01. Shell Script Introduction

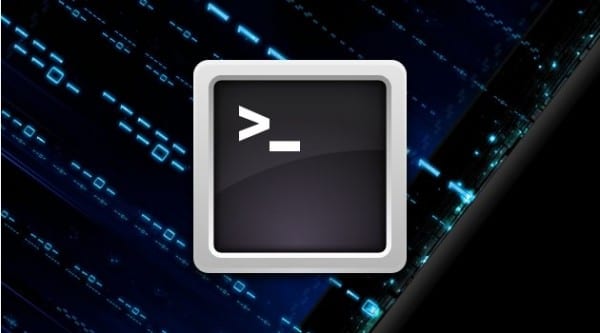
» Before starting to develop the script, it is important to have a clear idea of what we are developing it for. We must write down the exact steps and associated commands required to complete the task.
» Automating a set of processes and making the task easy can be done using shell scripting.
» It is important to name the shell script file in a way that makes sense. This will allow users using the script in future, to know exactly what the script does.
» We make the script file with extension .sh to indicate that it is a shell script.
» Need to execute the script using bash
command.
» During execution, the script file runs/executes each line one after another until all lines are complete. If one line takes a long time to execute, it will wait until it’s finished before proceeding to the next line.
There are different ways to run a shell script file
Using
bash
- We can configure our script file to run it as a command. (If we do this, it is recommended to not make the script file with .sh extension)
» To make a script run as a command, need to add the location of the script file path into the PATH variable. export PATH=$PATH:<script-file-path>
which <command>
To see the location of a command.
» Running a shell script file for the first time can encounter with the Permission denied error, if executable permission are not been set correctly.
ls -l
To check executable permission has been set or not.
chmod +x <file-name>
Making the file executable for all user, groups, and others.
Variables
» A variable is a value that can change or vary.
» To differentiate a static value from a variable —» A variable, when it is used, always needs to add ‘$’ sign before the variable.
Note: When we are setting/initializing a value for a variable, we do not use ‘$’ sign before the variable name.
» A variable name only contains alphanumeric or underscore.
» Varibale name is case-sensetive.
rocket_status=$(rocket-status $mission_name)
Storing the value of a command into a variable.
echo "Status of launch: $rocket_status"
Printing the status/value of a variable. —» We can use a variable within a string to show the value as part of the string (““).
cp ${file_name} ${file_name}_bkp
» Variable should be encapsulated within {}.
Command Line Arguments
Command Line Arguments in shell scripting are parameters that are passed to the script when it is executed. These arguments allow users to provide input to the script from the command line, making the script more dynamic and flexible. In a shell script, command line arguments are accessed using special variables:
$0
refers to the name of the script itself.$1
,$2
,$3
, etc., refer to the first, second, third, and subsequent arguments, respectively.$#
gives the total number of arguments passed to the script.$@
and$*
represent all the arguments passed to the script. However, they behave slightly differently when enclosed in double quotes.
These arguments can be used within the script to perform operations based on the input provided by the user.
Script Content:
echo "Capital city of $1 is $2"
./print-capital.sh Nigeria Abuja
Capital city of Nigeria is Abuja.
Note:
Design script to be reusable
Script should not required to be edited before running
Use command line arguments to pass inputs
Read Inputs
When we want to prompt the user to type the required data during the execution. This is typically done using the read
command, which allows the script to pause and wait for the user to type input. The input provided by the user can then be stored in a variable for further processing within the script.
echo "Enter your name:"
read user_name
echo "Hello, $user_name!"
Or, we can do it in a single line, as shown below.
read -p "Enter your name: " user_name
Q. What to use between command line argument and read input?
Use command line arguments for:
Automation
Non-interactive execution
Use the
read
command for:Interactive user input
Execution requiring user interaction
Consider a combined approach for:
Providing default values via the command line
Allowing interactive input when needed
Flexibility in handling both automated and interactive scenarios
Arithmetic Operation
In the shell script arithmetic operation can be performed in various way.
expr
$ expr 5 + 6
$ expr 5 - 6
$ expr 5 / 6
$ expr 5 \* 6
baskets=4
apples_per_basket=5
total_apples=`expr $baskets \* $apples_per_basket`
echo "Total Apples = $total_apples"
Note:
When using expr for performing arithmetic operations, need to use a space between the operator and operands
During multiplication using expr, we need to use a backslash (\) with aesthetic (*), because the aesthetic is a revered rejected character in shell, which typically means everything.
We can also use variable substitution as well (Can use variable instead of the numbers)
$ A=5
; $ B=6
$ expr $A + $B
$ expr $A - $B
$ expr $A / $B
$ expr $A \* $B
Double parentheses
Encapsulating the expressions inside a double parentheses prefixed with a dollar symbol.
$ echo $((A+B))
$ echo $((A-B))
$ echo $((A/B))
$ echo $((A*B))
We must either assign this operation to a variable or use echo to print them to the screen. Otherwise shell will try to execute the output of this operation and throw an error.
Within the parenteses we don’t need to use $ symbol to prefixing a variable
No longer need to separate operator and operand with a space
For multiplication no need to use backslash (\) with aesthetic
We can also use increment or decrement operators (also pre and post-fix)
$ echo $((++A))
$ echo $((--A))
$ echo $((A++))
$ echo $((A--))
Note: Both expr and double parentheses return decimal numbers, they do not support floating-point value.
To get a floating point number, we need to use bc (Basic calculation)
in linux with -l
for floating utility
e.g., $ echo $A / $B | bc -l
num1=$1
num2=$2
num3=$3
sum=$(( num1 + num2 + num3 ))
average=$(echo "$sum / 3" | bc -l)
echo $average
References
Subscribe to my newsletter
Read articles from Arindam Baidya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
