Understanding Circular Import Issues with Marshmallow in Flask
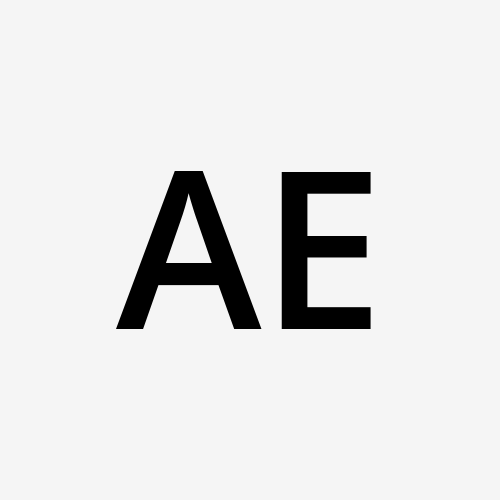
When developing a Flask application with SQLAlchemy and Marshmallow, you may encounter an issue known as circular imports. This happens when two or more modules depend on each other, creating a loop that prevents the application from properly loading. It's especially common when defining models and schemas in the same file or across multiple files.
The Circular Import Problem
In Flask, you typically define your models and schemas in separate modules or within the same file. For example, when using Marshmallow for serialization, you often define schemas that map to the corresponding models. These schemas are then used to serialize and deserialize data to and from JSON. However, when models reference each other and schemas also reference those models (either directly or indirectly), Flask might struggle to import everything correctly due to the circular dependencies between the files.
For instance, in the following case:
User model has a relationship with Plant.
Plant model has a relationship with Category.
CareNote model has a relationship with Plant.
Schemas for these models are defined and use
fields.Nested()
to include other schemas, leading to a circular import as Marshmallow schemas rely on the models to build relationships.
Flask might fail to load these modules, causing errors like:
lessCopy codesqlalchemy.exc.InvalidRequestError: When initializing mapper Mapper[User(users)], expression 'Plant' failed to locate a name ('Plant')...
Solving the Issue by Reordering Code
A simple yet effective solution to this circular import problem is to move the schema definitions to the end of the file. By doing so, you ensure that all models are loaded before their corresponding schemas attempt to use them. This allows Marshmallow schemas to reference models without causing circular dependency issues.
Here’s how this works:
Models First: Place all your SQLAlchemy model definitions at the top of the file.
Schemas After Models: Move the schema definitions (like
UserSchema
,PlantSchema
, etc.) to the end of the file. This ensures the models are fully defined before the schemas attempt to import them.
Why Does This Work?
When Python loads a file, it executes top-down. By placing models at the top, you ensure that the classes referenced by the schemas are already loaded into memory by the time they are needed. This solves the circular import issue because schemas now rely on fully defined models.
Conclusion
Circular imports can be a tricky issue when using Flask, SQLAlchemy, and Marshmallow. By restructuring your code—placing model definitions first and schemas at the end—you can avoid this issue and keep your application running smoothly.
Subscribe to my newsletter
Read articles from Amene Esnaashari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
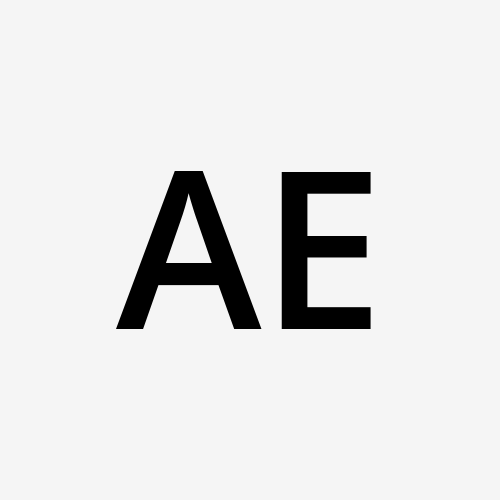
Amene Esnaashari
Amene Esnaashari
From studying the intricacies of the human mind to crafting the logic behind applications, Amene Esnaashari is on a journey of intellectual exploration. Having earned a Master's degree in Psychology from the University of Phoenix (3.7 GPA), she's now pursuing her passion for technology at Flatiron School's software engineering program. Her current focus includes JavaScript, React, Python, and Flask, as she builds the foundation for a career bridging psychology and software development.