Resolving cyclical dependency in Spring
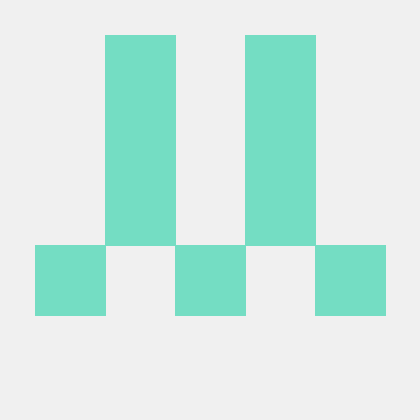
In this article we will go over a few ways we can handle cyclical dependencies in Spring.
Introduction
First, let’s go over what cyclical dependencies are. Cyclical dependencies occur when a dependency of a bean is either directly or indirectly dependent on it. In the example below, bean A has a property which references bean B, but bean B also has a property which references bean A. This would result in neither beans being able to get instantiated and an error would be thrown on startup.
To handle this, we can do this through several strategies.
Strategy 1 : Set the property of one bean later.
Set property programmatically
This can be done through separately setting the property in a class. Say for example we can remove the property setting of beanA in beanB from the XML. Then in a suitable class - eg. the main method we can separately set beanA in beanB.
Use @Lazy annotation
If we would like to keep the property setting in the Spring contexts, we can also add the `@Lazy` annotation to the setter method. That would allow our original spring xml configuration to be resolved.
class A {
private B b;
// If B is a constructor argument
public A(@Lazy B b) {
this.b = b;
}
public A() { }
// If B is set through a setter method
@Lazy
public void setB(B b) {
this.b = b;
}
}
The annotation tells Spring to inject a proxy of B
instead of the actual instance, and B
itself will not be instantiated until it is actually used (i.e. when any method is called on b through — eg. b.someMethod()
)
Strategy 2: Introduce a mediator class
This mediator class will contain references to class A
and class B
so that the two don’t have to depend on each other.
For example, a class C
could be the mediator class.
class C {
private final A a;
private final B b;
public C(A a, B b) {
this.a = a;
this.b = b;
}
public void someMethod() {
// C can act as a bridge between A and B
}
}
And that’s it for resolving Spring cyclical dependencies :)
Subscribe to my newsletter
Read articles from Software Engineering Blog directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
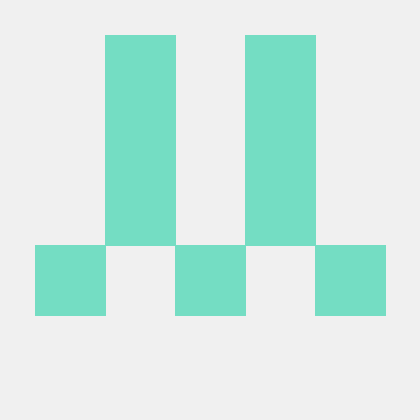