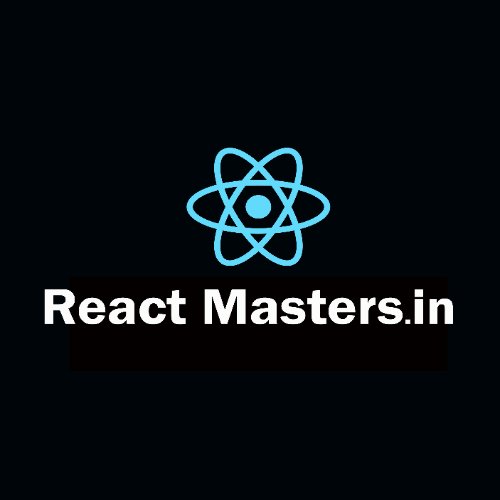
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.