Essential Notes on JavaScript DOM Manipulation

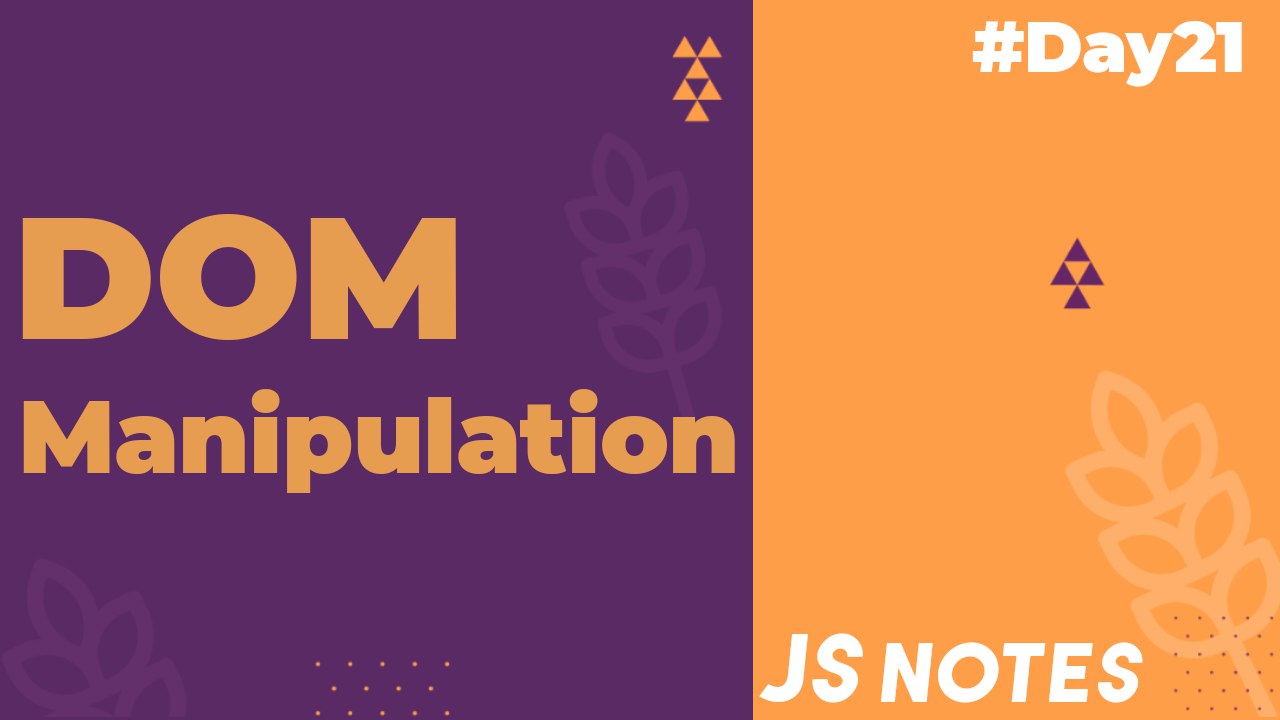
Creating Nodes
1. Create an Element
To create an HTML element dynamically in JavaScript:
const element = document.createElement('li');
element.innerHTML = 'List Item';
2. Create a Text Node
A text node can be created separately and appended to an element:
const textNode = document.createTextNode('Hridoy Chowdhury');
const parent = document.getElementById('root');
parent.append(textNode);
3. Create an Attribute Node
Attributes can be dynamically created and assigned to elements:
const attribute = document.createAttribute('class');
attribute.value = 'firstClass';
const element = document.querySelector('li');
element.setAttributeNode(attribute);
Accessing Attributes
1. getAttribute()
Retrieves the value of a specified attribute:
const element = document.getElementById('root');
console.log(element.getAttribute('id'));
2. setAttribute()
Sets the value of a specified attribute:
element.setAttribute('id', 'root1');
console.log(element.getAttribute('id'));
3. removeAttribute()
Removes a specified attribute from an element:
element.removeAttribute('id');
console.log(element.getAttribute('id')); // null
Adding Nodes to the DOM
1. appendChild(node)
Adds a node as the last child of a parent:
const parent = document.getElementById('root');
const element = document.createElement('li');
element.innerHTML = 'Item';
parent.appendChild(element);
2. append(node1, node2, ...)
