03. Shebang

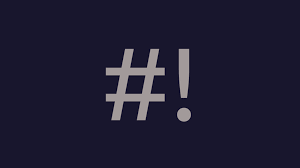
What is Shebang?
We often use many shells in Linux (such as bourne shell, bourne again shell, corn shell, Z shell, and C shell). There are many things common in most of the shells, although there are some fundamental differences between all of them.
We commonly develop a bash script for scripting or automation purposes and it is developed by keeping in mind the executable in the bourne again shell or bash shell.
It is possible, that we run a bash script in any of the other shells and that does not support the syntax/functionalities. e.g.,
for mission in {0..10}; do
echo $mission
done
The above example will work fine in the bash shell but not in the dash shell (Debian Almquist Shell).
To get rid of this problem, we use Shebang to specify and run a script through that shell mentioned in the Shebang.
#!/bin/bash
for mission in {0..10}; do
echo $mission
done
Exit codes
When a command is run, it either runs successfully or fails. If a command runs successfully, it returns an exit code/status of Zero (0). Similarly, when a command runs unsuccessfully/failed (which can happen if we try to execute a command that does not exist), it returns an exit code greater than 0 or it can be 1.
- We can check the status code of a command using
echo $?
write after the command is run.
if [ "$rocket_status" = "failed" ]
then
rocket-debug "$mission_name"
exit 1
fi
exit 1
: This command exits the script with a status code of 1, which typically indicates an error or failure.
Functions
The function is a block of code implemented to perform a particular task by maintaining a set of instructions. The purpose is to reuse the code without changing the script.
To run the function we need to call the function within the script
Additionally, we can pass function parameter/argument during the function call
Suppose, we defined a single function in a script, then we will get two parts of the script
One is the function definition
Another is the main part of the script that calls the function
A function must be defined at the top of the script (The function needs to be defined before calling it). Because in shell script it executes from the top of the script and works its way to the bottom.
#!/bin/bash
function check_even() {
if (( $1 % 2 == 0 )); then
return 0 # Return 0 for even numbers
else
return 1 # Return 1 for odd numbers
fi
}
number=4
check_even $number
if [ $? -eq 0 ]; then
echo "$number is even."
else
echo "$number is odd."
fi
Instead of using exit status in the function, we use the return statement
- The return statement within a function call helps in specifying the exit code for that function. Using the return statement, the script will not exit, It will only exit the function.
When to use the function?
To break up a large script that performs many different tasks:
Installing packages
Adding users
Configuring firewalls
Perform Mathematical calculations
#!/bin/bash
function add() {
sum=$(( $1 + $2 ))
echo $sum
}
result=$( add 3 5 )
echo "The sum is: $result"
The function must echo the result so that it can be captured in the result
variable.
function add(){
return $(($1 + $2))
}
add 3 5
sum=$?
The return
statement in shell scripting is used to return an exit status, which is an integer between 0 and 255. It is not used to return values like the result of an arithmetic operation.
In the script, return $(($1 + $2))
will attempt to return the sum as an exit status, which is not the intended use if the sum exceeds 255.
Tips and tricks for editor
For CLI based (Use VIM)
apt-get install shellcheck
oryum install shellcheck
install based on the OS. After installing runshellcheck menu.sh
» It would go through the utility and possible bugs, and will provide possible recommendation for improvement.
For GUI based
- Pycharm, Visual Studio Code, Atom
Resource
Subscribe to my newsletter
Read articles from Arindam Baidya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
