Implementing Unit Testing

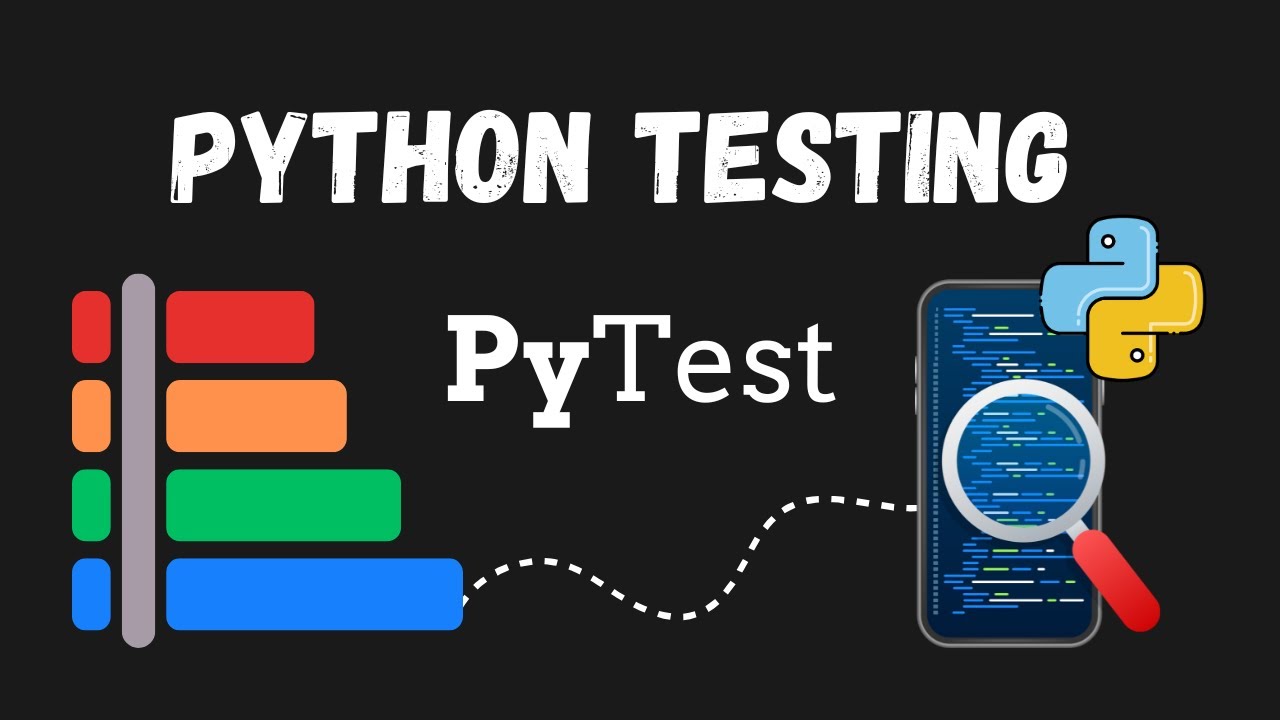
Introduction:
In the world of software development, ensuring code quality is paramount. While various testing methods exist, unit testing stands out as a fundamental practice. Today, we'll dive into the 'why' and 'how' of unit testing, providing a practical guide for intermediate developers.
What is Unit Testing?
"At its core, unit testing involves testing individual components or 'units' of your code in isolation. These units are typically functions or methods. The goal is to verify that each unit behaves as expected, reducing the likelihood of bugs and regressions."
Why Unit Testing Matters:
Early Bug Detection: "Identifying errors early in the development cycle saves time and resources."
Code Confidence: "Well-tested code instills confidence during refactoring and maintenance."
Improved Code Design: "Writing testable code often leads to cleaner and more modular designs."
Documentation: "Unit tests can serve as living documentation, showcasing how code should function."
Faster Debugging: "When a bug arises, unit tests help pinpoint the source of the problem."
Practical Implementation:
"Let's illustrate unit testing with a simple example using Python and the unittest
framework."
Python
import unittest
def add(x, y):
return x + y
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-2, -3), -5)
def test_add_mixed_numbers(self):
self.assertEqual(add(2, -3), -1)
if __name__ == '__main__':
unittest.main()
"In this example, we define a simple add
function and create a TestAddFunction
class that inherits from unittest.TestCase
. Each test_
method represents a specific test case. The assertEqual
method is used to verify the expected output."
Best Practices:
Test-Driven Development (TDD): "Consider writing tests before writing the actual code."
Test Coverage: "Aim for high test coverage, but prioritize testing critical code paths."
Keep Tests Isolated: "Ensure tests don't depend on external resources or databases."
Write Clear and Concise Tests: "Tests should be easy to understand and maintain."
Automate Tests: "Integrate unit tests into your CI/CD pipeline."
Conclusion:
"Unit testing is an indispensable practice for building robust and reliable software. By embracing unit testing, you can enhance code quality, boost confidence, and streamline the development process. Start implementing unit tests in your projects today, and experience the benefits firsthand."
Subscribe to my newsletter
Read articles from Abdullahi Tahliil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