Adds multiple nodes as the last child of a parent:
const parent = document.getElementById('root');
const element1 = document.createElement('li');
const element2 = document.createElement('li');
element1.innerHTML = 'Item 1';
element2.innerHTML = 'Item 2';
parent.append(element1, element2);
Difference between append()
and appendChild()
Feature | append() | appendChild() |
Content Type | Accepts nodes & strings | Accepts only nodes |
Multiple Arguments | Can append multiple elements or text at once | Can append only one node at a time |
Return Value | No return value | Returns the appended node |
Usage | parent.append(node, "text") | parent.appendChild(node) |
Example:
function attach(content){
const element = document.createElement('li');
element.innerHTML = content;
const element2 = document.createElement('li');
element2.innerHTML = content + ' V2.0';
const parent = document.getElementById('root');
// parent.appendChild(element,element2); // ❌ Invalid
// parent.appendChild(element); // ✅ Valid
parent.append(element,element2);
}
attach('TS')
attach('NODE')
3. insertBefore(newNode, referenceNode)
Inserts a node before a specified reference node:
const newElement = document.createElement('li');
newElement.innerHTML = 'Inserted Item';
const referenceNode = parent.children[1];
parent.insertBefore(newElement, referenceNode);
4. prepend(node)
Adds a node as the first child of a parent:
const child = document.createElement('li');
child.innerHTML = 'First Item';
parent.prepend(child);
5. replaceChild(newNode, oldNode)
Replaces an existing node with a new one:
const newChild = document.createElement('li');
newChild.innerHTML = 'MongoDB';
const oldChild = parent.children[2];
parent.replaceChild(newChild, oldChild);
6. Using innerHTML
Directly sets the HTML content of an element:
parent.innerHTML = '<li>New Content</li>';
7. Using insertAdjacentElement()
Inserts HTML at different positions relative to an element:
const newElement = document.createElement('div');
newElement.innerHTML = 'Hello!';
parent.insertAdjacentElement('beforebegin', newElement); // Before the parent element
parent.insertAdjacentElement('afterbegin', newElement); // Inside, before first child
parent.insertAdjacentElement('beforeend', newElement); // Inside, after last child
parent.insertAdjacentElement('afterend', newElement); // After the parent element
Valid Position Values:
Position | Meaning |
"beforebegin" | Inserts before the element itself |
"afterbegin" | Inserts inside the element, before its first child |
"beforeend" | Inserts inside the element, after its last child |
"afterend" | Inserts after the element itself |
8. Using insertAdjacentHTML()
Inserts HTML code at a specific position relative to an existing element in the DOM.
Syntax:
element.insertAdjacentHTML(position, text);
element
: The target DOM element.position
: A string indicating where to insert the HTML.text
: The HTML string to insert.
Example Usage:
const div = document.getElementById("container");
// Insert a new paragraph before the div
div.insertAdjacentHTML("beforebegin", "<p>Before the div</p>");
// Insert a new paragraph inside the div at the beginning
div.insertAdjacentHTML("afterbegin", "<p>Inside the div (start)</p>");
// Insert a new paragraph inside the div at the end
div.insertAdjacentHTML("beforeend", "<p>Inside the div (end)</p>");
// Insert a new paragraph after the div
div.insertAdjacentHTML("afterend", "<p>After the div</p>");
Difference between insertAdjacentHTML()
and insertAdjacentElement()
Feature | insertAdjacentHTML() | insertAdjacentElement() |
Input Type | Accepts an HTML string | Accepts a DOM element (Node) |
Usage | Inserts raw HTML as a string and parses it into elements | Inserts an actual existing element |
Security | Less secure (can introduce XSS if using user input) | More secure (only allows safe DOM elements) |
Multiple Inserts? | Yes, it can insert multiple elements at once | No, it inserts only a single element |
Returns | No return value | Returns the inserted element |
9. removeChild(node)
Removes a specified child node from a parent:
const childToRemove = document.querySelector('li');
parent.removeChild(childToRemove);
10. .remove()
Method
Removes an element from the DOM:
const element = document.querySelector('li');
element.remove();
Summary
Creating Nodes
Feature | Description | Example |
Create an Element | Dynamically creates an HTML element | document.createElement('li'); |
Create a Text Node | Creates a text node separately | document.createTextNode('Hridoy Chowdhury'); |
Create an Attribute Node | Dynamically creates an attribute and assigns it | document.createAttribute('class'); |
Accessing Attributes
Feature | Description | Example |
getAttribute() | Retrieves the value of a specified attribute | element.getAttribute('id'); |
setAttribute() | Sets the value of a specified attribute | element.setAttribute('id', 'root1'); |
removeAttribute() | Removes a specified attribute | element.removeAttribute('id'); |
Adding Nodes to the DOM
Feature | Description | Example |
appendChild(node) | Adds a node as the last child of a parent | parent.appendChild(element); |
append(node1, node2, ...) | Adds multiple nodes as the last child | parent.append(element1, element2); |
insertBefore(newNode, referenceNode) | Inserts a node before a specified reference node | parent.insertBefore(newElement, referenceNode); |
prepend(node) | Adds a node as the first child | parent.prepend(child); |
replaceChild(newNode, oldNode) | Replaces an existing node | parent.replaceChild(newChild, oldChild); |
Using innerHTML | Directly sets the HTML content of an element | parent.innerHTML = '<li>New Content</li>'; |
insertAdjacentElement() | Inserts an element at a specified position | parent.insertAdjacentElement('beforebegin', newElement); |
removeChild(node) | Removes a specified child node | parent.removeChild(childToRemove); |
remove() Method | Removes an element from the DOM | element.remove(); |
Subscribe to my newsletter
Read articles from Hridoy Chowdhury directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hridoy Chowdhury
Hridoy Chowdhury
As a sophomore pursuing a degree in Computer Science and Engineering, I am passionate about coding, problem-solving, and continuous learning. My academic journey has equipped me with a solid foundation in programming, particularly in C and C++, which I use to tackle complex problems and build efficient solutions. I am an avid LeetCode and Geeksforgeeks enthusiast, actively practicing and honing my skills in data structures and algorithms. This dedication not only helps me prepare for technical interviews but also keeps me engaged with the latest challenges in the field. Currently, I am expanding my technical repertoire by learning Web development. I believe in the power of versatile skill sets, and I am committed to broadening my knowledge and expertise in various programming languages and development tools. I am enthusiastic about collaborating on innovative projects and will contribute to open-source communities, and continually pushing the boundaries of what I can achieve in the realm of computer science.